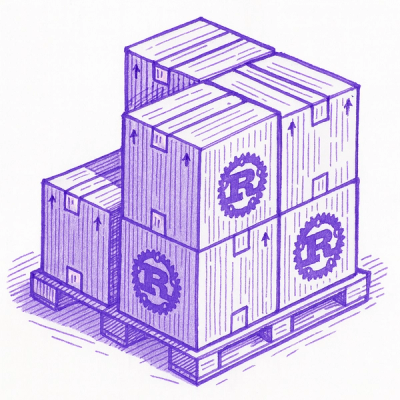
Security News
Crates.io Implements Trusted Publishing Support
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
@redis/graph
Advanced tools
@redis/graph is an npm package that provides a client for interacting with RedisGraph, a graph database module for Redis. It allows you to create, query, and manage graph data structures within a Redis database.
Creating Nodes and Relationships
This feature allows you to create nodes and relationships in a graph. The code sample demonstrates how to create a person node, a city node, and a relationship between them.
const { createClient } = require('@redis/graph');
async function createGraph() {
const client = createClient();
await client.connect();
const query = `
CREATE (p:Person {name: 'John Doe', age: 30})
CREATE (c:City {name: 'New York'})
CREATE (p)-[:LIVES_IN]->(c)
`;
await client.query('myGraph', query);
await client.disconnect();
}
createGraph();
Querying the Graph
This feature allows you to query the graph to retrieve data. The code sample demonstrates how to match a person node and a city node connected by a relationship and return their names.
const { createClient } = require('@redis/graph');
async function queryGraph() {
const client = createClient();
await client.connect();
const query = `
MATCH (p:Person)-[:LIVES_IN]->(c:City)
RETURN p.name, c.name
`;
const result = await client.query('myGraph', query);
console.log(result);
await client.disconnect();
}
queryGraph();
Updating Nodes and Relationships
This feature allows you to update properties of nodes and relationships in the graph. The code sample demonstrates how to update the age property of a person node.
const { createClient } = require('@redis/graph');
async function updateGraph() {
const client = createClient();
await client.connect();
const query = `
MATCH (p:Person {name: 'John Doe'})
SET p.age = 31
`;
await client.query('myGraph', query);
await client.disconnect();
}
updateGraph();
Deleting Nodes and Relationships
This feature allows you to delete nodes and relationships from the graph. The code sample demonstrates how to delete a person node and all its relationships.
const { createClient } = require('@redis/graph');
async function deleteGraph() {
const client = createClient();
await client.connect();
const query = `
MATCH (p:Person {name: 'John Doe'})
DETACH DELETE p
`;
await client.query('myGraph', query);
await client.disconnect();
}
deleteGraph();
The neo4j-driver package is the official driver for connecting to Neo4j, a popular graph database. It provides similar functionalities for creating, querying, updating, and deleting graph data. Compared to @redis/graph, neo4j-driver is specifically designed for Neo4j and offers more advanced features and optimizations for that database.
The gremlin package is a JavaScript client for Apache TinkerPop, a graph computing framework that works with various graph databases like JanusGraph and TinkerGraph. It provides a flexible and powerful way to interact with graph databases using the Gremlin query language. Compared to @redis/graph, gremlin supports a wider range of graph databases and offers more complex querying capabilities.
The arangodb package is a client for ArangoDB, a multi-model database that supports graph, document, and key-value data models. It allows you to perform graph operations such as creating nodes and edges, querying, and managing graph data. Compared to @redis/graph, arangodb offers a more versatile database solution with support for multiple data models.
FAQs
Example usage: ```javascript import { createClient, Graph } from 'redis';
The npm package @redis/graph receives a total of 2,783,314 weekly downloads. As such, @redis/graph popularity was classified as popular.
We found that @redis/graph demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.