anymatch
Advanced tools
Comparing version 2.0.0 to 3.0.0
141
index.js
'use strict'; | ||
var micromatch = require('micromatch'); | ||
var normalize = require('normalize-path'); | ||
var path = require('path'); // required for tests. | ||
var arrify = function(a) { return a == null ? [] : (Array.isArray(a) ? a : [a]); }; | ||
const picomatch = require('picomatch'); | ||
const normalizePath = require('normalize-path'); | ||
var anymatch = function(criteria, value, returnIndex, startIndex, endIndex) { | ||
criteria = arrify(criteria); | ||
value = arrify(value); | ||
if (arguments.length === 1) { | ||
return anymatch.bind(null, criteria.map(function(criterion) { | ||
return typeof criterion === 'string' && criterion[0] !== '!' ? | ||
micromatch.matcher(criterion) : criterion; | ||
})); | ||
/** | ||
* @typedef {(...args) => boolean} BooleanFn | ||
* @typedef {(string) => boolean} StrBoolFn | ||
* @typedef {BooleanFn|StrBoolFn} AnymatchFn | ||
* @typedef {string|RegExp|AnymatchFn} AnymatchPattern | ||
* @typedef {AnymatchPattern|Array<AnymatchPattern>} AnymatchMatcher | ||
*/ | ||
const BANG = '!'; | ||
const arrify = (item) => Array.isArray(item) ? item : [item]; | ||
/** | ||
* @param {AnymatchPattern} matcher | ||
* @returns {AnymatchFn} | ||
*/ | ||
const createPattern = (matcher) => { | ||
if (typeof matcher === 'function') { | ||
return matcher; | ||
} | ||
startIndex = startIndex || 0; | ||
var string = value[0]; | ||
var altString, altValue; | ||
var matched = false; | ||
var matchIndex = -1; | ||
function testCriteria(criterion, index) { | ||
var result; | ||
switch (Object.prototype.toString.call(criterion)) { | ||
case '[object String]': | ||
result = string === criterion || altString && altString === criterion; | ||
result = result || micromatch.isMatch(string, criterion); | ||
break; | ||
case '[object RegExp]': | ||
result = criterion.test(string) || altString && criterion.test(altString); | ||
break; | ||
case '[object Function]': | ||
result = criterion.apply(null, value); | ||
result = result || altValue && criterion.apply(null, altValue); | ||
break; | ||
default: | ||
result = false; | ||
if (typeof matcher === 'string') { | ||
const glob = picomatch(matcher); | ||
return (string) => matcher === string || glob(string); | ||
} | ||
if (matcher instanceof RegExp) { | ||
return (string) => matcher.test(string); | ||
} | ||
return (string) => false; | ||
}; | ||
/** | ||
* @param {Array<Function>} patterns | ||
* @param {Array<Function>} negatedGlobs | ||
* @param {String|Array} path | ||
* @param {Boolean} returnIndex | ||
*/ | ||
const matchPatterns = (patterns, negatedGlobs, path, returnIndex) => { | ||
const additionalArgs = Array.isArray(path); | ||
const upath = normalizePath(additionalArgs ? path[0] : path); | ||
if (negatedGlobs.length > 0) { | ||
for (let index = 0; index < negatedGlobs.length; index++) { | ||
const nglob = negatedGlobs[index]; | ||
if (nglob(upath)) { | ||
return returnIndex ? -1 : false; | ||
} | ||
} | ||
if (result) { | ||
matchIndex = index + startIndex; | ||
} | ||
return result; | ||
} | ||
var crit = criteria; | ||
var negGlobs = crit.reduce(function(arr, criterion, index) { | ||
if (typeof criterion === 'string' && criterion[0] === '!') { | ||
if (crit === criteria) { | ||
// make a copy before modifying | ||
crit = crit.slice(); | ||
