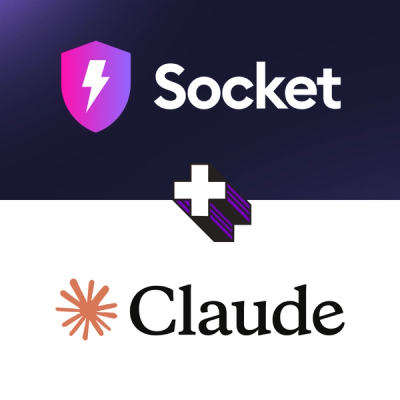
Product
Introducing Socket MCP for Claude Desktop
Add secure dependency scanning to Claude Desktop with Socket MCP, a one-click extension that keeps your coding conversations safe from malicious packages.
@upstash/redis
Advanced tools
An HTTP/REST based Redis client built on top of Upstash REST API.
@upstash/redis is an npm package that provides a serverless Redis solution. It allows developers to interact with a Redis database using a simple and efficient API. The package is designed to work seamlessly with serverless environments, making it ideal for modern cloud-based applications.
Basic Redis Commands
This feature allows you to perform basic Redis commands such as SET and GET. The code sample demonstrates how to set a key-value pair and retrieve it.
const { Redis } = require('@upstash/redis');
const redis = new Redis({ url: 'your-upstash-redis-url', token: 'your-upstash-token' });
async function run() {
await redis.set('key', 'value');
const value = await redis.get('key');
console.log(value); // Output: 'value'
}
run();
Pub/Sub
This feature allows you to use the Pub/Sub (publish/subscribe) pattern. The code sample demonstrates how to subscribe to a channel and publish a message to it.
const { Redis } = require('@upstash/redis');
const redis = new Redis({ url: 'your-upstash-redis-url', token: 'your-upstash-token' });
async function run() {
const subscriber = redis.duplicate();
await subscriber.subscribe('channel', (message) => {
console.log('Received message:', message);
});
await redis.publish('channel', 'Hello, World!');
}
run();
Transactions
This feature allows you to perform transactions in Redis. The code sample demonstrates how to use the MULTI and EXEC commands to execute multiple commands atomically.
const { Redis } = require('@upstash/redis');
const redis = new Redis({ url: 'your-upstash-redis-url', token: 'your-upstash-token' });
async function run() {
const transaction = redis.multi();
transaction.set('key1', 'value1');
transaction.set('key2', 'value2');
const results = await transaction.exec();
console.log(results); // Output: [ 'OK', 'OK' ]
}
run();
ioredis is a robust, full-featured Redis client for Node.js. It supports all Redis commands, including Pub/Sub and transactions, and provides high availability and cluster support. Compared to @upstash/redis, ioredis is more suitable for traditional server-based environments rather than serverless architectures.
redis is the official Redis client for Node.js. It provides a straightforward API for interacting with a Redis database and supports all standard Redis commands. While it is highly reliable and widely used, it does not offer the serverless-specific optimizations that @upstash/redis provides.
node-redis is another popular Redis client for Node.js. It offers a simple and efficient API for performing Redis operations and supports features like Pub/Sub and transactions. Similar to the official redis package, it is designed for traditional server environments rather than serverless setups.
@upstash/redis
is an HTTP/REST based Redis client for typescript, built on top
of Upstash REST API.
[!NOTE]
This project is in GA Stage.The Upstash Professional Support fully covers this project. It receives regular updates, and bug fixes. The Upstash team is committed to maintaining and improving its functionality.
It is the only connectionless (HTTP based) Redis client and designed for:
See the list of APIs supported.
npm install @upstash/redis
import { Redis } from "https://esm.sh/@upstash/redis";
Create a new redis database on upstash
import { Redis } from "@upstash/redis"
const redis = new Redis({
url: <UPSTASH_REDIS_REST_URL>,
token: <UPSTASH_REDIS_REST_TOKEN>,
})
// string
await redis.set('key', 'value');
let data = await redis.get('key');
console.log(data)
await redis.set('key3', 'value3', {ex: 1});
// sorted set
await redis.zadd('scores', { score: 1, member: 'team1' })
data = await redis.zrange('scores', 0, 100 )
console.log(data)
// list
await redis.lpush('elements', 'magnesium')
data = await redis.lrange('elements', 0, 100 )
console.log(data)
// hash
await redis.hset('people', {name: 'joe'})
data = await redis.hget('people', 'name' )
console.log(data)
// sets
await redis.sadd('animals', 'cat')
data = await redis.spop('animals', 1)
console.log(data)
We have a dedicated page for common problems. If you can't find a solution, please open an issue.
See the documentation for details.
Create a new redis database on upstash and copy the url and token
bun run test
bun run build
This library sends anonymous telemetry data to help us improve your experience. We collect the following:
You can opt out by setting the UPSTASH_DISABLE_TELEMETRY
environment variable
to any truthy value.
UPSTASH_DISABLE_TELEMETRY=1
FAQs
An HTTP/REST based Redis client built on top of Upstash REST API.
The npm package @upstash/redis receives a total of 482,531 weekly downloads. As such, @upstash/redis popularity was classified as popular.
We found that @upstash/redis demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Add secure dependency scanning to Claude Desktop with Socket MCP, a one-click extension that keeps your coding conversations safe from malicious packages.
Product
Socket now supports Scala and Kotlin, bringing AI-powered threat detection to JVM projects with easy manifest generation and fast, accurate scans.
Application Security
/Security News
Socket CEO Feross Aboukhadijeh and a16z partner Joel de la Garza discuss vibe coding, AI-driven software development, and how the rise of LLMs, despite their risks, still points toward a more secure and innovative future.