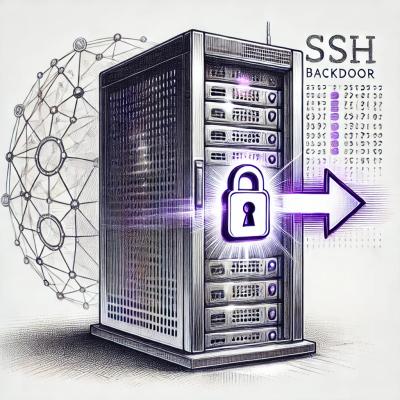
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
addok-cluster
Advanced tools
A very fast and efficient way to use addok geocoding capabilities in your Node.js process.
AbortController
npm install addok-cluster
import {createCluster} from 'addok-cluster'
const cluster = await createCluster(options)
Environment variable name | Option name | Description |
---|---|---|
PYTHON_PATH | pythonPath | Path to python executable to use |
ADDOK_CLUSTER_NUM_NODES | numNodes | Number of nodes to instantiate (default to number of CPUs) |
ADDOK_CONFIG_MODULE | addokConfigModule | Path to addok configuration file |
ADDOK_REDIS_URL | addokRedisUrl | Connection string to addok Redis instance (can be an array) |
ADDOK_REDIS_DATA_DIR | redisDataDir | Path to Redis data directory (enable managed Redis) |
ADDOK_REDIS_STARTUP_TIMEOUT | redisStartupTimeout | Duration in milliseconds allowed to Redis to start (managed Redis only) |
const params = {
q: '1 rue de la paix 75002 Paris',
autocomplete: false,
lon: null,
lat: null,
limit: 5,
filters: {
postcode: '75002',
citycode: '75102'
}
}
const options = {
priority: 'medium',
signal: null
}
const results = await cluster.geocode(params, options)
Param | Description | Default |
---|---|---|
q | Text input to geocode (required) | |
autocomplete | Auto-complete mode (boolean ) | false |
lon , lat | Coordinates of reference position | |
limit | Number of returned results | 5 |
filters | Additional filters (depend on addok config) | {} |
const params = {
lon: null,
lat: null,
limit: 5,
filters: {
type: 'housenumber'
}
}
const options = {
priority: 'high',
signal: null
}
const results = await cluster.reverse(params, options)
Param | Description | Default |
---|---|---|
lon , lat | Coordinates of reference position (required) | |
limit | Number of returned results | 5 |
filters | Additional filters (depend on addok config) | {} |
priority
: low
| medium
(default) | high
Define the priority level of the request. Requests are processed following FIFO rule, from high to low.
signal
: AbortSignal
instance (optional)
If an AbortSignal
instance is provided, its status is checked just before forwarding to addok.
cluster.end()
This software is maintained by Living Data, thanks to the support of partners such as Etalab (API Adresse) and IGN (Géoplateforme).
FAQs
Clustering addok geocoding capabilities from your Node.js process
We found that addok-cluster demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.