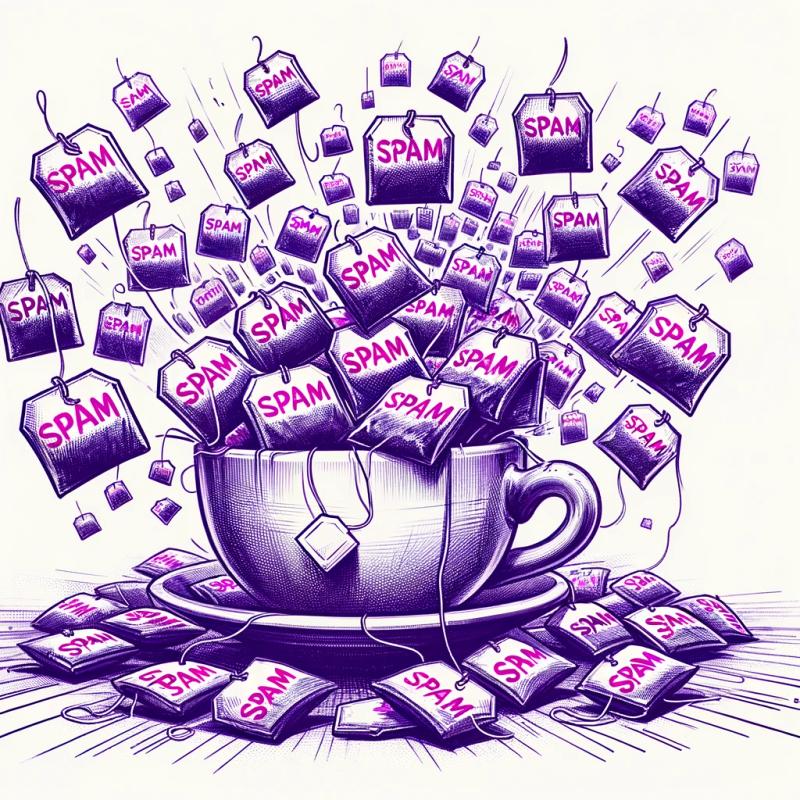
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@toplinker/vue3-axios-request
Advanced tools
Readme
A wrapper for Axios with Vue3 componsition API, It's easy to use and support polling, retry, cache, debounce, throttle...
npm install @toplinker/vue3-axios-request
# or
yarn add @toplinker/vue3-axios-request
<template>
<button @click.prevent="res.run()">Load Data</button>
<div>
<div>loading={{ res.loading }}</div>
<div>error={{ res.error }}</div>
<div>data={{ res.data }}</div>
</div>
</template>
<script lang="ts">
import { defineComponent } from "vue";
/**
* 1. import `useAxiosRequest`
*/
import { useAxiosRequest } from "@toplinker/vue3-axios-request";
export default defineComponent({
setup() {
/**
* 2. create a http instance
*/
const http = useAxiosRequest();
/**
* 3. use http.get(...) or http.post(..) similar as axios usage,
* only with an extra ExecuteOptions.
*/
const res = http.get(
"https://api.github.com/search/repositories", // URL
{ params: { q: "vue3-axios-request" } }, // AxiosRequestConfig
{ retryCount: 10, retryInterval: 100 } // ExecuteOptions
);
/**
* 4. return a result, include data, error, loading, run(), ... Thoses values
* are Ref<>, so it can directly used in html template
*/
return { res };
},
});
</script>
useAxiosGet
,useAxioPost
...As a alternative, you can use useAxiosGet
, useAxiosPost
to realize the same function without create a useAxiosRequest
instance.
<template>
<button @click.prevent="res.run()">Load Data</button>
<div>
<div>loading={{ res.loading }}</div>
<div>error={{ res.error }}</div>
<div>data={{ res.data }}</div>
</div>
</template>
<script lang="ts">
import { defineComponent } from "vue";
/**
* 1. improt `useAxiosGet`,`useAxiosPost` etc.
*/
import { useAxiosGet } from "@toplinker/vue3-axios-request";
export default defineComponent({
setup() {
/**
* 2. direct call function `useAxiosGet`.
*/
const res = useAxiosGet(
"https://api.github.com/search/repositories", // URL
{ params: { q: "vue3-axios-request" } }, // AxiosRequestConfig
{ retryCount: 10, retryInterval: 100 } // ExecuteOptions
);
/**
* 3. return a result, include data, error, loading, run(), ... Thoses values
* are Ref<>, so it can directly used in html template
*/
return { res };
},
});
</script>
When create useAxiosRequest
instance. you can preset some default AxiosRequestConfig
and ExecuteOptions
.
The full AxiosRequestConfig
API, please see Axios Official Doc.
