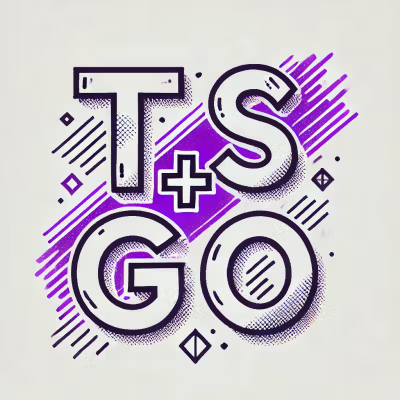
Security News
TypeScript is Porting Its Compiler to Go for 10x Faster Builds
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
amazon-connect-chatjs
Advanced tools
Important note: Amazon Connect has migrated the
Connection Acknowledgement(ConnAck)
from the SendEvent API to the CreateParticipant API. Please upgrade your ChatJS to 1.4.0 or a newer version to complete the migration by 12/31/2024.
The Amazon Connect Chat javascript library (ChatJS) gives you the power to build your own chat widget to customize the chat experience. This can be used for both the agent user interface, in conjunction with Amazon Connect Streams, and for the customer chat interface.
There is a Chat UI reference implementation here. This will help you deploy an API Gateway and Lambda function for initiating chat from your webpage. From there you can use the ChatJS library to build a custom widget.
To learn more about Amazon Connect and its capabilities, please check out the Amazon Connect User Guide.
New to Amazon Connect and looking to onboard with Chat/Messaging capabilities? Refer to the “Amazon Connect Chat Open Source Walkthrough” documentation, and “Hosted Widget vs Custom Builder Solution” if building a customer-facing chat interface.
The AWS-SDK is, by default, included in ChatJS as a "baked-in" dependency. You can view it at ./client/aws-sdk-connectparticipant.js
. In ./client/client.js
we import ConnectParticipant
from this file. This file and import can be removed while using the AWS SDK imported through a script in the page file of your application, assuming that version of the AWS SDK has the ConnectParticipant
service included.
Incidentally, Amazon Connect Streams also contains a "baked-in" AWS SDK. This SDK cannot be removed, as it contains unreleased APIs that will not be available in the SDK you include as a script in the page file.
Therefore, there are several occasions where implementations can run into AWS SDK issues.
Ensure you import ChatJS after Streams.
Import Streams, then ChatJS, then the SDK. Ensure that your AWS SDK includes the ConnectParticipant Service (it is relatively new, so make sure you have an up-to-date AWS SDK version [^2.597.0]).
No need to worry here, this will always work.
Import ChatJS before the AWS SDK, and ensure the AWS SDK version you are using contains the ConnectParticipant Service.
When using the SDK and ChatJS, you may remove the SDK from ChatJS to ensure lack of import conflicts. However, this should not be relevant if the order in which you are importing these libraries is the order reflected above.
If you have replaced ./client/aws-sdk-connectparticipant.js
and use @aws-sdk/client-connectparticipant
, make sure to import the aws-sdk after ChatJS
import 'amazon-connect-streams'; // <-- (optional) MUST be before ChatJS
import 'amazon-connect-chatjs';
import '@aws-sdk/client-connect'; // or 'aws-sdk'
import '@aws-sdk/clients/connectparticipant'; // <-- IMPORTANT - should be last
npm install amazon-connect-chatjs
amazon-connect-chat.js
bundle file is also available over a CDN.
<script src="https://unpkg.com/amazon-connect-chatjs@1.5.1"></script>
<!-- OR -->
<script src="https://cdn.jsdelivr.net/npm/amazon-connect-chatjs@1.5.1/dist/amazon-connect-chat.js"></script>
<!-- Specify exact version -->
<script src="https://unpkg.com/amazon-connect-chatjs@1.5.1"></script>
<script src="https://unpkg.com/amazon-connect-chatjs@1"></script>
<script src="https://unpkg.com/amazon-connect-chatjs"></script>
<!-- Use crossorigin if needed -->
<script crossorigin src="https://unpkg.com/amazon-connect-chatjs"></script>
import "amazon-connect-chatjs"
Note: this will apply the global connect
variable to your current scope.
amazon-connect-chatjs
is compatible with TypeScript. You'll need to use version typescript@^3.0.1
or higher:
import "amazon-connect-streams";
connect.ChatSession.create({ /* ... */ });
git clone https://github.com/amazon-connect/amazon-connect-chatjs
npm install
npm run devo
for a non-minified build.npm run release
for a minified build.npm run test
npm run clean
npm run watch
Find build artifacts in dist directory - This will generate a file called amazon-connect-chat.js
- this is the full Connect ChatJS API which you will want to include in your page.
