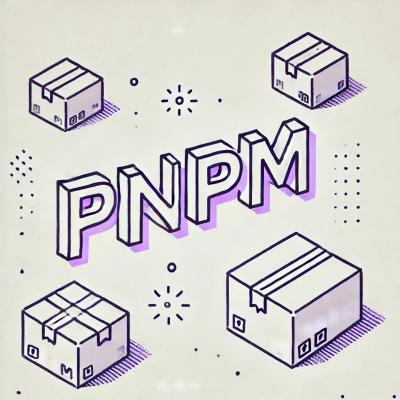
Security News
pnpm 10.12 Introduces Global Virtual Store and Expanded Version Catalogs
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.
addvansed-hash
Advanced tools
Release Date: 2025-03-03
Author: [tarek]
This major release brings robust RSA key handling, enhanced SHA-256 signing/verification, and a revamped Password module supporting both synchronous and asynchronous operations. We have also standardized error messages, enriched TypeScript definitions, and introduced additional security best practices.
If you’re upgrading from a previous version, check out the Migration Notes for essential changes.
Async RSA Key Generation
JWT.RSASync.GenrateKeys()
now relies on the non-blocking generateKeyPair
under the hood.Consistent PEM/DER Format
toString("hex")
for private keys. The library stores RSA keys in standard PEM format.DECODER routines::unsupported
errors when encrypting or decrypting with Node.js.Unified RSA Encryption API
publicEncrypt
is used by default. If it fails, we gracefully fallback to privateEncrypt
only for specialized use cases.Sign / Verify with Extended Options
JWT.SHA256.Sign
and JWT.SHA256.Verify
can now handle typical JWT-like fields: iat
, exp
, nbf
, iss
, sub
, aud
, jti
.exp
(expiration) and nbf
(not before) automatically throw an error or return Error
if the token is expired or inactive.Async Wrapper
JWT.SHA256Sync
(previously SHA256Sync
) returns Promises instead of direct values, letting you integrate with async/await more easily.Improved Defaults
encoding
is "hex"
, but you can switch to "base64"
, "base64url"
, or "latin1"
to meet your project’s requirements.Synchronous (Hash.Password
)
bcrypt
with optional encryption logic.Error
if something goes wrong.Asynchronous (Hash.PasswordSync
)
Promise<string | void>
, ideal for non-blocking environments.Promise<boolean | Error>
for streamlined error handling.Clearer Error Handling
Unified Error Format
Error
object with standardized messages.erroHandel
has been renamed to errorHandle
for clarity.Enhanced Logging
TokenExpiredError
) can be integrated in future expansions.Sha256SignOptions
& Sha256DecryptOptions
extended for additional JWT-like fields (iss
, aud
, sub
, nbf
).manual-test.ts
for quick local checks without a full test framework.jest.config
for ts-jest
usage, so you can run advanced test suites if needed.Private Key Format
erroHandel
→ errorHandle
erroHandel
function have been replaced. Update your calls accordingly if you used that function name.New Default Encodings
"hex"
or "base64"
. If you previously forced "binary"
, confirm your tokens or hashed values still parse correctly.Signature Checking
JWT.SHA256.Verify
re-computes the signature using the token’s last segment for encoding
. Ensure your tokens or code references the correct encoding in the final step.PEM Keys
.toString("hex")
calls. Store your private/public keys as standard PEM strings (with -----BEGIN RSA PRIVATE KEY-----
blocks).Async Patterns
Hash.PasswordSync
) if you want non-blocking password hashing in high-traffic apps.Error Handling
erroHandel
with errorHandle
.Testing
manual-test.ts
to ensure your environment is set up to handle class fields, TypeScript, and Node.js crypto.preset: 'ts-jest'
or Babel is configured properly.import { Hash } from "addvansed-hash";
// Synchronous approach
const hashed = Hash.Password.Hash("myPassword", "myKey");
if (typeof hashed === "string") {
const match = Hash.Password.Match(hashed, "myPassword", "myKey");
console.log("Password match result:", match);
}
// Async approach
(async () => {
const hashedAsync = await Hash.PasswordSync.Hash("myPassword", "myKey");
if (typeof hashedAsync === "string") {
const matchAsync = await Hash.PasswordSync.Match(hashedAsync, "myPassword", "myKey");
console.log("Password match async result:", matchAsync);
}
})();
import { JWT } from "addvansed-hash";
(async () => {
const keys = await JWT.RSASync.GenrateKeys();
if (keys) {
const ciphertext = await JWT.RSASync.Encrypt("Hello RSA!", keys.publicKey);
console.log("Encrypted text:", ciphertext);
const plaintext = await JWT.RSASync.Decrypt(ciphertext, keys.privateKey);
console.log("Decrypted text:", plaintext);
}
})();
import { JWT } from "addvansed-hash";
const secret = "mySecretKey";
const token = JWT.SHA256.Sign({ userId: 123 }, secret, { expiresIn: 3600, iat: true });
console.log("Generated token:", token);
if (typeof token === "string") {
const verification = JWT.SHA256.Verify(token, secret, {});
if (verification instanceof Error) {
console.error("Token verification failed:", verification.message);
} else {
console.log("Verified payload:", verification);
}
}
Distributed under the MIT License—you are free to use, modify, and distribute. See the LICENSE file for details.
Enjoy the “addvansed” level of security and performance!
We look forward to your feedback and contributions to keep addvansed-hash moving forward.
FAQs
A my package hashing (support deno/bun/npm)
The npm package addvansed-hash receives a total of 12 weekly downloads. As such, addvansed-hash popularity was classified as not popular.
We found that addvansed-hash demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.
Security News
Amaro 1.0 lays the groundwork for stable TypeScript support in Node.js, bringing official .ts loading closer to reality.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.