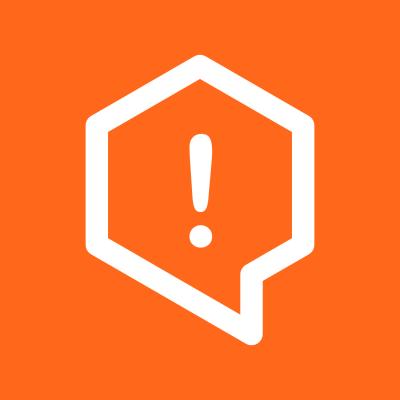
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
@types/seedrandom
Advanced tools
TypeScript definitions for seedrandom
@types/seedrandom is a TypeScript type definition package for the seedrandom library, which is used to create seeded random number generators. This allows for reproducible random number sequences, which can be useful in simulations, games, and other applications where predictable randomness is needed.
Creating a Seeded Random Number Generator
This feature allows you to create a random number generator that produces the same sequence of numbers each time it is initialized with the same seed. This is useful for reproducibility in tests and simulations.
const seedrandom = require('seedrandom');
const rng = seedrandom('my-seed');
console.log(rng());
Using Different Algorithms
Seedrandom supports different algorithms for generating random numbers. This example shows how to use the 'alea' algorithm.
const seedrandom = require('seedrandom');
const rng = seedrandom('my-seed', { algorithm: 'alea' });
console.log(rng());
Generating Random Numbers in a Range
This feature demonstrates how to generate random numbers within a specific range using a seeded random number generator.
const seedrandom = require('seedrandom');
const rng = seedrandom('my-seed');
const randomInRange = (min, max) => min + rng() * (max - min);
console.log(randomInRange(1, 10));
random-seed is another library that provides seeded random number generation. It is simpler and has fewer features compared to seedrandom, but it is still useful for basic seeded random number generation.
chance is a library that provides a wide range of random data generators, including seeded random number generation. It offers more functionality than seedrandom, such as generating random names, addresses, and other data types.
random-js is a library that provides a variety of random number generators, including seeded generators. It offers more control over the random number generation process and supports multiple algorithms.
npm install --save @types/seedrandom
This package contains type definitions for seedrandom (https://github.com/davidbau/seedrandom).
Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/seedrandom.
These definitions were written by Kern Handa, Eugene Zaretskiy, Martin Badin, and Martijn van der Ven.
FAQs
TypeScript definitions for seedrandom
The npm package @types/seedrandom receives a total of 461,269 weekly downloads. As such, @types/seedrandom popularity was classified as popular.
We found that @types/seedrandom demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.