Additional configuration is required to support ChatJS in React Native applications. Use amazon-connect-chatjs@^1.5.0
and follow the documenation: ReactNativeSupport.md
A demo application implementing basic ChatJS functionality is also available in the ui-examples repository: connectReactNativeChat
connect.ChatSession
APIThis is the main entry point to amazon-connect-chatjs
.
All your interactions with the library start here.
connect.ChatSession.setGlobalConfig()
connect.ChatSession.setGlobalConfig({
loggerConfig: { // optional, the logging configuration. If omitted, no logging occurs
// You can provide your own logger here, otherwise this property is optional
customizedLogger: {
debug: (...msg) => console.debug(...msg), // REQUIRED, can be any function
info: (...msg) => console.info(...msg), // REQUIRED, can be any function
warn: (...msg) => console.warn(...msg), // REQUIRED, can be any function
error: (...msg) => console.error(...msg) // REQUIRED, can be any function
},
// There are five levels available - DEBUG, INFO, WARN, ERROR, ADVANCED_LOG. Default is INFO
level: connect.LogLevel.INFO,
// Choose if you want to use the default logger
useDefaultLogger: true
},
region: "us-east-1", // optional, defaults to: "us-west-2"
//Control switch for enabling/disabling message-receipts (Read/Delivered) for messages
//message receipts use sendEvent API for sending Read/Delivered events https://docs.aws.amazon.com/connect-participant/latest/APIReference/API_SendEvent.html
features: {
messageReceipts: {
shouldSendMessageReceipts: true, // DEFAULT: true, set to false to disable Read/Delivered receipts
throttleTime: 5000 //default throttle time - time to wait before sending Read/Delivered receipt.
}
}
});
Set the global configuration to use. If this method is not called, the defaults of loggerConfig
and region
are used.
This method should be called before connect.ChatSession.create()
.
Customizing loggerConfig
for ChatJS:
customizedLogger
, and add customizedLogger
object as the value of loggerConfig.customizedLogger
, then set the lowest logger level. globalConfig.loggerConfig.useDefaultLogger
is not required.useDefaultLogger
to true. loggerConfig.customizedLogger
is not required.useDefaultLogger
to true, your own logger will be overwritten by the default logger.amazon-connect-chatjs/src/log.js
- has the logic to select LogLevel. Default value is INFO - which cause all logs with higher priority than INFO to be logged. eg: by default info, warn, error and advancedLog messages will be logged.connect.ChatSession.create()
const customerChatSession = connect.ChatSession.create({
chatDetails: { // REQUIRED
contactId: "...", // REQUIRED
participantId: "...", // REQUIRED
participantToken: "...", // REQUIRED
},
options: { // optional
region: "us-east-1", // optional, defaults to `region` set in `connect.ChatSession.setGlobalConfig()`
},
type: "CUSTOMER", // REQUIRED
});
Creates an instance of AgentChatSession
or CustomerChatSession
, depending on the specified type
.
If you're creating a CustomerChatSession
, the chatDetails
field should be populated with the response of the StartChatContact API.
If you're creating an AgentChatSession
, you must also include amazon-connect-streams
. For example:
// order is important, alternatively use <script> tags
import "amazon-connect-streams";
import "amazon-connect-chatjs";
connect.contact(contact => {
if (contact.getType() !== connect.ContactType.CHAT) {
// applies only to CHAT contacts
return;
}
// recommended: calls `connect.ChatSession.setGlobalConfig()` and `connect.ChatSession.create()` internally
contact.onAccepted(async () => {
const cnn = contact.getConnections().find(cnn => cnn.getType() === connect.ConnectionType.AGENT);
const agentChatSession = await cnn.getMediaController();
});
// alternative: if you want control over the args of `connect.ChatSession.setGlobalConfig()` and `connect.ChatSession.create()`
contact.onAccepted(() => {
const cnn = contact.getConnections().find(cnn => cnn.getType() === connect.ConnectionType.AGENT);
const agentChatSession = connect.ChatSession.create({
chatDetails: cnn.getMediaInfo(), // REQUIRED
options: { // REQUIRED
region: "us-east-1", // REQUIRED, must match the value provided to `connect.core.initCCP()`
},
type: connect.ChatSession.SessionTypes.AGENT, // REQUIRED
websocketManager: connect.core.getWebSocketManager() // REQUIRED
});
});
});
See the amazon-connect-streams
API documentation for more information on the methods not documented here.