for (let index = 0; index < patterns.length; index++) { | ||
const pattern = patterns[index]; | ||
if (additionalArgs) { | ||
if (pattern(...path)) { | ||
return returnIndex ? index : true; | ||
} | ||
crit[index] = null; | ||
arr.push(criterion.substr(1)); | ||
} else { | ||
if (pattern(upath)) { | ||
return returnIndex ? index : true; | ||
} | ||
} | ||
return arr; | ||
}, []); | ||
if (!negGlobs.length || !micromatch.any(string, negGlobs)) { | ||
if (path.sep === '\\' && typeof string === 'string') { | ||
altString = normalize(string); | ||
altString = altString === string ? null : altString; | ||
if (altString) altValue = [altString].concat(value.slice(1)); | ||
} | ||
return returnIndex ? -1 : false; | ||
}; | ||
/** | ||
* @param {AnymatchMatcher} matchers | ||
* @param {Array|string} testString | ||
* @param {Boolean=} returnIndex | ||
* @returns {boolean|number|Function} | ||
*/ | ||
const anymatch = (matchers, testString, returnIndex = false) => { | ||
if (matchers == null) { | ||
throw new TypeError('anymatch: specify first argument'); | ||
} | ||
// Early cache for matchers. | ||
const mtchers = arrify(matchers); | ||
const negatedGlobs = mtchers | ||
.filter(item => typeof item === 'string' && item.charAt(0) === BANG) | ||
.map(item => item.slice(1)) | ||
.map(item => picomatch(item)); | ||
const patterns = mtchers.map(createPattern); | ||
if (testString == null) { | ||
return (testString, ri = false) => { | ||
const returnIndex = typeof ri === 'boolean' ? ri : false; | ||
return matchPatterns(patterns, negatedGlobs, testString, returnIndex); | ||
} | ||
matched = crit.slice(startIndex, endIndex).some(testCriteria); | ||
} | ||
return returnIndex === true ? matchIndex : matched; | ||
if (!Array.isArray(testString) && typeof testString !== 'string') { | ||
throw new TypeError('anymatch: second argument must be a string: got ' + | ||
Object.prototype.toString.call(testString)) | ||
} | ||
return matchPatterns(patterns, negatedGlobs, testString, returnIndex); | ||
}; | ||
module.exports = anymatch; |
{ | ||
"name": "anymatch", | ||
"version": "2.0.0", | ||
"version": "3.0.0", | ||
"description": "Matches strings against configurable strings, globs, regular expressions, and/or functions", | ||
"files": [ | ||
"index.js" | ||
"index.js", | ||
"index.d.ts" | ||
], | ||
@@ -36,13 +37,13 @@ "author": { | ||
"scripts": { | ||
"test": "istanbul cover _mocha && cat ./coverage/lcov.info | coveralls" | ||
"test": "nyc mocha", | ||
"mocha": "mocha" | ||
}, | ||
"dependencies": { | ||
"micromatch": "^3.1.4", | ||
"normalize-path": "^2.1.1" | ||
"normalize-path": "^3.0.0", | ||
"picomatch": "^2.0.3" | ||
}, | ||
"devDependencies": { | ||
"coveralls": "^2.7.0", | ||
"istanbul": "^0.4.5", | ||
"mocha": "^3.0.0" | ||
"mocha": "^6.1.2", | ||
"nyc": "^13.3.0" | ||
} | ||
} |
@@ -10,4 +10,2 @@ anymatch [](https://travis-ci.org/micromatch/anymatch) [](https://coveralls.io/r/micromatch/anymatch?branch=master) | ||
[](https://nodei.co/npm/anymatch/) | ||
[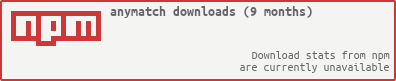](https://nodei.co/npm-dl/anymatch/) | ||
@@ -17,6 +15,6 @@ Usage | ||
```sh | ||
npm install anymatch --save | ||
npm install anymatch | ||
``` | ||
#### anymatch (matchers, testString, [returnIndex], [startIndex], [endIndex]) | ||
#### anymatch(matchers, testString, [returnIndex]) | ||
* __matchers__: (_Array|String|RegExp|Function_) | ||
