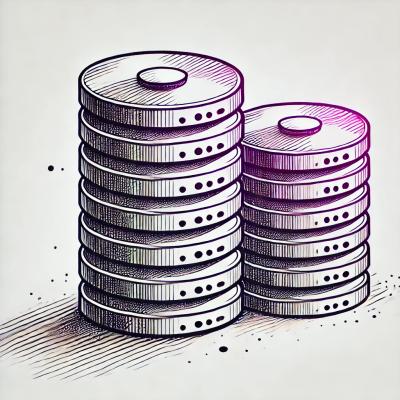
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Enzyme is a JavaScript testing utility for React that makes it easier to assert, manipulate, and traverse your React Components' output. It is designed to work with test runners like Jest or Mocha, and it provides a more intuitive and flexible API for interacting with React component trees.
Shallow Rendering
Shallow rendering is useful to constrain yourself to testing a component as a unit, and to ensure that your tests aren't indirectly asserting on behavior of child components.
import { shallow } from 'enzyme';
import MyComponent from './MyComponent';
const wrapper = shallow(<MyComponent />);
expect(wrapper.find('.my-class').length).toBe(1);
Full DOM Rendering
Full DOM rendering is ideal for use cases where you have components that may interact with DOM APIs, or may require the full lifecycle in order to fully test the component (i.e., componentDidMount etc.).
import { mount } from 'enzyme';
import MyComponent from './MyComponent';
const wrapper = mount(<MyComponent />);
expect(wrapper.find('.my-class').length).toBe(1);
Static Rendering
Static rendering is used to render the component to static HTML. It's useful for rendering components to strings, for instance, to send in emails, or for analyzing the markup structure.
import { render } from 'enzyme';
import MyComponent from './MyComponent';
const wrapper = render(<MyComponent />);
expect(wrapper.find('.my-class').length).toBe(1);
React Testing Library is a very popular alternative to Enzyme that provides light utility functions on top of react-dom and react-dom/test-utils, in a way that encourages better testing practices. It focuses on testing components 'as the user would' rather than testing implementation details.
Jest is a delightful JavaScript Testing Framework with a focus on simplicity. It works well with React and can be used as a test runner, assertion library, and mocking library. Jest's snapshot testing feature can be seen as an alternative to Enzyme's rendering capabilities.
Enzyme is a JavaScript Testing utility for React that makes it easier to test your React Components' output. You can also manipulate, traverse, and in some ways simulate runtime given the output.
Enzyme's API is meant to be intuitive and flexible by mimicking jQuery's API for DOM manipulation and traversal.
Are you here to check whether or not Enzyme is compatible with React 16? Are you currently using Enzyme 2.x? Great! Check out our migration guide for help moving on to Enzyme v3 where React 16 is supported.
To get started with enzyme, you can simply install it via npm. You will need to install enzyme along with an Adapter corresponding to the version of react (or other UI Component library) you are using. For instance, if you are using enzyme with React 16, you can run:
npm i --save-dev enzyme enzyme-adapter-react-16
Each adapter may have additional peer dependencies which you will need to install as well. For instance,
enzyme-adapter-react-16
has peer dependencies on react
and react-dom
.
At the moment, Enzyme has adapters that provide compatibility with React 16.x
, React 15.x
,
React 0.14.x
and React 0.13.x
.
The following adapters are officially provided by enzyme, and have the following compatibility with React:
Enzyme Adapter Package | React semver compatibility |
---|---|
enzyme-adapter-react-16 | ^16.4.0-0 |
enzyme-adapter-react-16.3 | ~16.3.0-0 |
enzyme-adapter-react-16.2 | ~16.2 |
enzyme-adapter-react-16.1 | ~16.0.0-0 || ~16.1 |
enzyme-adapter-react-15 | ^15.5.0 |
enzyme-adapter-react-15.4 | 15.0.0-0 - 15.4.x |
enzyme-adapter-react-14 | ^0.14.0 |
enzyme-adapter-react-13 | ^0.13.0 |
Finally, you need to configure enzyme to use the adapter you want it to use. To do this, you can use
the top level configure(...)
API.
import Enzyme from 'enzyme';
import Adapter from 'enzyme-adapter-react-16';
Enzyme.configure({ adapter: new Adapter() });
It is possible for the community to create additional (non-official) adapters that will make enzyme work with other libraries. If you have made one and it's not included in the list below, feel free to make a PR to this README and add a link to it! The known 3rd party adapters are:
Adapter Package | For Library | Status |
---|---|---|
enzyme-adapter-preact-pure | preact | (stable) |
enzyme-adapter-inferno | inferno | (work in progress) |
Enzyme is unopinionated regarding which test runner or assertion library you use, and should be compatible with all major test runners and assertion libraries out there. The documentation and examples for enzyme use mocha and chai, but you should be able to extrapolate to your framework of choice.