Note: AgentChatSession
and CustomerChatSession
are logical concepts.
As a result, the instanceof
operator will not work how you expect:
if (connect.ChatSession.create(/* ... */) instanceof connect.ChatSession) {
// this will never execute
}
connect.ChatSession.LogLevel
connect.ChatSession.LogLevel = {
DEBUG: /* ... */,
INFO: /* ... */,
WARN: /* ... */,
ERROR: /* ... */
};
Enumerates the logging levels.
connect.ChatSession.SessionTypes
connect.ChatSession.SessionTypes = {
AGENT: /* ... */,
CUSTOMER: /* ... */
};
Enumerates the session types.
The ChatSession
API divided into three sections: Amazon Connect Participant Service API wrappers, events, and other.
Functions in this section:
Promise<Response>
(except for chatSession.connect()
), where:
Response
is an aws-sdk
Response object.Promise
rejects, the error will still be a Response
object. However, the data
field will not be populated while the error
field will.metadata
arg field (except for customerChatSession.disconnectParticipant()
). The metadata
arg field is not used directly by amazon-connect-chatjs
, rather it's merely copied to the response object for usage by developers.For example:
function handleResponse(response) {
// `response` is an aws-sdk `Response` object
// `data` contains the response data
// `metadata` === "foo"
const { data, metadata } = response;
// ...
}
function handleError(response) {
// `response` is an aws-sdk `Response` object
// `error` contains the response error
// `metadata` === "foo"
const { error, metadata } = response;
// ...
}
chatSession
.getTranscript({ metadata: "foo" })
.then(handleResponse, handleError);
chatSession.connect()
// connectCalled: indicates whether the Amazon Connect Participant Service was called
// connectSuccess: indicates whether the operation succeeded
const { connectCalled, connectSuccess } = await chatSession.connect();
Wraps the CreateParticipantConnection API.
The arguments and response do not overlap with the API request or response.
Note: If the operation fails, the Promise
will reject, but the error will have the same schema as a successful response.
chatSession.getTranscript()
const awsSdkResponse = await chatSession.getTranscript({
maxResults: 100,
sortOrder: "ASCENDING"
});
const { InitialContactId, NextToken, Transcript } = awsSdkResponse.data;
Wraps the GetTranscript API.
The arguments are based on the API request body with the following differences:
camelCase
.MaxResults
defaults to 15
.ScanDirection
defaults to BACKWARD
always.SortOrder
defaults to ASCENDING
.The response data
is the same as the API response body.
Important note: In order to specify scanDirection
as FORWARD
, you need to explicitly include a startPosition
.
This is because the default startPosition
is at the most recent update to the transcript, so requesting a transcript in the FORWARD
direction from the default startPosition
is equivalent to asking for a transcript containing only messages more recent than the present (you are asking for messages in the future!).
chatSession.getAuthenticationUrl()
const awsSdkResponse = await chatSession.getAuthenticationUrl({
redirectUri: 'www.example.com',
sessionId: 'exampleId' //This comes from the authentication.initiated event
});
const authenticationUrl = getAuthenticationUrlResponse?.data?.AuthenticationUrl
Wraps the GetAuthenticationUrl API.
The arguments are based on the API request body
The response data
is the same as the API response body.
Important note: The session id is only available from the authentication.initiated event which is only emitted when the authenticate customer contact flow block is used. The session id is a 1 time use code for this api. It can be re used in the cancelParticipantAuthentication api below
chatSession.cancelParticipantAuthentication()
const awsSdkResponse = await chatSession.cancelParticipantAuthentication({
sessionId: 'exampleId' //This comes from the authentication.initiated event
});
Wraps the CancelParticipantAuthentication API.
The arguments are based on the API request body
The response data
is the same as the API response body.
