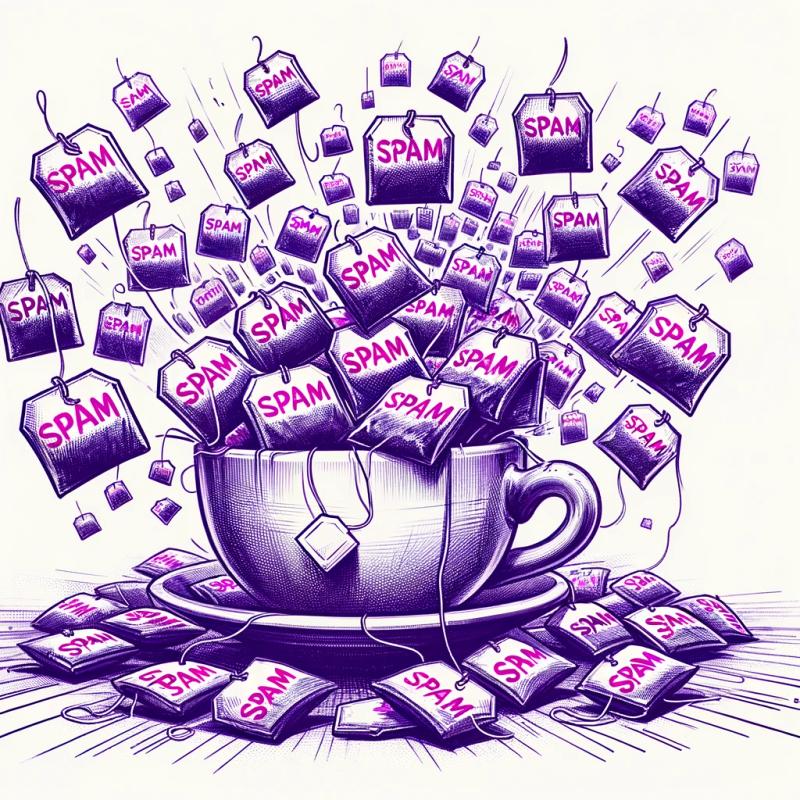
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@superkoders/modal
Advanced tools
Readme
Modal window/popup which main purpose is to open as image galery. But it can handle also other type of content like Youtube, Vimeo or HTML5 videos, iframes, custom HTML content or HTML content from some existing element on the page. It can recognize type of media from the source url. If it is not possible to recognize media type, default type is iframe. You can force media type by media
parameter (see examples below).
Using npm
$ npm install @superkoders/modal
Using yarn
$ yarn add @superkoders/modal
There are two methods exported: initModal
and createModal
. First one adds click listeners to each element in given context which matches modalSelector
option (by default '[data-modal]'
). It returns array of modal instances created. Second one is for creating custom modal instance.
(options: ISKModalOptions) => ISKModal[]
import { initModal } from '@superkoders/modal';
// use default options
initModal();
// or set some custom options
initModal({
customWrapperClass: 'my-custom-modal',
closeOnBgClick: true,
});
The easiest way is to do it with an a
tag using its href
attribute as an url for modal.
<a href="https://www.youtube.com/watch?v=RhMYBfF7-hE" data-modal>Open Youtube video in the modal</a>
If you want to use some other element like button
, you need to specify url in the data-modal
attribute.
<button data-modal='{"url": "https://www.youtube.com/watch?v=RhMYBfF7-hE"}'>Open Youtube video in the modal</button>
(items: ISKModalItem | ISKModalItem[], options?: Partial<ISKModalOptions>) => ISKModal
In this case, there are no triggers which open modal. Once you create an instance, you need to open it by yourself. Also you need to pass modal items, where only mandatory property is url
. In our example below the modal will have two items, first is some image, second is some video.
import { createModal } from '@superkoders/modal';
const items = [
{
url: 'url/to/some/image.jpg'
},
{
url: 'url/to/some/video.mp4'
}
]
// create instance
const customModal = createModal(items, {
customWrapperClass: 'my-custom-modal',
closeOnBgClick: true,
});
// open modal
customModal.methods.open();
// go to next slide
customModal.methods.next();
// close modal
customModal.methods.close();
ISKModalOptions
These options you can adjust during initialization.
Element | Document
Context element, in which to search for modal items (triggers)
Default value: document
Element
Element, which is used as a parent for modal element in DOM
Default value: document.body
string
Element selector, which is used for modal triggers
Default value: '[data-modal]'
string
Element selector, which is used to close the modal
Default value: '.js-modal-close'
string
Custom CSS class, which is added to the modal element
Default value: undefined
boolean
It determines if modal items are shown in a loop
Default value: false
boolean
It determines if modal will close after background click
Default value: undefined
ISKModalPlugin[]
Plugins array to initialize
Default value: undefined
boolean
It determines if the navigation (arrows, bullets) is hidden or not
Default value: undefined
any
Any data which you can use for example in plugins
Default value: undefined
string
String which represents an icon for close button, it can be also HTML code
Default value: '×'
string
String which represents an icon for previous button, it can be also HTML code
Default value: '←'
string
String which represents an icon for next button, it can be also HTML code
Default value: '→'
string
String which represents an icon for loader, it can be also HTML code
Default value: '<span class="b-modal__loader-icon"><span>↺</span></span>'
boolean
It determines if modal element will be removed from DOM after close
Default value: false
boolean
Enables or disables scroll lock.
