appbase-js
Advanced tools
Comparing version 4.2.0 to 4.2.1
@@ -8,3 +8,2 @@ 'use strict'; | ||
var fetch = _interopDefault(require('cross-fetch')); | ||
var stringify = _interopDefault(require('json-stable-stringify')); | ||
@@ -45,11 +44,3 @@ var _typeof = typeof Symbol === "function" && typeof Symbol.iterator === "symbol" ? function (obj) { | ||
} | ||
function uuidv4() { | ||
return 'xxxxxxxx-xxxx-4xxx-yxxx-xxxxxxxxxxxx'.replace(/[xy]/g, function (c) { | ||
var r = Math.random() * 16 | 0; // eslint-disable-line no-bitwise | ||
var v = c === 'x' ? r : r & 0x3 | 0x8; // eslint-disable-line no-bitwise | ||
return v.toString(16); | ||
}); | ||
} | ||
function validateRSQuery(query) { | ||
@@ -112,19 +103,2 @@ if (query && Object.prototype.toString.call(query) === '[object Array]') { | ||
/** | ||
* Send only when a connection is opened | ||
* @param {Object} socket | ||
* @param {Function} callback | ||
*/ | ||
function waitForSocketConnection(socket, callback) { | ||
setTimeout(function () { | ||
if (socket.readyState === 1) { | ||
if (callback != null) { | ||
callback(); | ||
} | ||
} else { | ||
waitForSocketConnection(socket, callback); | ||
} | ||
}, 5); // wait 5 ms for the connection... | ||
} | ||
function encodeHeaders() { | ||
@@ -154,2 +128,3 @@ var headers = arguments.length > 0 && arguments[0] !== undefined ? arguments[0] : {}; | ||
* @param {String} config.password | ||
* @param {Boolean} config.enableTelemetry | ||
* A callback function which will be invoked before a fetch request made | ||
@@ -202,3 +177,10 @@ */ | ||
this.credentials = credentials; | ||
this.headers = {}; | ||
this.headers = { | ||
'X-Search-Client': 'Appbase JS' | ||
}; | ||
if (config.enableTelemetry === false) { | ||
Object.assign(this.headers, { | ||
'X-Enable-Telemetry': config.enableTelemetry | ||
}); | ||
} | ||
} | ||
@@ -345,155 +327,3 @@ | ||
var WebSocket = typeof window !== 'undefined' ? window.WebSocket : require('ws'); | ||
/** | ||
* To connect a web socket | ||
* @param {Object} args | ||
* @param {String} args.method | ||
* @param {String} args.path | ||
* @param {Object} args.params | ||
* @param {Object} args.body | ||
*/ | ||
function wsRequest(args, onData, onError, onClose) { | ||
var _this = this; | ||
try { | ||
var parsedArgs = removeUndefined(args); | ||
var method = parsedArgs.method, | ||
path = parsedArgs.path, | ||
params = parsedArgs.params; | ||
var bodyCopy = args.body; | ||
if (!bodyCopy || (typeof bodyCopy === 'undefined' ? 'undefined' : _typeof(bodyCopy)) !== 'object') { | ||
bodyCopy = {}; | ||
} | ||
var init = function init() { | ||
_this.ws = new WebSocket('wss://' + _this.url + '/' + _this.app); | ||
_this.id = uuidv4(); | ||
_this.request = { | ||
id: _this.id, | ||
path: _this.app + '/' + path + '?' + querystring.stringify(params), | ||
method: method, | ||
body: bodyCopy | ||
}; | ||
if (_this.credentials) { | ||
_this.request.authorization = 'Basic ' + btoa(_this.credentials); | ||
} | ||
_this.result = {}; | ||
_this.closeHandler = function () { | ||
_this.wsClosed(); | ||
}; | ||
_this.errorHandler = function (err) { | ||
_this.processError.apply(_this, [err]); | ||
}; | ||
_this.messageHandler = function (message) { | ||
var dataObj = JSON.parse(message.data); | ||
if (dataObj.body && dataObj.body.status >= 400) { | ||
_this.processError.apply(_this, [dataObj]); | ||
} else { | ||
_this.processMessage.apply(_this, [dataObj]); | ||
} | ||
}; | ||
_this.send = function (request) { | ||
waitForSocketConnection(_this.ws, function () { | ||
try { | ||
_this.ws.send(JSON.stringify(request)); | ||
} catch (e) { | ||
console.warn(e); | ||
} | ||
}); | ||
}; | ||
_this.ws.onmessage = _this.messageHandler; | ||
_this.ws.onerror = _this.errorHandler; | ||
_this.ws.onclose = _this.closeHandler; | ||
_this.send(_this.request); | ||
_this.result.stop = _this.stop; | ||
_this.result.reconnect = _this.reconnect; | ||
return _this.result; | ||
}; | ||
this.wsClosed = function () { | ||
if (onClose) { | ||
onClose(); | ||
} | ||
}; | ||
this.stop = function () { | ||
_this.ws.onmessage = undefined; | ||
_this.ws.onclose = undefined; | ||
_this.ws.onerror = undefined; | ||
_this.wsClosed(); | ||
var unsubRequest = JSON.parse(JSON.stringify(_this.request)); | ||
unsubRequest.unsubscribe = true; | ||
if (_this.unsubscribed !== true) { | ||
_this.send(unsubRequest); | ||
} | ||
_this.unsubscribed = true; | ||
}; | ||
this.reconnect = function () { | ||
_this.stop(); | ||
return wsRequest(args, onData, onError, onClose); | ||
}; | ||
this.processError = function (err) { | ||
if (onError) { | ||
onError(err); | ||
} else { | ||
console.warn(err); | ||
} | ||
}; | ||
this.processMessage = function (origDataObj) { | ||
var dataObj = JSON.parse(JSON.stringify(origDataObj)); | ||
if (!dataObj.id && dataObj.message) { | ||
if (onError) { | ||
onError(dataObj); | ||
} | ||
return; | ||
} | ||
if (dataObj.id === _this.id) { | ||
if (dataObj.message) { | ||
delete dataObj.id; | ||
if (onError) { | ||
onError(dataObj); | ||
} | ||
return; | ||
} | ||
if (dataObj.query_id) { | ||
_this.query_id = dataObj.query_id; | ||
} | ||
if (dataObj.channel) { | ||
_this.channel = dataObj.channel; | ||
} | ||
if (dataObj.body && dataObj.body !== '') { | ||
if (onData) { | ||
onData(dataObj.body); | ||
} | ||
} | ||
return; | ||
} | ||
if (!dataObj.id && dataObj.channel && dataObj.channel === _this.channel) { | ||
if (onData) { | ||
onData(dataObj.event); | ||
} | ||
} | ||
}; | ||
return init(); | ||
} catch (e) { | ||
if (onError) { | ||
onError(e); | ||
} else { | ||
console.warn(e); | ||
} | ||
return null; | ||
} | ||
} | ||
/** | ||
* Index Service | ||
@@ -800,241 +630,2 @@ * @param {Object} args | ||
/** | ||
* Stream Service | ||
* @param {Object} args | ||
* @param {String} args.type | ||
* @param {Boolean} args.stream | ||
* @param {String} args.id | ||
* @param {Function} onData | ||
* @param {Function} onError | ||
* @param {Function} onClose | ||
*/ | ||
function getStream(args) { | ||
var parsedArgs = removeUndefined(args); | ||
// Validate arguments | ||
var valid = validate(parsedArgs, { | ||
type: 'string', | ||
id: 'string|number' | ||
}); | ||
if (valid !== true) { | ||
throw valid; | ||
} | ||
var type = parsedArgs.type, | ||
id = parsedArgs.id; | ||
delete parsedArgs.type; | ||
delete parsedArgs.id; | ||
delete parsedArgs.stream; | ||
if (parsedArgs.stream === true) { | ||
parsedArgs.stream = 'true'; | ||
} else { | ||
delete parsedArgs.stream; | ||
parsedArgs.streamonly = 'true'; | ||
} | ||
for (var _len = arguments.length, rest = Array(_len > 1 ? _len - 1 : 0), _key = 1; _key < _len; _key++) { | ||
rest[_key - 1] = arguments[_key]; | ||
} | ||
return this.performWsRequest.apply(this, [{ | ||
method: 'GET', | ||
path: type + '/' + encodeURIComponent(id), | ||
params: parsedArgs | ||
}].concat(rest)); | ||
} | ||
/** | ||
* Search Stream | ||
* @param {Object} args | ||
* @param {String} args.type | ||
* @param {Object} args.body | ||
* @param {Boolean} args.stream | ||
* @param {Function} onData | ||
* @param {Function} onError | ||
* @param {Function} onClose | ||
*/ | ||
function searchStreamApi(args) { | ||
var parsedArgs = removeUndefined(args); | ||
// Validate arguments | ||
var valid = validate(parsedArgs, { | ||
body: 'object' | ||
}); | ||
if (valid !== true) { | ||
throw valid; | ||
} | ||
if (parsedArgs.type === undefined || Array.isArray(parsedArgs.type) && parsedArgs.type.length === 0) { | ||
throw new Error('Missing fields: type'); | ||
} | ||
var type = void 0; | ||
if (Array.isArray(parsedArgs.type)) { | ||
type = parsedArgs.type.join(); | ||
} else { | ||
type = parsedArgs.type; | ||
} | ||
var body = parsedArgs.body; | ||
delete parsedArgs.type; | ||
delete parsedArgs.body; | ||
delete parsedArgs.stream; | ||
parsedArgs.streamonly = 'true'; | ||
for (var _len = arguments.length, rest = Array(_len > 1 ? _len - 1 : 0), _key = 1; _key < _len; _key++) { | ||
rest[_key - 1] = arguments[_key]; | ||
} | ||
return this.performWsRequest.apply(this, [{ | ||
method: 'POST', | ||
path: type + '/_search', | ||
params: parsedArgs, | ||
body: body | ||
}].concat(rest)); | ||
} | ||
/** | ||
* Webhook Service | ||
* @param {Object} args | ||
* @param {String} args.type | ||
* @param {Object} args.body | ||
* @param {Object} webhook | ||
* @param {Function} onData | ||
* @param {Function} onError | ||
* @param {Function} onClose | ||
*/ | ||
function searchStreamToURLApi(args, webhook) { | ||
for (var _len = arguments.length, rest = Array(_len > 2 ? _len - 2 : 0), _key = 2; _key < _len; _key++) { | ||
rest[_key - 2] = arguments[_key]; | ||
} | ||
var _this = this; | ||
var parsedArgs = removeUndefined(args); | ||
var bodyCopy = parsedArgs.body; | ||
var type = void 0; | ||
var typeString = void 0; | ||
// Validate arguments | ||
var valid = validate(parsedArgs, { | ||
body: 'object' | ||
}); | ||
if (valid !== true) { | ||
throw valid; | ||
} | ||
if (parsedArgs.type === undefined || !(typeof parsedArgs.type === 'string' || Array.isArray(parsedArgs.type)) || parsedArgs.type === '' || parsedArgs.type.length === 0) { | ||
throw new Error('fields missing: type'); | ||
} | ||
valid = validate(parsedArgs.body, { | ||
query: 'object' | ||
}); | ||
if (valid !== true) { | ||