Important note: The session id is only available from the authentication.initiated event which is only emitted when the authenticate customer contact flow block is used. The session id is a 1 time use code.
chatSession.sendEvent()
const awsSdkResponse = await chatSession.sendEvent({
contentType: "application/vnd.amazonaws.connect.event.typing"
});
const { AbsoluteTime, Id } = awsSdkResponse.data;
Wraps the SendEvent API.
The arguments are based on the API request body with the following differences:
camelCase
.ClientToken
cannot be specified.ContentType
allows the following values:
"application/vnd.amazonaws.connect.event.typing"
"application/vnd.amazonaws.connect.event.connection.acknowledged"
"application/vnd.amazonaws.connect.event.message.delivered"
"application/vnd.amazonaws.connect.event.message.read"
The response data
is the same as the API response body.
chatSession.sendMessage()
const awsSdkResponse = await chatSession.sendMessage({
contentType: "text/plain",
message: "Hello World!"
});
const { AbsoluteTime, Id } = awsSdkResponse.data;
Wraps the SendMessage API.
The arguments are based on the API request body with the following differences:
camelCase
.ClientToken
cannot be specified.The response data
is the same as the API response body.
chatSession.sendAttachment()
/**
* Attachment Object - the actual file to be sent between the agent and end-customer.
* Documentation: https://developer.mozilla.org/en-US/docs/Web/API/File
* @property {number} lastModified - The last modified timestamp of the file.
* @property {string} name - The name of the file.
* @property {number} size - The size of the file.
* @property {string} type - The type of the file.
* @property {string} webkitRelativePath - The relative path of the file specific to the WebKit engine.
*/
const awsSdkResponse = await chatSession.sendAttachment({
attachment: attachment
});
// Example usage
var input = document.createElement('input');
input.type = 'file';
input.addEventListener('change', (e) => {
const file = e.target.files[0];
chatSession.sendAttachment({ attachment: file })
});
Wraps the StartAttachmentUpload and CompleteAttachmentUpload API. The arguments are based on the StartAttachmentUpload and CompleteAttachmentUpload API request body with the following differences:
camelCase
.
The response data
is the same as the StartAttachmentUpload and CompleteAttachmentUpload API response body.
chatSession.sendAttachment()
invokes the StartAttachmentUpload API, uploads the Attachment to the S3 bucket using the pre-signed URL received in the StartAttachmentUpload API response and invokes the CompleteAttachmentUpload API to finish the Attachment upload process.chatSession.downloadAttachment()
const awsSdkResponse = await chatSession.downloadAttachment({
attachmentId: "string"
});
const { attachment } = awsSdkResponse.data;
/*
Attachment Object - This is the actual file that will be downloaded by either agent or end-customer.
attachment => {
lastModified: long
name: "string"
size: long
type: "string"
webkitRelativePath: "string"
}
*/
Wraps the GetAttachment API. The arguments are based on the API request body with the following differences:
camelCase
.
The response data
is the same as the API response body.
chatSession.downloadAttachment()
invokes the GetAttachment using the AttachmentId as a request parameter and fetches the Attachment from the S3 bucket using the pre-signed URL received in the GetAttachment API response.customerChatSession.disconnectParticipant()
const awsSdkResponse = await customerChatSession.disconnectParticipant();
Wraps the DisconnectParticipant API.
The arguments and response do not overlap with the API request or response.
Once this method is called, the CustomerChatSession
cannot be used anymore.
Applies only for CustomerChatSession
. See connect.ChatSession.create()
for more info.
Function in this section:
event
object that contains a chatDetails
field. See chatSession.getChatDetails()
for more info.chatSession.onConnectionBroken()
chatSession.onConnectionBroken(event => {
const { chatDetails } = event;
// ...
});
Subscribes an event handler that triggers when the session connection is broken.
chatSession.onConnectionEstablished()
chatSession.onConnectionEstablished(event => {
const { chatDetails } = event;
// ...
});
Subscribes an event handler that triggers when the session connection is established. In the case where the end customer loses network and reconnects to the chat, you can call getTranscript in this event handler to display any messages sent by the agent while end customer was offline. Make sure the next token is null in this call to getTranscript so that it returns the latest messages.
chatSession.onEnded()
chatSession.onEnded(event => {
const { chatDetails, data } = event;
// ...
