ansi-colors
Advanced tools
Comparing version 3.1.0 to 3.2.0
31
index.js
@@ -6,10 +6,10 @@ 'use strict'; | ||
if ('FORCE_COLOR' in process.env) { | ||
colors.enabled = process.env.FORCE_COLOR !== '0' ? true : false; | ||
colors.enabled = process.env.FORCE_COLOR !== '0'; | ||
} | ||
const ansi = codes => { | ||
codes.open = `\u001b[${codes[0]}m`; | ||
codes.close = `\u001b[${codes[1]}m`; | ||
codes.regex = new RegExp(`\\u001b\\[${codes[1]}m`, 'g'); | ||
return codes; | ||
const ansi = style => { | ||
style.open = `\u001b[${style.codes[0]}m`; | ||
style.close = `\u001b[${style.codes[1]}m`; | ||
style.regex = new RegExp(`\\u001b\\[${style.codes[1]}m`, 'g'); | ||
return style; | ||
}; | ||
@@ -19,3 +19,3 @@ | ||
let { open, close, regex } = style; | ||
str = open + (str.includes(close) ? str.replace(regex, open) : str) + close; | ||
str = open + (str.includes(close) ? str.replace(regex, close + open) : str) + close; | ||
// see https://github.com/chalk/chalk/pull/92, thanks to the | ||
@@ -39,3 +39,3 @@ // chalk contributors for this fix. However, we've confirmed that | ||
const define = (name, codes, type) => { | ||
colors.styles[name] = ansi(codes); | ||
colors.styles[name] = ansi({ name, codes }); | ||
let t = colors.keys[type] || (colors.keys[type] = []); | ||
@@ -102,14 +102,9 @@ t.push(name); | ||
/* eslint-disable no-control-regex */ | ||
// ansiRegex modified from node.js readline: https://git.io/fNWFP, which itself | ||
// is adopted from regex used for ansi escape code splitting in ansi-regex | ||
// Adopted from https://github.com/chalk/ansi-regex/blob/master/index.js | ||
// License: MIT, authors: @sindresorhus, Qix-, and arjunmehta Matches all | ||
// ansi escape code sequences in a string | ||
colors.ansiRegex = /[\u001b\u009b][[()#;?]*(?:[0-9]{1,4}(?:;[0-9]{0,4})*)?[0-9A-ORZcf-nqry=><]/gm; | ||
colors.hasAnsi = str => str && typeof str === 'string' && colors.ansiRegex.test(str); | ||
colors.unstyle = str => typeof str === 'string' ? str.replace(colors.ansiRegex, '') : str; | ||
colors.none = colors.clear = str => str; // noop, for programmatic usage | ||
const re = colors.ansiRegex = /\u001b\[\d+m/gm; | ||
colors.hasColor = colors.hasAnsi = str => !!str && typeof str === 'string' && re.test(str); | ||
colors.unstyle = str => typeof str === 'string' ? str.replace(re, '') : str; | ||
colors.none = colors.clear = colors.noop = str => str; // no-op, for programmatic usage | ||
colors.stripColor = colors.unstyle; | ||
colors.hasColor = colors.hasAnsi; | ||
colors.symbols = require('./symbols'); | ||
colors.define = define; | ||
module.exports = colors; |
{ | ||
"name": "ansi-colors", | ||
"description": "Easily add ANSI colors to your text and symbols in the terminal. A faster drop-in replacement for chalk, kleur and turbocolor (without the dependencies and rendering bugs).", | ||
"version": "3.1.0", | ||
"version": "3.2.0", | ||
"homepage": "https://github.com/doowb/ansi-colors", | ||