The full ExecuteOptions
API, see below [API/ExecuteOptions] section.
<template>
<button @click.prevent="res.run()">Load Data</button>
<div>
<div>loading={{ res.loading }}</div>
<div>error={{ res.error }}</div>
<div>data={{ res.data }}</div>
</div>
</template>
<script lang="ts">
import { defineComponent } from "vue";
import { useAxiosRequest } from "@toplinker/vue3-axios-request";
export default defineComponent({
setup() {
/**
* 1. When create a AxiosRequest instance, preset some ExecuteOptions and AxiosRequestConfig
*/
const http = useAxiosRequest(
{
debounceInterval: 300,
loadingDelay: 200,
retryCount: 10,
retryInterval: 100,
manual: true,
},
{ baseURL: "https://api.github.com/" }
);
const res = http.get(
"/search/repositories", // URL
{ params: { q: "vue3-axios-request" } } // AxiosRequestConfig
// ExecuteOptions with preset setting
);
return { res };
},
});
</script>
You can use useExecuteOptions('name')
function to create a ExecuteOptions
with a global name
.
When execute useExecuteOptions('name')
, if the name
never used before, it will create a new one.
If the name
alreay exists, it will return from global.
Similar with useExecuteOptions('name')
, useAxios('name')
can create multiple global AxiosInstance
with different default request config, or different interceptors.
useExecuteOptions()
anduseAxios()
without name, it will set the name asdefault
<template>
<button @click.prevent="res.run()">Load Data</button>
<div>
<div>loading={{ res.loading }}</div>
<div>error={{ res.error }}</div>
<div>data={{ res.data }}</div>
</div>
</template>
<script lang="ts">
import { defineComponent } from "vue";
import { useAxiosRequest, useExecuteOptions, useAxios } from "@toplinker/vue3-axios-request";
export default defineComponent({
setup() {
/**
* 1. Create a new global ExecuteOptions with name `MyOptions`,
* and set it's default value
*/
let myoptions = useExecuteOptions("MyOptions");
myoptions.debounceInterval = 300;
myoptions.retryCount = 10;
myoptions.retryInterval = 100;
myoptions.manual = true;
// myoptions....
/**
* 2. Create a new global AxiosInstance with name `MyAxios`,
* then you can set the default AxiosRequestConfig and
* request or response interceptors
*/
let myaxios = useAxios("MyAxios");
myaxios.defaults.baseURL = "https://api.github.com/";
// myaxios.interceptors.request.use(...)
// myaxios.interceptors.response.use(...)
/**
* 3. Create AxiosRequest with global Axios and ExecuteOption by name.
* The name is case-insensitive
*/
const http = useAxiosRequest("myoptions", "myaxios");
const res = http.get(
"/search/repositories", // URL
{ params: { q: "vue3-axios-request" } } // AxiosRequestConfig
// ExecuteOptions with global setting
);
return { res };
},
});
As a alternative with static AxiosRequestConfig, you can also use a function with some parameters and return a AxiosRequestConfig.
After with functional AxiosRequestConfig, use run(...)
can change the parameter to get different result.
<template>
<button @click.prevent="res.run('vue2-axios')">Load Data</button>
<div>
<div>loading={{ res.loading }}</div>
<div>error={{ res.error }}</div>
<div>data={{ res.data }}</div>
</div>
</template>
<script lang="ts">
import { defineComponent } from "vue";
import { useAxiosRequest } from "@toplinker/vue3-axios-request";
export default defineComponent({
setup() {
/**
* 1. Create a function return AxiosRequestConfig.
* it will use ExecutionOptions's `defaultParams` as arguments
*/
const res = useAxiosRequest().get(
"https://api.github.com/search/repositories",
(search: string) => {
return { params: { q: search } };
}, // arrow function return a AxiosRequestConfig
{ defaultParams: ["vue3-axios-request"] } // ExecuteOptions with defaultParams
);
return { res };
/**
* 2. You can use `res.run(...)`, set new parameters to change the AxiosRequestConfig,
* then it will get different result data.
* (see in html template `@click.prevent="res.run('vue2-axios')`, [Line 2])
*/
},
});
</script>
We also provide useRequest
to execute any Promise
function. the useage is same as useAxiosRequest
,
just replace the AxiosRequestConfig
with a Promise
function.
Below example, we use fetch
to replace axios
. but any Promise
function (even without network request) still works.