If you are interested in using enzyme with custom assertions and convenience functions for testing your React components, you can consider using:
chai-enzyme
with Mocha/Chai.jasmine-enzyme
with Jasmine.jest-enzyme
with Jest.should-enzyme
for should.js.expect-enzyme
for expect.Using Enzyme with React Native
Using Enzyme with Tape and AVA
import React from 'react';
import { expect } from 'chai';
import { shallow } from 'enzyme';
import sinon from 'sinon';
import MyComponent from './MyComponent';
import Foo from './Foo';
describe('<MyComponent />', () => {
it('renders three <Foo /> components', () => {
const wrapper = shallow(<MyComponent />);
expect(wrapper.find(Foo)).to.have.lengthOf(3);
});
it('renders an `.icon-star`', () => {
const wrapper = shallow(<MyComponent />);
expect(wrapper.find('.icon-star')).to.have.lengthOf(1);
});
it('renders children when passed in', () => {
const wrapper = shallow((
<MyComponent>
<div className="unique" />
</MyComponent>
));
expect(wrapper.contains(<div className="unique" />)).to.equal(true);
});
it('simulates click events', () => {
const onButtonClick = sinon.spy();
const wrapper = shallow(<Foo onButtonClick={onButtonClick} />);
wrapper.find('button').simulate('click');
expect(onButtonClick).to.have.property('callCount', 1);
});
});
Read the full API Documentation
import React from 'react';
import sinon from 'sinon';
import { expect } from 'chai';
import { mount } from 'enzyme';
import Foo from './Foo';
describe('<Foo />', () => {
it('allows us to set props', () => {
const wrapper = mount(<Foo bar="baz" />);
expect(wrapper.props().bar).to.equal('baz');
wrapper.setProps({ bar: 'foo' });
expect(wrapper.props().bar).to.equal('foo');
});
it('simulates click events', () => {
const onButtonClick = sinon.spy();
const wrapper = mount((
<Foo onButtonClick={onButtonClick} />
));
wrapper.find('button').simulate('click');
expect(onButtonClick).to.have.property('callCount', 1);
});
it('calls componentDidMount', () => {
sinon.spy(Foo.prototype, 'componentDidMount');
const wrapper = mount(<Foo />);
expect(Foo.prototype.componentDidMount).to.have.property('callCount', 1);
Foo.prototype.componentDidMount.restore();
});
});
Read the full API Documentation
import React from 'react';
import { expect } from 'chai';
import { render } from 'enzyme';
import Foo from './Foo';
describe('<Foo />', () => {
it('renders three `.foo-bar`s', () => {
const wrapper = render(<Foo />);
expect(wrapper.find('.foo-bar')).to.have.lengthOf(3);
});
it('renders the title', () => {
const wrapper = render(<Foo title="unique" />);
expect(wrapper.text()).to.contain('unique');
});
});
Read the full API Documentation
Enzyme supports react hooks with some limitations in .shallow()
due to upstream issues in React's shallow renderer:
useEffect()
and useLayoutEffect()
don't get called in the React shallow renderer. Related issue
useCallback()
doesn't memoize callback in React shallow renderer. Related issue
ReactTestUtils.act()
wrapIf you're using React 16.8+ and .mount()
, Enzyme will wrap apis including .simulate()
, .setProps()
, .setContext()
, .invoke()
with ReactTestUtils.act()
so you don't need to manually wrap it.
A common pattern to trigger handlers with .act()
and assert is:
const wrapper = mount(<SomeComponent />);
act(() => wrapper.prop('handler')());
wrapper.update();
expect(/* ... */);
We cannot wrap the result of .prop()
(or .props()
) with .act()
in Enzyme internally since it will break the equality of the returned value.
However, you could use .invoke()
to simplify the code:
const wrapper = mount(<SomeComponent />);
wrapper.invoke('handler')();
expect(/* ... */);
See the Contributors Guide
Organizations and projects using enzyme
can list themselves here.
3.11.0
render
: handle Fiber strings and numbers (#2221)shallow
: Share child context logic between shallow
and dive
(#2296)mount
: children
: include text nodes ($2269)mount
: invoke
: use adapter’s wrapInvoke
if present (#2158)mount
/shallow
: closest
/parent
: Add missing arguments description (#2264)mount
/shallow
: fix pluralization of “exist” (#2262)shallow
/mount
: simulate
: added functional component example to simulate doc (#2248)mount
: debug
: add missing verbose option flag (#2184)mount
/shallow
: update
: fix semantics description (#2194)invoke
: Add missing backticks to end of codeblock (#2160)invoke
: Fix typo (#2167)funding
fieldis-boolean-object
, is-callable
, is-number-object
, is-string
, enzyme-shallow-equal
, array.prototype.flat
, function.prototype.name
, html-element-map
, is-regex
, object-inspect
, object-is
, object.entries
, object.vales
, raf
, string.prototype.trim
eslint
, eslint-plugin-import
, eslint-plugin-markdown
, eslint-plugin-react
, safe-publish-latest
, eslint-config-airbnb
, rimraf
, safe-publish-latest
, karma-firefox-launcher
, babel-preset-airbnb
, glob-gitignore
, semver
, eslint-plugin-jsx-a11y
FAQs
JavaScript Testing utilities for React
The npm package enzyme receives a total of 1,068,224 weekly downloads. As such, enzyme popularity was classified as popular.
We found that enzyme demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 8 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.