Default value: true
boolean
Enables or disables focus trap.
Default value: true
FocusTrapOptions
Focus trap custom options. See Focus trap package page for more info Default value:
{
allowOutsideClick: true,
}
boolean
Enables or disables swipe slide change.
Default value: true
(modal: Element, fragments: IModalFragments) => void
Method which builds the modal HTML structure, not really necessary to change if you use grid for CSS
Default value:
(modal, {
header,
titleElem,
descElem,
content,
prevElem,
nextElem,
navElem,
loader,
bg,
}) => {
const wrapper = document.createElement('div');
wrapper.classList.add('b-modal__wrapper');
wrapper.appendChild(header);
wrapper.appendChild(titleElem);
wrapper.appendChild(descElem);
wrapper.appendChild(content);
wrapper.appendChild(prevElem);
wrapper.appendChild(nextElem);
wrapper.appendChild(navElem);
modal.appendChild(wrapper);
modal.appendChild(loader);
modal.appendChild(bg);
}
(options?: ISKModalOptions) => string
Method for header template
Default value:
(options) => `
<button type="button" class="b-modal__close ${ModalClasses.CLOSE}">
<span class="b-modal__close-text u-vhide">Close</span>
${options.closeIcon}
</button>
`
(text: string) => string
Method for title template
Default value:
(text: string) => `<h3>${text}</h3>`
(text: string) => string
Method for description template
Default value:
(text: string) => `<p>${text}<p>`
(options?: ISKModalOptions) => string
Method for previous button template
Default value:
(options) => `<button type="button" class="b-modal__prev-btn ${ModalClasses.PREV_TRIGGER}">${options.prevIcon}</button>`
(options?: ISKModalOptions) => string
Method for next button template
Default value:
(options) => `<button type="button" class="b-modal__next-btn ${ModalClasses.NEXT_TRIGGER}">${options.nextIcon}</button>`
(items: ISKModalItem[]) => string
Method for navigation (bullets) template
Default value:
(items) =>
items
.map((_item, index) => `<span class="b-modal__nav-item ${ModalClasses.NAV_TRIGGER}" data-modal-index="${index}"></span>`)
.join('')
(options?: ISKModalOptions) => string
Method for loader template
Default value:
(options) => `<span class="b-modal__loader-icon"><span>${options.loadIcon}</span></span>`
(src: string) => Promise<Node>
Method which is used for loading images
Default value:
(src) => {
return new Promise((resolve, reject) => {
let img = new Image();
img.addEventListener('load', () => resolve(img));
img.addEventListener('error', () => {
reject(new Error(`Failed to load image with src: ${src}`));
});
img.draggable = false;
img.src = src;
});
}
(url: string, element?: Element) => Promise<string>
Method which is used for fetching content from a server
Default value:
async (url) => await fetch(url).then((response) => response.text())
(event?: Event, data?: { id: number }) => void
Handler, which runs after modal is opened
Default value: undefined
(event?: Event, data?: { id: number }) => void
Handler, which runs after modal is closed
Default value: undefined
(event?: Event, data?: { modal: ISKModal }) => void
Handler, which runs after modal content is loaded
Default value: undefined
(event?: Event, data?: { page: number }) => void
Handler, which runs after modal slide has changed
Default value: undefined
All these parameters is possible to add to data-modal
attribute. In case of createModal
, these are properties of the modal item object.
string | string[]
Media url. In case of images, it can be an array. Then more images are shown on one slide.
ModalMedium
Media type. Posible values in ModalMedium
enum exported from @superkoders/modal
string
Text, which will appear in modal title
string
Text, which will appear in modal description
string
Gallery key, which is used for grouping items into one modal. If it is undefined, each modal uses its own popup.
any
It is possible to add more custom parameters, which can be used in plugins for example.
Plugins enable another modal customization. It has these three parameters:
Init method
Destroy method, it runs after modal redraw (if reInitOnRedraw
is set to true
) or after modal destroy
my-plugin.js
export const myPlugin = {
init: (modal) => {
// do some stuff like add event listeners or add some elements to DOM
},
destroy: (modal) => {
// use when you need to remove some listeners or remove some elements from DOM
},
reInitOnRedraw: true,
}
modal.js
import { initModal } from '@superkoders/modal';
import { myPlugin } from './my-plugin.js';
initModal({
plugins: [myPlugin]
});
Určuje, zda se má plugin reinicializovat při překreslení modalu
Modal uses scroll-lock
npm package when it is opened. If you use some fixed elements on your page, like fixed (sticky) header, then add data-scroll-lock-fill-gap
data attribute, which guarantees that the content of the fixed element does not jump.
FAQs
Fancybox like modal
The npm package @superkoders/modal receives a total of 89 weekly downloads. As such, @superkoders/modal popularity was classified as not popular.
We found that @superkoders/modal demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.