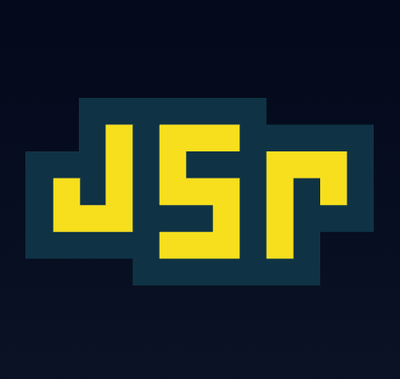
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
apollo-upload-client
Advanced tools
A terminating Apollo Link for Apollo Client that fetches a GraphQL multipart request if the GraphQL variables contain files (by default FileList, File, or Blob instances), or else fetches a regular GraphQL POST or GET request (depending on the config and
The apollo-upload-client package is a client for Apollo GraphQL that enables file uploads via GraphQL mutations. It extends Apollo Client to support file uploads using the GraphQL multipart request specification.
File Upload
This feature allows you to upload files via GraphQL mutations. The code sample demonstrates how to set up an Apollo Client with the upload link and perform a file upload mutation.
const { ApolloClient, InMemoryCache, HttpLink } = require('@apollo/client');
const { createUploadLink } = require('apollo-upload-client');
const link = createUploadLink({ uri: 'http://localhost:4000/graphql' });
const client = new ApolloClient({
link,
cache: new InMemoryCache()
});
const UPLOAD_FILE = gql`
mutation($file: Upload!) {
uploadFile(file: $file) {
url
}
}
`;
const file = new File(['foo'], 'foo.txt', {
type: 'text/plain',
});
client.mutate({
mutation: UPLOAD_FILE,
variables: { file }
}).then(response => {
console.log(response.data.uploadFile.url);
});
This package allows you to call REST endpoints from Apollo Client. While it does not specifically handle file uploads, it can be used to integrate RESTful file upload endpoints with Apollo Client.
A minimal GraphQL client that supports file uploads via the multipart request specification. It is more lightweight compared to apollo-upload-client and can be used in environments where a full Apollo Client setup is not required.
A terminating Apollo Link for Apollo Client that fetches a GraphQL multipart request if the GraphQL variables contain files (by default FileList
, File
, or Blob
instances), or else fetches a regular GraphQL POST or GET request (depending on the config and GraphQL operation).
To install with npm, run:
npm install apollo-upload-client
Polyfill any required globals (see Requirements) that are missing in your server and client environments.
Remove any uri
, credentials
, or headers
options from the ApolloClient
constructor.
Apollo Client can only have 1 terminating Apollo Link that sends the GraphQL requests; if one such as HttpLink
is already setup, remove it.
Initialize the client with a terminating Apollo Link using the function createUploadLink
.
Also ensure the GraphQL server implements the GraphQL multipart request spec and that uploads are handled correctly in resolvers.
Use FileList
, File
, or Blob
instances anywhere within query or mutation variables to send a GraphQL multipart request.
See also the example API and client.
FileList
import { gql, useMutation } from "@apollo/client";
const MUTATION = gql`
mutation ($files: [Upload!]!) {
uploadFiles(files: $files) {
success
}
}
`;
function UploadFiles() {
const [mutate] = useMutation(MUTATION);
return (
<input
type="file"
multiple
required
onChange={({ target: { validity, files } }) => {
if (validity.valid)
mutate({
variables: {
files,
},
});
}}
/>
);
}
File
import { gql, useMutation } from "@apollo/client";
const MUTATION = gql`
mutation ($file: Upload!) {
uploadFile(file: $file) {
success
}
}
`;
function UploadFile() {
const [mutate] = useMutation(MUTATION);
return (
<input
type="file"
required
onChange={({
target: {
validity,
files: [file],
},
}) => {
if (validity.valid)
mutate({
variables: {
file,
},
});
}}
/>
);
}
Blob
import { gql, useMutation } from "@apollo/client";
const MUTATION = gql`
mutation ($file: Upload!) {
uploadFile(file: $file) {
success
}
}
`;
function UploadFile() {
const [mutate] = useMutation(MUTATION);
return (
<input
type="text"
required
onChange={({ target: { validity, value } }) => {
if (validity.valid) {
const file = new Blob([value], { type: "text/plain" });
// Optional, defaults to `blob`.
file.name = "text.txt";
mutate({
variables: {
file,
},
});
}
}}
/>
);
}
^18.15.0 || >=20.4.0
.> 0.5%, not OperaMini all, not dead
.Consider polyfilling:
Projects must configure TypeScript to use types from the ECMAScript modules that have a // @ts-check
comment:
compilerOptions.allowJs
should be true
.compilerOptions.maxNodeModuleJsDepth
should be reasonably large, e.g. 10
.compilerOptions.module
should be "node16"
or "nodenext"
.The npm package apollo-upload-client
features optimal JavaScript module design. It doesn’t have a main index module, so use deep imports from the ECMAScript modules that are exported via the package.json
field exports
:
FAQs
A terminating Apollo Link for Apollo Client that fetches a GraphQL multipart request if the GraphQL variables contain files (by default FileList, File, or Blob instances), or else fetches a regular GraphQL POST or GET request (depending on the config and
We found that apollo-upload-client demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.