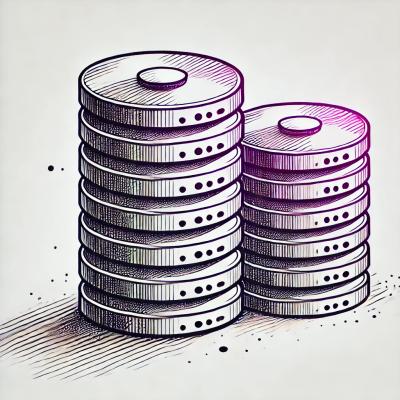
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
@testing-library/react-native
Advanced tools
Simple and complete React Native testing utilities that encourage good testing practices.
@testing-library/react-native is a testing library for React Native applications. It provides utilities to test React Native components in a way that resembles how users interact with your app. The library encourages writing tests that are more maintainable and less coupled to the implementation details of the components.
Rendering Components
This feature allows you to render React Native components and query them for assertions. The example demonstrates rendering a simple Text component and verifying its content.
const { render } = require('@testing-library/react-native');
const { Text } = require('react-native');
const { getByText } = render(<Text>Hello, World!</Text>);
expect(getByText('Hello, World!')).toBeTruthy();
Fire Events
This feature allows you to simulate user interactions such as pressing a button. The example shows how to render a Button component and simulate a press event.
const { render, fireEvent } = require('@testing-library/react-native');
const { Button } = require('react-native');
const handlePress = jest.fn();
const { getByText } = render(<Button title="Press me" onPress={handlePress} />);
fireEvent.press(getByText('Press me'));
expect(handlePress).toHaveBeenCalled();
Async Utilities
This feature provides utilities to handle asynchronous operations in your components. The example demonstrates how to wait for a state change in a component that updates after a timeout.
const { render, waitFor } = require('@testing-library/react-native');
const { useEffect, useState } = require('react');
const { Text } = require('react-native');
const AsyncComponent = () => {
const [data, setData] = useState(null);
useEffect(() => {
setTimeout(() => {
setData('Loaded');
}, 1000);
}, []);
return <Text>{data}</Text>;
};
const { getByText } = render(<AsyncComponent />);
await waitFor(() => expect(getByText('Loaded')).toBeTruthy());
Enzyme is a JavaScript testing utility for React that makes it easier to test your React Components' output. It provides a more detailed API for interacting with components, but it is more tightly coupled to the implementation details compared to @testing-library/react-native.
React Test Renderer is a package from the React team that allows you to render React components to pure JavaScript objects without depending on the DOM or a native mobile environment. It is useful for snapshot testing but does not provide the user-centric querying and event simulation features of @testing-library/react-native.
Jest is a delightful JavaScript Testing Framework with a focus on simplicity. While Jest itself is not specific to React Native, it is often used in conjunction with @testing-library/react-native for assertions and mocking. Jest provides a comprehensive testing solution but lacks the specific utilities for interacting with React Native components that @testing-library/react-native offers.
Developer-friendly and complete React Native testing utilities that encourage good testing practices.
You want to write maintainable tests for your React Native components. As a part of this goal, you want your tests to avoid including implementation details of your components and rather focus on making your tests give you the confidence for which they are intended. As part of this, you want your tests to be maintainable in the long run so refactors of your components (changes to implementation but not functionality) don't break your tests and slow you and your team down.
The React Native Testing Library (RNTL) is a comprehensive solution for testing React Native components. It provides React Native runtime simulation on top of react-test-renderer
, in a way that encourages better testing practices. Its primary guiding principle is:
The more your tests resemble the way your software is used, the more confidence they can give you.
This project is inspired by React Testing Library. Tested to work with Jest, but it should work with other test runners as well.
Open a Terminal in your project's folder and run:
# Yarn install:
yarn add --dev @testing-library/react-native
# NPM install
npm install --save-dev @testing-library/react-native
This library has a peerDependencies
listing for react-test-renderer
. Make sure that your react-test-renderer
version matches exactly the react
version, avoid using ^
in version number.
You can use the built-in Jest matchers automatically by having any import from @testing-library/react-native
in your test.
import { render, screen, userEvent } from '@testing-library/react-native';
import { QuestionsBoard } from '../QuestionsBoard';
// It is recommended to use userEvent with fake timers
// Some events involve duration so your tests may take a long time to run.
jest.useFakeTimers();
test('form submits two answers', async () => {
const questions = ['q1', 'q2'];
const onSubmit = jest.fn();
const user = userEvent.setup();
render(<QuestionsBoard questions={questions} onSubmit={onSubmit} />);
const answerInputs = screen.getAllByLabelText('answer input');
// simulates the user focusing on TextInput and typing text one char at a time
await user.type(answerInputs[0], 'a1');
await user.type(answerInputs[1], 'a2');
// simulates the user pressing on any pressable element
await user.press(screen.getByRole('button', { name: 'Submit' }));
expect(onSubmit).toHaveBeenCalledWith({
1: { q: 'q1', a: 'a1' },
2: { q: 'q2', a: 'a2' },
});
});
You can find the source of QuestionsBoard
component and this example here.
React Native Testing Library consists of following APIs:
render
function - render your UI components for testing purposesscreen
object - access rendered UI:
press
or type
in a realistic wayrenderHook
function - render hooks for testing purposesfindBy*
queries, waitFor
, waitForElementToBeRemoved
configure
, resetToDefaults
isHiddenFromAccessibility
within
, act
, cleanup
Check out our list of Community Resources about RNTL.
React Native Testing Library is an open source project and will always remain free to use. If you think it's cool, please star it 🌟. Callstack is a group of React and React Native geeks, contact us at hello@callstack.com if you need any help with these or just want to say hi!
Like the project? ⚛️ Join the team who does amazing stuff for clients and drives React Native Open Source! 🔥
Supported and used by Rally Health.
FAQs
Simple and complete React Native testing utilities that encourage good testing practices.
The npm package @testing-library/react-native receives a total of 755,714 weekly downloads. As such, @testing-library/react-native popularity was classified as popular.
We found that @testing-library/react-native demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 16 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.