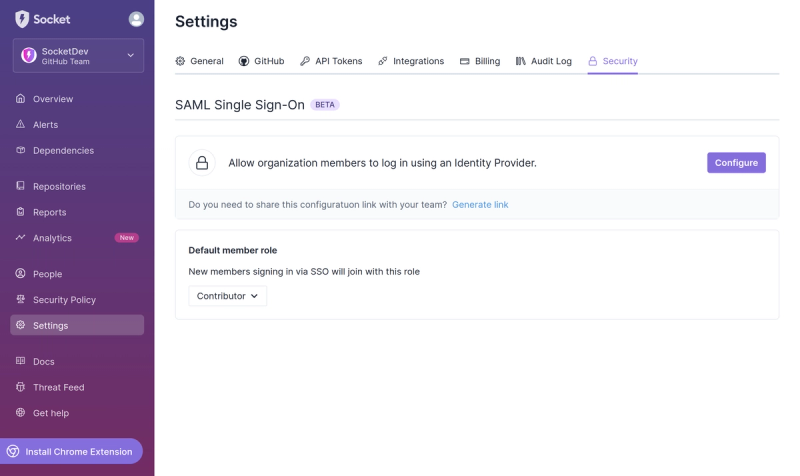
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
@types/which
Advanced tools
Readme
npm install --save @types/which
This package contains type definitions for which (https://github.com/isaacs/node-which).
Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/which.
/** Finds all instances of a specified executable in the PATH environment variable */
declare function which(cmd: string, options: which.Options & which.AsyncOptions & which.OptionsAll): Promise<string[]>;
declare function which(cmd: string, options?: which.Options & which.AsyncOptions & which.OptionsFirst): Promise<string>;
declare namespace which {
/** Finds all instances of a specified executable in the PATH environment variable */
function sync(cmd: string, options: Options & OptionsAll & OptionsNoThrow): readonly string[] | null;
function sync(cmd: string, options: Options & OptionsFirst & OptionsNoThrow): string | null;
function sync(cmd: string, options: Options & OptionsAll & OptionsThrow): readonly string[];
function sync(cmd: string, options?: Options & OptionsFirst & OptionsThrow): string;
function sync(cmd: string, options: Options): string | readonly string[] | null;
/** Options that ask for all matches. */
interface OptionsAll extends AsyncOptions {
all: true;
}
/** Options that ask for the first match (the default behavior) */
interface OptionsFirst extends AsyncOptions {
all?: false | undefined;
}
/** Options that ask to receive null instead of a thrown error */
interface OptionsNoThrow extends Options {
nothrow: true;
}
/** Options that ask for a thrown error if executable is not found (the default behavior) */
interface OptionsThrow extends Options {
nothrow?: false | undefined;
}
/** Options for which() async API */
interface AsyncOptions {
/** If true, return all matches, instead of just the first one. Note that this means the function returns an array of strings instead of a single string. */
all?: boolean | undefined;
/** Use instead of the PATH environment variable. */
path?: string | undefined;
/** Use instead of the PATHEXT environment variable. */
pathExt?: string | undefined;
}
/** Options for which() sync and async APIs */
interface Options extends AsyncOptions {
/** If true, returns null when not found */
nothrow?: boolean | undefined;
}
}
export = which;
These definitions were written by vvakame, cspotcode, and Piotr Błażejewicz.
FAQs
TypeScript definitions for which
The npm package @types/which receives a total of 953,491 weekly downloads. As such, @types/which popularity was classified as popular.
We found that @types/which demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.