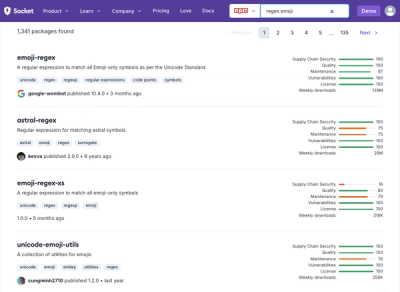
Security News
Weekly Downloads Now Available in npm Package Search Results
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
agora-chat-uikit
Advanced tools
English | 中文
agora-chat-uikit is a React UI component library built on top of Agora Chat SDK. It provides a set of general UI components, a conversation module, and a chat module that enable developers to easily craft a chat app to suit actual business needs. Also, this library calls APIs in Agora Chat SDK to implement related chat logics and data processing, allowing developers to only focus on their own business and personalized extensions.
The Web Chat UIKit has two components:
EaseApp
, which contains the conversation list and applies to use cases where you want to quickly launch a real-time chat app.
EaseChat
, which contains a conversation box and applies to most chat use cases such as sending and receiving messages, displaying the message on the UI, and managing unread messages.
The Web Chat UIKit, by default, provides the following functions:
Agora provides an open-source Web Chat UIKit project for Agora Chat. You can clone or run the project or create a project to integrate the Web Chat UIKit by reference to the open-source project.
Source code URL of Agora Chat UIKit for Web:
URL of Agora Chat app using Agora Chat UIKit for Web:
In order to follow the procedure in this page, you must have:
Browser | Supported Version |
---|---|
IE | 11 or later |
Edge | 43 or later |
Firefox | 10 or later |
Chrome | 54 or later |
Safari | 11 or later |
# Install a CLI tool.
npm install create-react-app
# Create an my-app project.
npx create-react-app my-app
cd my-app
The project directory.
├── package.json
├── public # The static directory of Webpack.
│ ├── favicon.ico
│ ├── index.html # The default single-page app.
│ └── manifest.json
├── src
│ ├── App.css # The CSS of the app's root component.
│ ├── App.js # The app component code.
│ ├── App.test.js
│ ├── index.css # The style of the startup file.
│ ├── index.js # The startup file.
│ ├── logo.svg
│ └── serviceWorker.js
└── yarn.lock
npm install agora-chat-uikit --save
yarn add agora-chat-uikit
Import agora-chat-uikit into your code.
// App.js
import React, {Component} from 'react';
import { EaseApp } from "agora-chat-uikit"
import './App.scss';
class App extends Component {
render() {
return (
<div className="container">
<EaseApp
appkey="xxx", // Your registered App Key.
username="xxx", // The user ID of the current user.
agoraToken="xxx" // The Agora token. For how to obtain an Agora token, see descriptions in the Reference.
/>
</div>
);
}
}
export default App;
/** App.css */
.container {
height: 100%;
width: 100%;
}
Now, you can run your app to send messages. In this example, you can use the default App Key, but need to register your own App Key in the formal development environment. When the default App Key is used, a user will receive a one-to-one chat message and a group chat message upon the first login and can type the first message in a type of conversation and send it.
Note
If a custom App Key is used, no contact is available by default and you need to first add contacts or join a group.
npm run start
Now, you can see your app in the browser.
EaseApp provides a list of attributes for customization. You can customize the features and layout by setting these attributes. To ensure the functionality of EaseApp, ensure that you set all the required parameters.
import { EaseApp } from "agora-chat-uikit";
const header = () => {
return <div>Hello Word</div>;
};
const app = () => {
return (
<EaseApp
appkey={"xxx"}
username={"xxx"}
agoraToken={"xxx"}
header={header} // Custom header.
/>
);
};
Attribute | Type | Required | Description |
---|---|---|---|
appkey | String | Yes | The unique identifier that the Chat service assigns to each app. The rule is You can't use 'macro parameter character #' in math mode ${org_name}#${app_name} . |
username | String | Yes | The user ID. |
agoraToken | String | Yes | The Agora token. |
header | ReactNode | No | The title bar above the conversation list. |
isShowUnread | Boolean | No | Whether to show the number of unread messages: - (Default) true : Yes. - false : No. |
unreadType | Boolean | No | The display style of unread messages: - (Default) true : Displays the number of unread messages; - false : Displays a red dot. |
onConversationClick | function({ item, key }) | No | The callback for clicking a contact on the conversation list. |
showByselfAvatar | Bool | No | Whether to display the avatar of the current user: - (Default) true : Yes.- false : No. |
easeInputMenu | String | No | The mode of the input menu. - (Default) all : The complete mode.- noAudio : No audio.- noEmoji : No emoji.- noAudioAndEmoji : No audio or emoji.- onlyText : Only text. |
menuList | Array | No | menuList:[ {name:'Send a pic',value:'img},{name:'Send a file',value:'file}] |
handleMenuItem | function({item, key}) | No | The callback event triggered by clicking on the right panel of the input box. |
successLoginCallback | function(res) | No | The callback event for a successful login. |
failCallback | function(err) | No | The callback event for a failed method call. |
onChatAvatarClick | Function | No | The callback for clicking the avatar on the top of the conversation. |
isShowReaction | Boolean | No | Whether to show the Reaction function. |
customMessageList | Array | No | Custom shortcut menu items for messages. |
deleteSessionAndMessage | Function | No | The method for deleting a conversation and its messages. |
customMessageClick | Function | No | The callback for clicking a custom shortcut menu item for messages. |
onEditThreadPanel | Function | No | The callback for editing a thread. |
onOpenThreadPanel | Function | No | The callback for opening a thread. |
isShowRTC | Boolean | No | Whether to use the RTC function. |
agoraUid | String | No | Agora UID required to use RTC. |
appId | String | No | The App ID required to use RTC. |
getRTCToken | Function | No | The method for getting the Agora token to use RTC. |
getIdMap | Function | No | The method for getting the mapping between the Agora Chat user ID and the user ID you expect to display on the Web UI, when RTC is used. |
You may need to embed your business logic in various message callbacks in certain scenarios. For this purpose, the Web Chat UIKit provides the following solution.
