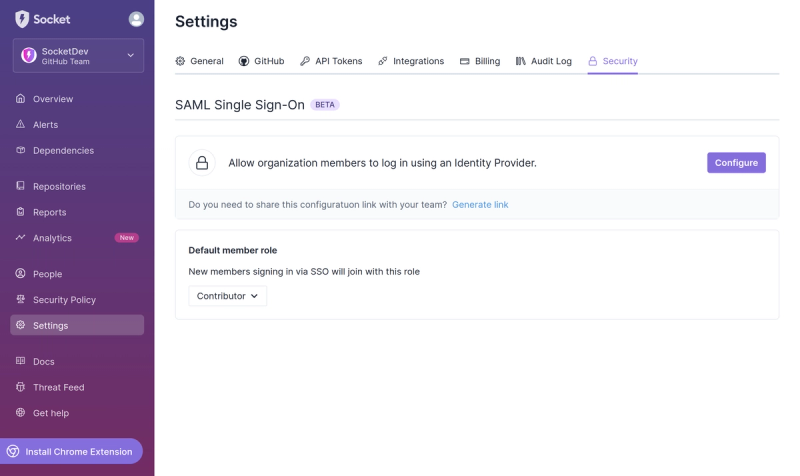
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
@xrpl/ai-web
Advanced tools
Readme
@xrpl/ai-web
A prebuilt version of the XRPL AI dialog, based on @xrplai/ai-react
, built with Preact for bundle-size savings. Viable for use from vanilla JavaScript or any framework.
# In the base folder
npm i
npm run build:packages
Now open ./webetest.html
in your web browser and begin interacting with the bot icon in the bottom right. This test is configured to hit the XRPL AI dev deployment.
Install the package from NPM:
npm add @xrpl/ai-web @xrpl/ai-css
Include the CSS on your page, via a link tag or by importing it in your JavaScript:
<!-- load from a CDN: -->
<!-- Replace with your local build of the css -->
<!-- <link rel="stylesheet" href="https://esm.sh/@markprompt/css@0.5.1?css" /> -->
import '@xrpl/ai-css';
Call the markprompt
function with your project key:
import { markprompt } from '@xrplai/ai-web';
const xrplaiEl = document.querySelector('#xrplai');
markprompt('YOUR-PROJECT-KEY', xrplaiEl, {
chat: {
apiUrl: 'https://api.yourdomain/v1/chat'
},
prompt: {
apiUrl: 'https://api.yourdomain/completions'
},
references: {
getHref: (reference) => reference.file.path.replace(/\.[^.]+$/, '');
getLabel: (reference) => {
return reference.meta?.leadHeading?.value || reference.file?.title;
}
},
});
where YOUR-PROJECT-KEY
can be obtained in your project settings on the XRPL AI Admin Backend.
Options are optional and allow you to configure the texts and links used in the
component to some extent. You will most likely want to pass references.getHref
and reference.getLabel
to transform your prompt references into links to your
corresponding documentation, and search.getHref
to transform search result
paths into links to your documentation.
import type {
SubmitFeedbackOptions,
SubmitPromptOptions,
SubmitSearchQueryOptions,
} from '@xrpl/ai-core';
interface MarkpromptOptions {
/**
* Display format.
* @default "dialog"
**/
display?: 'plain' | 'dialog';
/**
* Enable and configure search functionality.
* @default "search"
* */
defaultView?: 'search' | 'chat' | 'prompt';
close?: {
/**
* `aria-label` for the close modal button
* @default "Close Markprompt"
**/
label?: string;
/**
* Show the close button
* @default true
**/
visible?: boolean;
};
description?: {
/**
* Visually hide the description
* @default true
**/
hide?: boolean;
/**
* Description text
**/
text?: string;
};
feedback?: SubmitFeedbackOptions & {
/**
* Enable feedback functionality, shows a thumbs up/down button after a
* prompt was submitted.
* @default false
* */
enabled?: boolean;
/**
* Heading above the form
* @default "Was this response helpful?"
**/
heading?: string;
/**
* Called when feedback is submitted
* @default undefined
*/
onFeedbackSubmit?: (
feedback: PromptFeedback,
messages: ChatViewMessage[],
promptId?: string,
) => void;
};
/**
* Enable and configure chat functionality. Allows users to have a conversation with an assistant.
* Enabling chat functionality will disable prompt functionality.
*/
chat?: SubmitChatOptions & {
/**
* Show a chat-like prompt input allowing for conversation-style interaction
* rather than single question prompts.
* @default false
**/
enabled?: boolean;
/**
* Label for the chat input
* @default "Ask AI"
**/
label?: string;
/**
* Label for the tab bar
* @default "Ask AI"
**/
tabLabel?: string;
/**
* Placeholder for the chat input
* @default "Ask AI…"
**/
placeholder?: string;
/**
* Show sender info, like avatar
* @default true
**/
showSender?: boolean;
/**
* Enable chat history features
* - enable saving chat history to local storage
* - show chat history UI
* - resume chat conversations
* @default true
*/
history?: boolean;
};
/**
* Enable and configure prompt functionality. Allows users to ask a single question to an assistant
*/
prompt?: SubmitChatOptions & {
/**
* Label for the prompt input
* @default "Ask AI"
**/
label?: string;
/**
* Label for the tab bar
* @default "Ask AI"
**/
tabLabel?: string;
/**
* Placeholder for the prompt input
* @default "Ask AI…"
**/
placeholder?: string;
};
references?: {
/**
* Display mode for the references. References can either be
* displayed after the response or not displayed at all.