});
Subscribes an event handler that triggers when the session is ended.
chatSession.onAuthenticationInitiated()
chatSession.onAuthenticationInitiated(event => {
const eventDetails = event?.data;
try {
content = JSON.parse(eventDetails?.Content);
} catch (error) {
console.error("Invalid JSON content", error);
}
const sessionId = content.SessionId;
// use the session id to call getAuthenticationUrl
});
Subscribes an event handler that triggers when the contact flow reaches the authenticate customer flow block.
chatSession.onAuthenticationSuccessful()
chatSession.onAuthenticationSuccessful(event => {
const { data } = event;
});
Subscribes an event handler that triggers when authenticate customer flow block takes the success branch.
chatSession.onAuthenticationFailed()
chatSession.onAuthenticationFailed(event => {
const { data } = event;
// ...
});
Subscribes an event handler that triggers when the authenticate customer flow block takes the failed branch.
chatSession.onAuthenticationTimeout()
chatSession.onAuthenticationTimeout(event => {
const { data } = event;
// ...
});
Subscribes an event handler that triggers when the authenticate customer flow block has timed out.
chatSession.onAuthenticationCanceled()
chatSession.onAuthenticationCanceled(event => {
const { data } = event;
// ...
});
Subscribes an event handler that triggers when the contact flow reaches the authenticate customer flow block and the cancelParticipantAuthentication API is called.
chatSession.onParticipantDisplayNameUpdated()
chatSession.onParticipantDisplayNameUpdated(event => {
const authenticatedParticipantDisplayName = event.data?.DisplayName;
// ...
});
Subscribes an event handler that triggers when the authenticate customer flow block takes the success branch and there is a customer profile associated with the user. The new display name will come in this event
chatSession.onMessage()
chatSession.onMessage(event => {
const { chatDetails, data } = event;
switch (data.ContentType) {
// ...
}
});
Subscribes an event handler that triggers whenever a message or an event (except for application/vnd.amazonaws.connect.event.typing
) is created by any participant.
The data
field has the same schema as the Item
data type from the Amazon Connect Participant Service with the addition of the following optional fields: ContactId
, InitialContactId
.
Warning The
messages
received over websocket are not guranteed to be in order!
Here is the code reference on how messages should be handled in the FrontEnd Amazon-Connect-Chat-Interface (This code handles removing messages with missing messageIds, duplicate messageIds and, sorting messages to handle display order of messages):
this.ChatJSClient.onMessage((data) => {
//deserialize message based on what UI component understands
const message = createTranscriptItem(
PARTICIPANT_MESSAGE,
{
data: data.text,
type: data.type || ContentType.MESSAGE_CONTENT_TYPE.TEXT_PLAIN,
},
this.thisParticipant
);
this._addItemsToTranscript([message]);
});
const _addItemsToTranscript = (items) => {
//filter and ignore messages not required for display
items = items.filter((item) => !_isSystemEvent(item));
//remove duplicate messageIds
const newItemMap = items.reduce((acc, item) =>
({ ...acc, [item.id]: item }), {});
//remove messages missing messageIds
const newTranscript = this.transcript.filter((item) =>
newItemMap[item.id] === undefined);
newTranscript.push(...items);
newTranscript.sort((a, b) => {
const isASending = a.transportDetails.status === Status.Sending;
const isBSending = b.transportDetails.status === Status.Sending;
if ((isASending && !isBSending) || (!isASending && isBSending)) {
return isASending ? 1 : -1;
}
return a.transportDetails.sentTime - b.transportDetails.sentTime;
});
this._updateTranscript(newTranscript);
}
const _isSystemEvent = (item) {
return Object.values(EVENT_CONTENT_TYPE).indexOf(item.contentType) !== -1;
}
const EVENT_CONTENT_TYPE: {
TYPING: "application/vnd.amazonaws.connect.event.typing",
READ_RECEIPT: "application/vnd.amazonaws.connect.event.message.read",
DELIVERED_RECEIPT: "application/vnd.amazonaws.connect.event.message.delivered",
PARTICIPANT_JOINED: "application/vnd.amazonaws.connect.event.participant.joined",
PARTICIPANT_LEFT: "application/vnd.amazonaws.connect.event.participant.left",
PARTICIPANT_INVITED: "application/vnd.amazonaws.connect.event.participant.invited",
TRANSFER_SUCCEEDED: "application/vnd.amazonaws.connect.event.transfer.succeed",
TRANSFER_FAILED: "application/vnd.amazonaws.connect.event.transfer.failed",
CONNECTION_ACKNOWLEDGED: "application/vnd.amazonaws.connect.event.connection.acknowledged",
CHAT_ENDED: "application/vnd.amazonaws.connect.event.chat.ended"
}
//send data to update Store or UI component waiting for next chat-message
_updateTranscript(transcript) {
this._triggerEvent("transcript-changed", transcript);
}
chatSession.onTyping()
chatSession.onTyping(event => {
const { chatDetails, data } = event;
if (data.ParticipantRole === "AGENT") {
// ...