const WebIM = EaseApp.getSdk({ appkey: "xxx" });
// Note: The App Key is not required if you log in to the chat app successfully.
WebIM.conn.addEventHandler('handlerName'),{
onConnected:()=>{},
onTextMessage:()=>{},
// ....
})
const conversationItem = {
conversationType: "singleChat", // The conversation type. 'singleChat': one-to-one chat. 'groupChat': group chat.
conversationId: "TOM", //The conversation ID. One-to-one chat: the user ID of the message recipient. group chat: The group ID.
};
EaseApp.addConversationItem(conversationItem);
With the EaseApp component, the user, in theory, only needs to pass the above three required parameters for automatic login, instead of implementing the login function. However, in some scenarios, if you do not need implement login inside UIKit, you can call EaseApp.getSdk() to get the Agora Chat SDK object and call the SDK to implement the login function.
// Manual login.
WebIM.conn.open({
user: "xxxx",
agoraToken: "xxxx",
appKey: "xxxx",
});
Likewise, to ensure the normal operation of EaseChat, you must fill in the required attribute parameters. As an independent conversation component, EaseChat is widely available and can easily implement most scenarios.
For example, pop up a specific dialog box upon a click event and customize the listener after a successful login:
import React, { useState } from "react";
import { EaseChat } from "agora-chat-uikit";
const addListener = (res) => {
if(res.isLogin){
const WebIM = EaseChat.getSdk()
WebIM.conn.addEventHandler('testListen',{
onTextMessage:()=>{},
onError:()=>{},
// ...
})
}
}
const chat = () => {
return (
<div>
<EaseChat
appkey={'xxx'}
username={'xxx'}
agoraToken={'xxx'}
to={'xxx'}
successLoginCallback={addListener}
/>
<div/>
)
}
const app = () =>{
const [showSession, setShowSession] = useState(false);
return(
<div>
{ showSession && chat()}
<button onClick={()=>setShowSession(true)}>打开会话</button>
<button onClick={()=>setShowSession(false)}>关闭会话</button>
<div/>
)
}
Attribute | Type | Required | Description |
---|---|---|---|
appkey | String | Yes | The unique identifier that the Chat service assigns to each app. |
username | String | Yes | The user ID. |
agoraToken | String | Yes | The Agora token. |
chatType | String | Yes | The chat type: - singleChat : one-to-one chat. - groupChat : group chat. |
to | String | Yes | The message recipient or the group: - one-to-one chat: The user ID of the message recipient. - group chat: The group ID. |
showByselfAvatar | Bool | No | Whether to display the avatar of the current user. - (Default) true : Yes. - false : No. |
easeInputMenu | String | No | The mode of the input menu. - (Default) all : The complete mode.- noAudio : No audio.- noEmoji : No emoji.- noAudioAndEmoji : No audio or emoji.- onlyText : Only text. |
menuList | Array | No | The extensions of the input box on the right panel. - (Default) menuList:[ {name:'Send a pic',value:'img},{name:'Send a file',value:'file}] |
handleMenuItem | function({item, key}) | No | The callback event triggered by clicking on the right panel of the input box. |
successLoginCallback | function(res) | No | The callback event for a successful login. |
failCallback | function(err) | No | The callback event for a failed method call (including a failed login and all failure events of the SDK). |
Both EaseChat
and EaseApp
support advanced customizations.
const WebIM = EaseChat.getSdk({ appkey: "xxx" });
// The App Key is not required when you successfully log in to the Chat app.
WebIM.conn.addEventHandler("handlerName", {
onConnected: () => {},
onTextMessage: () => {},
// ...
});
EaseChat implements automatic login after you fill in required parameters, saving you the trouble of login. If you need to implement manual login outside EaseChat, follow the steps above mentioned.
handlerName
indicates the custom event handler. You can add multiple event handlers to satisfy your business requirements.
For the UI styles, we provide the [source code of Web Chat UIKit] (https://github.com/AgoraIO-Usecase/AgoraChat-UIKit-web) to satisfy your customization requirements to the maximum extent possible.
If you want to add extra functions to EaseChat to share with others, you can fork our repository on GitHub and create a pull request. For any questions, you can also create a pull request. Thank you for your contributions.
If you have any problems or suggestions regarding the sample projects, feel free to file an issue.
The sample projects are under the MIT license.
FAQs
## Overview
The npm package agora-chat-uikit receives a total of 100 weekly downloads. As such, agora-chat-uikit popularity was classified as not popular.
We found that agora-chat-uikit demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
Security News
A Stanford study reveals 9.5% of engineers contribute almost nothing, costing tech $90B annually, with remote work fueling the rise of "ghost engineers."
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.