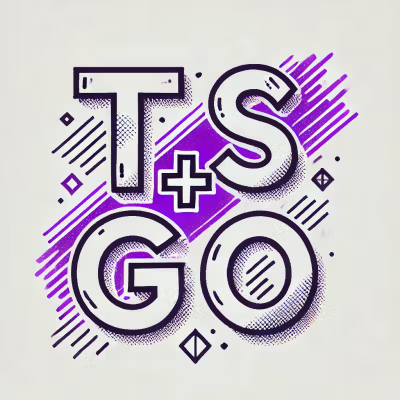
Security News
TypeScript is Porting Its Compiler to Go for 10x Faster Builds
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
A fast postgres CRUD ORM
Original base work was a forked from @abeai/node-utils
PGEncryptModel
Postgres Active Model class to extend a custom model from.
Postgres Base Model class to extend a custom model from.
Postgres Connecter to initialize the singleton for connection.
PGBaseModel
Postgres Encryption Model class to extend a custom model from.
The types of fields for Postgres Models.
PGEncryptModel
Postgres Active Model class to extend a custom model from.
Kind: global class
Extends: PGEncryptModel
PGEncryptModel
PGActiveModel
PGActiveModel
PGActiveModel
PGActiveModel
PGActiveModel
PGActiveModel
PGActiveModel
PGActiveModel
String
PGActiveModel
PGActiveModel
Array.<PGActiveModel>
Array.<PGActiveModel>
Array.<PGActiveModel>
PGActiveModel
Array.<PGActiveModel>
Array.<PGActiveModel>
Array.<PGActiveModel>
PGActiveModel
Array.<PGActiveModel>
Array.<PGActiveModel>
Array.<PGActiveModel>
Adds a property to this model that does not affect it from a database perspective.
Kind: instance method of PGActiveModel
Param | Type | Description |
---|---|---|
name | String | The name of the property. |
value | Any | The the value to set the property. |
PGActiveModel
Retrieves the current model by its set field with type PGTypes.PK
Kind: instance method of PGActiveModel
Returns: PGActiveModel
- Returns it's self.
PGActiveModel
Deletes the current model by its set field with type PGTypes.PK
Kind: instance method of PGActiveModel
Returns: PGActiveModel
- Returns it's self.
PGActiveModel
Creates a new row with the currently set properties.
Kind: instance method of PGActiveModel
Returns: PGActiveModel
- Returns it's self.
PGActiveModel
Saves the model with its changed properties.
Kind: instance method of PGActiveModel
Returns: PGActiveModel
- Returns it's self.
PGActiveModel
Updates the model with the passed in changed properties.
Kind: instance method of PGActiveModel
Returns: PGActiveModel
- Returns it's self.
PGActiveModel
Decrypts the properties on the model based on which stringed names are passed in as arguments.
Kind: instance method of PGActiveModel
Returns: PGActiveModel
- Returns it's self.
Param | Type | Description |
---|---|---|
...props | String | name of each property. |
PGActiveModel
Encrypts the properties on the model based on which stringed names are passed in as arguments.
Kind: instance method of PGActiveModel
Returns: PGActiveModel
- Returns it's self.
Param | Type | Description |
---|---|---|
...props | String | name of each property. |
PGActiveModel
Redacts all encrypted fields from the model.
Kind: instance method of PGActiveModel
Returns: PGActiveModel
- Returns it's self.
Param | Type | Default | Description |
---|---|---|---|
redactionCensor | String | "[redacted]" | The string to replace the encrypted values with. |
String
Gets the encrypted profile that the model has set.
Kind: instance method of PGActiveModel
Returns: String
- Returns it's self.
PGActiveModel
Creates a new row with the passed in props and values.
Kind: static method of PGActiveModel
Returns: PGActiveModel
- Returns it's self.
Param | Type | Description |
---|---|---|
model | Object | A plain object with the name of the properties and their values to be set with the new model. |
Example
create({
username: 'foo',
email: 'test@test.com',
});
PGActiveModel
Retrives a model by it's PK.
Kind: static method of PGActiveModel
Returns: PGActiveModel
- Returns a new model.
Param | Type | Description |
---|---|---|
id | String | The PK of the model to retrieve. |
Array.<PGActiveModel>
Retrives a limited amount models by the passed in fieldValues
.
Kind: static method of PGActiveModel
Returns: Array.<PGActiveModel>
- Returns an array of new models.