}
});
Subscribes an event handler that triggers whenever a application/vnd.amazonaws.connect.event.typing
event is created by any participant.
The data
field has the same schema as chatSession.onMessage()
.
chatSession.onParticipantInvited()
/**
* Subscribes an event handler that triggers whenever a "application/vnd.amazonaws.connect.event.participant.invited" event is created by any participant.
* @param {
AbsoluteTime?: string,
ContentType?: string,
Type?: string,
ParticipantId?: string,
DisplayName?: string,
ParticipantRole?: string,
InitialContactId?: string
} event.data
*/
chatSession.onParticipantInvited(event => {
const { chatDetails, data } = event;
if (data.ParticipantRole === "AGENT") {
// ...
}
});
chatSession.onParticipantIdle()
/**
* Subscribes an event handler that triggers whenever a "application/vnd.amazonaws.connect.event.participant.idle" event is created by any participant.
* @param {
AbsoluteTime?: string,
ContentType?: string,
Type?: string,
ParticipantId?: string,
DisplayName?: string,
ParticipantRole?: string,
InitialContactId?: string
} event.data
*/
chatSession.onParticipantIdle(event => {
const { chatDetails, data } = event;
if (data.ParticipantRole === "AGENT") {
// ...
}
});
chatSession.onParticipantReturned()
/**
* Subscribes an event handler that triggers whenever a "application/vnd.amazonaws.connect.event.participant.returned" event is created by any participant.
* @param {
AbsoluteTime?: string,
ContentType?: string,
Type?: string,
ParticipantId?: string,
DisplayName?: string,
ParticipantRole?: string,
InitialContactId?: string
} event.data
*/
chatSession.onParticipantReturned(event => {
const { chatDetails, data } = event;
if (data.ParticipantRole === "AGENT") {
// ...
}
});
chatSession.onAutoDisconnection()
/**
* Subscribes an event handler that triggers whenever a "application/vnd.amazonaws.connect.event.participant.autodisconnection" event is created by any participant.
* @param {
AbsoluteTime?: string,
ContentType?: string,
Type?: string,
ParticipantId?: string,
DisplayName?: string,
ParticipantRole?: string,
InitialContactId?: string
} event.data
*/
chatSession.onAutoDisconnection(event => {
const { chatDetails, data } = event;
if (data.ParticipantRole === "AGENT") {
// ...
}
});
onParticipantIdle
, onParticipantReturned
, and onAutoDisconnection
are related to set up chat timeouts for chat participants.
chatSession.onConnectionLost()
chatSession.onConnectionLost(event => {
const { chatDetails, data } = event;
// ...
});
Subscribes an event handler that triggers when the session is lost.
chatSession.onDeepHeartbeatFailure()
chatSession.onDeepHeartbeatFailure(event => {
const { chatDetails, data } = event;
// ...
});
Subscribes an event handler that triggers when deep heartbeat fails.
chatSession.onChatRehydrated()
Note: Only when persistent chat is enabled.
/**
* Subscribes an event handler that triggers whenever a "application/vnd.amazonaws.connect.event.chat.rehydrated" event is fired.
* @param {
AbsoluteTime?: string,
ContentType?: string,
Type?: string,
ParticipantId?: string,
DisplayName?: string,
ParticipantRole?: string,
InitialContactId?: string
} event.data
*/
chatSession.onChatRehydrated(event => {
const { chatDetails, data } = event;
// Load previous transcript...
