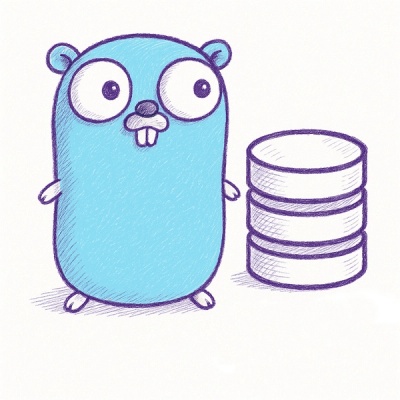
Security News
Official Go SDK for MCP in Development, Stable Release Expected in August
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.
@types/react-grid-layout
Advanced tools
TypeScript definitions for react-grid-layout
@types/react-grid-layout provides TypeScript definitions for the react-grid-layout library, which is a powerful and flexible grid layout system for React applications. It allows developers to create responsive, draggable, and resizable grid layouts with ease.
Creating a Basic Grid Layout
This code demonstrates how to create a basic grid layout using react-grid-layout. The layout array defines the position and size of each grid item.
import React from 'react';
import GridLayout from 'react-grid-layout';
const BasicGrid = () => {
const layout = [
{ i: 'a', x: 0, y: 0, w: 1, h: 2 },
{ i: 'b', x: 1, y: 0, w: 3, h: 2 },
{ i: 'c', x: 4, y: 0, w: 1, h: 2 }
];
return (
<GridLayout className="layout" layout={layout} cols={12} rowHeight={30} width={1200}>
<div key="a">A</div>
<div key="b">B</div>
<div key="c">C</div>
</GridLayout>
);
};
export default BasicGrid;
Draggable and Resizable Grid Items
This code shows how to make grid items draggable and resizable. The isDraggable and isResizable properties are set to true for each grid item.
import React from 'react';
import GridLayout from 'react-grid-layout';
const DraggableResizableGrid = () => {
const layout = [
{ i: 'a', x: 0, y: 0, w: 1, h: 2, isDraggable: true, isResizable: true },
{ i: 'b', x: 1, y: 0, w: 3, h: 2, isDraggable: true, isResizable: true },
{ i: 'c', x: 4, y: 0, w: 1, h: 2, isDraggable: true, isResizable: true }
];
return (
<GridLayout className="layout" layout={layout} cols={12} rowHeight={30} width={1200}>
<div key="a">A</div>
<div key="b">B</div>
<div key="c">C</div>
</GridLayout>
);
};
export default DraggableResizableGrid;
Responsive Grid Layout
This code demonstrates how to create a responsive grid layout that adapts to different screen sizes. The layouts object defines the layout for different breakpoints.
import React from 'react';
import { Responsive, WidthProvider } from 'react-grid-layout';
const ResponsiveGridLayout = WidthProvider(Responsive);
const ResponsiveGrid = () => {
const layouts = {
lg: [
{ i: 'a', x: 0, y: 0, w: 1, h: 2 },
{ i: 'b', x: 1, y: 0, w: 3, h: 2 },
{ i: 'c', x: 4, y: 0, w: 1, h: 2 }
],
md: [
{ i: 'a', x: 0, y: 0, w: 1, h: 2 },
{ i: 'b', x: 1, y: 0, w: 2, h: 2 },
{ i: 'c', x: 3, y: 0, w: 1, h: 2 }
]
};
return (
<ResponsiveGridLayout className="layout" layouts={layouts} breakpoints={{ lg: 1200, md: 996 }} cols={{ lg: 12, md: 10 }}>
<div key="a">A</div>
<div key="b">B</div>
<div key="c">C</div>
</ResponsiveGridLayout>
);
};
export default ResponsiveGrid;
react-grid-system is a responsive grid layout system for React that provides a simple and flexible way to create responsive layouts. It is similar to react-grid-layout but focuses more on simplicity and ease of use, with less emphasis on advanced features like drag-and-drop and resizing.
react-masonry-component is a React wrapper for the Masonry layout library. It allows for the creation of dynamic, grid-based layouts with a focus on positioning elements in an optimal, space-efficient manner. Unlike react-grid-layout, it does not support drag-and-drop or resizing but excels in creating visually appealing, Pinterest-like layouts.
react-flexbox-grid is a grid system based on the flexbox layout model. It provides a set of React components to create responsive grid layouts using flexbox. While it does not offer drag-and-drop or resizing capabilities, it is a lightweight and easy-to-use alternative for creating flexible and responsive layouts.
npm install --save @types/react-grid-layout
This package contains type definitions for react-grid-layout (https://github.com/STRML/react-grid-layout).
Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/react-grid-layout.
These definitions were written by Andrew Birkholz, Ali Taheri, Zheyang Song, Andrew Hathaway, Manav Mishra, and Alexey Fyodorov.
FAQs
TypeScript definitions for react-grid-layout
The npm package @types/react-grid-layout receives a total of 501,657 weekly downloads. As such, @types/react-grid-layout popularity was classified as popular.
We found that @types/react-grid-layout demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.
Security News
New research reveals that LLMs often fake understanding, passing benchmarks but failing to apply concepts or stay internally consistent.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.