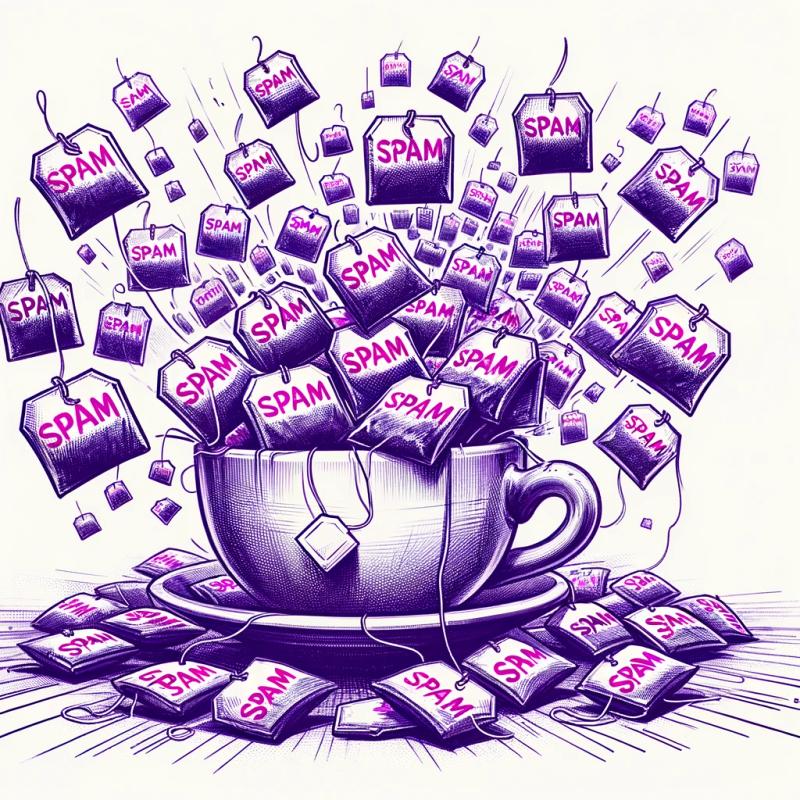
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
grapnel.js
Advanced tools
Readme
Download Source:
Install with npm
npm install grapnel
Or by using bower:
bower install grapnel
Server only: (with HTTP methods added, more info)
npm install grapnel-server
pushState
or hashchange
concurrently#
or #!
for hashchange
routingconst router = new Grapnel();
router.get('products/:category/:id?', function(req) {
let id = req.params.id;
let category = req.params.category;
// GET http://mysite.com/#products/widgets/134
console.log(category, id);
// => widgets 134
});
const router = new Grapnel({ pushState : true });
router.get('/products/:category/:id?', function(req) {
let id = req.params.id;
let category = req.params.category;
console.log(category, id);
});
router.navigate('/products/widgets/134');
// => widgets 134
Grapnel supports regex style routes similar to Sinatra, Restify, and Express. The properties are mapped to the parameters in the request.
router.get('products/:id?', function(req) {
// GET /file.html#products/134
console.log(req.params.id);
// => 134
});
router.get('products/*', function(req) {
// The wildcard/asterisk will match anything after that point in the URL
// Parameters are provided req.params using req.params[n], where n is the nth capture
});
Grapnel also supports middleware:
let auth = function(req, event, next) {
user.auth(function(err) {
req.user = this;
next();
});
}
router.get('/*', auth, function(req) {
console.log(req.user);
});
You can add context to a route and even use it with middleware:
let usersRoute = router.context('/user/:id', getUser, getFollowers); // Middleware can be used here
usersRoute('/', function(req, event) {
console.log('Profile', req.params.id);
});
usersRoute('/followers', otherMiddleware, function(req, event) { // Middleware can be used here too
console.log('Followers', req.params.id);
});
router.navigate('/user/13589');
// => Profile 13589
router.navigate('/user/13589/followers');
// => Followers 13589
import { createServer } from 'http';
import Grapnel from 'grapnel';
const app = new Grapnel();
app.get('/', function(req, route) {
route.res.end('Hello World!', 200);
});
createServer(function(req, res) {
app.once('match', function(route) {
route.res = res;
}).navigate(req.url);
}).listen(3000);
This is now simplified as a separate package (more info)
npm install grapnel-server
let routes = {
'products' : function(req) {
// GET /file.html#products
},
'products/:category/:id?' : function(req) {
// GET /file.html#products/widgets/35
console.log(req.params.category);
// => widgets
}
}
Grapnel.listen(routes);
const router = new Grapnel({ pushState : true, root : '/' });
router.on('navigate', function(event){
// GET /foo/bar
console.log('URL changed to %s', this.path());
// => URL changed to /foo/bar
});
Grapnel allows RegEx when defining a route:
const router = new Grapnel();
let expression = /^food\/tacos\/(.*)$/i;
router.get(expression, function(req, event){
// GET http://mysite.com/page#food/tacos/good
console.log('I think tacos are %s.', req.params[0]);
// => "He thinks tacos are good."