@@ -103,7 +103,8 @@ "author": "Brian Woodward (https://github.com/doowb)", | ||
"reflinks": [ | ||
"chalk", | ||
"colorette", | ||
"colors", | ||
"kleur", | ||
"supports-color" | ||
"kleur" | ||
] | ||
} | ||
} |
@@ -21,3 +21,3 @@ # ansi-colors [](https://www.npmjs.com/package/ansi-colors) [](https://npmjs.org/package/ansi-colors) [](https://npmjs.org/package/ansi-colors) [](https://travis-ci.org/doowb/ansi-colors) [](https://ci.appveyor.com/project/doowb/ansi-colors) | ||
* _Blazing fast_ - fastest terminal styling library in node.js, 10-20x faster than chalk! (See [Beware of false claims!](#beware-of-false-claims))! | ||
* _Blazing fast_ - Fastest terminal styling library in node.js, 10-20x faster than chalk! | ||
@@ -28,3 +28,3 @@ * _Drop-in replacement_ for [chalk](https://github.com/chalk/chalk). | ||
* _Safe_ - Does not modify the `String.prototype` like [colors](https://github.com/Marak/colors.js). | ||
* Supports [nested colors](#nested-colors). | ||
* Supports [nested colors](#nested-colors), **and does not have the [nested styling bug](#nested styling bug) that is present in [colorette](https://github.com/jorgebucaran/colorette), [chalk](https://github.com/chalk/chalk), and [kleur](https://github.com/lukeed/kleur)**. | ||
* Supports [chained colors](#chained-colors). | ||
@@ -46,3 +46,3 @@ * [Toggle color support](#toggle-color-support) on or off. | ||
### Chained colors | ||
## Chained colors | ||
@@ -57,3 +57,3 @@ ```js | ||
### Nested colors | ||
## Nested colors | ||
@@ -66,4 +66,28 @@ ```js | ||
### Toggle color support | ||
### Nested styling bug | ||
`ansi-colors` does not have the nested styling bug found in [colorette](https://github.com/jorgebucaran/colorette), [chalk](https://github.com/chalk/chalk), and [kleur](https://github.com/lukeed/kleur). | ||
```js | ||
const { bold, red } = require('ansi-styles'); | ||
console.log(bold(`foo ${red.dim('bar')} baz`)); | ||
const colorette = require('colorette'); | ||
console.log(colorette.bold(`foo ${colorette.red(colorette.dim('bar'))} baz`)); | ||
const kleur = require('kleur'); | ||
console.log(kleur.bold(`foo ${kleur.red.dim('bar')} baz`)); | ||
const chalk = require('chalk'); | ||
console.log(chalk.bold(`foo ${chalk.red.dim('bar')} baz`)); | ||
``` | ||
**Results in the following** | ||
(sans icons and labels) | ||
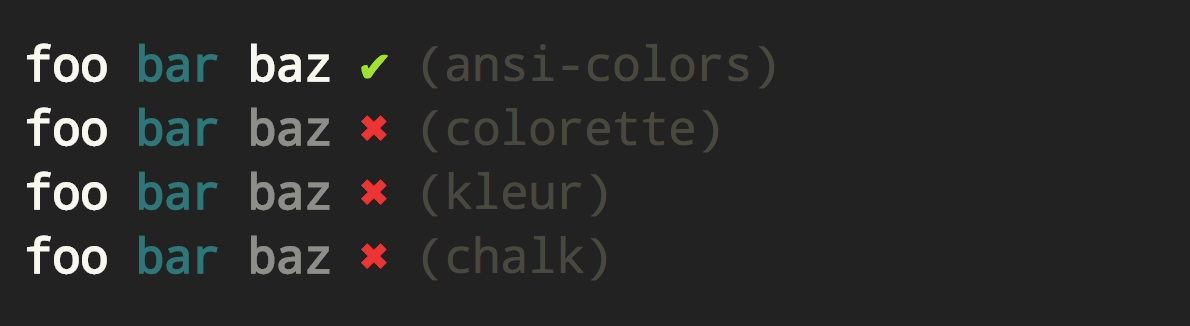 | ||
## Toggle color support | ||
Easily enable/disable colors. | ||
@@ -132,49 +156,2 @@ | ||
<details> | ||
<summary><strong>Beware of false claims!</strong></summary> | ||
### Kleur and turbocolor are buggy and incomplete | ||
tldr; kleur and turbocolor do not have parity with chalk or ansi-colors, and they fail too many of the unit tests to be included in our benchmarks. | ||
