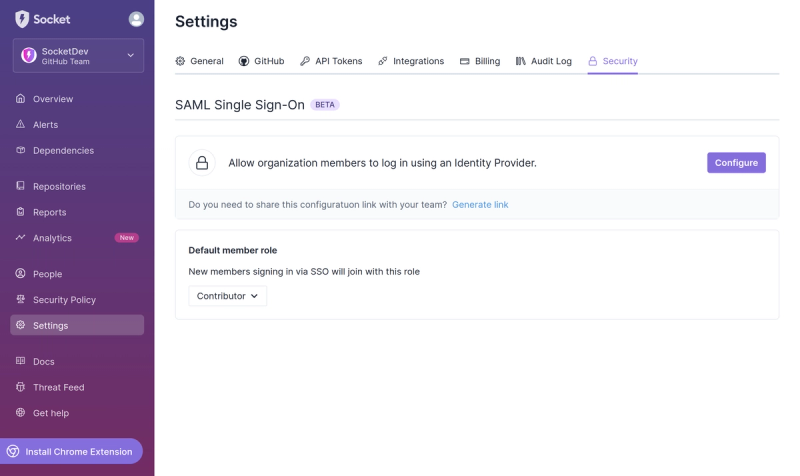
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
amp-utils
Advanced tools
Readme
A collection of handy utilities.
npm install amp-utils
const amp = require('amp-utils');
amp.string.slug('This string will be slugged.');
amp.array.move(array, from, to)
Move an item within an array. Returns Array
.
array
: The target Array
from
: The old Integer
index of the array itemto
: The new Integer
index of the array itemconst breakfast = [
'eggs',
'toast',
'bacon',
];
amp.array.move(breakfast, 2, 0); // ['bacon', 'eggs', 'toast']
amp.array.unique(array)
Get unique items from an array. Returns Array
.
array
: The target Array
amp.array.unique(['a', 'a', 'b', 'c', 'c']); // ['a', 'b', 'c']
amp.html.closest(start, selector)
Get the closest matching HTML element parent by CSS selector. Returns HTMLElement
or null
(if no matching parent is found).
start
: The starting HTMLElement
selector
: A valid CSS selector String
for matching parent elementsamp.html.closest(document.getElementById('menu'), '.menu-container');
amp.html.matches(el, selector)
Does this DOM element match the provided CSS selector? Returns Boolean
.
el
: The HTMLElement
for comparisonselector
: A valid CSS selector String
for matchingamp.html.matches(document.getElementById('menu'), '.menu');
amp.object.byPath(search, path, value)
Get or set object value by key path. Returns Object
or null
if the target object is not found.
search
: The Object
that will be searchedpath
: The String
path to the target object or value in dot notation (for example, 'parent.child.grandchild')value
: Set target object to this value instead of getting its valueconst ride = {
type: 'Truck',
wheels: 4,
passengers: {
driver: {
name: 'Edith',
age: 30,
},
shotgun: {
name: 'Edmund',
age: 29,
}
},
};
amp.object.byPath(ride, 'type'); // 'Truck'
amp.object.byPath(ride, 'passengers.driver.name'); // 'Edith'
amp.object.byPath(ride, 'passengers.shotgun.name', 'Joe'); // Object ('Edmund' is now 'Joe')
amp.object.clone(obj)
Clone an object. Returns Object
.
obj
: The Object
that will be clonedconst original = {
a: 'one',
b: {
one: [1, 2, 3],
},
};
const clone = amp.object.clone(original);
amp.object.equal(a, b)
Compare two Objects
for equality. Returns Boolean
.
a
: The first Object
for comparisonb
: The second Object
for comparisonamp.object.equal({ a: 'A'}, { a: 'A'}); // true
amp.object.equal({ a: 'A' }, { a: 'B' }); // false
amp.object.is(obj)
Is this an object? Returns Boolean
.
obj
: The Object
in questionamp.object.is({ a: 'A' }); // true
amp.object.is('A string!'); // false
amp.object.merge(target, ...sources)
Deep merge two or more objects. Returns Object
.
target
: Properties will be copied into this Object
sources
: One or more source Objects
to merge into the targetamp.object.merge({ a: 'A' }, { b: 'B' }); // { a: 'A', b: 'B' }
amp.object.merge({ a: 'A' }, { a: 'B' }); // { a: 'B' }
amp.object.options(defaultConfig, config)
Build a configuration object with default values. Returns Object
. This is similar to the amp.object.merge()
method, but does not overwrite the default configuration values. Note that you may also use amp.options()
, a synonym for this method.
defaultConfig
: Default configuration options Object
config
: Configuration options Object
, will overwrite default optionsconst getAnimal = (config) => {
const options = amp.object.options({
name: 'Moby',
type: 'dog',
}, config);
return options;
};
getAnimal({ name: 'Mighty' }); // { name: 'Mighty', type: 'dog' }
getAnimal({ name: 'Edith', type: 'cat' }); // { name: 'Edith', type: 'cat' }
getAnimal({ name: 'T-Bone', type: 'bird', age: 3 }); // { name: 'T-Bone', type: 'bird', age: 3 }
amp.queryString.get(uri, key)
Parse query string for a parameter value. Returns String
or null
if no value is found.
uri
: The URI or query String
key
: The query string parameter name (String
)const url = '?name=Edmund&type=cat';
amp.queryString.get(url, 'name'); // 'Edmund'
amp.queryString.get(url, 'type'); // 'cat'
amp.queryString.set(uri, key, value)
Update query string with a new parameter value. Returns String
.
uri
: The URI or query String
key
: The query string parameter name (String
)value
: The new parameter valueconst url = '?name=Edmund&type=cat';
amp.queryString.set(url, 'name', 'Edith'); // '?name=Edith&type=cat'
amp.queryString.set(url, 'age', '3'); // '?name=Edmund&type=cat&age=3'
amp.string.guid()
Generate a globally unique identifier (GUID) string. Returns String
.
amp.string.guid(); // '5aac52a7-d431-aecc-8d35-ef18bf1cbb85'
amp.string.guid(); // 'f2295534-89e3-50cd-18d7-120c62311ac7'
amp.string.slug(str)
Slugify the given string. Returns String
.
str
: The String
to slugifyamp.string.slug('Pomp & Circumstance'); // 'pomp-circumstance'
amp.string.titleCase(str)
Transform a string to title case. Returns String
.
str
: The String
to transformamp.string.titleCase('eine kleine nachtmusik'); // 'Eine Kleine Nachtmusik'
amp.string.trimSlashes(path)
Trim slashes from a string or path. Returns String
.
path
: The String
that will be trimmedamp.string.trimSlashes('/dogs/moby/fetch/'); // 'dogs/moby/fetch'
FAQs
A collection of handy utilities.
The npm package amp-utils receives a total of 5 weekly downloads. As such, amp-utils popularity was classified as not popular.
We found that amp-utils demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.