throw valid; | ||
} | ||
if (Array.isArray(parsedArgs.type)) { | ||
type = parsedArgs.type; | ||
typeString = parsedArgs.type.join(); | ||
} else { | ||
type = [parsedArgs.type]; | ||
typeString = parsedArgs.type; | ||
} | ||
var webhooks = []; | ||
var _bodyCopy = bodyCopy, | ||
query = _bodyCopy.query; | ||
if (typeof webhook === 'string') { | ||
var webHookObj = {}; | ||
webHookObj.url = webhook; | ||
webHookObj.method = 'GET'; | ||
webhooks.push(webHookObj); | ||
} else if (webhook.constructor === Array) { | ||
webhooks = webhook; | ||
} else if (webhook === Object(webhook)) { | ||
webhooks.push(webhook); | ||
} else { | ||
throw new Error('fields missing: second argument(webhook) is necessary'); | ||
} | ||
var populateBody = function populateBody() { | ||
bodyCopy = {}; | ||
bodyCopy.webhooks = webhooks; | ||
bodyCopy.query = query; | ||
bodyCopy.type = type; | ||
}; | ||
populateBody(); | ||
var encode64 = btoa(stringify(query)); | ||
var path = '.percolator/webhooks-0-' + typeString + '-0-' + encode64; | ||
this.change = function () { | ||
webhooks = []; | ||
if (typeof parsedArgs === 'string') { | ||
var webhook2 = {}; | ||
webhook2.url = parsedArgs; | ||
webhook2.method = 'POST'; | ||
webhooks.push(webhook2); | ||
} else if (parsedArgs.constructor === Array) { | ||
webhooks = parsedArgs; | ||
} else if (parsedArgs === Object(parsedArgs)) { | ||
webhooks.push(parsedArgs); | ||
} else { | ||
throw new Error('fields missing: one of webhook or url fields is required'); | ||
} | ||
populateBody(); | ||
return _this.performRequest('POST'); | ||
}; | ||
this.stop = function () { | ||
bodyCopy = undefined; | ||
return _this.performRequest('DELETE'); | ||
}; | ||
this.performRequest = function (method) { | ||
var res = _this.performWsRequest.apply(_this, [{ | ||
method: method, | ||
path: path, | ||
body: bodyCopy | ||
}].concat(rest)); | ||
res.change = _this.change; | ||
res.stop = _this.stop; | ||
return res; | ||
}; | ||
return this.performRequest('POST'); | ||
} | ||
/** | ||
* To get types | ||
*/ | ||
function getTypesService() { | ||
var _this = this; | ||
return new Promise(function (resolve, reject) { | ||
try { | ||
return _this.performFetchRequest({ | ||
method: 'GET', | ||
path: '_mapping' | ||
}).then(function (data) { | ||
var types = Object.keys(data[_this.app].mappings).filter(function (type) { | ||
return type !== '_default_'; | ||
}); | ||
return resolve(types); | ||
}); | ||
} catch (e) { | ||
return reject(e); | ||
} | ||
}); | ||
} | ||
/** | ||
* To get mappings | ||
@@ -1082,55 +673,45 @@ */ | ||
function index (config) { | ||
var client = new AppBase(config); | ||
function appbasejs(config) { | ||
var client = new AppBase(config); | ||
AppBase.prototype.performFetchRequest = fetchRequest; | ||
AppBase.prototype.performFetchRequest = fetchRequest; | ||
AppBase.prototype.performWsRequest = wsRequest; | ||
AppBase.prototype.index = indexApi; | ||
AppBase.prototype.index = indexApi; | ||
AppBase.prototype.get = getApi; | ||
AppBase.prototype.get = getApi; | ||
AppBase.prototype.update = updateApi; | ||
AppBase.prototype.update = updateApi; | ||
AppBase.prototype.delete = deleteApi; | ||
AppBase.prototype.delete = deleteApi; | ||
AppBase.prototype.bulk = bulkApi; | ||
AppBase.prototype.bulk = bulkApi; | ||
AppBase.prototype.search = searchApi; | ||
AppBase.prototype.search = searchApi; | ||
AppBase.prototype.msearch = msearchApi; | ||
AppBase.prototype.msearch = msearchApi; | ||
AppBase.prototype.reactiveSearchv3 = reactiveSearchv3Api; | ||
AppBase.prototype.reactiveSearchv3 = reactiveSearchv3Api; | ||
AppBase.prototype.getQuerySuggestions = getSuggestionsv3Api; | ||
AppBase.prototype.getQuerySuggestions = getSuggestionsv3Api; | ||
AppBase.prototype.getMappings = getMappings; | ||
AppBase.prototype.getStream = getStream; | ||
AppBase.prototype.setHeaders = function setHeaders() { | ||
var headers = arguments.length > 0 && arguments[0] !== undefined ? arguments[0] : {}; | ||
var shouldEncode = arguments.length > 1 && arguments[1] !== undefined ? arguments[1] : false; | ||
AppBase.prototype.searchStream = searchStreamApi; | ||
// Encode headers | ||
if (shouldEncode) { | ||
this.headers = encodeHeaders(headers); | ||
} else { | ||
this.headers = headers; | ||
} | ||
}; | ||
AppBase.prototype.searchStreamToURL = searchStreamToURLApi; | ||
AppBase.prototype.getTypes = getTypesService; | ||
AppBase.prototype.getMappings = getMappings; | ||
AppBase.prototype.setHeaders = function setHeaders() { | ||
var headers = arguments.length > 0 && arguments[0] !== undefined ? arguments[0] : {}; | ||
var shouldEncode = arguments.length > 1 && arguments[1] !== undefined ? arguments[1] : false; | ||
// Encode headers | ||
if (shouldEncode) { | ||
this.headers = encodeHeaders(headers); | ||
} else { | ||
this.headers = headers; | ||
} | ||
}; | ||
if (typeof window !== 'undefined') { | ||
window.Appbase = client; | ||
} | ||
return client; | ||
if (typeof window !== 'undefined') { | ||
window.Appbase = client; | ||
} | ||
return client; | ||
} | ||
module.exports = index; | ||
module.exports = appbasejs; |
import URL from 'url-parser-lite'; | ||
import querystring from 'querystring'; | ||
import fetch from 'cross-fetch'; | ||
import stringify from 'json-stable-stringify'; | ||
@@ -40,11 +39,3 @@ var _typeof = typeof Symbol === "function" && typeof Symbol.iterator === "symbol" ? function (obj) { | ||
} | ||
function uuidv4() { | ||
return 'xxxxxxxx-xxxx-4xxx-yxxx-xxxxxxxxxxxx'.replace(/[xy]/g, function (c) { | ||
var r = Math.random() * 16 | 0; // eslint-disable-line no-bitwise | ||
var v = c === 'x' ? r : r & 0x3 | 0x8; // eslint-disable-line no-bitwise | ||
return v.toString(16); | ||
}); | ||
} | ||
function validateRSQuery(query) { | ||
@@ -107,19 +98,2 @@ if (query && Object.prototype.toString.call(query) === '[object Array]') { | ||
/** | ||
* Send only when a connection is opened | ||
* @param {Object} socket | ||
* @param {Function} callback | ||
*/ | ||
function waitForSocketConnection(socket, callback) { | ||
setTimeout(function () { | ||
if (socket.readyState === 1) { | ||
if (callback != null) { | ||
callback(); | ||
} | ||
} else { | ||
waitForSocketConnection(socket, callback); | ||
} | ||
}, 5); // wait 5 ms for the connection... | ||
} | ||
function encodeHeaders() { | ||
@@ -149,2 +123,3 @@ var headers = arguments.length > 0 && arguments[0] !== undefined ? arguments[0] : {}; | ||
* @param {String} config.password | ||
* @param {Boolean} config.enableTelemetry | ||
* A callback function which will be invoked before a fetch request made | ||
@@ -197,3 +172,10 @@ */ | ||
this.credentials = credentials; | ||
this.headers = {}; | ||
this.headers = { | ||
'X-Search-Client': 'Appbase JS' | ||
}; | ||
if (config.enableTelemetry === false) { | ||
Object.assign(this.headers, { | ||
'X-Enable-Telemetry': config.enableTelemetry | ||
}); | ||
} | ||
} | ||
@@ -340,155 +322,3 @@ | ||
var WebSocket = typeof window !== 'undefined' ? window.WebSocket : require('ws'); | ||
/** | ||
* To connect a web socket | ||
* @param {Object} args | ||
* @param {String} args.method | ||
* @param {String} args.path | ||
* @param {Object} args.params | ||
* @param {Object} args.body | ||
*/ | ||
function wsRequest(args, onData, onError, onClose) { | ||
var _this = this; | ||
try { | ||
var parsedArgs = removeUndefined(args); | ||
var method = parsedArgs.method, | ||
path = parsedArgs.path, | ||
params = parsedArgs.params; | ||
var bodyCopy = args.body; | ||
if (!bodyCopy || (typeof bodyCopy === 'undefined' ? 'undefined' : _typeof(bodyCopy)) !== 'object') { | ||
bodyCopy = {}; | ||
} | ||
var init = function init() { | ||
_this.ws = new WebSocket('wss://' + _this.url + '/' + _this.app); | ||
_this.id = uuidv4(); | ||
_this.request = { | ||
id: _this.id, | ||
path: _this.app + '/' + path + '?' + querystring.stringify(params), | ||
method: method, | ||
body: bodyCopy | ||
}; | ||
if (_this.credentials) { | ||
_this.request.authorization = 'Basic ' + btoa(_this.credentials); | ||
} | ||
_this.result = {}; | ||
_this.closeHandler = function () { | ||
_this.wsClosed(); | ||
}; | ||
_this.errorHandler = function (err) { | ||
_this.processError.apply(_this, [err]); | ||
}; | ||
_this.messageHandler = function (message) { | ||
var dataObj = JSON.parse(message.data); | ||
if (dataObj.body && dataObj.body.status >= 400) { | ||
_this.processError.apply(_this, [dataObj]); | ||
} else { | ||
_this.processMessage.apply(_this, [dataObj]); | ||
} | ||
}; | ||
_this.send = function (request) { | ||
waitForSocketConnection(_this.ws, function () { | ||
try { | ||
_this.ws.send(JSON.stringify(request)); | ||
} catch (e) { | ||
console.warn(e); | ||
} | ||
}); | ||
}; | ||
_this.ws.onmessage = _this.messageHandler; | ||
_this.ws.onerror = _this.errorHandler; | ||
_this.ws.onclose = _this.closeHandler; | ||
_this.send(_this.request); | ||
_this.result.stop = _this.stop; | ||
_this.result.reconnect = _this.reconnect; | ||
return _this.result; | ||
}; | ||
this.wsClosed = function () { | ||
if (onClose) { | ||
onClose(); | ||
} | ||
}; | ||
this.stop = function () { | ||
_this.ws.onmessage = undefined; | ||
_this.ws.onclose = undefined; | ||
_this.ws.onerror = undefined; | ||
_this.wsClosed(); | ||
