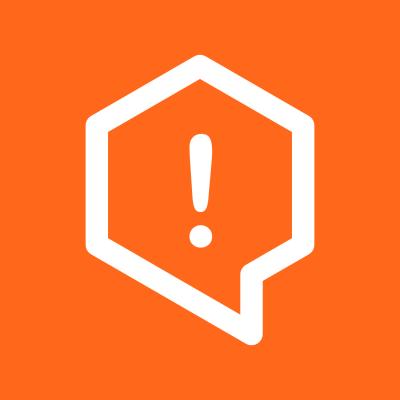
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
apollo3-cache-persist
Advanced tools
The apollo3-cache-persist package is used to persist and rehydrate the Apollo Cache in JavaScript applications. This is particularly useful for offline support and improving the performance of your application by caching GraphQL query results.
Persist Cache
This feature allows you to persist the Apollo Cache to a storage medium, such as localStorage. This ensures that the cache is saved and can be rehydrated when the application is reloaded.
const { persistCache } = require('apollo3-cache-persist');
const { InMemoryCache } = require('@apollo/client');
const cache = new InMemoryCache();
persistCache({
cache,
storage: window.localStorage,
});
Rehydrate Cache
This feature allows you to rehydrate the Apollo Cache from a storage medium. This is useful for restoring the cache state when the application is reloaded, providing a seamless user experience.
const { persistCache } = require('apollo3-cache-persist');
const { InMemoryCache } = require('@apollo/client');
const cache = new InMemoryCache();
persistCache({
cache,
storage: window.localStorage,
}).then(() => {
// Now the cache is rehydrated and ready to use
});
Custom Storage
This feature allows you to use a custom storage solution for persisting the Apollo Cache. You can define your own getItem, setItem, and removeItem methods to integrate with any storage system.
const { persistCache } = require('apollo3-cache-persist');
const { InMemoryCache } = require('@apollo/client');
const cache = new InMemoryCache();
const customStorage = {
getItem: async (key) => { /* custom get item logic */ },
setItem: async (key, value) => { /* custom set item logic */ },
removeItem: async (key) => { /* custom remove item logic */ },
};
persistCache({
cache,
storage: customStorage,
});
redux-persist is a library used to persist and rehydrate a Redux store. It provides similar functionality to apollo3-cache-persist but is designed for use with Redux rather than Apollo Client. It supports various storage backends and offers more configuration options for handling persisted state.
localforage is a library for offline storage that supports multiple storage drivers like IndexedDB, WebSQL, and localStorage. While it is not specifically designed for persisting Apollo Cache, it can be used as a storage backend for apollo3-cache-persist or other caching solutions.
idb-keyval is a small library for managing key-value pairs in IndexedDB. It provides a simple API for storing and retrieving data, making it a good choice for custom storage solutions in apollo3-cache-persist. It is lightweight and easy to use, but lacks some of the advanced features of more comprehensive libraries.
Simple persistence for all Apollo Client 3.0 cache implementations, including
InMemoryCache
and Hermes
.
Supports web and React Native. See all storage providers.
npm install --save apollo3-cache-persist
or
yarn add apollo3-cache-persist
To get started, simply pass your Apollo cache and an
underlying storage provider to persistCache
.
By default, the contents of your Apollo cache will be immediately restored (asynchronously, see how to persist data before rendering), and will be persisted upon every write to the cache (with a short debounce interval).
import AsyncStorage from '@react-native-async-storage/async-storage';
import { InMemoryCache } from '@apollo/client/core';
import { persistCache, AsyncStorageWrapper } from 'apollo3-cache-persist';
const cache = new InMemoryCache({...});
// await before instantiating ApolloClient, else queries might run before the cache is persisted
await persistCache({
cache,
storage: new AsyncStorageWrapper(AsyncStorage),
});
// Continue setting up Apollo as usual.
const client = new ApolloClient({
cache,
...
});
import { InMemoryCache } from '@apollo/client/core';
import { persistCache, LocalStorageWrapper } from 'apollo3-cache-persist';
const cache = new InMemoryCache({...});
// await before instantiating ApolloClient, else queries might run before the cache is persisted
await persistCache({
cache,
storage: new LocalStorageWrapper(window.localStorage),
});
// Continue setting up Apollo as usual.
const client = new ApolloClient({
cache,
...
});
See a complete example in the web example.
Want to make the project better? Awesome! Please read through our Contributing Guidelines.
We all do this for free... so please be nice 😁.
FAQs
Simple persistence for all Apollo cache implementations
The npm package apollo3-cache-persist receives a total of 179,077 weekly downloads. As such, apollo3-cache-persist popularity was classified as popular.
We found that apollo3-cache-persist demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.