@@ -33,19 +31,7 @@ String to be directly matched, string with glob patterns, regular expression | ||
boolean result. | ||
* __startIndex, endIndex__: (_Integer [optional]_) Can be used to define a | ||
subset out of the array of provided matchers to test against. Can be useful | ||
with bound matcher functions (see below). When used with `returnIndex = true` | ||
preserves original indexing. Behaves the same as `Array.prototype.slice` (i.e. | ||
includes array members up to, but not including endIndex). | ||
```js | ||
var anymatch = require('anymatch'); | ||
const anymatch = require('anymatch'); | ||
var matchers = [ | ||
'path/to/file.js', | ||
'path/anyjs/**/*.js', | ||
/foo\.js$/, | ||
function (string) { | ||
return string.indexOf('bar') !== -1 && string.length > 10 | ||
} | ||
]; | ||
const matchers = [ 'path/to/file.js', 'path/anyjs/**/*.js', /foo.js$/, string => string.includes('bar') && string.length > 10 ] ; | ||
@@ -62,7 +48,2 @@ anymatch(matchers, 'path/to/file.js'); // true | ||
// skip matchers | ||
anymatch(matchers, 'path/to/file.js', false, 1); // false | ||
anymatch(matchers, 'path/anyjs/foo.js', true, 2, 3); // 2 | ||
anymatch(matchers, 'path/to/bar.js', true, 0, 3); // -1 | ||
// using globs to match directories and their children | ||
@@ -74,8 +55,13 @@ anymatch('node_modules', 'node_modules'); // true | ||
anymatch('**/node_modules/**', '/absolute/path/to/node_modules/somelib/index.js'); // true | ||
const matcher = anymatch(matchers); | ||
['foo.js', 'bar.js'].filter(matcher); // [ 'foo.js' ] | ||
anymatch master* ❯ | ||
``` | ||
#### anymatch (matchers) | ||
#### anymatch(matchers) | ||
You can also pass in only your matcher(s) to get a curried function that has | ||
already been bound to the provided matching criteria. This can be used as an | ||
`Array.prototype.filter` callback. | ||
`Array#filter` callback. | ||
@@ -87,3 +73,2 @@ ```js | ||
matcher('path/anyjs/baz.js', true); // 1 | ||
matcher('path/anyjs/baz.js', true, 2); // -1 | ||
@@ -97,5 +82,5 @@ ['foo.js', 'bar.js'].filter(matcher); // ['foo.js'] | ||
NOTE: As of v2.0.0, [micromatch](https://github.com/jonschlinkert/micromatch) moves away from minimatch-parity and inline with Bash. This includes handling backslashes differently (see https://github.com/micromatch/micromatch#backslashes for more information). | ||
NOTE: As of v1.2.0, anymatch uses [micromatch](https://github.com/jonschlinkert/micromatch) | ||
- **v3.0:**: Removed `startIndex` and `endIndex` arguments. | ||
- **v2.0:** [micromatch](https://github.com/jonschlinkert/micromatch) moves away from minimatch-parity and inline with Bash. This includes handling backslashes differently (see https://github.com/micromatch/micromatch#backslashes for more information). | ||
- **v1.2:** anymatch uses [micromatch](https://github.com/jonschlinkert/micromatch) | ||
for glob pattern matching. Issues with glob pattern matching should be | ||
@@ -102,0 +87,0 @@ reported directly to the [micromatch issue tracker](https://github.com/jonschlinkert/micromatch/issues). |
New author
Supply chain riskA new npm collaborator published a version of the package for the first time. New collaborators are usually benign additions to a project, but do indicate a change to the security surface area of a package.