var unsubRequest = JSON.parse(JSON.stringify(_this.request)); | ||
unsubRequest.unsubscribe = true; | ||
if (_this.unsubscribed !== true) { | ||
_this.send(unsubRequest); | ||
} | ||
_this.unsubscribed = true; | ||
}; | ||
this.reconnect = function () { | ||
_this.stop(); | ||
return wsRequest(args, onData, onError, onClose); | ||
}; | ||
this.processError = function (err) { | ||
if (onError) { | ||
onError(err); | ||
} else { | ||
console.warn(err); | ||
} | ||
}; | ||
this.processMessage = function (origDataObj) { | ||
var dataObj = JSON.parse(JSON.stringify(origDataObj)); | ||
if (!dataObj.id && dataObj.message) { | ||
if (onError) { | ||
onError(dataObj); | ||
} | ||
return; | ||
} | ||
if (dataObj.id === _this.id) { | ||
if (dataObj.message) { | ||
delete dataObj.id; | ||
if (onError) { | ||
onError(dataObj); | ||
} | ||
return; | ||
} | ||
if (dataObj.query_id) { | ||
_this.query_id = dataObj.query_id; | ||
} | ||
if (dataObj.channel) { | ||
_this.channel = dataObj.channel; | ||
} | ||
if (dataObj.body && dataObj.body !== '') { | ||
if (onData) { | ||
onData(dataObj.body); | ||
} | ||
} | ||
return; | ||
} | ||
if (!dataObj.id && dataObj.channel && dataObj.channel === _this.channel) { | ||
if (onData) { | ||
onData(dataObj.event); | ||
} | ||
} | ||
}; | ||
return init(); | ||
} catch (e) { | ||
if (onError) { | ||
onError(e); | ||
} else { | ||
console.warn(e); | ||
} | ||
return null; | ||
} | ||
} | ||
/** | ||
* Index Service | ||
@@ -795,241 +625,2 @@ * @param {Object} args | ||
/** | ||
* Stream Service | ||
* @param {Object} args | ||
* @param {String} args.type | ||
* @param {Boolean} args.stream | ||
* @param {String} args.id | ||
* @param {Function} onData | ||
* @param {Function} onError | ||
* @param {Function} onClose | ||
*/ | ||
function getStream(args) { | ||
var parsedArgs = removeUndefined(args); | ||
// Validate arguments | ||
var valid = validate(parsedArgs, { | ||
type: 'string', | ||
id: 'string|number' | ||
}); | ||
if (valid !== true) { | ||
throw valid; | ||
} | ||
var type = parsedArgs.type, | ||
id = parsedArgs.id; | ||
delete parsedArgs.type; | ||
delete parsedArgs.id; | ||
delete parsedArgs.stream; | ||
if (parsedArgs.stream === true) { | ||
parsedArgs.stream = 'true'; | ||
} else { | ||
delete parsedArgs.stream; | ||
parsedArgs.streamonly = 'true'; | ||
} | ||
for (var _len = arguments.length, rest = Array(_len > 1 ? _len - 1 : 0), _key = 1; _key < _len; _key++) { | ||
rest[_key - 1] = arguments[_key]; | ||
} | ||
return this.performWsRequest.apply(this, [{ | ||
method: 'GET', | ||
path: type + '/' + encodeURIComponent(id), | ||
params: parsedArgs | ||
}].concat(rest)); | ||
} | ||
/** | ||
* Search Stream | ||
* @param {Object} args | ||
* @param {String} args.type | ||
* @param {Object} args.body | ||
* @param {Boolean} args.stream | ||
* @param {Function} onData | ||
* @param {Function} onError | ||
* @param {Function} onClose | ||
*/ | ||
function searchStreamApi(args) { | ||
var parsedArgs = removeUndefined(args); | ||
// Validate arguments | ||
var valid = validate(parsedArgs, { | ||
body: 'object' | ||
}); | ||
if (valid !== true) { | ||
throw valid; | ||
} | ||
if (parsedArgs.type === undefined || Array.isArray(parsedArgs.type) && parsedArgs.type.length === 0) { | ||
throw new Error('Missing fields: type'); | ||
} | ||
var type = void 0; | ||
if (Array.isArray(parsedArgs.type)) { | ||
type = parsedArgs.type.join(); | ||
} else { | ||
type = parsedArgs.type; | ||
} | ||
var body = parsedArgs.body; | ||
delete parsedArgs.type; | ||
delete parsedArgs.body; | ||
delete parsedArgs.stream; | ||
parsedArgs.streamonly = 'true'; | ||
for (var _len = arguments.length, rest = Array(_len > 1 ? _len - 1 : 0), _key = 1; _key < _len; _key++) { | ||
rest[_key - 1] = arguments[_key]; | ||
} | ||
return this.performWsRequest.apply(this, [{ | ||
method: 'POST', | ||
path: type + '/_search', | ||
params: parsedArgs, | ||
body: body | ||
}].concat(rest)); | ||
} | ||
/** | ||
* Webhook Service | ||
* @param {Object} args | ||
* @param {String} args.type | ||
* @param {Object} args.body | ||
* @param {Object} webhook | ||
* @param {Function} onData | ||
* @param {Function} onError | ||
* @param {Function} onClose | ||
*/ | ||
function searchStreamToURLApi(args, webhook) { | ||
for (var _len = arguments.length, rest = Array(_len > 2 ? _len - 2 : 0), _key = 2; _key < _len; _key++) { | ||
rest[_key - 2] = arguments[_key]; | ||
} | ||
var _this = this; | ||
var parsedArgs = removeUndefined(args); | ||
var bodyCopy = parsedArgs.body; | ||
var type = void 0; | ||
var typeString = void 0; | ||
// Validate arguments | ||
var valid = validate(parsedArgs, { | ||
body: 'object' | ||
}); | ||
if (valid !== true) { | ||
throw valid; | ||
} | ||
if (parsedArgs.type === undefined || !(typeof parsedArgs.type === 'string' || Array.isArray(parsedArgs.type)) || parsedArgs.type === '' || parsedArgs.type.length === 0) { | ||
throw new Error('fields missing: type'); | ||
} | ||
valid = validate(parsedArgs.body, { | ||
query: 'object' | ||
}); | ||
if (valid !== true) { | ||
throw valid; | ||
} | ||
if (Array.isArray(parsedArgs.type)) { | ||
type = parsedArgs.type; | ||
typeString = parsedArgs.type.join(); | ||
} else { | ||
type = [parsedArgs.type]; | ||
typeString = parsedArgs.type; | ||
} | ||
var webhooks = []; | ||
var _bodyCopy = bodyCopy, | ||
query = _bodyCopy.query; | ||
if (typeof webhook === 'string') { | ||
var webHookObj = {}; | ||
webHookObj.url = webhook; | ||
webHookObj.method = 'GET'; | ||
webhooks.push(webHookObj); | ||
} else if (webhook.constructor === Array) { | ||
webhooks = webhook; | ||
} else if (webhook === Object(webhook)) { | ||
webhooks.push(webhook); | ||
} else { | ||
throw new Error('fields missing: second argument(webhook) is necessary'); | ||
} | ||
var populateBody = function populateBody() { | ||
bodyCopy = {}; | ||
bodyCopy.webhooks = webhooks; | ||
bodyCopy.query = query; | ||
bodyCopy.type = type; | ||
}; | ||
populateBody(); | ||
var encode64 = btoa(stringify(query)); | ||
var path = '.percolator/webhooks-0-' + typeString + '-0-' + encode64; | ||
this.change = function () { | ||
webhooks = []; | ||
if (typeof parsedArgs === 'string') { | ||
var webhook2 = {}; | ||
webhook2.url = parsedArgs; | ||
webhook2.method = 'POST'; | ||
webhooks.push(webhook2); | ||
} else if (parsedArgs.constructor === Array) { | ||
webhooks = parsedArgs; | ||
} else if (parsedArgs === Object(parsedArgs)) { | ||
webhooks.push(parsedArgs); | ||
} else { | ||
throw new Error('fields missing: one of webhook or url fields is required'); | ||
} | ||
populateBody(); | ||
return _this.performRequest('POST'); | ||
}; | ||
this.stop = function () { | ||
bodyCopy = undefined; | ||
return _this.performRequest('DELETE'); | ||
}; | ||
this.performRequest = function (method) { | ||
var res = _this.performWsRequest.apply(_this, [{ | ||
method: method, | ||
path: path, | ||
body: bodyCopy | ||
}].concat(rest)); | ||
res.change = _this.change; | ||
res.stop = _this.stop; | ||
return res; | ||
}; | ||
return this.performRequest('POST'); | ||
} | ||
/** | ||
* To get types | ||
*/ | ||
function getTypesService() { | ||
var _this = this; | ||
return new Promise(function (resolve, reject) { | ||
try { | ||
return _this.performFetchRequest({ | ||
method: 'GET', | ||
path: '_mapping' | ||
}).then(function (data) { | ||
var types = Object.keys(data[_this.app].mappings).filter(function (type) { | ||
return type !== '_default_'; | ||
}); | ||
return resolve(types); | ||
}); | ||
} catch (e) { | ||
return reject(e); | ||
} | ||
}); | ||
} | ||
/** | ||
* To get mappings | ||
@@ -1077,55 +668,45 @@ */ | ||
function index (config) { | ||
var client = new AppBase(config); | ||
function appbasejs(config) { | ||
var client = new AppBase(config); | ||
AppBase.prototype.performFetchRequest = fetchRequest; | ||
AppBase.prototype.performFetchRequest = fetchRequest; | ||
AppBase.prototype.performWsRequest = wsRequest; | ||
AppBase.prototype.index = indexApi; | ||
AppBase.prototype.index = indexApi; | ||
AppBase.prototype.get = getApi; | ||
AppBase.prototype.get = getApi; | ||
AppBase.prototype.update = updateApi; | ||
AppBase.prototype.update = updateApi; | ||
AppBase.prototype.delete = deleteApi; | ||
AppBase.prototype.delete = deleteApi; | ||
AppBase.prototype.bulk = bulkApi; | ||
AppBase.prototype.bulk = bulkApi; | ||
AppBase.prototype.search = searchApi; | ||
AppBase.prototype.search = searchApi; | ||
AppBase.prototype.msearch = msearchApi; | ||
AppBase.prototype.msearch = msearchApi; | ||
AppBase.prototype.reactiveSearchv3 = reactiveSearchv3Api; | ||
AppBase.prototype.reactiveSearchv3 = reactiveSearchv3Api; | ||
AppBase.prototype.getQuerySuggestions = getSuggestionsv3Api; | ||
AppBase.prototype.getQuerySuggestions = getSuggestionsv3Api; | ||
AppBase.prototype.getMappings = getMappings; | ||
AppBase.prototype.getStream = getStream; | ||
AppBase.prototype.setHeaders = function setHeaders() { | ||
var headers = arguments.length > 0 && arguments[0] !== undefined ? arguments[0] : {}; | ||
var shouldEncode = arguments.length > 1 && arguments[1] !== undefined ? arguments[1] : false; | ||
AppBase.prototype.searchStream = searchStreamApi; | ||
// Encode headers | ||
if (shouldEncode) { | ||
this.headers = encodeHeaders(headers); | ||
} else { | ||
