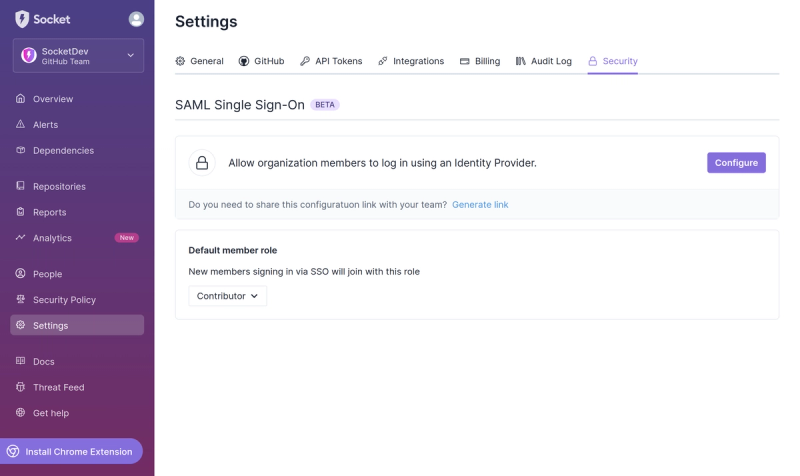
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
@threadsjs/threads.js
Advanced tools
Readme
thread.js is a Node.js library that allows you to interact with the Threads API
npm install @threadsjs/threads.js
const { Client } = require('@threadsjs/threads.js');
(async () => {
const client = new Client();
// You can also specify a token: const client = new Client({ token: 'token' });
await client.login('username', 'password');
await client.users.fetch(25025320).then(user => {
console.log(user);
});
})();
In the parameters, pass the user id (supported as string and number) of the user whose information you want to get.
await client.users.fetch(1)
Pass the query as the first parameter, and the number of objects in the response as the second parameter (by default - 30). The minimum is 30.
await client.users.search("zuck", 10)
In the parameters, pass the user id (supported as string and number) of the user you want to restrict.
await client.users.restrict(1)
In the parameters, pass the user id (supported as string and number) of the user you want to unrestrict.
await client.users.unrestrict(1)
In the parameters, pass the user id (supported as string and number) of the user whose friendship status information you want to get.
await client.friendships.show(1)
Pass the user id (supported as string and number) of the user you want to subscribe to in the parameters
await client.friendships.follow(1)
Pass the user id (supported as string and number) of the user you want to unsubscribe from in the parameters
await client.friendships.unfollow(1)
In the parameters, pass the user id (supported as string and number) of the user whose followers you want to get.
await client.friendships.followers(1)
In the parameters, pass the user id (supported as string and number) of the user whose followings you want to get.
await client.friendships.following(1)
In the parameters, pass the user id (supported as string and number) of the user you want to mute.
await client.friendships.mute(1)
In the parameters, pass the user id (supported as string and number) of the user you want to unmute.
await client.friendships.unmute(1)
In the parameters, pass the user id (supported as string and number) of the user you want to block.
await client.friendships.block(1)
In the parameters, pass the user id (supported as string and number) of the user you want to unblock.
await client.friendships.unblock(1)
Gets the default feed. In the parameters, pass the optional max_id of the previous response's next_max_id.
await client.feeds.fetch()
await client.feeds.fetch("aAaAAAaaa")
In the parameters, pass the user id (supported as string and number) of the user whose threads you want to get, and an optional max_id of the previous response's next_max_id.
await client.feeds.fetchThreads(1),
await client.feeds.fetchThreads(1, "aAaAAAaaa")
In the parameters, pass the user id (supported as string and number) of the user whose replies you want to get, and an optional max_id of the previous response's next_max_id.
await client.feeds.fetchReplies(1)
await client.feeds.fetchReplies(1, "aAaAAAaaa")
Getting a list of recommendations. In the parameters, pass the optional paging_token of the previous response.
await client.feeds.recommended()
await client.feeds.recommended(15)
Getting a list of recommendations. In the parameters, pass an optional filter type and an optional pagination object with max_id and pagination_first_record_timestamp from the previous response. Valid filter types:
let pagination = {
max_id: "1688921943.766884",
pagination_first_record_timestamp: "1689094189.845912"
}
await client.feeds.notifications()
await client.feeds.notifications(null, pagination)
await client.feeds.notifications("text_post_app_replies")
await client.feeds.notifications("text_post_app_replies", pagination)
Clears all notifications. You might want to do this after client.feeds.notifications() and checking new_stories for what wasn't seen.
await client.feeds.notificationseen()
In the parameters pass the id of the post you want to get information about, and an optional pagination token from the previous request.
await client.posts.fetch("aAaAAAaaa")
await client.posts.fetch("aAaAAAaaa", "aAaAAAaaa")
In the parameters pass the id of the post whose likes you want to get
await client.posts.likers("aAaAAAaaa")
The method is used to create a thread. Pass the text of the thread as the first parameter, and the user id (supported as string and number) as the second
await client.posts.create(1, { contents: "Hello World!" })
The method is used to create reply to a thread. Pass the text of the reply as the first parameter, the user id (supported as string and number) as the second, and post id as the third
await client.posts.reply(1, { contents: "Hello World!", post: "aAaAAAaaa" })
The method is used to create a quote thread. Pass the text of the quote comment as the first parameter, the user id as the second, and post id as the third
await client.posts.quote(1, { contents: "Hello World!", post: "aAaAAAaaa" })
The method is used to delete a thread. Pass the post id as the first parameter, and the user id (supported as string and number) as the second
await client.posts.delete("aAaAAAaaa", 1)
The method is used to like a thread. Pass the post id as the first parameter, and the user id (supported as string and number) as the second
await client.posts.like("aAaAAAaaa", 1)
The method is used to unlike a thread. Pass the post id as the first parameter, and the user id (supported as string and number) as the second
await client.posts.unlike("aAaAAAaaa", 1)
The method is used to repost a thread. Pass the post id as the only parameter
await client.posts.repost("aAaAAAaaa")
The method is used to un-repost a thread. Pass the post id as the only parameter
await client.posts.unrepost("aAaAAAaaa")
FAQs
Unknown package
The npm package @threadsjs/threads.js receives a total of 40 weekly downloads. As such, @threadsjs/threads.js popularity was classified as not popular.
We found that @threadsjs/threads.js demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.