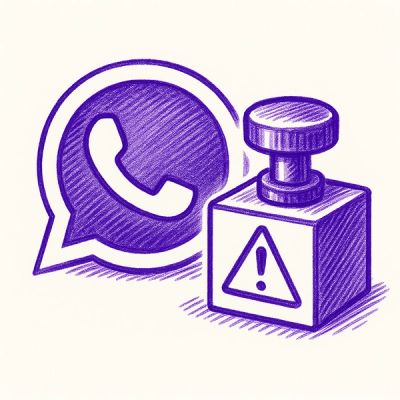
Research
/Security News
Malicious npm Packages Target WhatsApp Developers with Remote Kill Switch
Two npm packages masquerading as WhatsApp developer libraries include a kill switch that deletes all files if the phone number isn’t whitelisted.
@unifig/core
Advanced tools
Unifig aims to provides simple and abstract way of handling app's configuration. It allows to load configuration data from multiple sources without changing defined config template. Many templates can be defined to further organize the code eg. MainConfiguration and ModuleConfiguration.
Adapted configuration data is transformed into templates and validated via class-transformer and class-validator. Once initialized configurations can be reloaded without app restart.
npm i @unifig/core
# or
yarn add @unifig/core
Unifig centralizes configuration management in classes called templates. They are resposible for deserializing config values from sources into rich js / ts objects and validating them afterwards.
import { From, Nested } from '@unifig/core';
import { Transform } from 'class-transformer';
import { IsString, IsArray } from 'class-validator';
class DbSettings {
@From({ key: 'DB_URL', default: 'localhost' })
@IsString()
url: string;
@From('DB_PASSWORD')
@IsString()
password: string;
@From('DB_RECONNECT_DELAYS')
@Transform(({ value }) => value.split(',').map((n) => Number(n)))
@IsArray()
reconnectDelays: number[];
}
Allows to keep config structure organized by grouping inseparable properties and allowing reusage of them. The subtemplate itself is declared just as regular template, including option to nest further subtemplates in it.
export class AppSettings {
@From('PORT')
@IsInt()
port: number;
@Nested(() => DbSettings)
db: DbSettings;
}
Templates should be loaded before any other action in the application takes place. After that configuration can be accessed from any place in the app via global Config
reference.
import { Config, PlainConfigAdapter } from '@unifig/core';
async function bootstrap() {
const validationError = await Config.register({
template: AppSettings,
adapter: async () => ({
PORT: 3000,
DB_URL: 'localhost:5467',
DB_PASSWORD: 'password',
DB_RECONNECT_DELAYS: '56,98,34,72',
}),
});
if (validationError) {
console.error(validationError.message);
process.exit(1);
}
const options = Config.getValues(AppSettings);
console.log(options.port); // output: 3000
console.log(options.db.url); // output: localhost:5467
}
bootstrap();
Unifig allows to easily swap config values sources or introduce new ones.
Implementation of the adapter consist of class which exposes load
method, which is called upon config initialization. The method should return dictionary with keys used in @From
decorators in templates and underlying values.
import { ConfigAdapter, ConfigSource } from '@unifig/core';
export class CustomAdapter implements ConfigSyncAdapter {
load(): ConfigSource {
return {
PORT: '3000', // will be parsed to number as declared in template
DB_URL: 'localhost:5467',
DB_PASSWORD: 'password',
DB_RECONNECT_DELAYS: '56,98,34,72',
};
}
}
Config.registerSync({
template: AppSettings,
adapter: new CustomAdapter(),
});
In case of asynchronous way of loading config (like cloud remote configuration service) the adapter needs to implement ConfigAdapter
interface.
import { ConfigAdapter, ConfigSource } from '@unifig/core';
export class RemoteConfigAdapter implements ConfigAdapter {
async load(): Promise<ConfigSource> {
return { ... };
}
}
Such adapter requires to be used by async register
method.
await Config.register({
template: AppSettings,
adapter: new RemoteConfigAdapter(),
});
See full list of adapters here.
Alternatively adapter can be defined as standalone sync or async function with same rules applied.
Config.registerSync({
template: AppSettings,
adapter: () => ({
PORT: '3000',
DB_URL: 'localhost:5467',
DB_PASSWORD: 'password',
DB_RECONNECT_DELAYS: '56,98,34,72',
}),
});
When loading configuration from predefined objects it's handy to disable the default behavior of implicit properties types conversion.
await Config.register({
template: AppSettings,
enableImplicitConversion: false,
adapter: new CustomAdapter(),
});
In case no single configuration root (AppSettings
in above example), templates need to be registered separately.
await Config.register(
{ template: DbSettings, adapter: ... },
{ template: AuthSettings, adapter: ... },
{ templates: [FilesStorageSettings, CacheSettings], adapter: ... },
);
Nested config values can be accessed directly after parent initialization.
class DbConfig {
@IsString()
url: string;
}
class AppConfig {
@IsInt()
port: number;
@Nested(() => DbConfig)
db: DbConfig;
}
Config.registerSync({
template: AppConfig,
adapter: () => ({ port: 3000, db: { url: 'db://localhost:5467' } }),
});
console.log('Db url: ' + Config.getValues(DbConfig).url); // => Db url: db://localhost:5467
To throw validation exception right away after encountering errors instead of returning it use
await Config.registerOrReject({ template: DbSettings, adapter: ... });
Upon changing application's configuration one must be usually restarted to re-fetch new values. Unifig delivers an option to reload registered configurations in real time without app's restart.
await Config.getContainer(Settings).refresh();
Template errors can be handled in various ways, usually exiting the application early to prevent unexpected behavior.
const validationError = Config.registerSync({ ... });
if (validationError) {
console.error(validationError.message);
process.exit(1);
}
Message contains list of templates names that failed validation. The errors object contains details about what and why doesn't fullfil requirements. Presenters are to utilize this information in a readable manner.
Validation report involves properties values that didn't pass validation. In some cases it's required to hide them. For such cases there is a Secret
decorator.
export class DbConfigMock {
@From('DB_URL')
@IsString()
url: string;
@From('DB_PASSWORD')
@Secret()
@IsString()
password: string;
}
With it applied, password
value will be transformed into ******
in potential validation report.
This project is licensed under the MIT License - see the LICENSE file for details.
FAQs
Universal, typed and validated configuration manager
The npm package @unifig/core receives a total of 1,385 weekly downloads. As such, @unifig/core popularity was classified as popular.
We found that @unifig/core demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
Two npm packages masquerading as WhatsApp developer libraries include a kill switch that deletes all files if the phone number isn’t whitelisted.
Research
/Security News
Socket uncovered 11 malicious Go packages using obfuscated loaders to fetch and execute second-stage payloads via C2 domains.
Security News
TC39 advances 11 JavaScript proposals, with two moving to Stage 4, bringing better math, binary APIs, and more features one step closer to the ECMAScript spec.