});
In version 1.2.0
the client side metric(CSM) service is added into this library. Client side metric can provide insights into the real performance and usability, it helps us to understand how customers are actually using the website and what UI experiences they prefer. This feature is enabled by default. User can also disable this feature by passing a flag: disableCSM
when they create a new chat session:
const customerChatSession = connect.ChatSession.create({
...,
disableCSM: true
});
This section contains all the functions that do not fall under the previous two categories.
chatSession.getChatDetails()
const {
contactId,
initialContactId,
participantId,
participantToken,
} = chatSession.getChatDetails();
Gets the chat session details.
chatSession.describeView()
const {
View
} = chatSession.describeView({
viewToken: "QVFJREFIaGIyaHZJWUZzNlVmMGVIY2NXdUVMMzdBTnprOGZkc3huRzhkSXR6eExOeXdGYTFwYitiOGRybklmMEZHbjBGZU1sQUFBQWJqQnNCZ2txaGtpRzl3MEJCd2FnWHpCZEFnRUFNRmdHQ1NxR1NJYjNEUUVIQVRBZUJnbGdoa2dCWlFNRUFTNHdFUVFNKys3ei9KTU41dG1BMWF4UkFnRVFnQ3NLckhKTEdXMUsyR1kvVHBUWWp0SmZxSG83VlcvOTg5WGZvckpMWDhOcGVJVHcrWUtnWjVwN3NxNGk6OlJ6STltam5rZjBiNENhOVdzM0wwaWxjR1dWUUxnb1Y1dmxNaEE5aGRkemZuV09hY0JEZFlpWFhpWnRPQlowNW9HT28xb0VnZ3JWV21aeWN0anhZZi9lOUdrYklSZVR5N2tpQmRRelFXSGpXZHpFSUExRCtvcWl5VGMzMzJoaWRldU5IaWwreEkvNmNmWUtpMXd5Qnh1aG0yY1AyWmk2byt2bTRDalFhWGxaM0Zrb1dqLy91aDVvRmtZcDY4UERuU0ZVQ1AyUU0zcjhNazI1ckZ2M0p6Z210bnMrSzVYY2VPN0xqWE1JMHZ0RE5uVEVYR1ZDcnc3SE82R0JITjV4NWporWGM9\\\", //REQUIRED
metadata: "foo" //OPTIONAL
}).data;
Wraps the DescribeView API.
The arguments are based on the API model with the following differences:
ChatJS automatically supplies the connectionToken via the session's internal data.
This api will only function after chatSession.connect()
succeeds.
agentChatSession.cleanUpOnParticipantDisconnect()
agentChatSession.cleanUpOnParticipantDisconnect();
Cleans up all event handlers.
Applies only for AgentChatSession
. See connect.ChatSession.create()
for more info.
Reconnect to an active chat after refreshing the browser. Call the CreateParticipantConnection
API on refresh with the same ParticipantToken
generated from the initial StartChatContact
request.
StartChatContact
API: initiate the chat contact [Documentation]CreateParticipantConnection
API: create the participant's connection [Documentation]ParticipantToken
gets stored// Option #1 - Invoking the startChatContactAPI lambda CloudFormation template
// https://github.com/amazon-connect/amazon-connect-chat-ui-examples/tree/master/cloudformationTemplates/startChatContactAPI
var contactFlowId = "12345678-1234-1234-1234-123456789012";
var instanceId = "12345678-1234-1234-1234-123456789012";
var apiGatewayEndpoint = "https://<api-id>.execute-api.<region>.amazonaws.com/Prod/";
var region = "<region>";
// Details passed to the lambda
const initiateChatRequest = {
ParticipantDetails: {
DisplayName: name
},
ContactFlowId: contactFlowId,
InstanceId: instanceId,
Attributes: JSON.stringify({
"customerName": name // pass this to the Contact flow
}),
SupportedMessagingContentTypes: ["text/plain", "text/markdown"]
};
window.fetch(apiGatewayEndpoint, {
method: 'post',
body: JSON.stringify(initiateChatRequest),
})
.then((res) => res.json())
.then((res) => {
return res.data.startChatResult;
})
.catch((err) => {
console.error('StartChatContact Failure', err)
});
// StartChatContact response gets stored
const initialStartChatResponse = {
ContactId,
ParticipantId,
ParticipantToken
};
// Option #2 - Invoking the AWK SDK `connect.startChatContact`
var AWS = require('aws-sdk');
AWS.config.update({region: process.env.REGION});
var connect = new AWS.Connect();
// https://docs.aws.amazon.com/connect/latest/APIReference/API_StartChatContact.html
const startChatRequest = {
ParticipantDetails: {
DisplayName: "Customer1"
},
ContactFlowId: contactFlowId,
InstanceId: instanceId,
Attributes: JSON.stringify({
customerName: "Customer1" // pass this to the Contact flow
}),
SupportedMessagingContentTypes: ["text/plain", "text/markdown"]
};
// Initial StartChatContact call
connect.startChatContact(startChatRequest, function(err, data) {
if (err) {
console.log("Error starting the chat.", err);
reject(err);
} else {
console.log("Start chat succeeded with the response: " + JSON.stringify(data));
resolve(data);
}
});
CreateParticipantConnection
request is made, customer initializes chat session// global "connect" imported from `amazon-connect-chatjs`
var chatSession;
// Initial CreateParticipantConnection call
chatSession = await connect.ChatSession.create({
options: { // optional
region: "us-west-2", // optional, defaults to `region` set in `connect.ChatSession.setGlobalConfig()`
},
chatDetails: {
ContactId
ParticipantId
ParticipantToken // <---- from initialStartChatResponse
},
type: "CUSTOMER",
});
await chatSession.connect();
await chatSession.sendMessage({
contentType: "text/plain",
message: "Hello World!"
