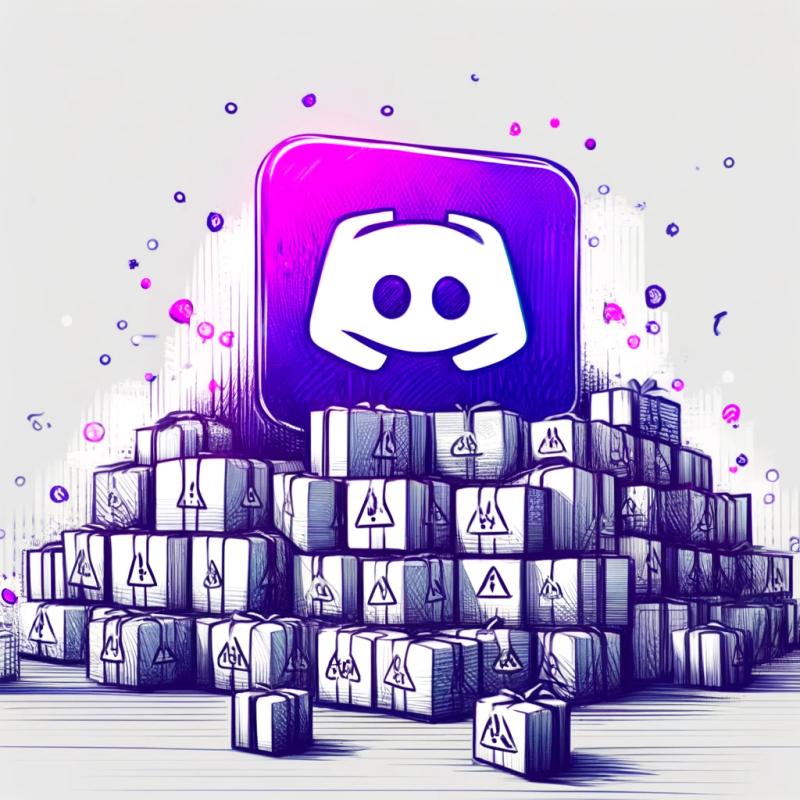
Research
Recent Trends in Malicious Packages Targeting Discord
The Socket research team breaks down a sampling of malicious packages that download and execute files, among other suspicious behaviors, targeting the popular Discord platform.
@windmillcode/angular-wml-notify
Advanced tools
The `wml-notify` library is an Angular-based toolkit designed to enhance user notifications and messaging within applications. Its primary objective is to offer a streamlined and efficient way for developers to integrate and manage notifications, alerts,
Readme
The wml-notify
library is an Angular-based toolkit designed to enhance user notifications and messaging within applications. Its primary objective is to offer a streamlined and efficient way for developers to integrate and manage notifications, alerts, and informational messages in their Angular projects. The library provides a set of components and services that enable the display of various types of messages, such as errors, information, success, and warnings, with customizable options to cater to different needs and scenarios. It leverages Angular's powerful features to create a responsive and interactive user experience, addressing common challenges in handling user notifications with ease and precision.
At the heart of the wml-notify
library are two central components: WmlNotifyComponent
and WmlNotifyMsgComponent
. WmlNotifyComponent
acts as a container for individual notifications, managing their display and behavior, including auto-hiding, user-initiated closure, and actions. It integrates with the WmlNotifyService
to listen for notification events and render them appropriately. WmlNotifyMsgComponent
, on the other hand, is responsible for rendering the message content, supporting both default text messages and custom Angular components for more dynamic and interactive content. Developers can customize notifications using various attributes and methods provided by these components, such as message types, auto-hide functionality, and custom action handlers. The library encourages a modular approach, allowing developers to leverage these components either independently or together, facilitating a versatile implementation that aligns with the specific requirements of their Angular applications.
npm install -d @windmillcode/angular-wml-notify
To effectively utilize the wml-notify
library in your Angular applications, you can follow the examples below which demonstrate various use cases catering to different developer needs. These examples show how to integrate WmlNotifyComponent
and WmlNotifyMsgComponent
within your Angular project.
