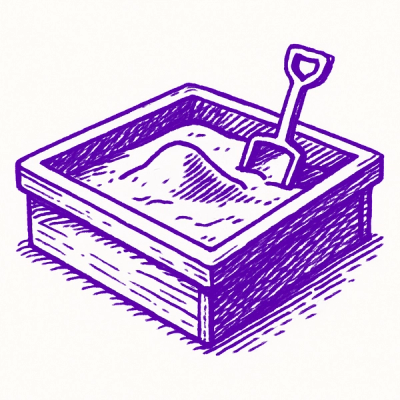
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
@techolution-ai/computer-vision
Advanced tools
A JavaScript/TypeScript library for computer vision applications, providing tools for image processing, scanning, and MQTT-based messaging.
A JavaScript/TypeScript library for computer vision applications, providing tools for image processing, scanning, and MQTT-based messaging.
The library provides several modules:
Use scanner to stream the camera's live recording to web using useScanner
hook.
import { useMemo } from 'react';
import { useScanner } from '@techolution-ai/computer-vision/scanner';
function App(){
const scanner = useScanner({ baseUrl: 'http://0.0.0.0:8501' })
const streamUrl = useMemo(()=>scanner.getVideoStreamUrl(),[scanner]);
// render the image with steaming url
return <ScanImage src={streamUrl} />
}
We can use useMessages
to connect, subscribe to certain topics, and can receive the messages on onMessage
callback.
We must use the MessagesProvider
to use useMessages
hook. It can be put at the root (or one level up) to the component's tree.
import {
MessagesProvider,
useMessages,
} from '@techolution-ai/computer-vision/messages'
function MessagesListener() {
const handleReceiveMessage = (topic: string, message: any) => {
// your topic and message is here
// add your logic here
}
useMessages({
topics: ['current_status', 'products_scanned'], // subscribing to topics
onMessage: handleReceiveMessage, // message handler
})
}
function App(){
<MessagesProvider url="ws://0.0.0.0:9001">
<MessagesListener />
</MessagesProvider>
}
We can combine above examples to do a full integration.
We also have a ready-to-use react component: ScannerStatus
with default options added which can be used to show different statuses in the UI.
import { useMemo, useState } from 'react';
import {
ScanImage,
useScanner,
ScannerStatus,
defaultStatusMap,
TStatusMap,
} from '@techolution-ai/computer-vision/scanner';
import {
MessagesProvider,
useMessages,
} from '@techolution-ai/computer-vision/messages';
function MessagesListener() {
const scanner = useScanner({ baseUrl: 'http://0.0.0.0:8501' })
const streamUrl = useMemo(() => scanner.getVideoStreamUrl(), [scanner])
const [statusMap, setStatusMap] = useState<TStatusMap | null>(
defaultStatusMap,
)
const [status, setStatus] = useState('idle')
const [messages, setMessages] = useState<{ topic: string; message: any }[]>([])
const handleReceiveMessage = (topic: string, message: any) => {
try {
const value = JSON.parse(message.toString())
if (topic === 'current_status') {
setStatus(value.status)
} else if (topic === 'item_scanned') {
setMessages((prev) => [...prev, { topic, message: value }])
}
} catch (e) {
console.error('Error parsing message:', e)
}
}
useMessages({
topics: ['current_status', 'item_scanned'],
onMessage: handleReceiveMessage,
})
return (
<ScannerStatus
style={{
border: '4px solid #4b2f99',
borderRadius: 16,
overflow: 'hidden',
position: 'relative',
width: '100%',
height: '100%',
}}
status={status}
statusMap={statusMap}
>
<ScanImage
src={streamUrl}
style={{
width: '100%',
height: '100%',
objectFit: 'cover',
position: 'absolute',
top: 0,
left: 0,
bottom: 0,
right: 0,
}}
/>
</ScannerStatus>
)
}
function App() {
return (
<MessagesProvider url="ws://0.0.0.0:9001">
<MessagesListener />
</MessagesProvider>
)
}
The library exposes three main modules:
Scanner Module
@techolution-ai/computer-vision/scanner
Messages Module
@techolution-ai/computer-vision/messages
Provider for MQTT connection.
Prop | Type | Default Value | Remarks |
---|---|---|---|
url | string | -- | Required |
enableDebugging | boolean | false | Optional, to enable logging |
Take all HTMLDivElement
props as root
Prop | Type | Default Value | Remarks |
---|---|---|---|
status | string | -- | Required |
statusMap | TStatusMap (Object) | null | Optional, uses default statusMap internally if not passed |
Prop | Type | Default Value | Remarks |
---|---|---|---|
topics | string[] | -- | Required |
onMessage | (topic:string,message:Buffer)=>void | callback to receive messages | Required |
onError | function | Callback function when an error occurs. (not implemented) | |
onConnect | function | Callback function when the connection is established. (not implemented) | |
onClose | function | Callback function when the connection is closed. (not implemented) |
Prop | Type | Default Value | Remarks |
---|---|---|---|
baseUrl | string | -- | Required |
endpoints | object | Custom endpoints for the scanner API. (Optional) |
API | Remarks |
---|---|
getVideoStreamUrl() | Returns the URL for the video stream. |
startInference() | Starts the inference process. |
stopInference() | Stops the inference process. |
captureFrame() | Captures the current frame. |
This project is licensed under the MIT License - see the LICENSE file for details.
Please read our contributing guidelines and code of conduct before submitting pull requests.
For issues and feature requests, please use the GitHub issue tracker.
FAQs
A JavaScript/TypeScript library for computer vision applications, providing tools for image processing, scanning, and MQTT-based messaging.
The npm package @techolution-ai/computer-vision receives a total of 3 weekly downloads. As such, @techolution-ai/computer-vision popularity was classified as not popular.
We found that @techolution-ai/computer-vision demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.