});
const router = new Grapnel();
const router = new Grapnel({ pushState : true });
You can also specify a root URL by setting it as an option:
const router = new Grapnel({ root : '/app', pushState : true });
The root may require a beginning slash and a trailing slash depending on how you set up your routes.
Grapnel uses middleware similar to how Express uses middleware. Middleware has access to the req
object, route
object, and the next middleware in the call stack (commonly denoted as next
). Middleware must call next()
to pass control to the next middleware, otherwise the router will stop.
For more information about how middleware works, see Using Middleware.
let user = function(req, route, next) {
user.get(function(err) {
req.user = this;
next();
});
}
router.get('/user/*', user, function(req) {
console.log(req.user);
});
Grapnel.listen({
'products/:id' : function(req) {
// Handler
}
});
When declaring routes with a literal object, router options can be passed as the first parameter:
let opts = { pushState : true };
Grapnel.listen(opts, routes);
If pushState is enabled, you can navigate through your application with router.navigate
:
router.navigate('/products/123');
router.on('match', function(routeEvent) {
routeEvent.preventDefault(); // Stops event handler
});
router.get('/products/:id', function(req, routeEvent) {
routeEvent.stopPropagation(); // Stops propagation of the event
});
router.get('/products/widgets', function(req, routeEvent) {
// This will not be executed
});
router.navigate('/products/widgets');
You can specify a route that only uses a wildcard *
as your final route, then use route.parent()
which returns false
if the call stack doesn't have any other routes to run.
let routes = {
'/' : function(req, route) {
// Handle route
},
'/store/products/:id' : function(req, route) {
// Handle route
},
'/category/:id' : function(req, route) {
// Handle route
},
'/*' : function(req, route) {
if(!route.parent()){
// Handle 404
}
}
}
Grapnel.listen({ pushState : true }, routes);
router.navigate('/', {
state: { ...windowState }
});
get
Adds a listeners and middleware for routes/**
* @param {String|RegExp} path
* @param {Function} [[middleware], callback]
*/
router.get('/store/:category/:id?', function(req, route){
let category = req.params.category;
let id = req.params.id;
console.log('Product #%s in %s', id, category);
});
navigate
Navigate through application/**
* @param {String} path relative to root
* @param {Object} options navigation options
*/
router.navigate('/products/123', ...options);
on
Adds a new event listener/**
* @param {String} event name (multiple events can be called when separated by a space " ")
* @param {Function} callback
*/
router.on('myevent', function(event) {
console.log('Grapnel works!');
});
once
A version of on
except its handler will only be called once/**
* @param {String} event name (multiple events can be called when separated by a space " ")
* @param {Function} callback
*/
router.once('init', function() {
console.log('This will only be executed once');
});
emit
Triggers an event/**
* @param {String} event name
* @param {...Mixed} attributes Parameters that will be applied to event handler
*/
router.emit('event', eventArg1, eventArg2, ...etc);
context
Returns a function that can be called with a specific route in context.Both the router.context
method and the function it returns can accept middleware. Note: when calling route.context
, you should omit the trailing slash.
/**
* @param {String} Route context (without trailing slash)
* @param {[Function]} Middleware (optional)
* @return {Function} Adds route to context
*/
let usersRoute = router.context('/user/:id');
usersRoute('/followers', function(req, route) {
console.log('Followers', req.params.id);
});
router.navigate('/user/13589/followers');
// => Followers 13589
path
router.path('string')
Sets a new path or hashrouter.path()
Gets path or hashrouter.path(false)
Clears the path or hashbind
An alias of on
trigger
An alias of emit
add
An alias of get
pushState
Enable pushState, allowing manipulation of browser history instead of using the #
and hashchange
eventroot
Root of your app, all navigation will be relative to thistarget
Target object where the router will apply its changes (default: window
)hashBang
Enable #!
as the anchor of a hashchange
router instead of using just a #
navigate
Fires when router navigates through historymatch
Fires when a new match is found, but before the handler is calledhashchange
Fires when hashtag is changedFAQs
The first (and smallest!) JavaScript Router with PushState, Middleware, and Named Parameter support
The npm package grapnel.js receives a total of 25 weekly downloads. As such, grapnel.js popularity was classified as not popular.
We found that grapnel.js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.