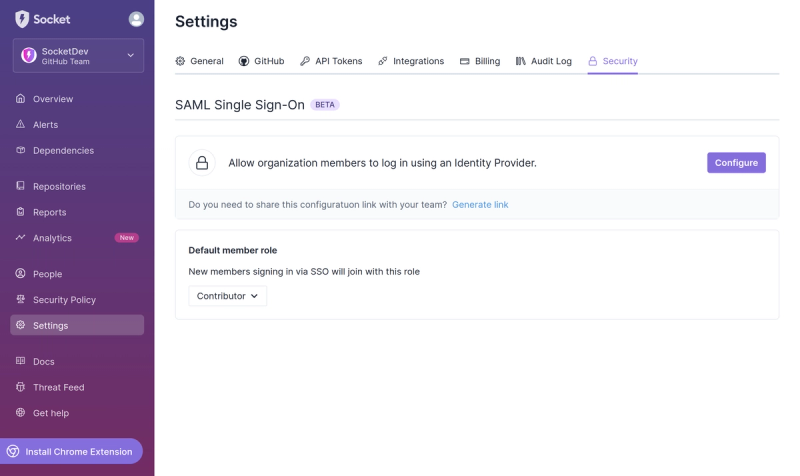
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
@yandex/ymaps3-entity-tile-loader
Advanced tools
Yandex Maps JS API 3.0 example @yandex/ymaps3-entity-tile-loader
Readme
Yandex JS API package loading data by tiles.
Allows you to load and display on the map only those objects that are included in the tile areas displayed on the map.
The data is loaded tile-by-tile, so you don't have to load all the data at once.
The package is located in the dist
folder:
dist/types
TypeScript typesdist/esm
es6 modules for direct connection in your projectdist/index.js
Yandex JS Moduleto use Yandex JS Module you need to add your module loading handler to JS API
Recommended use YMapEntityTileLoader
as usual npm package:
npm i @yandex/ymaps3-entity-tile-loader
and dynamic import
const {YMapEntityTileLoader} = await import('@yandex/ymaps3-entity-tile-loader/dist/esm/index');
But you can use CDN:
ymaps3.import.loaders.unshift(async (pkg) => {
if (!pkg.startsWith('@yandex/ymaps3-entity-tile-loader')) {
return;
}
await ymaps3.import.script(`./node_modules/@yandex/ymaps3-entity-tile-loader/dist/index.js`);
return window[`${pkg}`];
});
ymaps3.import.loaders.unshift(async (pkg) => {
if (!pkg.includes('@yandex/ymaps3-entity-tile-loader')) {
return;
}
// You can use another CDN
await ymaps3.import.script(`https://unpkg.com/${pkg}/dist/index.js`);
return window[`${pkg}`];
});
and in your final code just use ymaps3.import
const {YMapFeature, YMapDefaultFeaturesLayer} = ymaps3;
const {YMapEntityTileLoader} = await ymaps3.import('@yandex/ymaps3-entity-tile-loader@1.0.0');
map.addChild(new YMapDefaultFeaturesLayer());
map.addChild(
new YMapEntityTileLoader({
/**
* By default, when changing tiles, old points are immediately deleted.
* But the same points may appear in the new tile, then there was no point in deleting them.
* Set the delay for applying deletion operations
*/
removalDelay: 500,
tileSize: 256, // World is 256x256 pixels on 0 zoom in Yandex
getFeatureId: (feature) => feature.id,
fetchTile: ({tx, ty, tz, sginal}) => {
return fetch(`https://geodata.example/${tx}/${ty}/${tz}`, {signal}).then((r) => r.json());
},
entity: (feature) =>
new YMapFeature({id: feature.id.toString(), geometry: feature.geometry, properties: feature.properties}),
onFeatureAdd: (feature) => {
console.log(feature);
},
onFeatureRemove: (feature) => {
console.log(feature);
}
})
);
Constructor parameters YMapEntityTileLoader
:
import type {GenericFeature, LngLat, YMapEntity} from '@yandex/ymaps3-types';
export type GeojsonFeature = GenericFeature<LngLat>;
export interface YMapEntityTileLoaderProps {
/** Tile size in pixels. World is 256x256 pixels on 0 zoom in Yandex */
readonly tileSize: number;
/**
* Function for loading data by tile, should return an array of GeoJSON features
*/
fetchTile: (args: {tx: number; ty: number; tz: number; signal: AbortSignal}) => Promise<GeojsonFeature[]>;
/**
* Function for getting the id of the feature.