this.headers = headers; | ||
} | ||
}; | ||
AppBase.prototype.searchStreamToURL = searchStreamToURLApi; | ||
AppBase.prototype.getTypes = getTypesService; | ||
AppBase.prototype.getMappings = getMappings; | ||
AppBase.prototype.setHeaders = function setHeaders() { | ||
var headers = arguments.length > 0 && arguments[0] !== undefined ? arguments[0] : {}; | ||
var shouldEncode = arguments.length > 1 && arguments[1] !== undefined ? arguments[1] : false; | ||
// Encode headers | ||
if (shouldEncode) { | ||
this.headers = encodeHeaders(headers); | ||
} else { | ||
this.headers = headers; | ||
} | ||
}; | ||
if (typeof window !== 'undefined') { | ||
window.Appbase = client; | ||
} | ||
return client; | ||
if (typeof window !== 'undefined') { | ||
window.Appbase = client; | ||
} | ||
return client; | ||
} | ||
export default index; | ||
export default appbasejs; |
@@ -1,2 +0,2 @@ | ||
!function(e,t){"object"==typeof exports&&"undefined"!=typeof module?module.exports=t():"function"==typeof define&&define.amd?define(t):e.Appbase=t()}(this,function(){"use strict";var e=function(e){var t=RegExp("^(([^:/?#]*)?://)?(((.*)?@)?([^/?#]*)?)([^?#]*)(\\?([^#]*))?(#(.*))?"),r=e.match(t);return{protocol:r[2],auth:r[5],host:r[6],path:r[7],query:r[9],hash:r[11]}},t="function"==typeof Symbol&&"symbol"==typeof Symbol.iterator?function(e){return typeof e}:function(e){return e&&"function"==typeof Symbol&&e.constructor===Symbol&&e!==Symbol.prototype?"symbol":typeof e};function r(){for(var e,t=arguments.length>0&&void 0!==arguments[0]?arguments[0]:"",r="",n=0,o=0,i="ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/=";t.charAt(0|o)||(i="=",o%1);r+=i.charAt(63&n>>8-o%1*8)){if((e=t.charCodeAt(o+=.75))>255)throw new Error('"btoa" failed: The string to be encoded contains characters outside of the Latin1 range.');n=n<<8|e}return r}function n(e){if(e&&"[object Array]"===Object.prototype.toString.call(e)){for(var t=0;t<e.length;t+=1){var r=e[t];if(!r)return new Error("query object can not have an empty value");if(!r.id)return new Error("'id' field must be present in query object")}return!0}return new Error("invalid query value, 'query' value must be an array")}function o(e,r){var n=[],o={object:null,string:"",number:0};Object.keys(r).forEach(function(i){var s=r[i].split("|").find(function(r){return t(e[i])===r});s&&e[i]!==o[s]||n.push(i)});for(var i="",s=0;s<n.length;s+=1)i+=n[s]+", ";return!(n.length>0)||new Error("fields missing: "+i)}function i(){var e=arguments.length>0&&void 0!==arguments[0]?arguments[0]:{};return e||0!==Object.keys(e).length||e.constructor!==Object?JSON.parse(JSON.stringify(e)):null}function s(t){var r=e(t.url||""),n=r.auth,o=void 0===n?null:n,i=r.host,s=void 0===i?"":i,a=r.path,u=void 0===a?"":a,f=r.protocol,c=void 0===f?"":f,p=s+u;if("string"!=typeof p||""===p)throw new Error("URL not present in options.");if("string"!=typeof t.app||""===t.app)throw new Error("App name is not present in options.");if("string"!=typeof c||""===c)throw new Error("Protocol is not present in url. URL should be of the form https://scalr.api.appbase.io");"/"===p.slice(-1)&&(p=p.slice(0,-1));var d=o||null;if("string"==typeof t.credentials&&""!==t.credentials?d=t.credentials:"string"==typeof t.username&&""!==t.username&&"string"==typeof t.password&&""!==t.password&&(d=t.username+":"+t.password),function(e){return t="scalr.api.appbase.io",-1!==e.indexOf(t);var t}(p)&&null===d)throw new Error("Authentication information is not present. Did you add credentials?");this.url=p,this.protocol=c,this.app=t.app,this.credentials=d,this.headers={}}var a="undefined"!=typeof window?window:"undefined"!=typeof global?global:"undefined"!=typeof self?self:{};function u(e,t){return e(t={exports:{}},t.exports),t.exports}function f(e,t){return Object.prototype.hasOwnProperty.call(e,t)}var c=function(e,t,r,n){t=t||"&",r=r||"=";var o={};if("string"!=typeof e||0===e.length)return o;var i=/\+/g;e=e.split(t);var s=1e3;n&&"number"==typeof n.maxKeys&&(s=n.maxKeys);var a=e.length;s>0&&a>s&&(a=s);for(var u=0;u<a;++u){var c,p,d,y,h=e[u].replace(i,"%20"),l=h.indexOf(r);l>=0?(c=h.substr(0,l),p=h.substr(l+1)):(c=h,p=""),d=decodeURIComponent(c),y=decodeURIComponent(p),f(o,d)?Array.isArray(o[d])?o[d].push(y):o[d]=[o[d],y]:o[d]=y}return o},p=function(e){switch(typeof e){case"string":return e;case"boolean":return e?"true":"false";case"number":return isFinite(e)?e:"";default:return""}},d=function(e,t,r,n){return t=t||"&",r=r||"=",null===e&&(e=void 0),"object"==typeof e?Object.keys(e).map(function(n){var o=encodeURIComponent(p(n))+r;return Array.isArray(e[n])?e[n].map(function(e){return o+encodeURIComponent(p(e))}).join(t):o+encodeURIComponent(p(e[n]))}).join(t):n?encodeURIComponent(p(n))+r+encodeURIComponent(p(e)):""},y=u(function(e,t){t.decode=t.parse=c,t.encode=t.stringify=d}),h=(y.decode,y.parse,y.encode,y.stringify,u(function(e){var t=function(e){function t(){this.fetch=!1}return t.prototype=e,new t}("undefined"!=typeof self?self:a);(function(e){!function(e){if(!e.fetch){var t={searchParams:"URLSearchParams"in e,iterable:"Symbol"in e&&"iterator"in Symbol,blob:"FileReader"in e&&"Blob"in e&&function(){try{return new Blob,!0}catch(e){return!1}}(),formData:"FormData"in e,arrayBuffer:"ArrayBuffer"in e};if(t.arrayBuffer)var r=["[object Int8Array]","[object Uint8Array]","[object Uint8ClampedArray]","[object Int16Array]","[object Uint16Array]","[object Int32Array]","[object Uint32Array]","[object Float32Array]","[object Float64Array]"],n=function(e){return e&&DataView.prototype.isPrototypeOf(e)},o=ArrayBuffer.isView||function(e){return e&&r.indexOf(Object.prototype.toString.call(e))>-1};c.prototype.append=function(e,t){e=a(e),t=u(t);var r=this.map[e];this.map[e]=r?r+","+t:t},c.prototype.delete=function(e){delete this.map[a(e)]},c.prototype.get=function(e){return e=a(e),this.has(e)?this.map[e]:null},c.prototype.has=function(e){return this.map.hasOwnProperty(a(e))},c.prototype.set=function(e,t){this.map[a(e)]=u(t)},c.prototype.forEach=function(e,t){for(var r in this.map)this.map.hasOwnProperty(r)&&e.call(t,this.map[r],r,this)},c.prototype.keys=function(){var e=[];return this.forEach(function(t,r){e.push(r)}),f(e)},c.prototype.values=function(){var e=[];return this.forEach(function(t){e.push(t)}),f(e)},c.prototype.entries=function(){var e=[];return this.forEach(function(t,r){e.push([r,t])}),f(e)},t.iterable&&(c.prototype[Symbol.iterator]=c.prototype.entries);var i=["DELETE","GET","HEAD","OPTIONS","POST","PUT"];b.prototype.clone=function(){return new b(this,{body:this._bodyInit})},l.call(b.prototype),l.call(v.prototype),v.prototype.clone=function(){return new v(this._bodyInit,{status:this.status,statusText:this.statusText,headers:new c(this.headers),url:this.url})},v.error=function(){var e=new v(null,{status:0,statusText:""});return e.type="error",e};var s=[301,302,303,307,308];v.redirect=function(e,t){if(-1===s.indexOf(t))throw new RangeError("Invalid status code");return new v(null,{status:t,headers:{location:e}})},e.Headers=c,e.Request=b,e.Response=v,e.fetch=function(e,r){return new Promise(function(n,o){var i=new b(e,r),s=new XMLHttpRequest;s.onload=function(){var e,t,r={status:s.status,statusText:s.statusText,headers:(e=s.getAllResponseHeaders()||"",t=new c,e.replace(/\r?\n[\t ]+/g," ").split(/\r?\n/).forEach(function(e){var r=e.split(":"),n=r.shift().trim();if(n){var o=r.join(":").trim();t.append(n,o)}}),t)};r.url="responseURL"in s?s.responseURL:r.headers.get("X-Request-URL");var o="response"in s?s.response:s.responseText;n(new v(o,r))},s.onerror=function(){o(new TypeError("Network request failed"))},s.ontimeout=function(){o(new TypeError("Network request failed"))},s.open(i.method,i.url,!0),"include"===i.credentials?s.withCredentials=!0:"omit"===i.credentials&&(s.withCredentials=!1),"responseType"in s&&t.blob&&(s.responseType="blob"),i.headers.forEach(function(e,t){s.setRequestHeader(t,e)}),s.send(void 0===i._bodyInit?null:i._bodyInit)})},e.fetch.polyfill=!0}function a(e){if("string"!=typeof e&&(e=String(e)),/[^a-z0-9\-#$%&'*+.\^_`|~]/i.test(e))throw new TypeError("Invalid character in header field name");return e.toLowerCase()}function u(e){return"string"!