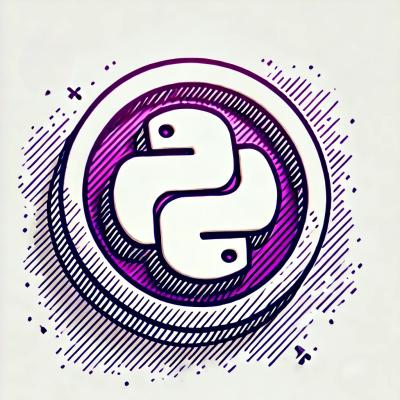
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
@tldraw/core
Advanced tools
This package contains the core of the tldraw
library. It includes:
Renderer
- a React componentTLShapeUtility
- an abstract class for custom shape utilitiestypes
yarn add @tldraw/core
Import the Renderer
component and pass it the required props.
Renderer
page
- A TLPage
object.pageState
- A TLPageState
object.shapeUtils
- A table of TLShapeUtils
classes.theme
- (optional) an object with overrides for the Renderer's default colors.
foreground
- The primary (usually "text") colorbackground
- The default page's background colorbrushFill
- The fill color of the brush selection boxbrushStroke
- The stroke color of the brush selection boxselectFill
- The fill color of the selection boundsselectStroke
- The stroke color of the selection bounds and handlesTip: If providing an object for the
theme
prop, memoize it first withReact.useMemo
.
The renderer also accepts many (optional) event callbacks.
onPan
- The user panned with the mouse wheelonZoom
- The user zoomed with the mouse wheelonPinch
- The user moved their pointers during a pinchonPinchEnd
- The user stopped a two-pointer pinchonPinchStart
- The user began a two-pointer pinchonPointerMove
- The user moved their pointeronStopPointing
- The user ended a pointonPointShape
- The user pointed a shapeonDoublePointShape
- The user double-pointed a shapeonRightPointShape
- The user right-pointed a shapeonMoveOverShape
- The user moved their pointer a shapeonHoverShape
- The user moved their pointer onto a shapeonUnhoverShape
- The user moved their pointer off of a shapeonPointHandle
- The user pointed a shape handleonDoublePointHandle
- The user double-pointed a shape handleonRightPointHandle
- The user right-pointed a shape handleonMoveOverHandle
- The user moved their pointer over a shape handleonHoverHandle
- The user moved their pointer onto a shape handleonUnhoverHandle
- The user moved their pointer off of a shape handleonPointCanvas
- The user pointed the canvasonDoublePointCanvas
- The user double-pointed the canvasonRightPointCanvas
- The user right-pointed the canvasonPointBounds
- The user pointed the selection boundsonDoublePointBounds
- The user double-pointed the selection boundsonRightPointBounds
- The user right-pointed the selection boundsonPointBoundsHandle
- The user pointed a selection bounds edge or corneronDoublePointBoundsHandle
- The user double-pointed a selection bounds edge or corneronBlurEditingShape
- The user blurred an editing (text) shapeonError
- The renderer encountered an errorTLPage
An object describing the current page. It contains:
id
- a unique id for the pageshapes
- a table of TLShape
objectsbindings
- a table of TLBinding
objectsbackgroundColor
- (optional) the page's background fill colorTLPageState
An object describing the current page. It contains:
id
- the corresponding page's idcurrentParentId
: the current parent id for selectionselectedIds
: an array of selected shape idscamera
: an object describing the camera state
point
- the camera's [x, y]
coordinateszoom
- the camera's zoom levelbrush
: (optional) a Bounds
for the current selection boxpointedId
: (optional) the currently pointed shape idhoveredId
: (optional) the currently hovered shape ideditingId
: (optional) the currently editing shape ideditingBindingId
: (optional) the currently editing binding idTLShape
An object that describes a shape on the page. The shapes in your document should extend this interface with other properties. See Guide: Create a Custom Shape.
id
: stringtype
- the type of the shape, corresponding to the type
of a TLShapeUtil
.parentId
- the id of the shape's parent (either the current page or another shape).childIndex
- the order of the shape among its parent's childrenname
- the name of the shapepoint
- the shape's current [x, y]
coordinates on the pagerotation
- the shape's current rotation in radianschildren
- (optional) An array containing the ids of this shape's childrenhandles
- (optional) A table of TLHandle
objectsisLocked
- (optional) True if the shape is lockedisHidden
- (optional) True if the shape is hiddenisEditing
- (optional) True if the shape is currently editingisGenerated
- (optional) True if the shape is generated programaticallyisAspectRatioLocked
- (optional) True if the shape's aspect ratio is lockedTLBinding
An object that describes a relationship between two shapes on the page.
id
- a unique id for the bindingtype
- the binding's typefromId
- the id of the shape where the binding beginstoId
- the id of the shape where the binding beginsTLShapeUtil
The TLShapeUtil
is an abstract class that you can extend to create utilities for your custom shapes. See Guide: Create a Custom Shape.
Utils
A general purpose utility class.
Vec
A utility class for vector math and related methods.
Svg
A utility class for creating SVG path data through imperative commands.
Intersect
A utility class for finding intersections between various geometric shapes.
...
import * as React from "react"
import { Renderer, TLShape, TLShapeUtil, Vec } from '@tldraw/core'
interface RectangleShape extends TLShape {
type: "rectangle",
size: number[]
}
class Rectangle extends TLShapeUtil<RectangleShape> {
// See the "Create a Custom Shape" guide
}
const myShapes = { rectangle: new Rectangle() }
function App() {
const [page, setPage] = React.useState({
id: "page1"
shapes: {
"rect1": {
id: 'rect1',
parentId: 'page1',
name: 'Rectangle',
childIndex: 0,
type: 'rectangle',
point: [0, 0],
rotation: 0,
size: [100, 100],
}
},
bindings: {}
})
const [pageState, setPageState] = React.useState({
id: "page1",
selectedIds: [],
camera: {
point: [0,0],
zoom: 1
}
})
const handlePan = React.useCallback((delta: number[]) => {
setPageState(pageState => {
...pageState,
camera: {
zoom,
point: Vec.sub(pageState.point, Vec.div(delta, pageState.zoom)),
},
})
}, [])
return (<Renderer
shapes={myShapes}
page={page}
pageState={pageState}
onPan={handlePan}
/>)
}
Run yarn
to install dependencies.
Run yarn start
to begin the monorepo's development server (@tldraw/site
).
Run nx test core
to execute unit tests via Jest.
To contribute, visit the Discord channel.
FAQs
The tldraw core renderer and utilities.
We found that @tldraw/core demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.