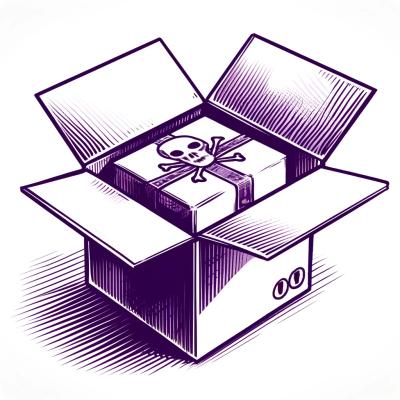
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Functions that surround a string with ansicolor codes so it prints in color.
The ansicolors npm package is designed for adding color and style to text in the console. It provides a simple and lightweight way to enhance the readability and appearance of console output by applying various color and style transformations.
Text Coloring
This feature allows you to change the color of your text output in the console. The example demonstrates how to make text appear in blue.
"const ansicolors = require('ansicolors');\nconsole.log(ansicolors.blue('Hello world in blue'));"
Text Styling
This feature enables you to apply styles such as bolding to your text output. The example shows how to make text bold.
"const ansicolors = require('ansicolors');\nconsole.log(ansicolors.bold('Bold text'));"
Background Coloring
With this feature, you can set the background color of your text output. The example illustrates setting a green background for the text.
"const ansicolors = require('ansicolors');\nconsole.log(ansicolors.bgGreen('Text with green background'));"
Chalk is a popular npm package that offers a wide range of text styling options, including color, background color, and text styles. It has a more fluent interface compared to ansicolors, allowing for chaining styles together.
The colors package also allows for adding colors and styles to text in the console. It extends String.prototype to add color and style methods directly to strings, which is a different approach compared to ansicolors that uses function calls.
Functions that surround a string with ansicolor codes so it prints in color.
In case you need styles, like bold
, have a look at ansistyles.
npm install ansicolors
var colors = require('ansicolors');
// foreground colors
var redHerring = colors.red('herring');
var blueMoon = colors.blue('moon');
var brighBlueMoon = colors.brightBlue('moon');
console.log(redHerring); // this will print 'herring' in red
console.log(blueMoon); // this 'moon' in blue
console.log(brightBlueMoon); // I think you got the idea
// background colors
console.log(colors.bgYellow('printed on yellow background'));
console.log(colors.bgBrightBlue('printed on bright blue background'));
// mixing background and foreground colors
// below two lines have same result (order in which bg and fg are combined doesn't matter)
console.log(colors.bgYellow(colors.blue('printed on yellow background in blue')));
console.log(colors.blue(colors.bgYellow('printed on yellow background in blue')));
ansicolors allows you to access opening and closing escape sequences separately.
var colors = require('ansicolors');
function inspect(obj, depth) {
return require('util').inspect(obj, false, depth || 5, true);
}
console.log('open blue', inspect(colors.open.blue));
console.log('close bgBlack', inspect(colors.close.bgBlack));
// => open blue '\u001b[34m'
// close bgBlack '\u001b[49m'
Look at the tests to see more examples and/or run them via:
npm explore ansicolors && npm test
ansicolors tries to meet simple use cases with a very simple API. However, if you need a more powerful ansi formatting tool, I'd suggest to look at the features of the ansi module.
FAQs
Functions that surround a string with ansicolor codes so it prints in color.
The npm package ansicolors receives a total of 1,957,863 weekly downloads. As such, ansicolors popularity was classified as popular.
We found that ansicolors demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.