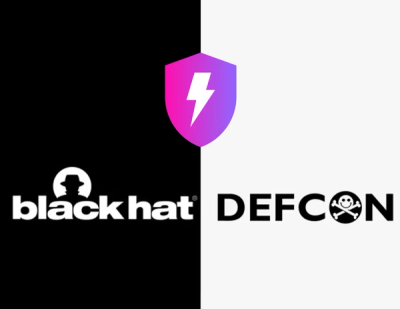
Security News
Meet Socket at Black Hat and DEF CON 2025 in Las Vegas
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
@ucast/mongo
Advanced tools
@ucast/mongo is a package that allows you to apply conditions and filters to MongoDB queries using a unified and flexible syntax. It is part of the UCAS (Universal Conditions and Specifications) library, which provides a way to define and evaluate conditions in a consistent manner across different platforms and databases.
Creating Conditions
This feature allows you to create complex conditions using MongoDB's query syntax. The example demonstrates how to create a condition that checks if the age is greater than 18 and the name matches a regex pattern.
const { createCondition } = require('@ucast/mongo');
const condition = createCondition({
$and: [
{ age: { $gt: 18 } },
{ name: { $regex: 'John' } }
]
});
console.log(condition);
Evaluating Conditions
This feature allows you to evaluate conditions against a given data object. The example shows how to evaluate if the age in the data object is greater than 18.
const { evaluateCondition } = require('@ucast/mongo');
const condition = { age: { $gt: 18 } };
const data = { age: 20 };
const result = evaluateCondition(condition, data);
console.log(result); // true
Combining Conditions
This feature allows you to combine multiple conditions using logical operators like $and, $or, etc. The example demonstrates how to combine two conditions using the $and operator.
const { combineConditions } = require('@ucast/mongo');
const condition1 = { age: { $gt: 18 } };
const condition2 = { name: { $regex: 'John' } };
const combinedCondition = combineConditions('$and', [condition1, condition2]);
console.log(combinedCondition);
Mongoose is an ODM (Object Data Modeling) library for MongoDB and Node.js. It provides a higher-level abstraction for interacting with MongoDB, including schema definitions, model validation, and query building. Unlike @ucast/mongo, which focuses on condition creation and evaluation, Mongoose offers a full suite of tools for working with MongoDB in a more structured way.
The official MongoDB driver for Node.js provides a low-level API for interacting with MongoDB databases. It allows you to perform CRUD operations, manage indexes, and run aggregation pipelines. While it offers more control and flexibility, it lacks the higher-level condition creation and evaluation features provided by @ucast/mongo.
json-rules-engine is a powerful, lightweight rules engine for Node.js. It allows you to define and evaluate complex business rules in JSON format. While it is not specific to MongoDB, it offers similar functionality in terms of condition evaluation and can be used in a broader range of applications.
This package is a part of ucast ecosystem. It provides a parser that can parse MongoDB query into conditions AST.
npm i @ucast/mongo
# or
yarn add @ucast/mongo
# or
pnpm add @ucast/mongo
To parse MongoDB query into conditions AST, you need to create a MongoQueryParser
instance:
import { MongoQueryParser, allParsingInstructions } from '@ucast/mongo';
const parser = new MongoQueryParser(allParsingInstructions);
const ast = parser.parse({
id: 1,
active: true
});
To create a parser you need to pass in it parsing instruction. Parsing instruction is an object that defines how a particular operator should be parsed. There are 3 types of ParsingInstruction
, one for each AST node type:
field
represents an instruction for an operator which operates in a field context only. For example, operators $eq
, $lt
, $not
, $regex
compound
represents an instruction for an operator that aggregates nested queries. For example, operators $and
, $or
, $nor
document
represents an instruction for an operator which operates in a document context only. For example, $where
or $jsonSchema
It's important to understand that it's not required that parsing instruction with type field
should be parsed into FieldCondition
. It can be parsed into CompoundCondition
as it's done for $not
operator.
A parsing instruction is an object of 3 fields:
const parsingInstruction = {
type: 'field' | 'document' | 'compound',
validate?(instruction, value) { // optional
// throw exception if something is wrong
},
parse?(instruction, schema, context) { // optional
/*
* custom logic to parse operator,
* returns FieldCondition | DocumentCondition | CompoundCondition
*/
}
}
Some operators like $and
and $or
optimize their parsing logic, so if one of that operators contain a single condition it will be resolved to that condition without additional wrapping. They also recursively collapse conditions from nested operators with the same name. Let's see an example to understand what this means:
const ast = parser.parse({
a: 1
$and: [
{ b: 2 },
{ c: 3 }
]
});
console.dir(ast, { depth: null })
/*
CompoundCondition {
operator: "and",
value: [
FieldCondition { operator: "eq", field: "a", value: 1 },
FieldCondition { operator: "eq", field: "b", value: 2 },
FieldCondition { operator: "eq", field: "c", value: 3 },
]
}
*/
This optimization logic helps to speed up interpreter's execution time, instead of going deeply over tree-like structure we have a plain structure of all conditions under a single compound condition.
Pay attention: parser removes $
prefix from operator names
In order for an operator to be parsed, it needs to define a parsing instruction. Let's implement a custom instruction which checks that object corresponds to a particular json schema.
First of all, we need to understand on which level this operator operates (field or document). In this case, $jsonSchema
clearly operates on document level. It doesn't contain nested MongoDB queries, so it's not a compound
instruction. So, we are left only with document
one.
To test that document corresponds to provided json schema, we use ajv but it's also possible to use a library of your preference.
// operators/jsonSchema.js
import { DocumentInstruction, DocumentCondition } from '@ucast/core';
import Ajv from 'ajv';
export const $jsonSchema: DocumentInstruction = {
type: 'document',
validate(instruction, value) {
if (!value || typeof value !== 'object') {
throw new Error(`"${instruction.name}" expects to receive an object`)
}
},
parse(instruction, schema) {
const ajv = new Ajv();
return new DocumentCondition(instruction.name, ajv.compile(schema));
}
};
In order to use this operator, we need to pass this instruction into MongoQueryParser
constructor:
import { MongoQueryParser, allParsingInstructions } from '@ucast/core';
import { $jsonSchema } from './operators/jsonSchema';
const parser = new MongoQueryParser({
...allParsingInstructions,
$jsonSchema
});
const ast = parser.parse({
$jsonSchema: {
type: 'object',
properties: {
firstName: { type: 'string' },
lastName: { type: 'string' },
},
additionalProperties: false,
}
});
console.dir(ast, { depth: null });
/*
DocumentCondition { operator: "jsonSchema", value: [Function: validate] }
*/
The only thing which is left is to implement a corresponding JavaScript interpreter:
function jsonSchema(condition, object) { // interpreter
return condition.value(object);
}
Want to file a bug, contribute some code, or improve documentation? Excellent! Read up on guidelines for contributing
FAQs
git@github.com:stalniy/ucast.git
The npm package @ucast/mongo receives a total of 590,489 weekly downloads. As such, @ucast/mongo popularity was classified as popular.
We found that @ucast/mongo demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.