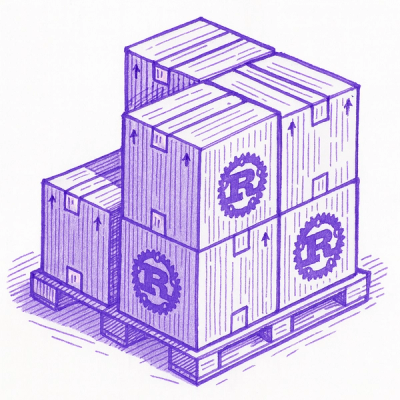
Security News
Crates.io Implements Trusted Publishing Support
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
@salesforce/command
Advanced tools
This is the base command class that plugin command authors will extend for convenient access to common SFDX flags, a logger, CLI output formatting, scratch orgs, and devhubs. It extends @oclif/command and is available within a plugin generated by the Salesforce Plugin Generator.
As a beta feature, the Salesforce command module is a preview and isn’t part of the “Services” under your master subscription agreement with Salesforce. Use this feature at your sole discretion, and make your purchase decisions only on the basis of generally available products and features. Salesforce doesn’t guarantee general availability of this feature within any particular time frame or at all, and we can discontinue it at any time. This feature is for evaluation purposes only, not for production use. It’s offered as is and isn’t supported, and Salesforce has no liability for any harm or damage arising out of or in connection with it. All restrictions, Salesforce reservation of rights, obligations concerning the Services, and terms for related Non-Salesforce Applications and Content apply equally to your use of this feature. You can provide feedback and suggestions for the Salesforce command module in the issues section of this repo.
$ sfdx --version
Commands that extend SfdxCommand can only be used with SFDX CLI version 6.8.2 or later.
There are many features that can be enabled with SfdxCommand subclasses simply by setting static properties on the subclassed command. Other features will always be available for your command such as a logger and output renderer.
--json
and --loglevel
flags automatically added to their command. Other SFDX Flags are enabled either by setting static properties directly on the command or within the flagsConfig static property.SfdxError
is not thrown then the error handler wraps it in an SfdxError for consistent error display.true
to add the --targetusername (-u)
and --apiversion
flags to this command, and have the org added (if provided) as an instance property which you can access in your command as this.org
.static supportsUsername = true;
true
to add the --targetusername (-u)
and --apiversion
flags to this command and require that a targetusername is set, either via the flag or by having a default set in your SFDX config.static requiresUsername = true;
true
to add the --targetdevhubusername (-v)
and --apiversion
flags to this command, and have the dev hub org added (if provided) as an instance property which you can access in your command as this.hubOrg
.static supportsDevhubUsername = true;
true
to add the --targetdevhubusername (-v)
and --apiversion
flags to this command and require that a targetdevhubusername is set, either via the flag or by having a default set in your SFDX config.static requiresDevhubUsername = true;
true
if this command must be run within a SFDX project, and have the project object added as an instance property which you can access in your command as this.project
.static requiresProject = true;
static flagsConfig = {
name: flags.string({char: 'n', description: messages.getMessage('nameFlagDescription')}),
force: flags.boolean({char: 'f'})
};
static tableColumnData = ['id', 'name', 'description'];
static result = {
tableColumnData: [
{key: 'id', label: 'ID'},
{key: 'name', label: 'Name'},
{key: 'description', label: 'Description'}
],
display(): {
if (Array.isArray(this.data) && this.data.length) {
if (this.data.length > 100) {
// special display for large number of results
} else {
this.ux.table(this.data, this.tableColumnData);
}
}
}
};
--targetusername (-u)
flag or a default defined in the SFDX config.--targetdevhubusername (-v)
flag or a default defined in the SFDX config.--json
flag so that output is supressed when set.run
method has completed.There are many SFDX flags and flag types made available by extending SfdxCommand. As mentioned above, some of these flags are added when static properties are set on your command such as supportsUsername, some are added necessarily such as --json
and --loglevel
, and some can be added or removed from your command via the flagsConfig static property. You can also override the flags implementation completely by defining your own static flags
property. See the oclif flags docs for details.
this.ux.*
methods and formats output as JSON. A boolean flag that is necessarily on all SfdxCommands.--myflag=first,second,third
--myflag=01-02-2000
--myflag=01/02/2000 01:02:34
--myflag=/my/path/to
--myflag=me@my.org
--myflag=/my/path/to/myfile.json
--myflag=00Dxxxxxxxxxxxx
--myflag=42
--myflag=01:02:03
--myflag=http://www.salesforce.com
static flagsConfig = {
names: {name: 'names', char: 'n', required: true, type: 'array'}, // use an SFDX flag type
force: flags.boolean({char: 'f'}), // use an oclif flag builder
verbose: true, // enable the SFDX verbose flag
apiversion: false, // disable the SFDX apiversion flag
targetusername: {required: true} // override default targetusername behavior by making it required
};
by specifying that your command supports a username your command will automatically have the --targetusername (-u)
and apiversion
flags added. The --json
and --loglevel
flags are also added by default.
SfdxCommand handles runtime errors in the catch
method for consistent error handling format and behavior. By default, the exit code will be 1
unless otherwise specified within the SfdxError. Stack traces are supressed unless the SFDX_ENV
environment variable is set to "development". Override the catch
method if you'd prefer to handle errors within your command.
FAQs
Salesforce CLI base command class
The npm package @salesforce/command receives a total of 66,854 weekly downloads. As such, @salesforce/command popularity was classified as popular.
We found that @salesforce/command demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 54 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.