=typeof e&&(e=String(e)),e}function f(e){var r={next:function(){var t=e.shift();return{done:void 0===t,value:t}}};return t.iterable&&(r[Symbol.iterator]=function(){return r}),r}function c(e){this.map={},e instanceof c?e.forEach(function(e,t){this.append(t,e)},this):Array.isArray(e)?e.forEach(function(e){this.append(e[0],e[1])},this):e&&Object.getOwnPropertyNames(e).forEach(function(t){this.append(t,e[t])},this)}function p(e){if(e.bodyUsed)return Promise.reject(new TypeError("Already read"));e.bodyUsed=!0}function d(e){return new Promise(function(t,r){e.onload=function(){t(e.result)},e.onerror=function(){r(e.error)}})}function y(e){var t=new FileReader,r=d(t);return t.readAsArrayBuffer(e),r}function h(e){if(e.slice)return e.slice(0);var t=new Uint8Array(e.byteLength);return t.set(new Uint8Array(e)),t.buffer}function l(){return this.bodyUsed=!1,this._initBody=function(e){if(this._bodyInit=e,e)if("string"==typeof e)this._bodyText=e;else if(t.blob&&Blob.prototype.isPrototypeOf(e))this._bodyBlob=e;else if(t.formData&&FormData.prototype.isPrototypeOf(e))this._bodyFormData=e;else if(t.searchParams&&URLSearchParams.prototype.isPrototypeOf(e))this._bodyText=e.toString();else if(t.arrayBuffer&&t.blob&&n(e))this._bodyArrayBuffer=h(e.buffer),this._bodyInit=new Blob([this._bodyArrayBuffer]);else{if(!t.arrayBuffer||!ArrayBuffer.prototype.isPrototypeOf(e)&&!o(e))throw new Error("unsupported BodyInit type");this._bodyArrayBuffer=h(e)}else this._bodyText="";this.headers.get("content-type")||("string"==typeof e?this.headers.set("content-type","text/plain;charset=UTF-8"):this._bodyBlob&&this._bodyBlob.type?this.headers.set("content-type",this._bodyBlob.type):t.searchParams&&URLSearchParams.prototype.isPrototypeOf(e)&&this.headers.set("content-type","application/x-www-form-urlencoded;charset=UTF-8"))},t.blob&&(this.blob=function(){var e=p(this);if(e)return e;if(this._bodyBlob)return Promise.resolve(this._bodyBlob);if(this._bodyArrayBuffer)return Promise.resolve(new Blob([this._bodyArrayBuffer]));if(this._bodyFormData)throw new Error("could not read FormData body as blob");return Promise.resolve(new Blob([this._bodyText]))},this.arrayBuffer=function(){return this._bodyArrayBuffer?p(this)||Promise.resolve(this._bodyArrayBuffer):this.blob().then(y)}),this.text=function(){var e,t,r,n=p(this);if(n)return n;if(this._bodyBlob)return e=this._bodyBlob,t=new FileReader,r=d(t),t.readAsText(e),r;if(this._bodyArrayBuffer)return Promise.resolve(function(e){for(var t=new Uint8Array(e),r=new Array(t.length),n=0;n<t.length;n++)r[n]=String.fromCharCode(t[n]);return r.join("")}(this._bodyArrayBuffer));if(this._bodyFormData)throw new Error("could not read FormData body as text");return Promise.resolve(this._bodyText)},t.formData&&(this.formData=function(){return this.text().then(m)}),this.json=function(){return this.text().then(JSON.parse)},this}function b(e,t){var r,n,o=(t=t||{}).body;if(e instanceof b){if(e.bodyUsed)throw new TypeError("Already read");this.url=e.url,this.credentials=e.credentials,t.headers||(this.headers=new c(e.headers)),this.method=e.method,this.mode=e.mode,o||null==e._bodyInit||(o=e._bodyInit,e.bodyUsed=!0)}else this.url=String(e);if(this.credentials=t.credentials||this.credentials||"omit",!t.headers&&this.headers||(this.headers=new c(t.headers)),this.method=(r=t.method||this.method||"GET",n=r.toUpperCase(),i.indexOf(n)>-1?n:r),this.mode=t.mode||this.mode||null,this.referrer=null,("GET"===this.method||"HEAD"===this.method)&&o)throw new TypeError("Body not allowed for GET or HEAD requests");this._initBody(o)}function m(e){var t=new FormData;return e.trim().split("&").forEach(function(e){if(e){var r=e.split("="),n=r.shift().replace(/\+/g," "),o=r.join("=").replace(/\+/g," ");t.append(decodeURIComponent(n),decodeURIComponent(o))}}),t}function v(e,t){t||(t={}),this.type="default",this.status=void 0===t.status?200:t.status,this.ok=this.status>=200&&this.status<300,this.statusText="statusText"in t?t.statusText:"OK",this.headers=new c(t.headers),this.url=t.url||"",this._initBody(e)}}(void 0!==e?e:this)}).call(t,void 0);var r=t.fetch;r.Response=t.Response,r.Request=t.Request,r.Headers=t.Headers;e.exports&&(e.exports=r,e.exports.default=r)}));function l(e){var t=this;return new Promise(function(n,o){var s=i(e);try{var a=s.method,u=s.path,f=s.params,c=s.body,p=s.isRSAPI,d=s.isSuggestionsAPI?".suggestions":t.app,l=c,b=u.endsWith("msearch")||u.endsWith("bulk")?"application/x-ndjson":"application/json",m=Object.assign({},{Accept:"application/json","Content-Type":b},t.headers),v=Date.now();t.credentials&&(m.Authorization="Basic "+r(t.credentials));var w={method:a,headers:m};if(Array.isArray(l)){var g="";l.forEach(function(e){g+=JSON.stringify(e),g+="\n"}),l=g}else l=JSON.stringify(l)||{};0!==Object.keys(l).length&&(w.body=l);var x={},O="";f&&(O="?"+y.stringify(f));var j=t.protocol+"://"+t.url+"/"+d+"/"+u+O;return function(e){if(t.transformRequest&&"function"==typeof t.transformRequest){var r=t.transformRequest(e);return r instanceof Promise?r:Promise.resolve(r)}return Promise.resolve(e)}(Object.assign({},{url:j},w)).then(function(e){var t=Object.assign({},e),r=t.url;return delete t.url,h(r||j,t).then(function(e){return e.status>=500?o(e):(x=e.headers,e.json().then(function(t){if(e.status>=400)return o(e);if(t&&t.error)return o(t);if(p&&t&&"[object Object]"===Object.prototype.toString.call(t)&&c&&c.query&&c.query instanceof Array){var r=0,i=c.query.filter(function(e){return e.execute||void 0===e.execute}).length;if(t&&Object.keys(t).forEach(function(e){t[e]&&Object.prototype.hasOwnProperty.call(t[e],"error")&&(r+=1)}),r>0&&i===r)return o(t)}if(t&&t.responses instanceof Array&&t.responses.length===t.responses.filter(function(e){return Object.prototype.hasOwnProperty.call(e,"error")}).length)return o(t);var s=Object.assign({},t,{_timestamp:v,_headers:x});return n(s)}).catch(function(e){return o(e)}))}).catch(function(e){return o(e)})}).catch(function(e){return o(e)})}catch(e){return o(e)}})}var b="undefined"!=typeof window?window.WebSocket:require("ws");function m(e,n,o,s){var a=this;try{var u=i(e),f=u.method,c=u.path,p=u.params,d=e.body;d&&"object"===(void 0===d?"undefined":t(d))||(d={});return this.wsClosed=function(){s&&s()},this.stop=function(){a.ws.onmessage=void 0,a.ws.onclose=void 0,a.ws.onerror=void 0,a.wsClosed();var e=JSON.parse(JSON.stringify(a.request));e.unsubscribe=!0,!0!==a.unsubscribed&&a.send(e),a.unsubscribed=!0},this.reconnect=function(){return a.stop(),m(e,n,o,s)},this.processError=function(e){o?o(e):console.warn(e)},this.processMessage=function(e){var t=JSON.parse(JSON.stringify(e));if(t.id||!t.message)return t.id===a.id?t.message?(delete t.id,void(o&&o(t))):(t.query_id&&(a.query_id=t.query_id),t.channel&&(a.channel=t.channel),void(t.body&&""!==t.body&&n&&n(t.body))):void(!t.id&&t.channel&&t.channel===a.channel&&n&&n(t.event));o&&o(t)},a.ws=new b("wss://"+a.url+"/"+a.app),a.id="xxxxxxxx-xxxx-4xxx-yxxx-xxxxxxxxxxxx".replace(/[xy]/g,function(e){var t=16*Math.random()|0;return("x"===e?t:3&t|8).toString(16)}),a.request={id:a.id,path:a.app+"/"+c+"?"+y.stringify(p),method:f,body:d},a.credentials&&(a.request.authorization="Basic "+r(a.credentials)),a.result={},a.closeHandler=function(){a.wsClosed()},a.errorHandler=function(e){a.processError.apply(a,[e])},a.messageHandler=function(e){var t=JSON.parse(e.data);t.body&&t.body.status>=400?a.processError.apply(a,[t]):a.processMessage.apply(a,[t])},a.send=function(e){!function e(t,r){setTimeout(function(){1===t.readyState?null!=r&&r():e(t,r)},5)}(a.ws,function(){try{a.ws.send(JSON.stringify(e))}catch(e){console.warn(e)}})},a.ws.onmessage=a.messageHandler,a.ws.onerror=a.errorHandler,a.ws.onclose=a.closeHandler,a.send(a.request),a.result.stop=a.stop,a.result.reconnect=a.reconnect,a.result}catch(e){return o?o(e):console.warn(e),null}}function v(e){var t=i(e),r=o(t,{body:"object"});if(!0!==r)throw r;var n=t.type,s=void 0===n?"_doc":n,a=t.id,u=t.body;delete t.type,delete t.body,delete t.id;var f=void 0;return f=a?s?s+"/"+encodeURIComponent(a):encodeURIComponent(a):s,this.performFetchRequest({method:"POST",path:f,params:t,body:u})}function w(e){var t=i(e),r=o(t,{id:"string|number"});if(!0!==r)throw r;var n=t.type,s=void 0===n?"_doc":n,a=t.id;delete t.type,delete t.id;var u=s+"/"+encodeURIComponent(a);return this.performFetchRequest({method:"GET",path:u,params:t})}function g(e){var t=i(e),r=o(t,{id:"string|number",body:"object"});if(!0!==r)throw r;var n=t.type,s=void 0===n?"_doc":n,a=t.id,u=t.body;delete t.type,delete t.id,delete t.body;var f=s+"/"+encodeURIComponent(a)+"/_update";return this.performFetchRequest({method:"POST",path:f,params:t,body:u})}function x(e){var t=i(e),r=o(t,{id:"string|number"});if(!0!==r)throw r;var n=t.type,s=void 0===n?"_doc":n,a=t.id;delete t.type,delete t.id;var u=s+"/"+encodeURIComponent(a);return this.performFetchRequest({method:"DELETE",path:u,params:t})}function O(e){var t=i(e),r=o(t,{body:"object"});if(!0!==r)throw r;var n=t.type,s=t.body;delete t.type,delete t.body;var a=void 0;return a=n?n+"/_bulk":"_bulk",this.performFetchRequest({method:"POST",path:a,params:t,body:s})}function j(e){var t=i(e),r=o(t,{body:"object"});if(!0!==r)throw r;var n=void 0;n=Array.isArray(t.type)?t.type.join():t.type;var s=t.body;delete t.type,delete t.body;var a=void 0;return a=n?n+"/_search":"_search",this.performFetchRequest({method:"POST",path:a,params:t,body:s})}function A(e){var t=i(e),r=o(t,{body:"object"});if(!0!==r)throw r;var n=void 0;n=Array.isArray(t.type)?t.type.join():t.type;var s=t.body;delete t.type,delete t.body;var a=void 0;return a=n?n+"/_msearch":"_msearch",this.performFetchRequest({method:"POST",path:a,params:t,body:s})}function S(e,t){var r=i(t),o=n(e);if(!0!==o)throw o;var s={settings:r,query:e};return this.performFetchRequest({method:"POST",path:"_reactivesearch.v3",body:s,isRSAPI:!0})}function E(e){var t=i(e),r=o(t,{type:"string",id:"string|number"});if(!0!==r)throw r;var n=t.type,s=t.id;delete t.type,delete t.id,delete t.stream,!0===t.stream?t.stream="true":(delete t.stream,t.streamonly="true");for(var a=arguments.length,u=Array(a>1?a-1:0),f=1;f<a;f++)u[f-1]=arguments[f];return this.performWsRequest.apply(this,[{method:"GET",path:n+"/"+encodeURIComponent(s),params:t}].concat(u))}function R(e){var t=i(e),r=o(t,{body:"object"});if(!0!