*/
getFeatureId: (feature: GeojsonFeature) => string;
/**
* Function for creating an [YMapEntity](https://ymaps3.world/docs/js-api/ref/index.html#class-mmapentity) from a feature.
*/
entity: (feature: GeojsonFeature) => YMapEntity<unknown>;
/**
* Function is called when a feature is added to the map.
* If the function returns `false`, the feature will not be added to the map.
* In this case, you should add the feature to the map yourself.
*/
onFeatureAdd: (feature: GeojsonFeature) => void | false;
/**
* Function is called when a feature is removed from the map.
* If the function returns `false`, the feature will not be removed from the map.
* In this case, you should remove the feature from the map yourself.
*/
onFeatureRemove: (feature: GeojsonFeature) => void | false;
/**
* By default, when changing tiles, old features are immediately deleted.
* But the same points may appear in the new tile, then there was no point in deleting them.
* Set the delay for applying deletion operations.
* @default 0
*/
removalDelay?: number;
}
And a React version:
const BOUNDS = [
[53.20890963521473, 25.52765018907181],
[57.444403818421854, 24.71096299361919]
];
const LOCATION = {bounds: BOUNDS};
const [ymaps3React] = await Promise.all([ymaps3.import('@yandex/ymaps3-reactify'), ymaps3.ready]);
const reactify = ymaps3React.reactify.bindTo(React, ReactDOM);
const {YMap, YMapDefaultSchemeLayer, YMapDefaultFeaturesLayer, YMapControlButton, YMapControls, YMapFeature} =
reactify.module(ymaps3);
const {useState, useCallback} = React;
const {YMapZoomControl} = reactify.module(await ymaps3.import('@yandex/ymaps3-controls@0.0.1'));
const {YMapEntityTileLoader} = reactify.module(await ymaps3.import('@yandex/ymaps3-entity-tile-loader'));
function App() {
return (
<YMap location={LOCATION} ref={(x) => (map = x)}>
<YMapDefaultSchemeLayer />
<YMapDefaultFeaturesLayer />
<YMapControls position="right">
<YMapZoomControl />
</YMapControls>
<YMapControls position="top">
<YMapControlButton text={`urban area in loaded tiles: ${total.toFixed(2)} km2`} />
</YMapControls>
<YMapEntityTileLoader
renderDelay={100}
tileSize={TILE_SIZE}
getFeatureId={useCallback((feature) => feature.id, [])}
fetchTile={fetchTile}
entity={useCallback(
(feature) => (
<YMapFeature id={feature.id.toString()} geometry={feature.geometry} properties={feature.properties} />
),
[]
)}
onFeatureAdd={useCallback((entity) => {
setTotal((total) => total + entity.properties.area_sqkm);
}, [])}
onFeatureRemove={useCallback((entity) => {
setTotal((total) => total - entity.properties.area_sqkm);
}, [])}
/>
</YMap>
);
}
For react version, you can use renderDelay
parameter, because react will call render
function for each feature.
To avoid unnecessary calls, you can set the delay for applying the changes.
FAQs
Yandex Maps JS API 3.0 example @yandex/ymaps3-entity-tile-loader
The npm package @yandex/ymaps3-entity-tile-loader receives a total of 2 weekly downloads. As such, @yandex/ymaps3-entity-tile-loader popularity was classified as not popular.
We found that @yandex/ymaps3-entity-tile-loader demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.