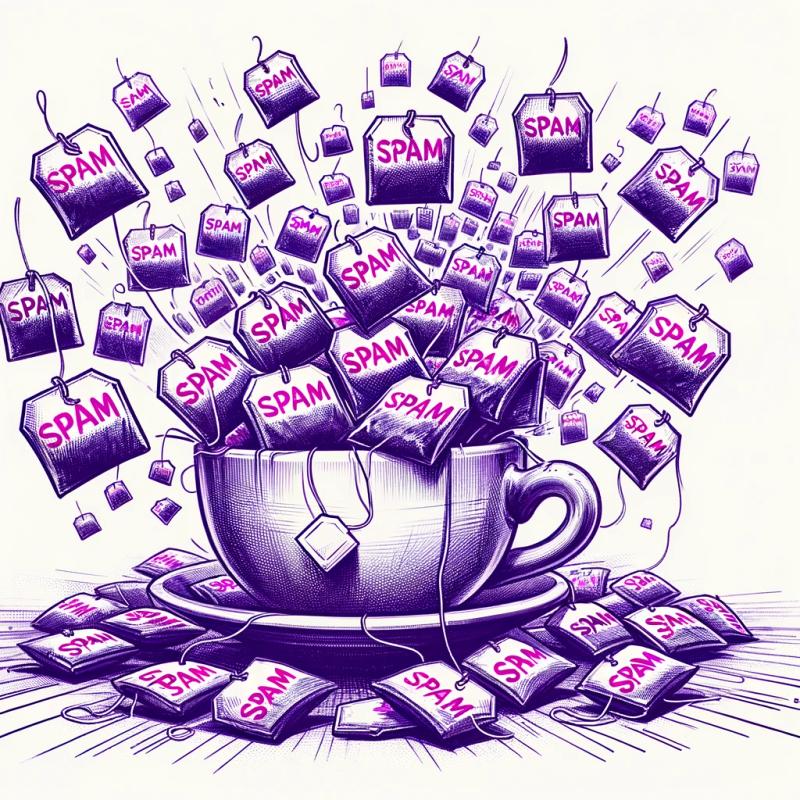
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
fiber.js
Advanced tools
Readme
Take a look at the performance tests to see how it compares against commonly used inheritance libraries.
[[constructor]].extend( function )
// Animal base class
var Animal = Fiber.extend(function() {
return {
// The `init` method serves as the constructor.
init: function() {
// Private
function private1(){}
function private2(){}
// Privileged
this.privileged1 = function(){}
this.privileged2 = function(){}
},
// Public
method1: function(){}
}
});
The init
method acts as the constructor, which is invoked when an instance is created:
var animal = new Animal(); // Create a new Animal instance
init
is invoked automatically.
// Extend the Animal class.
var Dog = Animal.extend(function() {
return {
// Override base class `method1`
method1: function(){
console.log('dog::method1');
},
scare: function(){
console.log('Dog::I scare you');
}
}
});
Create an instance of Dog
:
var husky = new Dog();
husky.scare(); // "Dog::I scare you'"
Every class definition has access to the parent's prototype via the first argument passed into the function:
// Extend the Animal class.
var Dog = Animal.extend(function( base ) {
return {
// Override base class `method1`
method1: function(){
// Call the parent method
base.method1.call(this);
},
scare: function(){
console.log('Dog::I scare you');
}
}
});
Mixins are a way to add functionality to a Fiber definition. Basically, they address the problem of "multiple inheritance". Read more.
Fiber.mixin( object, function1, function2, ... )
var Foo = Fiber.extend(function(base) {
return {
method1: function(){}
}
});
var f = new Foo();
f.method1();
var mix1 = function(base) {
return {
method2: function() {}
}
}
Fiber.mixin(Foo, mix1);
f.method2();
With decorators you can dynamically attach additional properties to an instance. Read more.
Fiber.decorate( instance, decorator_1, ... , decorator_n )
function CarWithPowerWindows(base) {
return {
roll: function() {}
}
}
Fiber.decorate(myCar, CarWithPowerWindows);
Fiber.proxy( base, instance )
// Extend the Animal class;
var Dog = Animal.extend(function(base) {
return {
init: function() {
this.base = Fiber.proxy(base, this);
this.base.init();
}
}
});
Fiber.noConflict()
Returns a reference to the Fiber object, and sets the Fiber
variable to its previous owner.
FAQs
Lightweight JavaScript inheritance library
The npm package fiber.js receives a total of 3 weekly downloads. As such, fiber.js popularity was classified as not popular.
We found that fiber.js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.