<wml-notify></wml-notify>
import { Component } from '@angular/core';
import { WmlNotifyService, WmlNotifyBarModel, WmlNotifyBarType } from '@windmillcode/angular-wml-notify';
@Component({
selector: 'app-your-component',
templateUrl: './your-component.component.html',
})
export class YourComponent {
constructor(private notifyService: WmlNotifyService) {}
showNotification() {
const notification = new WmlNotifyBarModel({
message: 'This is an info message!',
type: WmlNotifyBarType.Info,
});
this.notifyService.create(notification);
}
}
<wml-notify></wml-notify>
import { Component } from '@angular/core';
import { WmlNotifyService, WmlNotifyBarModel, WmlNotifyBarType, WMLCustomComponent } from '@windmillcode/angular-wml-notify';
@Component({
selector: 'app-your-component',
templateUrl: './your-component.component.html',
})
export class YourComponent {
constructor(private notifyService: WmlNotifyService) {}
showCustomNotification() {
const customNotification = new WmlNotifyBarModel({
message: 'This is a custom message!',
type: WmlNotifyBarType.Success,
msgtype: 'custom',
custom: new WMLCustomComponent({
cpnt: YourCustomComponent,
params: { /* your custom params */ },
}),
});
this.notifyService.create(customNotification);
}
}
<wml-notify (action)="onAction($event)" (closed)="onClosed($event)"></wml-notify>
import { Component } from '@angular/core';
import { WmlNotifyService, WmlNotifyBarModel, WmlNotifyBarType } from '@windmillcode/angular-wml-notify';
@Component({
selector: 'app-your-component',
templateUrl: './your-component.component.html',
})
export class YourComponent {
constructor(private notifyService: WmlNotifyService) {}
showActionNotification() {
const actionNotification = new WmlNotifyBarModel({
message: 'This message needs your action',
type: WmlNotifyBarType.Warning,
action: true,
actionText: 'Retry',
});
this.notifyService.create(actionNotification);
}
onAction(notification: WmlNotifyBarModel) {
console.log('Action clicked for notification:', notification);
}
onClosed(notification: WmlNotifyBarModel) {
console.log('Notification closed:', notification);
}
}
These examples demonstrate basic implementations of wml-notify
for various scenarios, providing a foundational understanding of how to integrate and customize the library within your Angular applications.
WmlNotifyBarModel
PropertiesProperty | Type | Description |
---|---|---|
message | string | The message to be displayed in the notification. |
type | WmlNotifyBarType | The type of the notification (Error, Info, Success, Warning). |
action | boolean | Indicates if the notification includes an action button. |
actionText | string | Text to display on the action button. |
autoHide | boolean | Determines if the notification should auto-hide. |
closed | boolean | Indicates if the notification is closed. |
closeable | boolean | Determines if the notification can be manually closed by the user. |
hideDelay | number | Time in milliseconds before the notification auto-hides. |
hideOnHover | boolean | Determines if the notification should hide when hovered. |
style | CSSStyleDeclaration | Styles to be applied to the notification bar. |
msgtype | string | Defines the message type (default or custom). |
custom | WMLCustomComponent | Custom component to be used as the notification content. |
WmlNotifyService
MethodsMethod | Parameters | Description |
---|---|---|
create | notification: WmlNotifyBarModel | Creates and displays a new notification. |
clear | None | Clears all displayed notifications. |
WmlNotifyComponent
EventsEvent | Parameters | Description |
---|---|---|
action | notification: WmlNotifyBarModel | Emitted when an action on a notification is triggered. |
closed | notification: WmlNotifyBarModel | Emitted when a notification is closed. |
WmlNotifyBarType
EnumerationValue | Description |
---|---|
Error | Represents an error notification. |
Info | Represents an informational notification. |
Success | Represents a success notification. |
Warning | Represents a warning notification. |
// translate
// first make sure to have ONLY ONE in the imports for AppModule
TranslateModule.forRoot({
defaultLanguage: 'en',
loader: {
provide: TranslateLoader,
useFactory: HttpLoaderFactory,
deps:[HttpClient]
}
}),
// then
WmlNotifyNGXTranslateModule
// for regular
WmlNotifyModule
%!(EXTRA string=
FAQs
The `wml-notify` library is an Angular-based toolkit designed to enhance user notifications and messaging within applications. Its primary objective is to offer a streamlined and efficient way for developers to integrate and manage notifications, alerts,
The npm package @windmillcode/angular-wml-notify receives a total of 54 weekly downloads. As such, @windmillcode/angular-wml-notify popularity was classified as not popular.
We found that @windmillcode/angular-wml-notify demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
The Socket research team breaks down a sampling of malicious packages that download and execute files, among other suspicious behaviors, targeting the popular Discord platform.
Security News
Socket CEO Feross Aboukhadijeh joins a16z partners to discuss how modern, sophisticated supply chain attacks require AI-driven defenses and explore the challenges and solutions in leveraging AI for threat detection early in the development life cycle.
Security News
NIST's new AI Risk Management Framework aims to enhance the security and reliability of generative AI systems and address the unique challenges of malicious AI exploits.