Found 1 instance in 1 package
8837
2
5
97
85
1
+ Addedpicomatch@^2.0.3
+ Addednormalize-path@3.0.0(transitive)
+ Addedpicomatch@2.3.1(transitive)
- Removedmicromatch@^3.1.4
- Removedarr-diff@4.0.0(transitive)
- Removedarr-flatten@1.1.0(transitive)
- Removedarr-union@3.1.0(transitive)
- Removedarray-unique@0.3.2(transitive)
- Removedassign-symbols@1.0.0(transitive)
- Removedatob@2.1.2(transitive)
- Removedbase@0.11.2(transitive)
- Removedbraces@2.3.2(transitive)
- Removedcache-base@1.0.1(transitive)
- Removedclass-utils@0.3.6(transitive)
- Removedcollection-visit@1.0.0(transitive)
- Removedcomponent-emitter@1.3.1(transitive)
- Removedcopy-descriptor@0.1.1(transitive)
- Removeddebug@2.6.9(transitive)
- Removeddecode-uri-component@0.2.2(transitive)
- Removeddefine-property@0.2.51.0.02.0.2(transitive)
- Removedexpand-brackets@2.1.4(transitive)
- Removedextend-shallow@2.0.13.0.2(transitive)
- Removedextglob@2.0.4(transitive)
- Removedfill-range@4.0.0(transitive)
- Removedfor-in@1.0.2(transitive)
- Removedfragment-cache@0.2.1(transitive)
- Removedfunction-bind@1.1.2(transitive)
- Removedget-value@2.0.6(transitive)
- Removedhas-value@0.3.11.0.0(transitive)
- Removedhas-values@0.1.41.0.0(transitive)
- Removedhasown@2.0.2(transitive)
- Removedis-accessor-descriptor@1.0.1(transitive)
- Removedis-buffer@1.1.6(transitive)
- Removedis-data-descriptor@1.0.1(transitive)
- Removedis-descriptor@0.1.71.0.3(transitive)
- Removedis-extendable@0.1.11.0.1(transitive)
- Removedis-number@3.0.0(transitive)
- Removedis-plain-object@2.0.4(transitive)
- Removedis-windows@1.0.2(transitive)
- Removedisarray@1.0.0(transitive)
- Removedisobject@2.1.03.0.1(transitive)
- Removedkind-of@3.2.24.0.06.0.3(transitive)
- Removedmap-cache@0.2.2(transitive)
- Removedmap-visit@1.0.0(transitive)
- Removedmicromatch@3.1.10(transitive)
- Removedmixin-deep@1.3.2(transitive)
- Removedms@2.0.0(transitive)
- Removednanomatch@1.2.13(transitive)
- Removednormalize-path@2.1.1(transitive)
- Removedobject-copy@0.1.0(transitive)
- Removedobject-visit@1.0.1(transitive)
- Removedobject.pick@1.3.0(transitive)
- Removedpascalcase@0.1.1(transitive)
- Removedposix-character-classes@0.1.1(transitive)
- Removedregex-not@1.0.2(transitive)
- Removedremove-trailing-separator@1.1.0(transitive)
- Removedrepeat-element@1.1.4(transitive)
- Removedrepeat-string@1.6.1(transitive)
- Removedresolve-url@0.2.1(transitive)
- Removedret@0.1.15(transitive)
- Removedsafe-regex@1.1.0(transitive)
- Removedset-value@2.0.1(transitive)
- Removedsnapdragon@0.8.2(transitive)
- Removedsnapdragon-node@2.1.1(transitive)
- Removedsnapdragon-util@3.0.1(transitive)
- Removedsource-map@0.5.7(transitive)
- Removedsource-map-resolve@0.5.3(transitive)
- Removedsource-map-url@0.4.1(transitive)
- Removedsplit-string@3.1.0(transitive)
- Removedstatic-extend@0.1.2(transitive)
- Removedto-object-path@0.3.0(transitive)
- Removedto-regex@3.0.2(transitive)
- Removedto-regex-range@2.1.1(transitive)
- Removedunion-value@1.0.1(transitive)
- Removedunset-value@1.0.0(transitive)
- Removedurix@0.1.0(transitive)
- Removeduse@3.1.1(transitive)
Updatednormalize-path@^3.0.0