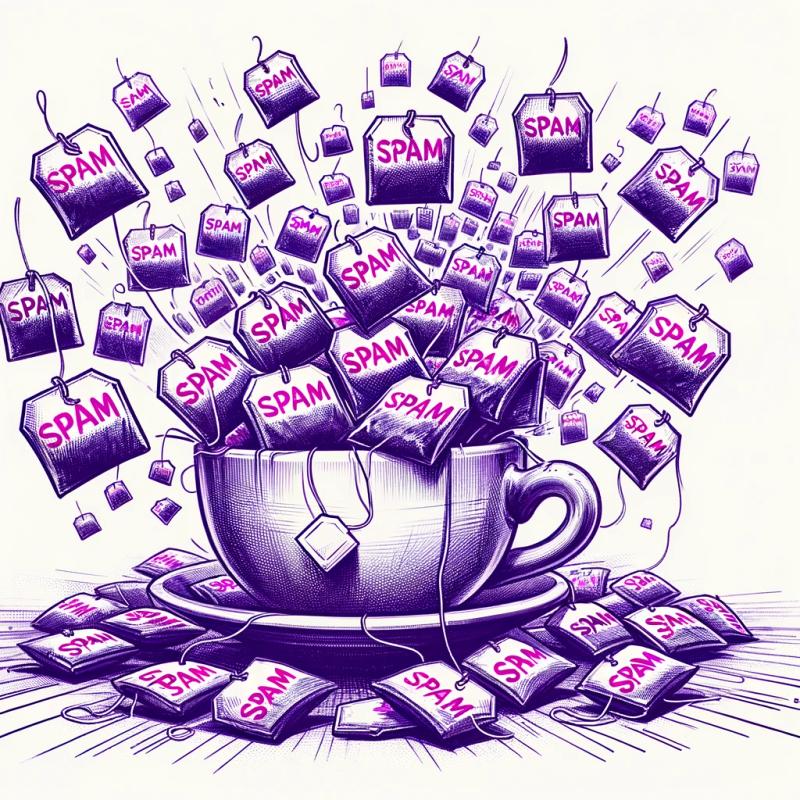
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@types/busboy
Advanced tools
Readme
npm install --save @types/busboy
This package contains type definitions for busboy (https://github.com/mscdex/busboy).
Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/busboy.
These definitions were written by Jacob Baskin, BendingBender, and Martin Badin.
FAQs
TypeScript definitions for busboy
The npm package @types/busboy receives a total of 374,603 weekly downloads. As such, @types/busboy popularity was classified as popular.
We found that @types/busboy demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.