==r)throw r;if(void 0===t.type||Array.isArray(t.type)&&0===t.type.length)throw new Error("Missing fields: type");var n=void 0;n=Array.isArray(t.type)?t.type.join():t.type;var s=t.body;delete t.type,delete t.body,delete t.stream,t.streamonly="true";for(var a=arguments.length,u=Array(a>1?a-1:0),f=1;f<a;f++)u[f-1]=arguments[f];return this.performWsRequest.apply(this,[{method:"POST",path:n+"/_search",params:t,body:s}].concat(u))}var _,T,P,q,B={'"':'"',"\\":"\\","/":"/",b:"\b",f:"\f",n:"\n",r:"\r",t:"\t"},U=function(e){throw{name:"SyntaxError",message:e,at:_,text:P}},I=function(e){return e&&e!==T&&U("Expected '"+e+"' instead of '"+T+"'"),T=P.charAt(_),_+=1,T},C=function(){var e,t="";for("-"===T&&(t="-",I("-"));T>="0"&&T<="9";)t+=T,I();if("."===T)for(t+=".";I()&&T>="0"&&T<="9";)t+=T;if("e"===T||"E"===T)for(t+=T,I(),"-"!==T&&"+"!==T||(t+=T,I());T>="0"&&T<="9";)t+=T,I();if(e=+t,isFinite(e))return e;U("Bad number")},F=function(){var e,t,r,n="";if('"'===T)for(;I();){if('"'===T)return I(),n;if("\\"===T)if(I(),"u"===T){for(r=0,t=0;t<4&&(e=parseInt(I(),16),isFinite(e));t+=1)r=16*r+e;n+=String.fromCharCode(r)}else{if("string"!=typeof B[T])break;n+=B[T]}else n+=T}U("Bad string")},k=function(){for(;T&&T<=" ";)I()};q=function(){switch(k(),T){case"{":return function(){var e,t={};if("{"===T){if(I("{"),k(),"}"===T)return I("}"),t;for(;T;){if(e=F(),k(),I(":"),Object.hasOwnProperty.call(t,e)&&U('Duplicate key "'+e+'"'),t[e]=q(),k(),"}"===T)return I("}"),t;I(","),k()}}U("Bad object")}();case"[":return function(){var e=[];if("["===T){if(I("["),k(),"]"===T)return I("]"),e;for(;T;){if(e.push(q()),k(),"]"===T)return I("]"),e;I(","),k()}}U("Bad array")}();case'"':return F();case"-":return C();default:return T>="0"&&T<="9"?C():function(){switch(T){case"t":return I("t"),I("r"),I("u"),I("e"),!0;case"f":return I("f"),I("a"),I("l"),I("s"),I("e"),!1;case"n":return I("n"),I("u"),I("l"),I("l"),null}U("Unexpected '"+T+"'")}()}};var N,D,J,H=/[\\\"\x00-\x1f\x7f-\x9f\u00ad\u0600-\u0604\u070f\u17b4\u17b5\u200c-\u200f\u2028-\u202f\u2060-\u206f\ufeff\ufff0-\uffff]/g,L={"\b":"\\b","\t":"\\t","\n":"\\n","\f":"\\f","\r":"\\r",'"':'\\"',"\\":"\\\\"};function G(e){return H.lastIndex=0,H.test(e)?'"'+e.replace(H,function(e){var t=L[e];return"string"==typeof t?t:"\\u"+("0000"+e.charCodeAt(0).toString(16)).slice(-4)})+'"':'"'+e+'"'}var W="undefined"!=typeof JSON?JSON:{parse:function(e,t){var r;return P=e,_=0,T=" ",r=q(),k(),T&&U("Syntax error"),"function"==typeof t?function e(r,n){var o,i,s=r[n];if(s&&"object"==typeof s)for(o in s)Object.prototype.hasOwnProperty.call(s,o)&&(void 0!==(i=e(s,o))?s[o]=i:delete s[o]);return t.call(r,n,s)}({"":r},""):r},stringify:function(e,t,r){var n;if(N="",D="","number"==typeof r)for(n=0;n<r;n+=1)D+=" ";else"string"==typeof r&&(D=r);if(J=t,t&&"function"!=typeof t&&("object"!=typeof t||"number"!=typeof t.length))throw new Error("JSON.stringify");return function e(t,r){var n,o,i,s,a,u=N,f=r[t];switch(f&&"object"==typeof f&&"function"==typeof f.toJSON&&(f=f.toJSON(t)),"function"==typeof J&&(f=J.call(r,t,f)),typeof f){case"string":return G(f);case"number":return isFinite(f)?String(f):"null";case"boolean":case"null":return String(f);case"object":if(!f)return"null";if(N+=D,a=[],"[object Array]"===Object.prototype.toString.apply(f)){for(s=f.length,n=0;n<s;n+=1)a[n]=e(n,f)||"null";return i=0===a.length?"[]":N?"[\n"+N+a.join(",\n"+N)+"\n"+u+"]":"["+a.join(",")+"]",N=u,i}if(J&&"object"==typeof J)for(s=J.length,n=0;n<s;n+=1)"string"==typeof(o=J[n])&&(i=e(o,f))&&a.push(G(o)+(N?": ":":")+i);else for(o in f)Object.prototype.hasOwnProperty.call(f,o)&&(i=e(o,f))&&a.push(G(o)+(N?": ":":")+i);return i=0===a.length?"{}":N?"{\n"+N+a.join(",\n"+N)+"\n"+u+"}":"{"+a.join(",")+"}",N=u,i}}("",{"":e})}},M=function(e,t){t||(t={}),"function"==typeof t&&(t={cmp:t});var r=t.space||"";"number"==typeof r&&(r=Array(r+1).join(" "));var n,o="boolean"==typeof t.cycles&&t.cycles,i=t.replacer||function(e,t){return t},s=t.cmp&&(n=t.cmp,function(e){return function(t,r){var o={key:t,value:e[t]},i={key:r,value:e[r]};return n(o,i)}}),a=[];return function e(t,n,u,f){var c=r?"\n"+new Array(f+1).join(r):"",p=r?": ":":";if(u&&u.toJSON&&"function"==typeof u.toJSON&&(u=u.toJSON()),void 0!==(u=i.call(t,n,u))){if("object"!=typeof u||null===u)return W.stringify(u);if(z(u)){for(var d=[],y=0;y<u.length;y++){var h=e(u,y,u[y],f+1)||W.stringify(null);d.push(c+r+h)}return"["+d.join(",")+c+"]"}if(-1!==a.indexOf(u)){if(o)return W.stringify("__cycle__");throw new TypeError("Converting circular structure to JSON")}a.push(u);var l=K(u).sort(s&&s(u));for(d=[],y=0;y<l.length;y++){var b=e(u,n=l[y],u[n],f+1);if(b){var m=W.stringify(n)+p+b;d.push(c+r+m)}}return a.splice(a.indexOf(u),1),"{"+d.join(",")+c+"}"}}({"":e},"",e,0)},z=Array.isArray||function(e){return"[object Array]"==={}.toString.call(e)},K=Object.keys||function(e){var t=Object.prototype.hasOwnProperty||function(){return!0},r=[];for(var n in e)t.call(e,n)&&r.push(n);return r};function V(e,t){for(var n=arguments.length,s=Array(n>2?n-2:0),a=2;a<n;a++)s[a-2]=arguments[a];var u=this,f=i(e),c=f.body,p=void 0,d=void 0,y=o(f,{body:"object"});if(!0!==y)throw y;if(void 0===f.type||"string"!=typeof f.type&&!Array.isArray(f.type)||""===f.type||0===f.type.length)throw new Error("fields missing: type");if(!0!==(y=o(f.body,{query:"object"})))throw y;Array.isArray(f.type)?(p=f.type,d=f.type.join()):(p=[f.type],d=f.type);var h=[],l=c.query;if("string"==typeof t){var b={};b.url=t,b.method="GET",h.push(b)}else if(t.constructor===Array)h=t;else{if(t!==Object(t))throw new Error("fields missing: second argument(webhook) is necessary");h.push(t)}var m=function(){(c={}).webhooks=h,c.query=l,c.type=p};m();var v=".percolator/webhooks-0-"+d+"-0-"+r(M(l));return this.change=function(){if(h=[],"string"==typeof f){var e={};e.url=f,e.method="POST",h.push(e)}else if(f.constructor===Array)h=f;else{if(f!==Object(f))throw new Error("fields missing: one of webhook or url fields is required");h.push(f)}return m(),u.performRequest("POST")},this.stop=function(){return c=void 0,u.performRequest("DELETE")},this.performRequest=function(e){var t=u.performWsRequest.apply(u,[{method:e,path:v,body:c}].concat(s));return t.change=u.change,t.stop=u.stop,t},this.performRequest("POST")}function X(){var e=this;return new Promise(function(t,r){try{return e.performFetchRequest({method:"GET",path:"_mapping"}).then(function(r){var n=Object.keys(r[e.app].mappings).filter(function(e){return"_default_"!==e});return t(n)})}catch(e){return r(e)}})}function Q(){return this.performFetchRequest({method:"GET",path:"_mapping"})}function Y(e,t){var r=i(t),o=n(e);if(!0!==o)throw o;var s={settings:r,query:e};return this.performFetchRequest({method:"POST",path:"_reactivesearch.v3",body:s,isRSAPI:!0,isSuggestionsAPI:!0})}return function(e){var t=new s(e);return s.prototype.performFetchRequest=l,s.prototype.performWsRequest=m,s.prototype.index=v,s.prototype.get=w,s.prototype.update=g,s.prototype.delete=x,s.prototype.bulk=O,s.prototype.search=j,s.prototype.msearch=A,s.prototype.reactiveSearchv3=S,s.prototype.getQuerySuggestions=Y,s.prototype.getStream=E,s.prototype.searchStream=R,s.prototype.searchStreamToURL=V,s.prototype.getTypes=X,s.prototype.getMappings=Q,s.prototype.setHeaders=function(){var e=arguments.length>0&&void 0!==arguments[0]?arguments[0]:{},t=arguments.length>1&&void 0!==arguments[1]&&arguments[1];this.headers=t?function(){var e=arguments.length>0&&void 0!==arguments[0]?arguments[0]:{},t=!(arguments.length>1&&void 0!==arguments[1])||arguments[1],r={};return t?Object.keys(e).forEach(function(t){r[t]=encodeURI(e[t])}):r=e,r}(e):e},"undefined"!=typeof window&&(window.Appbase=t),t}}); | ||
!function(e,t){"object"==typeof exports&&"undefined"!=typeof module?module.exports=t():"function"==typeof define&&define.amd?define(t):e.Appbase=t()}(this,function(){"use strict";var e=function(e){var t=RegExp("^(([^:/?#]*)?://)?(((.*)?@)?([^/?#]*)?)([^?#]*)(\\?([^#]*))?(#(.*))?"),r=e.match(t);return{protocol:r[2],auth:r[5],host:r[6],path:r[7],query:r[9],hash:r[11]}},t="function"==typeof Symbol&&"symbol"==typeof Symbol.iterator?function(e){return typeof e}:function(e){return e&&"function"==typeof Symbol&&e.constructor===Symbol&&e!==Symbol.prototype?"symbol":typeof e};function r(e){if(e&&"[object Array]"===Object.prototype.toString.call(e)){for(var t=0;t<e.length;t+=1){var r=e[t];if(!r)return new Error("query object can not have an empty value");if(!r.id)return new Error("'id' field must be present in query object")}return!0}return new Error("invalid query value, 'query' value must be an array")}function o(e,r){var o=[],n={object:null,string:"",number:0};Object.keys(r).forEach(function(i){var s=r[i].split("|").find(function(r){return t(e[i])===r});s&&e[i]!==n[s]||o.push(i)});for(var i="",s=0;s<o.length;s+=1)i+=o[s]+", ";return!(o.length>0)||new Error("fields missing: "+i)}function n(){var e=arguments.length>0&&void 0!==arguments[0]?arguments[0]:{};return e||0!==Object.keys(e).length||e.constructor!==Object?JSON.parse(JSON.stringify(e)):null}function i(t){var r=e(t.url||""),o=r.auth,n=void 0===o?null:o,i=r.host,s=void 0===i?"":i,a=r.path,u=void 0===a?"":a,p=r.protocol,f=void 0===p?"":p,d=s+u;if("string"!=typeof d||""===d)throw new Error("URL not present in options.");if("string"!=typeof t.app||""===t.app)throw new Error("App name is not present in options.");if("string"!