* @default 'end'
* */
display?: 'none' | 'end';
/** Callback to transform a reference into an href */
getHref?: (reference: FileSectionReference) => string | undefined;
/** Callback to transform a reference into a label */
getLabel?: (reference: FileSectionReference) => string | undefined;
/**
* Heading above the references
* @default "Answer generated from the following sources:"
**/
heading?: string;
/** Loading text, default: `Fetching relevant pages…` */
loadingText?: string;
/**
* Callback to transform a reference id into an href and text
* @deprecated Use `getHref` and `getLabel` instead
**/
transformReferenceId?: (referenceId: string) => {
href: string;
text: string;
};
};
/**
* Enable and configure search functionality
*/
search?: SubmitSearchQueryOptions & {
/**
* Enable search
* @default false
**/
enabled?: boolean;
/** Callback to transform a search result into an href */
getHref?: (
reference: SearchResult | AlgoliaDocSearchHit,
) => string | undefined;
/** Callback to transform a search result into a heading */
getHeading?: (
reference: SearchResult | AlgoliaDocSearchHit,
query: string,
) => string | undefined;
/** Callback to transform a search result into a title */
getTitle?: (
reference: SearchResult | AlgoliaDocSearchHit,
query: string,
) => string | undefined;
/** Callback to transform a search result into a subtitle */
getSubtitle?: (
reference: SearchResult | AlgoliaDocSearchHit,
query: string,
) => string | undefined;
/**
* Label for the search input, not shown but used for `aria-label`
* @default "Search docs…"
**/
label?: string;
/**
* Label for the tab bar
* @default "Search"
**/
tabLabel?: string;
/**
* Placeholder for the search input
* @default "Search docs…"
*/
placeholder?: string;
};
trigger?: {
/**
* `aria-label` for the open button
* @default "Open Markprompt"
**/
label?: string;
/**
* Placeholder text for non-floating element.
* @default "Ask docs"
**/
placeholder?: string;
/**
* Should the trigger button be displayed as a floating button at the bottom right of the page?
* Setting this to false will display a trigger button in the element passed
* to the `markprompt` function.
*/
floating?: boolean;
/** Do you use a custom element as the dialog trigger? */
customElement?: boolean;
};
title?: {
/**
* Visually hide the title
* @default true
**/
hide?: boolean;
/**
* Text for the title
* @default "Ask AI"
**/
text?: string;
};
/**
* Show Markprompt branding
* @default true
**/
showBranding?: boolean;
/**
* Display debug info
* @default false
**/
debug?: boolean;
}
Styles are easily overridable for customization via targeting classes. Additionally, see the styling section in our documentation for a full list of variables.
<script>
tagBesides initializing the Markprompt component yourselves from JavaScript, you can load the script from a CDN. You can attach the options for the Markprompt component to the window prior to loading our script:
<!-- Replace with your local build of the css -->
<!-- <link rel="stylesheet" href="https://esm.sh/@markprompt/css@0.13.x?css" /> -->
<script>
window.markprompt = {
projectKey: `YOUR-PROJECT-KEY`,
container: `#markprompt`,
options: {
references: {
getHref: (reference) => reference.file?.path?.replace(/\.[^.]+$/, ''),
getLabel: (reference) => {
return reference.meta?.leadHeading?.value || reference.file?.title;
},
},
},
};
</script>
<!-- Replace with your local build of the web component -->
<script
async
type="module"
src="https://esm.sh/@markprompt/web@0.16.x/init"
></script>
<div id="markprompt"></div>
markprompt(projectKey, container, options?)
Render a Markprompt dialog button.
projectKey
(string
): Your Markprompt project key.container
(HTMLElement | string
): The element or selector to render
Markprompt into.options
(object
): Options for customizing Markprompt, see above.When rendering the Markprompt component, it will render a search input-like button by default. You have two other options:
set trigger.floating = true
to render a floating button
set trigger.customElement = true
, then
import { openMarkprompt } from '@xrpl/ai-react'
and call
openMarkprompt()
from your code. This gives you the flexibility to render
your own trigger element and attach whatever event handlers you would like
and/or open the Markprompt dialog programmatically.
markpromptOpen()
Open the Markprompt dialog programmatically.
markpromptClose()
Close the Markprompt dialog programmatically.
markpromptChat(projectKey, container, options?)
Render the Markprompt chat view standalone, outside of a dialog.
projectKey
(string
): Your Markprompt project key.container
(HTMLElement | string
): The element or selector to render
Markprompt into.options
(object
): Options for customizing Markprompt, see above.options.chatOptions
(MarkpromptOptions.chat
): Enable and configure chat
functionality. Allows users to have a conversation with an assistant.
Enabling chat functionality will disable prompt functionality. See above for options.options.debug
(boolean
): Display debug infooptions.feedbackOptions
(MarkpromptOptions.feedback
): Enable feedback
functionality, shows a thumbs up/down button after a prompt was submitted. See above for options.options.referencesOptions
(MarkpromptOptions.references
): Enable and
configure references functionality. See above for options.MIT © XRPL AI Devs
FAQs
A web component for adding GPT-4 powered search using the XRPL AI API.
The npm package @xrpl/ai-web receives a total of 541 weekly downloads. As such, @xrpl/ai-web popularity was classified as not popular.
We found that @xrpl/ai-web demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.