Param | Type | Default | Description |
---|---|---|---|
fieldValues | Object | A plain object with the properties and their values to retrive by. | |
operator | String | AND | The query operator to use between each of the fieldValues [AND , OR , 'NOT'] |
limit | Number | The limit to stop searching when the records retrived are equal or greater than the set limit . |
Example
findLimtedBy({
username: ['user2', 'OR', 'user3'],
email: null,
}, 'AND', 5);
Array.<PGActiveModel>
Retrives all models by the passed in fieldValues. Will stop searching when the records retrived are equal or greater than limit
.
Kind: static method of PGActiveModel
Returns: Array.<PGActiveModel>
- Returns an array of new models.
Param | Type | Default | Description |
---|---|---|---|
fieldValues | Object | A plain object with the properties and their values to retrive by. | |
operator | String | AND | The query operator to use between each of the fieldValues [AND , OR , 'NOT'] |
Example
findAllBy({
username: ['user2', 'OR', 'user3'],
email: null,
}, 'AND');
Array.<PGActiveModel>
Retrives all rows in the table of the model.
Kind: static method of PGActiveModel
Returns: Array.<PGActiveModel>
- Returns an array of new models.
PGActiveModel
Deletes a model that is found by it's PK with the passed in props and values.
Kind: static method of PGActiveModel
Returns: PGActiveModel
- Returns a new model or null
Param | Type | Description |
---|---|---|
id | String | The PK of the model to delete. |
Array.<PGActiveModel>
Deletes a limited amount models by the passed in fieldValues.
Kind: static method of PGActiveModel
Returns: Array.<PGActiveModel>
- Returns an array of deleted models.
Param | Type | Default | Description |
---|---|---|---|
fieldValues | Object | A plain object with the properties and their values to delete by. | |
operator | String | AND | The query operator to use between each of the fieldValues [AND , OR , 'NOT'] |
limit | Number | The limit to stop deleting when the records retrived are equal or greater than the set limit . |
Example
deleteLimitedBy({
registered: false,
},'AND', 5);
Array.<PGActiveModel>
Deletes all models by the passed in fieldValues
.
Kind: static method of PGActiveModel
Returns: Array.<PGActiveModel>
- Returns an array of deleted models.
Param | Type | Default | Description |
---|---|---|---|
fieldValues | Object | A plain object with the properties and their values to delete by. | |
operator | String | AND | The query operator to use between each of the fieldValues [AND , OR , 'NOT'] |
Example
deleteAllBy({
registered: true,
});
Array.<PGActiveModel>
Deletes all models in their table.
Kind: static method of PGActiveModel
Returns: Array.<PGActiveModel>
- Returns an array of deleted models or null
PGActiveModel
Updates a model that is found by it's PK with the passed in props and values.
Kind: static method of PGActiveModel
Returns: PGActiveModel
- Returns a new model or null
Param | Type | Default | Description |
---|---|---|---|
id | String | The PK of the model to update. | |
model | Object | A plain object with the name of the properties and their values to update the model with. | |
returnModel | Boolean | true | If the updated model should be returned or not. It will return null if this is set to false. |
Example
updateById('09A75A84-A921-4F68-8FEF-B8392E3702C2',
{
password: 'bestpasswordinalltheland12346969420'
});
Array.<PGActiveModel>
Updates models that are found by the passed in fieldValues
with the passed in props and values of the model
.
Kind: static method of PGActiveModel
Returns: Array.<PGActiveModel>
- Returns an array of updated models or null
Param | Type | Default | Description |
---|---|---|---|
fieldValues | Object | A plain object with the properties and their values to update by. | |
model | Object | A plain object with the name of the properties and their values to update the model with. | |
operator | String | AND | The query operator to use between each of the fieldValues [AND , OR , 'NOT'] |
returnModel | Boolean | true | If the updated model should be returned or not. It will return null if this is set to false. |
limit | Number | The limit to stop searching when the records retrived are equal or greater than the set limit . |
Example
updateLimitedBy({
password: null
},
{
password: 'bestpasswordinalltheland12346969420'
},'AND', true, 5);
Array.<PGActiveModel>
Updates all models that are found by the passed in fieldValues
with the passed in props and values of the model
.
