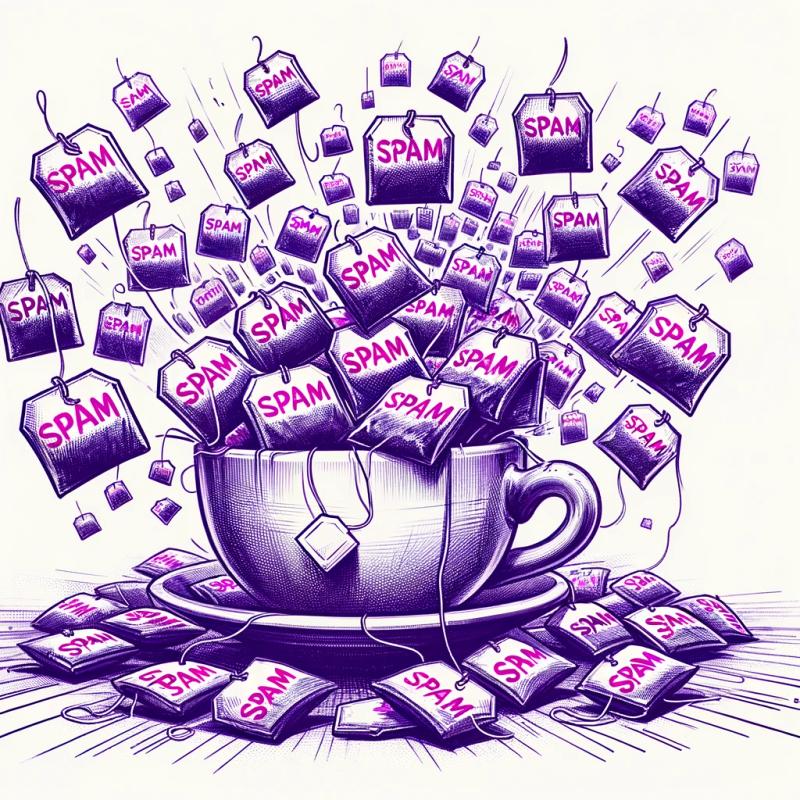
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Readme
Anzip is a library to unzip file archive for Node using only one async function.
yarn add anzip
Or using npm
npm add anzip
Now that ESM support is widely common,
require
should be forgotten.
import anzip from 'anzip';
Extract file.zip to current path
await anzip('file.zip');
Extract file.zip to the current path and get output
const output = await anzip('file.zip');
console.log('duration=', output.duration);
console.log('number of files=', output.files.length);
Extract only README.md from file.zip to current path
const output = await anzip('file.zip', { pattern: 'README.md', });
console.log('duration=', output.duration);
console.log('number of files=', output.files.length); // Should be one
Extract only README.md from file.zip to output content variable
const output = await anzip('file.zip', { pattern: 'README.md', outputContent: true });
console.log('duration=', output.duration);
console.log('content=', output.files[0].content);
Extract only README.md from file.zip to output content variable and currentpath
const output = await anzip('file.zip', { pattern: 'README.md', outputPath: './', outputContent: true });
console.log('duration=', output.duration);
console.log('content=', output.files[0].content);
Extract with an entryHandler to fliter entry
const outputPath = './path';
const entryHandler = async entry => {
let resp = true;
const fn = entry.name;
if (fn.endsWith('dummy.pdf')) {
try {
await entry.saveTo(outputPath);
resp = false;
} catch (e) {
//
}
}
return resp;
};
const output = await anzip('file.zip',
outputPath,
{ pattern: /(^(?!\.))(.+(.png|.jpeg|.jpg|.svg|.pdf|.json))$/i,, outputPath: './', entryHandler, outputContent: true });
console.log('duration=', output.duration);
// ./path/dummy.pdf should be saved
Extract using 2 rules and an entryHandler in one to fliter entry
const outputPath = './path';
const entryHandler = async entry => {
let resp = true;
const fn = entry.name;
if (fn.endsWith('dummy.pdf')) {
try {
await entry.saveTo(outputPath);
resp = false;
} catch (e) {
//
}
}
return resp;
};
const output = await anzip('file.zip',
outputPath,
pattern: /(^(?!\.))(.+(.png|.jpeg|.jpg|.svg|.pdf|.json))$/i,
flattenPath: true,
disableSave: true,
disableOutput: true,
rules: [
{ pattern: /(^(?!\.))(test.json)$/i, outputContent: true },
{ pattern: /(^(?!\.))(.+(.png|.jpeg|.jpg|.svg|.pdf))$/i, entryHandler },
],
});
console.log('duration=', output.duration);
// ./path/dummy.pdf should be saved
One function to rule them all.
output = await anzip(filename, {opts})
Function properties
parameters | type | description |
---|---|---|
filename | mandatory string | containing zip path to + file |
opts | optional object | containing optional parameters |
opts.outputPath | optional string | the directory where to to save extracted files |
opts.outputContent | optional boolean | if set to true, return file.content a Buffer containing file's content |
opts.disableSave | optional boolean | if set to true, don't save files |
opts.pattern | optional regex | if set only extract/proceed matching filenames |
opts.flattenPath | optional boolean | if set don't recreate zip's directories, all file are saved in outputPath |
opts.disableOutput | optional boolean | if set don't write files to output |
opts.entryHandler | optional promise | use it to add some extra processing to an entry, return true if stream is consumed otherwise false |
opts.rules | optional array | use it to add some fine tuned control how to handle each files |
opts.rules.pattern | mandatory regex | if it match entry will use rule's parameters instead of global's one |
Returned output is an object containing:
parameters | type | description |
---|---|---|
duration | number | how long it took to extract in seconds |
files | array | all files extracted or handled, otherwise empty |
files[x].name | string | the filename |
files[x].directory | string | the directory in archive (even if opts.flattenPath=true) |
files[x].saved | boolean | true if the file was saved to outputPath |
files[x].content | Buffer | the content of the file available if opts.outputContent=true or rule.outputContent=true |
files[x].error | Error | if an error occured |
Read Contributing Guide for development setup instructions.
FAQs
Node Async Unzip Lib
The npm package anzip receives a total of 256 weekly downloads. As such, anzip popularity was classified as not popular.
We found that anzip demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.