alarmist
Functional but still IN DEVELOPMENT - expect issues ;)

Monitor parallel jobs
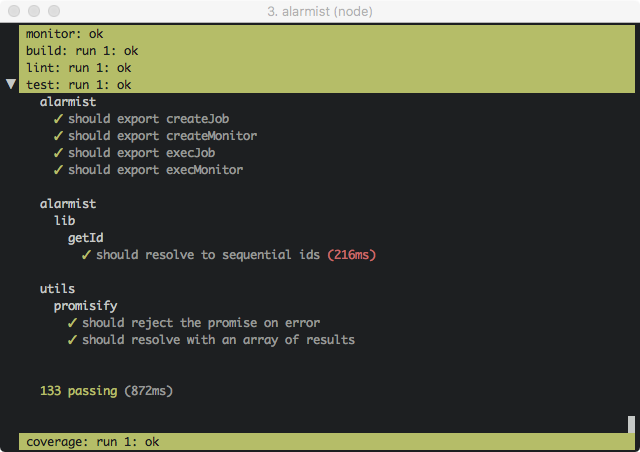
Install
npm install --save-dev alarmist
CLI
Execute a job
alarmist-job -n name my-command [args...]
Monitor jobs
alarmist-monitor my-watch-command [args...]
Jobs will appear on first run and can be expanded (one at a time) to display logs
- [CTRL-c] - stop the monitor
- [up, down, j, k] - select a job
- [enter, o] - expand/collapse job logs
- [up, down, j, k, g, SHIFT-g] - navigate log when expanded
API
var alarmist = require('alarmist');
Custom jobs
Create a job.
alarmist.createJob({
name: 'name'
}).then(function(job) {
...
});
The job will expose a log
write stream that you can use for logging.
job.log.write('this gets logged');
When the job is complete call the complete
method to signal success or failure with an exit code.
job.complete({
exitCode: 0
}).then(function() {
...
});
Execute a job
alarmist.execJob({
name: 'name',
command: 'my-command',
args: []
}).then(function() {
...
});
Monitor jobs and execute a watcher
Start a monitor and watcher process
alarmist.execMonitor({
command: 'my-watcher-command',
args: []
})
.then(function(monitor) {
...
});
Listen for start events when jobs start
monitor.on('start', function(job) {
console.log(job.id);
console.log(job.name);
console.log(job.startTime);
});
Listen for log events when jobs log data
monitor.on('log', function(job) {
console.log(job.id);
console.log(job.name);
console.log(job.data);
});
Listen for end events when jobs end
monitor.on('end', function(job) {
console.log(job.id);
console.log(job.name);
console.log(job.startTime);
console.log(job.endTime);
console.log(job.exitCode);
});
Read from the monitor log stream, for logging from the watcher command
monitor.log.on('data', function(data) {
console.log(data)
});
Listen for an exit
event that signifies an error as the watcher process should not exit
monitor.on('exit', function(code) {
console.log(code);
});
Stop a monitor
monitor.close();
Monitor jobs with your own watcher
Start a monitor
alarmist.createMonitor()
.then(function(monitor) {
...
});
Listen for job events as above
Log for your watcher process
monitor.log.write('output');
Signal the exit of the watcher process (watcher processes aren't meant to exit so this is really signalling an error)
monitor.exit(1);
Listen for exit events and close the monitor as above
Contributing
Run tests, etc before pushing changes/opening a PR
npm test
- lint and test
npm run build
- run tests then build
npm run watch
- watch for changes and run build
npm run ci
- run build and submit coverage to coveralls
npm start
- use alarmist to monitor its own build tasks in parallel :)