=typeof f||""===f)throw new Error("Protocol is not present in url. URL should be of the form https://scalr.api.appbase.io");"/"===d.slice(-1)&&(d=d.slice(0,-1));var c=n||null;if("string"==typeof t.credentials&&""!==t.credentials?c=t.credentials:"string"==typeof t.username&&""!==t.username&&"string"==typeof t.password&&""!==t.password&&(c=t.username+":"+t.password),function(e){return t="scalr.api.appbase.io",-1!==e.indexOf(t);var t}(d)&&null===c)throw new Error("Authentication information is not present. Did you add credentials?");this.url=d,this.protocol=f,this.app=t.app,this.credentials=c,this.headers={"X-Search-Client":"Appbase JS"},!1===t.enableTelemetry&&Object.assign(this.headers,{"X-Enable-Telemetry":t.enableTelemetry})}var s="undefined"!=typeof window?window:"undefined"!=typeof global?global:"undefined"!=typeof self?self:{};function a(e,t){return e(t={exports:{}},t.exports),t.exports}function u(e,t){return Object.prototype.hasOwnProperty.call(e,t)}var p=function(e,t,r,o){t=t||"&",r=r||"=";var n={};if("string"!=typeof e||0===e.length)return n;var i=/\+/g;e=e.split(t);var s=1e3;o&&"number"==typeof o.maxKeys&&(s=o.maxKeys);var a=e.length;s>0&&a>s&&(a=s);for(var p=0;p<a;++p){var f,d,c,h,y=e[p].replace(i,"%20"),l=y.indexOf(r);l>=0?(f=y.substr(0,l),d=y.substr(l+1)):(f=y,d=""),c=decodeURIComponent(f),h=decodeURIComponent(d),u(n,c)?Array.isArray(n[c])?n[c].push(h):n[c]=[n[c],h]:n[c]=h}return n},f=function(e){switch(typeof e){case"string":return e;case"boolean":return e?"true":"false";case"number":return isFinite(e)?e:"";default:return""}},d=function(e,t,r,o){return t=t||"&",r=r||"=",null===e&&(e=void 0),"object"==typeof e?Object.keys(e).map(function(o){var n=encodeURIComponent(f(o))+r;return Array.isArray(e[o])?e[o].map(function(e){return n+encodeURIComponent(f(e))}).join(t):n+encodeURIComponent(f(e[o]))}).join(t):o?encodeURIComponent(f(o))+r+encodeURIComponent(f(e)):""},c=a(function(e,t){t.decode=t.parse=p,t.encode=t.stringify=d}),h=(c.decode,c.parse,c.encode,c.stringify,a(function(e){var t=function(e){function t(){this.fetch=!1}return t.prototype=e,new t}("undefined"!=typeof self?self:s);(function(e){!function(e){if(!e.fetch){var t={searchParams:"URLSearchParams"in e,iterable:"Symbol"in e&&"iterator"in Symbol,blob:"FileReader"in e&&"Blob"in e&&function(){try{return new Blob,!0}catch(e){return!1}}(),formData:"FormData"in e,arrayBuffer:"ArrayBuffer"in e};if(t.arrayBuffer)var r=["[object Int8Array]","[object Uint8Array]","[object Uint8ClampedArray]","[object Int16Array]","[object Uint16Array]","[object Int32Array]","[object Uint32Array]","[object Float32Array]","[object Float64Array]"],o=function(e){return e&&DataView.prototype.isPrototypeOf(e)},n=ArrayBuffer.isView||function(e){return e&&r.indexOf(Object.prototype.toString.call(e))>-1};f.prototype.append=function(e,t){e=a(e),t=u(t);var r=this.map[e];this.map[e]=r?r+","+t:t},f.prototype.delete=function(e){delete this.map[a(e)]},f.prototype.get=function(e){return e=a(e),this.has(e)?this.map[e]:null},f.prototype.has=function(e){return this.map.hasOwnProperty(a(e))},f.prototype.set=function(e,t){this.map[a(e)]=u(t)},f.prototype.forEach=function(e,t){for(var r in this.map)this.map.hasOwnProperty(r)&&e.call(t,this.map[r],r,this)},f.prototype.keys=function(){var e=[];return this.forEach(function(t,r){e.push(r)}),p(e)},f.prototype.values=function(){var e=[];return this.forEach(function(t){e.push(t)}),p(e)},f.prototype.entries=function(){var e=[];return this.forEach(function(t,r){e.push([r,t])}),p(e)},t.iterable&&(f.prototype[Symbol.iterator]=f.prototype.entries);var i=["DELETE","GET","HEAD","OPTIONS","POST","PUT"];b.prototype.clone=function(){return new b(this,{body:this._bodyInit})},l.call(b.prototype),l.call(v.prototype),v.prototype.clone=function(){return new v(this._bodyInit,{status:this.status,statusText:this.statusText,headers:new f(this.headers),url:this.url})},v.error=function(){var e=new v(null,{status:0,statusText:""});return e.type="error",e};var s=[301,302,303,307,308];v.redirect=function(e,t){if(-1===s.indexOf(t))throw new RangeError("Invalid status code");return new v(null,{status:t,headers:{location:e}})},e.Headers=f,e.Request=b,e.Response=v,e.fetch=function(e,r){return new Promise(function(o,n){var i=new b(e,r),s=new XMLHttpRequest;s.onload=function(){var e,t,r={status:s.status,statusText:s.statusText,headers:(e=s.getAllResponseHeaders()||"",t=new f,e.replace(/\r?\n[\t ]+/g," ").split(/\r?\n/).forEach(function(e){var r=e.split(":"),o=r.shift().trim();if(o){var n=r.join(":").trim();t.append(o,n)}}),t)};r.url="responseURL"in s?s.responseURL:r.headers.get("X-Request-URL");var n="response"in s?s.response:s.responseText;o(new v(n,r))},s.onerror=function(){n(new TypeError("Network request failed"))},s.ontimeout=function(){n(new TypeError("Network request failed"))},s.open(i.method,i.url,!0),"include"===i.credentials?s.withCredentials=!0:"omit"===i.credentials&&(s.withCredentials=!1),"responseType"in s&&t.blob&&(s.responseType="blob"),i.headers.forEach(function(e,t){s.setRequestHeader(t,e)}),s.send(void 0===i._bodyInit?null:i._bodyInit)})},e.fetch.polyfill=!0}function a(e){if("string"!=typeof e&&(e=String(e)),/[^a-z0-9\-#$%&'*+.\^_`|~]/i.test(e))throw new TypeError("Invalid character in header field name");return e.toLowerCase()}function u(e){return"string"!=typeof e&&(e=String(e)),e}function p(e){var r={next:function(){var t=e.shift();return{done:void 0===t,value:t}}};return t.iterable&&(r[Symbol.iterator]=function(){return r}),r}function f(e){this.map={},e instanceof f?e.forEach(function(e,t){this.append(t,e)},this):Array.isArray(e)?e.forEach(function(e){this.append(e[0],e[1])},this):e&&Object.getOwnPropertyNames(e).forEach(function(t){this.append(t,e[t])},this)}function d(e){if(e.bodyUsed)return Promise.reject(new TypeError("Already read"));e.bodyUsed=!0}function c(e){return new Promise(function(t,r){e.onload=function(){t(e.result)},e.onerror=function(){r(e.error)}})}function h(e){var t=new FileReader,r=c(t);return t.readAsArrayBuffer(e),r}function y(e){if(e.slice)return e.slice(0);var t=new Uint8Array(e.byteLength);return t.set(new Uint8Array(e)),t.buffer}function l(){return this.bodyUsed=!1,this._initBody=function(e){if(this._bodyInit=e,e)if("string"==typeof e)this._bodyText=e;else if(t.blob&&Blob.prototype.isPrototypeOf(e))this._bodyBlob=e;else if(t.formData&&FormData.prototype.isPrototypeOf(e))this._bodyFormData=e;else if(t.searchParams&&URLSearchParams.prototype.isPrototypeOf(e))this._bodyText=e.toString();else if(t.arrayBuffer&&t.blob&&o(e))this._bodyArrayBuffer=y(e.buffer),this._bodyInit=new Blob([this._bodyArrayBuffer]);else{if(!t.arrayBuffer||!ArrayBuffer.prototype.isPrototypeOf(e)&&!n(e))throw new Error("unsupported BodyInit type");this._bodyArrayBuffer=y(e)}else this._bodyText="";this.headers.get("content-type")||("string"==typeof e?this.headers.set("content-type","text/plain;charset=UTF-8"):this._bodyBlob&&this._bodyBlob.type?this.headers.set("content-type",this._bodyBlob.type):t.searchParams&&URLSearchParams.prototype.isPrototypeOf(e)&&this.headers.set("content-type","application/x-www-form-urlencoded;charset=UTF-8"))},t.blob&&(this.blob=function(){var e=d(this);if(e)return e;if(this._bodyBlob)return Promise.resolve(this._bodyBlob);if(this._bodyArrayBuffer)return Promise.resolve(new Blob([this._bodyArrayBuffer]));if(this._bodyFormData)throw new Error("could not read FormData body as blob");return Promise.resolve(new Blob([this._bodyText]))},this.arrayBuffer=function(){return this._bodyArrayBuffer?d(this)||Promise.resolve(this._bodyArrayBuffer):this.blob().then(h)}),this.text=function(){var e,t,r,o=d(this);if(o)return o;if(this._bodyBlob)return e=this._bodyBlob,t=new FileReader,r=c(t),t.readAsText(e),r;if(this._bodyArrayBuffer)return Promise.resolve(function(e){for(var t=new Uint8Array(e),r=new Array(t.length),o=0;o<t.length;o++)r[o]=String.fromCharCode(t[o]);return r.join("")}(this._bodyArrayBuffer));if(this._bodyFormData)throw new Error("could not read FormData body as text");return Promise.resolve(this._bodyText)},t.formData&&(this.formData=function(){return this.text().then(m)}),this.json=function(){return this.text().then(JSON.parse)},this}function b(e,t){var r,o,n=(t=t||{}).body;if(e instanceof b){if(e.bodyUsed)throw new TypeError("Already read");this.url=e.url,this.credentials=e.credentials,t.headers||(this.headers=new f(e.headers)),this.method=e.method,this.mode=e.mode,n||null==e._bodyInit||(n=e._bodyInit,e.bodyUsed=!0)}else this.url=String(e);if(this.credentials=t.credentials||this.credentials||"omit",!t.headers&&this.headers||(this.headers=new f(t.headers)),this.method=(r=t.method||this.method||"GET",o=r.toUpperCase(),i.indexOf(o)>-1?o:r),this.mode=t.mode||this.mode||null,this.referrer=null,("GET"===this.method||"HEAD"===this.method)&&n)throw new TypeError("Body not allowed for GET or HEAD requests");this._initBody(n)}function m(e){var t=new FormData;return e.trim().split("&").forEach(function(e){if(e){var r=e.split("="),o=r.shift().replace(/\+/g," "),n=r.join("=").replace(/\+/g," ");t.append(decodeURIComponent(o),decodeURIComponent(n))}}),t}function v(e,t){t||(t={}),this.type="default",this.status=void 0===t.status?200:t.status,this.ok=this.status>=200&&this.status<300,this.statusText="statusText"in t?t.statusText:"OK",this.headers=new f(t.headers),this.url=t.url||"",this._initBody(e)}}(void 0!