You might have seen claims from [kleur](https://github.com/lukeed/kleur) or [turbocolor](https://github.com/jorgebucaran/turbocolor) that they are "faster than ansi-colors". Both libraries are unofficial forks of ansi-colors, and in an attempt to appear faster and differentiate from ansi-colors, _both libraries removed crucial code that was necessary for resetting chained colors_. | ||
To illustrate the bug, simply do the following with `kleur` (as of v2.0.1): | ||
```js | ||
const kleur = require('kleur'); | ||
const red = kleur.bold.underline.red; | ||
console.log(kleur.bold('I should be bold and white')); | ||
const blue = kleur.underline.blue; | ||
console.log(kleur.underline('I should be underlined and white')); | ||
``` | ||
Same with `turbocolor` (as of v2.4.5): | ||
```js | ||
const turbocolor = require('turbocolor'); | ||
const red = turbocolor.bold.underline.red; | ||
console.log(turbocolor.bold('I should be bold and white')); | ||
const blue = turbocolor.underline.blue; | ||
console.log(turbocolor.underline('I should be underlined and white')); | ||
``` | ||
Both libraries render the following: | ||
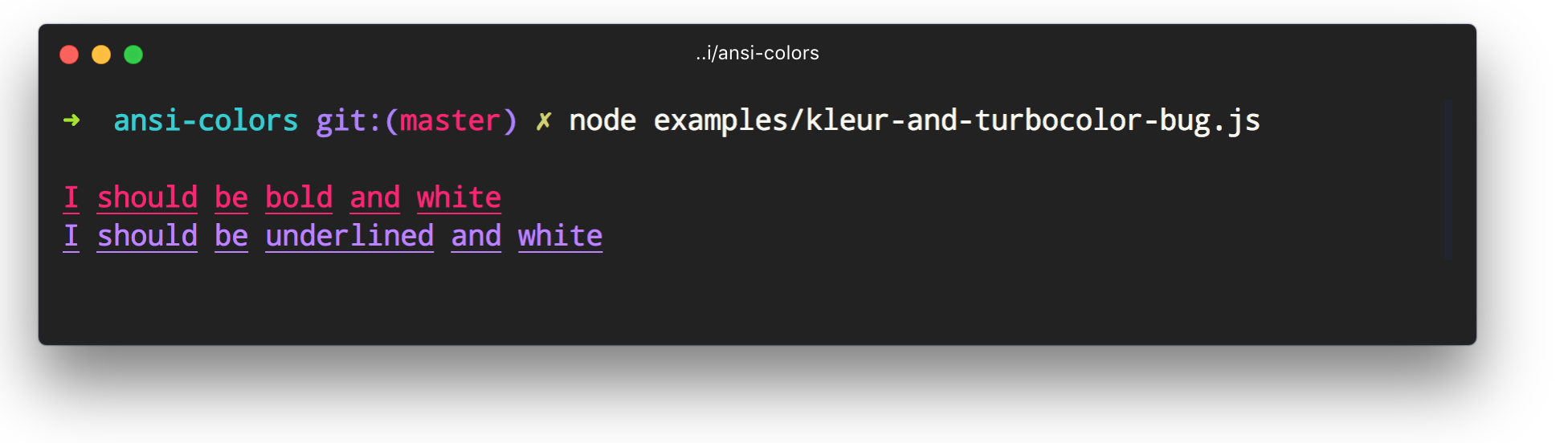 | ||
**Other pitfalls** | ||
Beyond the aforementioned rendering bug, neither kleur nor turbocolor can be used as a drop-in replacement for chalk: | ||
* both libraries omit code that prevents background bleed around newlines (try doing `console.log(kleur.bgRed('foo\nbar') + 'baz qux')` and `console.log(turbocolor.bgRed('foo\nbar') + 'baz qux')`). | ||
* both libraries fail half of the ansi-colors unit tests (chalk passes them all) | ||
* neither library supports bright colors (chalk and ansi-colors do) | ||
* neither library supports bright-background colors (chalk and ansi-colors do) | ||
* turbocolor swaps bright-background colors for background colors. (surprise! turbocolor gives you unexpected colors in the terminal!) | ||
</details> | ||
### Mac | ||
@@ -279,5 +256,6 @@ | ||
| 35 | [doowb](https://github.com/doowb) | | ||
| 26 | [jonschlinkert](https://github.com/jonschlinkert) | | ||
| 34 | [jonschlinkert](https://github.com/jonschlinkert) | | ||
| 6 | [lukeed](https://github.com/lukeed) | | ||
| 2 | [Silic0nS0ldier](https://github.com/Silic0nS0ldier) | | ||
| 1 | [dwieeb](https://github.com/dwieeb) | | ||
| 1 | [jorgebucaran](https://github.com/jorgebucaran) | | ||
@@ -302,2 +280,2 @@ | 1 | [madhavarshney](https://github.com/madhavarshney) | | ||
_This file was generated by [verb-generate-readme](https://github.com/verbose/verb-generate-readme), v0.6.0, on September 20, 2018._ | ||
_This file was generated by [verb-generate-readme](https://github.com/verbose/verb-generate-readme), v0.6.0, on October 22, 2018._ |
Environment variable access
Supply chain riskPackage accesses environment variables, which may be a sign of credential stuffing or data theft.
Found 1 instance in 1 package
Environment variable access
Supply chain riskPackage accesses environment variables, which may be a sign of credential stuffing or data theft.
Found 1 instance in 1 package
2
20292
276
273