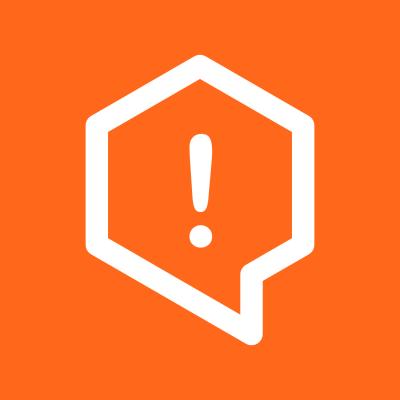
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
@storybook/addon-ondevice-actions
Advanced tools
@storybook/addon-ondevice-actions is an addon for Storybook that allows you to log actions and events directly on your device. This is particularly useful for mobile and tablet development, where you want to see the interactions and events happening in real-time on the actual device.
Logging Actions
This feature allows you to log actions such as button presses. The `action` function from `@storybook/addon-ondevice-actions` is used to log the event, which can then be viewed in the Storybook UI.
import { storiesOf } from '@storybook/react-native';
import { action } from '@storybook/addon-ondevice-actions';
import { Button } from 'react-native';
storiesOf('Button', module)
.add('with text', () => (
<Button
onPress={action('button-pressed')}
title="Press me"
/>
));
Custom Action Handlers
This feature allows you to create custom action handlers for different events. In this example, the `onChangeText` event of a `TextInput` component is logged using the `action` function.
import { storiesOf } from '@storybook/react-native';
import { action } from '@storybook/addon-ondevice-actions';
import { TextInput } from 'react-native';
storiesOf('TextInput', module)
.add('with placeholder', () => (
<TextInput
placeholder="Type here"
onChangeText={action('text-changed')}
/>
));
@storybook/addon-actions is a similar addon for Storybook, but it is designed for web applications. It allows you to log actions and events in the Storybook UI, making it easier to debug and understand component behavior.
@storybook/addon-knobs allows you to dynamically edit props of your components in the Storybook UI. While it doesn't log actions, it provides a way to interact with and test components in real-time, similar to how @storybook/addon-ondevice-actions allows you to see real-time interactions.
@storybook/addon-controls is another addon that allows you to interact with component props dynamically. It provides a more advanced and flexible way to manipulate component inputs compared to @storybook/addon-ondevice-actions, which focuses on logging actions.
Storybook Actions Addon allows you to log events/actions inside stories in Storybook.
yarn add -D @storybook/addon-ondevice-actions
Then, add following content to .storybook/main.ts
:
import { StorybookConfig } from '@storybook/react-native';
const main: StorybookConfig = {
addons: ['@storybook/addon-ondevice-actions'],
};
export default main;
The actions documentation may also be useful.
FAQs
Action Logger addon for react-native storybook
The npm package @storybook/addon-ondevice-actions receives a total of 137,160 weekly downloads. As such, @storybook/addon-ondevice-actions popularity was classified as popular.
We found that @storybook/addon-ondevice-actions demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 14 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.