});
// Browser is refreshed
location.reload();
CreateParticipantConnection
request is made with the initial ParticipantToken
// Second CreateParticipantConnection request
chatSession = await connect.ChatSession.create({
chatDetails: {
ContactId
ParticipantId
ParticipantToken // <---- from initialStartChatResponse
},
type: "CUSTOMER",
});
await chatSession.connect();
await chatSession.sendMessage({
contentType: "text/plain",
message: "Hello World!"
});
For latest documentation, please follow instructions in "Admin guide: Enable persistent chat"
Persistent chats enable customers to resume previous conversations with the context, metadata, and transcripts carried over, eliminating the need for customers to repeat themselves and allowing agents to provide personalized service with access to the entire conversation history. To set up persistent chat experiences, simply provide a previous contact id when calling the StartChatContact API to create a new chat contact.
Learn more about persistent chat: https://docs.aws.amazon.com/connect/latest/adminguide/chat-persistence.html
⚠️ Only chat sessions that have ended are allowed to rehydrate onto a new chat session.
Chat transcripts are pulled from previous chat contacts, and displayed to the customer and agent.
To enable persistent chat, provide the previous contactId
in the SourceContactId
parameter of StartChatContact
API.
PUT /contact/chat HTTP/1.1
Content-type: application/json
{
"Attributes": {
"string" : "string"
},
"ContactFlowId": "string",
"InitialMessage": {
"Content": "string",
"ContentType": "string"
},
"InstanceId": "string",
... // other chat fields
// NEW Attribute for persistent chat
"PersistentChat" : {
"SourceContactId": "2222222-aaaa-bbbb-2222-222222222222222"
"RehydrationType": "FROM_SEGMENT" // ENTIRE_PAST_SESSION | FROM_SEGMENT
}
}
You can configure Amazon Connect to scan attachments that are sent during a chat or uploaded to a case. You can scan attachments by using your preferred scanning application. For example, you can scan attachments for malware before they are approved to be shared between participants of a chat.
Learn more about attachment scanning: https://docs.aws.amazon.com/connect/latest/adminguide/setup-attachment-scanning.html
Amazon Connect will send the result (APPROVED | REJECTED) of the scanned attachment as a message in the websocket. To render it in your chat, you can use the following example code in the onMessage handler
this.client.onMessage(data => {
const attachmentStatus = data.data.Attachments?.[0]?.Status;
if (attachmentStatus === 'REJECTED') {
renderAttachmentError(attachmentStatus) //your custom implementation
}
});
[3.0.3]
FAQs
Provides chat support to AmazonConnect customers
The npm package amazon-connect-chatjs receives a total of 13,530 weekly downloads. As such, amazon-connect-chatjs popularity was classified as popular.
We found that amazon-connect-chatjs demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
Research
Security News
The Socket Research Team has discovered six new malicious npm packages linked to North Korea’s Lazarus Group, designed to steal credentials and deploy backdoors.
Security News
Socket CEO Feross Aboukhadijeh discusses the open web, open source security, and how Socket tackles software supply chain attacks on The Pair Program podcast.