<template>
<button @click.prevent="res.run('vue2-axios')">Load Data</button>
<div>
<div>loading={{ res.loading }}</div>
<div>error={{ res.error }}</div>
<div>data={{ res.data }}</div>
</div>
</template>
<script lang="ts">
import { defineComponent } from "vue";
import { useRequest } from "@toplinker/vue3-axios-request";
export default defineComponent({
setup() {
/**
* 1. Create any funtion which return a Promise<R>
*/
const query = (search: string) => {
return fetch("https://api.github.com/search/repositories?q=" + search);
};
/**
* 2. Execute `useRequest(...)` with default parameters
*/
const res = useRequest(query, { defaultParams: ["vue3-axios-request"] });
/**
* 3. All `ExecuteOptions` and result features same as `useAxiosRequest`
*/
return { res };
/**
* 4. Also can use `res.run(...)`, set new parameters to get different result data.
* (see in html template `@click.prevent="res.run('vue2-axios')`, [Line 2])
*/
},
});
</script>
Option | DataType | Description |
---|---|---|
initData | any | Initial result data |
defaultParams | any[] | Default parameters |
pollingInterval | number | Polling Interval (ms) |
debounceInterval | number | Debounce Interval (ms) |
throttleInterval | number | Throttle Interval (ms) |
cacheKey | string | Cache key, use with cacheTime , next time if the cache is valid, will return cache value |
cacheTime | number | Cache expire time (seconds) |
retryCount | number | Max error retry count |
retryInterval | number | Each error retry interval (ms) |
loadingDelay | number | Delay to set loading (ms) |
manual | boolean | If true will not auto execute, need manually call run() function |
onBefore | (params: P) => void | Before execution callback |
resultInterceptor | (data: R ) => any | If service is sucess, use this interceptor to throw logic error |
errorInterceptor | (error: Error) => Error | Error interceptor |
formatResult | (data: R ) => any | Data format callback(data is resultInterceptor returned value), the return value will set to res.data |
onSuccess | (data:R, params: P) => void | Success callback |
onError | (error: Error, params: P) => void | Error callback |
onAfter | (data: R , error: Error , params: P) | After callback, nomatter success or error |
defaultAxios | string or AxiosRequestConfig | Default global axios name or a specific AxiosRequestConfig |
defaultOptions | string or ExecuteOptions | Default global ExecuteOptions name or a specific settings |
Key | DataType | Description |
---|---|---|
loading | Ref<boolean> | Loading flag |
data | Ref<R> | Result data |
error | Ref<Error> | Error data |
params | Ref<any[]> | Last run parameters |
run | (...args: P) => Promise<R> | Run request with new parameters |
cancel | () => void | Cancel request |
refresh | () => Promise<R> | Run request wit last parameters |
mutate | (newdata: R) => void | Change result data |
Function | Description |
---|---|
useRequest(promise) | Execute a Promise function |
useAxiosRequest(options, axios) | Create a request instance with preset options and axios |
useAxiosRequest().get(url, params, options) | A wapper for axios.get() with options |
useAxiosRequest().post(url, params, options) | A wapper for axios.post() with options |
useAxiosRequest().delete(url, params, options) | A wapper for axios.delete() with options |
useAxiosRequest().put(url, params, options) | A wapper for axios.put() with options |
useAxiosRequest().patch(url, params, options) | A wapper for axios.patch() with options |
useAxiosRequest().head(url, params, options) | A wapper for axios.head() with options |
useAxiosRequest().request(params, options) | A wapper for axios.request() with options |
useAxiosGet(url, params, options) | A quick usage of useAxiosRequest().get(url, params, options) |
useAxiosPost(url, params, options) | A quick usage of useAxiosRequest().post(url, params, options) |
useAxiosDelete(url, params, options) | A quick usage of useAxiosRequest().delete(url, params, options) |
useAxiosPut(url, params, options) | A quick usage of useAxiosRequest().put(url, params, options) |
useAxiosPatch(url, params, options) | A quick usage of useAxiosRequest().patch(url, params, options) |
useAxiosHead(url, params, options) | A quick usage of useAxiosRequest().head(url, params, options) |
useAxios(name) | Create or get a global AxiosInstance |
useExecuteOptions(name) | Create or get a global ExecuteOption |
allAxiosInstance() | Return all global AxiosInstance |
allExecuteOptions() | Return all global ExecuteOptions |
run()
return type from Promise<void>
to Promise<R>
ExecuteOptions.errorInterceptor
ExecuteOptions.resultInterceptor
.post(url, data, options)
to .post(url, param, options)
.ESNext
to ES2015
.package.json
useOptions
to useExecuteOptions
, make it's meaning clearer.FAQs
A wrapper for Axios with Vue3 componsition API, It's easy to use and support polling, retry, cache, debounce, throttle...
The npm package @toplinker/vue3-axios-request receives a total of 3 weekly downloads. As such, @toplinker/vue3-axios-request popularity was classified as not popular.
We found that @toplinker/vue3-axios-request demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.