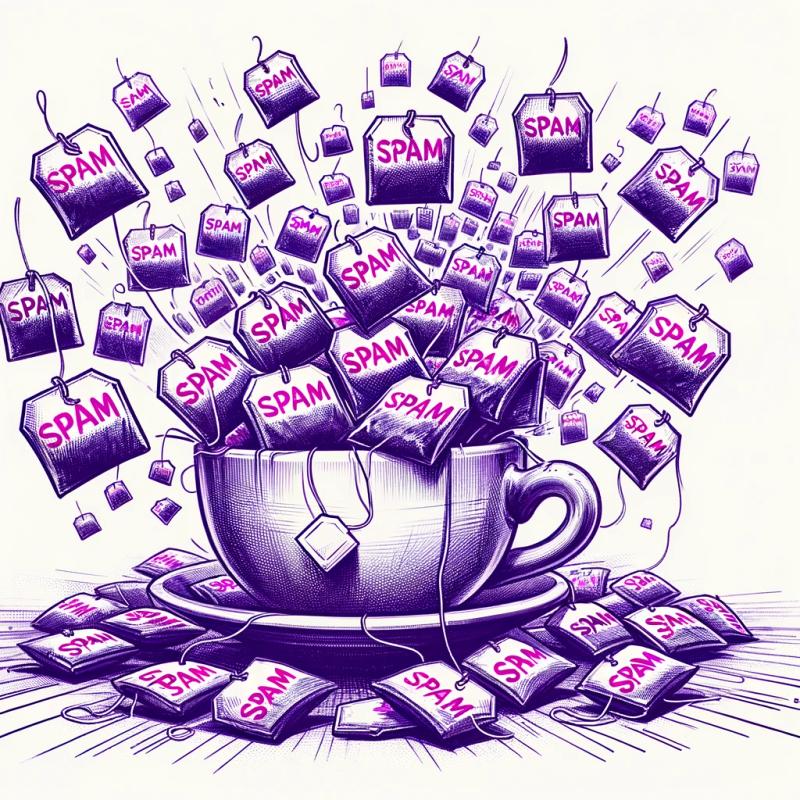
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
api-tools-ts
Advanced tools
Readme
A light-weight library to help you create APIs on the fly using express.js written in TypeScript.
Creating RESTful APIs is not always the easiest, especially when you have a lot of endpoints and functions for each of this endpoints. API-Tools-TS (the name will be changed LOL) provides an easy way to deal with express.js for beginners in more structured way.
npm i api-tools-ts
import { APIController } from "api-tools-ts";
const api = new APIController(endpoint); // the main endpoint for the api you wish to create
const { APIController } = require("api-tools-ts");
const api = new APIController(endpoint); // the main endpoint for the api you wish to create
import { APIController } from "api-tools-ts";
const api = new APIController("/api/v1");
api.AddEndPoint("/", "get", (req, res) => {
res.status(200).json({ home: "home is now accessible" });
});
api.AddEndPoint("/:random", "get", (req, res) => {
const random = req.params.random;
res.status(200).json({ test: Math.round(Math.random() * Number(random)) });
});
api.startServer({useDefaultMiddlewares: 'true'}); // to apply default middlewares ["cors", "morgan", "helmet"]
import { APIController } from "api-tools-ts";
const api = new APIController("/api/v1");
const postUser = (req, res) => {
res.status(200).json({ status: "ok", message: "User created successfully." });
}
const getUsers = (req, res) => {
res.status(200).json({ status: "ok", message: "Here are all users" });
}
const deleteUser = (req, res) => {
res.status(200).json({ status: "ok", message: "User deleted successfully" });
}
api.AddMultipleMethods("/users", ["get", "post", "delete"], [getUsers, postUser, deleteUser] );
api.startServer({useDefaultMiddlewares: 'true'});
api.AddEndPoint("/user", "get", (req, res) => {
res.status(200).json({ status: "ok", message: "Here are all users"});
})
api.AddMiddleWare("whatever", (req, res, next) => {
console.log("I am activated");
next();
})
api.startServer({useDefaultMiddlewares: 'true'});
api.port = <your port>
api.startServer({useDefaultMiddlewares: 'true'});
API-Tools-TS uses by default the following middlewares for better security and stability:
If you do not wish to create your api with these milddlewares, set useDefaultMiddlewares
to false when calling startServer.
api.startServer({useDefaultMiddlewares: 'false'});
FAQs
A light-weight library to help you create APIs on the fly using express.js written in TypeScript
The npm package api-tools-ts receives a total of 0 weekly downloads. As such, api-tools-ts popularity was classified as not popular.
We found that api-tools-ts demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.