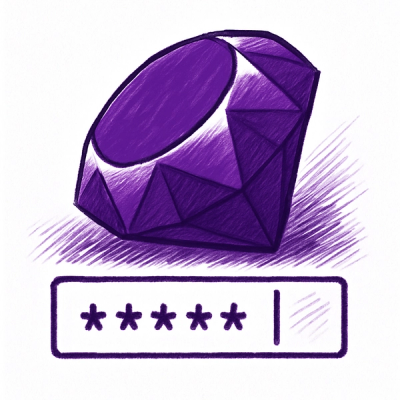
Research
/Security News
60 Malicious Ruby Gems Used in Targeted Credential Theft Campaign
A RubyGems malware campaign used 60 malicious packages posing as automation tools to steal credentials from social media and marketing tool users.
@stevenkellner/typescript-common-functionality
Advanced tools
Common Typescript functionality that I use regularly
This repository contains common Typescript functionality that I use regularly. It includes various utilities, types, and classes to facilitate development in Typescript.
To install the package, use npm:
npm install @stevenkellner/typescript-common-functionality
Import the necessary modules and use them in your TypeScript project:
import { UtcDate, Result, Logger } from '@stevenkellner/typescript-common-functionality';
// Example usage
const date = UtcDate.now;
const result = Result.success('Operation successful');
const logger = new Logger();
logger.info('ExampleFunction', 'This is an info message');
Contributions are welcome! Please open an issue or submit a pull request on GitHub.
On each push and pull request, the CI runs the build, test and lint script to verify the package.
The coverage report is generated automatically by the CI on each push and pull request. You can view the latest coverage report here.
The library is published automatically as a npm package and a github release is created by the CI on each new release tag. The npm package can be found here, you can install it with npm install @stevenkellner/typescript-common-functionality
.
This project is licensed under the MIT License. See the LICENSE file for details.
FAQs
Common Typescript functionality that I use regularly
The npm package @stevenkellner/typescript-common-functionality receives a total of 6 weekly downloads. As such, @stevenkellner/typescript-common-functionality popularity was classified as not popular.
We found that @stevenkellner/typescript-common-functionality demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A RubyGems malware campaign used 60 malicious packages posing as automation tools to steal credentials from social media and marketing tool users.
Security News
The CNA Scorecard ranks CVE issuers by data completeness, revealing major gaps in patch info and software identifiers across thousands of vulnerabilities.
Research
/Security News
Two npm packages masquerading as WhatsApp developer libraries include a kill switch that deletes all files if the phone number isn’t whitelisted.