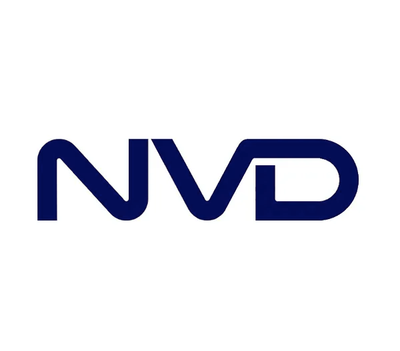
Security News
NIST Misses 2024 Deadline to Clear NVD Backlog
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.
@telnyx/react-client
Advanced tools
React wrapper for Telnyx Client
npm install --save @telnyx/react-client @telnyx/webrtc
// App.jsx
import { TelnyxRTCProvider } from '@telnyx/react-client';
function App() {
const credential = {
login_token: 'mytoken',
};
return (
<TelnyxRTCProvider credential={credential}>
<Phone />
</TelnyxRTCProvider>
);
}
// Phone.jsx
import { useNotification, Audio } from '@telnyx/react-client';
function Phone() {
const notification = useNotification();
const activeCall = notification && notification.call;
return (
<div>
{activeCall &&
activeCall.state === 'ringing' &&
'You have an incoming call.'}
<Audio stream={activeCall && activeCall.remoteStream} />
</div>
);
}
useCallbacks
import { useCallbacks } from '@telnyx/react-client';
function Phone() {
useCallbacks({
onReady: () => console.log('client ready'),
onError: () => console.log('client registration error'),
onSocketError: () => console.log('client socket error'),
onSocketClose: () => console.log('client disconnected'),
onNotification: (x) => console.log('received notification:', x),
});
// ...
}
useTelnyxRTC
If you need more fine-tuned control over TelnyxRTC, you also have access to useTelnyxRTC
directly.
import { useTelnyxRTC } from '@telnyx/react-client';
function Phone() {
const client = useTelnyxRTC({ login_token: 'mytoken' });
client.on('telnyx.ready', () => {
console.log('client ready');
});
// ...
}
Take care to use this hook only once in your application. For most cases, we recommend you use TelnyxRTCContext/TelnyxRTCProvider instead of this hook directly. This ensures that you only have one Telnyx client instance running at a time.
useContext
with TelnyxRTCContext
You can retrieve the current TelnyxRTC context value by using React's useContext
hook, as an alternative to TelnyxRTCContext.Consumer.
import React, { useContext } from 'react';
import { TelnyxRTCContext } from '@telnyx/react-client';
function Phone() {
const client = useContext(TelnyxRTCContext);
client.on('telnyx.ready', () => {
console.log('client ready');
});
// ...
}
TelnyxRTCContextProvider
import { TelnyxRTCProvider } from '@telnyx/react-client';
function App() {
const credential = {
// You can either use your On-Demand Credential token
// or your Telnyx SIP username and password
// login_token: 'mytoken',
login: 'myusername',
password: 'mypassword',
};
const options = {
ringtoneFile: 'https://example.com/sounds/incoming_call.mp3',
ringbackFile: 'https://example.com/sounds/ringback_tone.mp3',
};
return (
<TelnyxRTCProvider credential={credential} options={options}>
<Phone />
</TelnyxRTCProvider>
);
}
TelnyxRTCContext.Consumer
import { TelnyxRTCContext } from '@telnyx/react-client';
function PhoneWrapper() {
return (
<TelnyxRTCContext.Consumer>
{(context) => <Phone client={context} />}
</TelnyxRTCContext.Consumer>
);
}
Audio
import { Audio } from '@telnyx/react-client';
function Phone({ activeCall }) {
return (
<div>
<Audio stream={activeCall.remoteStream} />
</div>
);
}
Video
import { Video } from '@telnyx/react-client';
function VideoConference({ activeCall }) {
return (
<div>
<Video stream={activeCall.localStream} muted />
<Video stream={activeCall.remoteStream} />
</div>
);
}
Install dependencies:
yarn install
yarn start
yarn link
# in another tab:
git clone https://github.com/team-telnyx/webrtc-examples/tree/main/react-client/react-app
# fill in .env
yarn install
yarn link @telnyx/react-client
yarn start
FAQs
React wrapper for Telnyx Client
We found that @telnyx/react-client demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.