Kind: static method of PGActiveModel
Returns: Array.<PGActiveModel>
- Returns an array of updated models or null
Param | Type | Default | Description |
---|---|---|---|
fieldValues | Object | A plain object with the properties and their values to update by. | |
model | Object | A plain object with the name of the properties and their values to update the model with. | |
operator | String | AND | The query operator to use between each of the fieldValues [AND , OR , 'NOT'] |
returnModel | Boolean | true | If the updated model should be returned or not. It will return null if this is set to false. |
Example
updateAllBy({
password: null
},
{
password: 'bestpasswordinalltheland12346969420'
});
Array.<PGActiveModel>
Updates all models in their table with the passed in props and values of the model
.
Kind: static method of PGActiveModel
Returns: Array.<PGActiveModel>
- Returns an array of updated models or null
Param | Type | Description |
---|---|---|
model | Object | A plain object with the name of the properties and their values to update the models with. |
Example
updateAll({
password: 'bestpasswordinalltheland12346969420'
});
Postgres Base Model class to extend a custom model from.
Postgres Connecter to initialize the singleton for connection.
Param | Type | Default | Description |
---|---|---|---|
options | Object | The connection options. | |
options.crypto | Crypto | postgres/crypto/interface.js | The implemented crypto interface that follows postgres/crypto/interface.js |
options.pg | Object | The options object to pass into pg lib. | |
options.pg.user | String | process.env.PGUSER | User's name. |
options.pg.password | String | process.env.PGPASSWORD | User's password. |
options.pg.database | String | process.env.PGDATABASE | Database's name. |
options.pg.port | Number | process.env.PGPORT | Database's port. |
options.pg.connectionString | String | Postgres formated connection string. e.g. postgres://user:password@host:5432/database | |
options.pg.ssl | TLSSocket | Options to be passed into the native Node.js TLSSocket socket. | |
options.pg.types | pg.types | Custom type parsers. See node-postgres types for more details. | |
options.pg.statement_timeout | Number | 0 | Number of milliseconds before a statement in query will time out. |
options.pg.query_timeout | Number | 0 | number of milliseconds before a query call will timeout. |
options.pg.connectionTimeoutMillis | Number | 0 | Number of milliseconds to wait before timing out when connecting a new client. |
options.pg.idleTimeoutMillis | Number | 10000 | Number of milliseconds a client must sit idle in the pool and not be checked out before it is disconnected from the backend and discarded. |
options.pg.max | Number | 10 | Maximum number of clients the pool should contain. |
Example
var pgOptions = {
pg: {
connectionString: 'postgres://postgres@localhost/pgtest',
}
};
try {
pgOptions.crypto = require('@abeai/node-crypto').utils.pgCrypto;
} catch (_) {
console.log(_);
}
var pg = new PGConnecter(pgOptions);
PGBaseModel
Postgres Encryption Model class to extend a custom model from.
Kind: global class
Extends: PGBaseModel
The types of fields for Postgres Models.
Kind: global constant
The primary key of the table.
Kind: static property of PGTypes
Marks this field to auto encrypt/hash (for look up) but not auto decrypt it on retrieval.
The table will need to have a field with the same name as this set field with __
as a prefix.
Kind: static property of PGTypes
Example
//if you have an encrypted field called `phone` the sql query for creating the table may look like this
CREATE TABLE IF NOT EXISTS users (
phone VARCHAR (500),
__phone VARCHAR (500) UNIQUE,
);
Marks this field to auto encrypt but not auto decrypt it on retrieval.
Same as Encrypt
but with no lookup hash.
Kind: static property of PGTypes
Marks this field as the encryption profile for encrypting/decrypting/hashing utilizing aws kms.
Kind: static property of PGTypes
Marks this field to auto encrypt/hash (for look up) and to auto decrypt it on retrieval.
Kind: static property of PGTypes
Marks this field to auto encrypt and auto decrypt it on retrieval.
Same as AutoCrypt
but with no lookup hash.
Kind: static property of PGTypes
Marks this field to be hashed on creation (IE: Password and other information you want to protect)
Kind: static property of PGTypes
FAQs
A fast postgres CRUD ORM
The npm package adost receives a total of 892 weekly downloads. As such, adost popularity was classified as not popular.
We found that adost demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
Research
Security News
The Socket Research Team has discovered six new malicious npm packages linked to North Korea’s Lazarus Group, designed to steal credentials and deploy backdoors.
Security News
Socket CEO Feross Aboukhadijeh discusses the open web, open source security, and how Socket tackles software supply chain attacks on The Pair Program podcast.