==e?e:this)}).call(t,void 0);var r=t.fetch;r.Response=t.Response,r.Request=t.Request,r.Headers=t.Headers;e.exports&&(e.exports=r,e.exports.default=r)}));function y(e){var t=this;return new Promise(function(r,o){var i=n(e);try{var s=i.method,a=i.path,u=i.params,p=i.body,f=i.isRSAPI,d=i.isSuggestionsAPI?".suggestions":t.app,y=p,l=a.endsWith("msearch")||a.endsWith("bulk")?"application/x-ndjson":"application/json",b=Object.assign({},{Accept:"application/json","Content-Type":l},t.headers),m=Date.now();t.credentials&&(b.Authorization="Basic "+function(){for(var e,t=arguments.length>0&&void 0!==arguments[0]?arguments[0]:"",r="",o=0,n=0,i="ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/=";t.charAt(0|n)||(i="=",n%1);r+=i.charAt(63&o>>8-n%1*8)){if((e=t.charCodeAt(n+=.75))>255)throw new Error('"btoa" failed: The string to be encoded contains characters outside of the Latin1 range.');o=o<<8|e}return r}(t.credentials));var v={method:s,headers:b};if(Array.isArray(y)){var w="";y.forEach(function(e){w+=JSON.stringify(e),w+="\n"}),y=w}else y=JSON.stringify(y)||{};0!==Object.keys(y).length&&(v.body=y);var g={},A="";u&&(A="?"+c.stringify(u));var R=t.protocol+"://"+t.url+"/"+d+"/"+a+A;return function(e){if(t.transformRequest&&"function"==typeof t.transformRequest){var r=t.transformRequest(e);return r instanceof Promise?r:Promise.resolve(r)}return Promise.resolve(e)}(Object.assign({},{url:R},v)).then(function(e){var t=Object.assign({},e),n=t.url;return delete t.url,h(n||R,t).then(function(e){return e.status>=500?o(e):(g=e.headers,e.json().then(function(t){if(e.status>=400)return o(e);if(t&&t.error)return o(t);if(f&&t&&"[object Object]"===Object.prototype.toString.call(t)&&p&&p.query&&p.query instanceof Array){var n=0,i=p.query.filter(function(e){return e.execute||void 0===e.execute}).length;if(t&&Object.keys(t).forEach(function(e){t[e]&&Object.prototype.hasOwnProperty.call(t[e],"error")&&(n+=1)}),n>0&&i===n)return o(t)}if(t&&t.responses instanceof Array&&t.responses.length===t.responses.filter(function(e){return Object.prototype.hasOwnProperty.call(e,"error")}).length)return o(t);var s=Object.assign({},t,{_timestamp:m,_headers:g});return r(s)}).catch(function(e){return o(e)}))}).catch(function(e){return o(e)})}).catch(function(e){return o(e)})}catch(e){return o(e)}})}function l(e){var t=n(e),r=o(t,{body:"object"});if(!0!==r)throw r;var i=t.type,s=void 0===i?"_doc":i,a=t.id,u=t.body;delete t.type,delete t.body,delete t.id;var p=void 0;return p=a?s?s+"/"+encodeURIComponent(a):encodeURIComponent(a):s,this.performFetchRequest({method:"POST",path:p,params:t,body:u})}function b(e){var t=n(e),r=o(t,{id:"string|number"});if(!0!==r)throw r;var i=t.type,s=void 0===i?"_doc":i,a=t.id;delete t.type,delete t.id;var u=s+"/"+encodeURIComponent(a);return this.performFetchRequest({method:"GET",path:u,params:t})}function m(e){var t=n(e),r=o(t,{id:"string|number",body:"object"});if(!0!==r)throw r;var i=t.type,s=void 0===i?"_doc":i,a=t.id,u=t.body;delete t.type,delete t.id,delete t.body;var p=s+"/"+encodeURIComponent(a)+"/_update";return this.performFetchRequest({method:"POST",path:p,params:t,body:u})}function v(e){var t=n(e),r=o(t,{id:"string|number"});if(!0!==r)throw r;var i=t.type,s=void 0===i?"_doc":i,a=t.id;delete t.type,delete t.id;var u=s+"/"+encodeURIComponent(a);return this.performFetchRequest({method:"DELETE",path:u,params:t})}function w(e){var t=n(e),r=o(t,{body:"object"});if(!0!==r)throw r;var i=t.type,s=t.body;delete t.type,delete t.body;var a=void 0;return a=i?i+"/_bulk":"_bulk",this.performFetchRequest({method:"POST",path:a,params:t,body:s})}function g(e){var t=n(e),r=o(t,{body:"object"});if(!0!==r)throw r;var i=void 0;i=Array.isArray(t.type)?t.type.join():t.type;var s=t.body;delete t.type,delete t.body;var a=void 0;return a=i?i+"/_search":"_search",this.performFetchRequest({method:"POST",path:a,params:t,body:s})}function A(e){var t=n(e),r=o(t,{body:"object"});if(!0!==r)throw r;var i=void 0;i=Array.isArray(t.type)?t.type.join():t.type;var s=t.body;delete t.type,delete t.body;var a=void 0;return a=i?i+"/_msearch":"_msearch",this.performFetchRequest({method:"POST",path:a,params:t,body:s})}function R(e,t){var o=n(t),i=r(e);if(!0!==i)throw i;var s={settings:o,query:e};return this.performFetchRequest({method:"POST",path:"_reactivesearch.v3",body:s,isRSAPI:!0})}function j(){return this.performFetchRequest({method:"GET",path:"_mapping"})}function O(e,t){var o=n(t),i=r(e);if(!0!==i)throw i;var s={settings:o,query:e};return this.performFetchRequest({method:"POST",path:"_reactivesearch.v3",body:s,isRSAPI:!0,isSuggestionsAPI:!0})}return function(e){var t=new i(e);return i.prototype.performFetchRequest=y,i.prototype.index=l,i.prototype.get=b,i.prototype.update=m,i.prototype.delete=v,i.prototype.bulk=w,i.prototype.search=g,i.prototype.msearch=A,i.prototype.reactiveSearchv3=R,i.prototype.getQuerySuggestions=O,i.prototype.getMappings=j,i.prototype.setHeaders=function(){var e=arguments.length>0&&void 0!==arguments[0]?arguments[0]:{},t=arguments.length>1&&void 0!==arguments[1]&&arguments[1];this.headers=t?function(){var e=arguments.length>0&&void 0!==arguments[0]?arguments[0]:{},t=!(arguments.length>1&&void 0!==arguments[1])||arguments[1],r={};return t?Object.keys(e).forEach(function(t){r[t]=encodeURI(e[t])}):r=e,r}(e):e},"undefined"!=typeof window&&(window.Appbase=t),t}}); | ||
//# sourceMappingURL=appbase-js.umd.min.js.map |
{ | ||
"name": "appbase-js", | ||
"version": "4.2.0", | ||
"version": "4.2.1", | ||
"main": "dist/appbase-js.cjs.js", | ||
@@ -8,2 +8,3 @@ "jsnext:main": "dist/appbase-js.es.js", | ||
"description": "Appbase.io streaming client lib for Javascript", | ||
"types": "dist/index.d.ts", | ||
"keywords": [ | ||
@@ -34,2 +35,3 @@ "appbase", | ||
"rollup-plugin-commonjs": "^9.1.0", | ||
"rollup-plugin-copy": "^3.4.0", | ||
"rollup-plugin-node-builtins": "^2.1.2", | ||
@@ -58,11 +60,5 @@ "rollup-plugin-node-resolve": "^3.3.0", | ||
"cross-fetch": "^2.2.2", | ||
"json-stable-stringify": "^1.0.1", | ||
"querystring": "^0.2.0", | ||
"stream": "^0.0.2", | ||
"url-parser-lite": "^0.1.0", | ||
"ws": "^6.1.2" | ||
}, | ||
"browser": { | ||
"ws": "./lib/ws-shim.js" | ||
"url-parser-lite": "^0.1.0" | ||
} | ||
} |
197
README.md
@@ -1,165 +0,114 @@ | ||
 | ||
##  | ||
# appbase-js | ||
<h2 id="top" align="center"> | ||
<img src="https://i.imgur.com/iiR9wAs.png" alt="appbase-js" title="appbase-js" width="200" /> | ||
<br /> | ||
appbase-js | ||
<br /> | ||
</h2> | ||
Appbase.io is a data streams library for Node.JS and Javascript (browser UMD build is in the [dist/](https://github.com/appbaseio/appbase-js/tree/master/dist) directory); compatible with [elasticsearch.js](https://www.elastic.co/guide/en/elasticsearch/client/javascript-api/current/index.html). | ||
[appbase-js](https://github.com/appbaseio/appbase-js) is a universal JavaScript client library for working with the appbase.io database, for Node.JS and Javascript (browser UMD build is in the [dist/](https://github.com/appbaseio/appbase-js/tree/master/dist) directory); compatible with [elasticsearch.js](https://www.elastic.co/guide/en/elasticsearch/client/javascript-api/current/index.html). | ||
An up-to-date documentation for Node.JS API is available at http://docs.appbase.io/javascript/quickstart.html. | ||
## Quick Example | ||
## TOC | ||
Working code snippets where each step builds on the previous ones. | ||
1. **[appbase-js: Intro](#1-appbase-js-intro)** | ||
2. **[Features](#2-features)** | ||
3. **[Live Examples](#3-live-examples)** | ||
4. **[Installation](#4-installation)** | ||
5. **[Docs Manual](#5-docs-manual)** | ||
6. **[Other Projects You Might Like](#6-other-projects-you-might-like)** | ||
#### Step 1: Add some data into the app (uses elasticsearch.js) | ||
<br /> | ||
## 1. appbase-js: Intro | ||
```js | ||
// app and authentication configurations | ||
const HOST_URL = "https://scalr.api.appbase.io"; | ||
const APPNAME = "createnewtestapp01"; | ||
const CREDENTIALS = "RIvfxo1u1:dee8ee52-8b75-4b5b-be4f-9df3c364f59f"; | ||
[appbase-js](https://github.com/appbaseio/appbase-js) is a universal JavaScript client library for working with the appbase.io database. | ||
// Add data into our ES "app index" | ||
var Appbase = require("appbase-js"); | ||
var appbase = Appbase({ | ||
url: HOST_URL, | ||
app: APPNAME, | ||
credentials: CREDENTIALS | ||
}); | ||
appbase | ||
.index({ | ||
type: "product", | ||
id: "1", | ||
body: { | ||
name: "A green door", | ||
price: 12.5, | ||
tags: ["home", "green"], | ||
stores: ["Walmart", "Target"] | ||
} | ||
}) | ||
.then(res => { | ||
console.log(res); | ||
}) | ||
.catch(err => { | ||
console.log(err); | ||
}); | ||
``` | ||
## 2. Features | ||
#### Step 2: Read the data stream from a particular DB location | ||
It can: | ||
Returns continous updates on a JSON document from a particular `type`. | ||
- Index new documents or update / delete existing ones. | ||
- Work universally with Node.JS, Browser, and React Native. | ||
```js | ||
appbase.getStream( | ||
{ | ||
type: "product", | ||
id: "1" | ||
}, | ||
data => { | ||
// "data" handler is triggered every time there is a **new** document update. | ||
console.log(data); | ||
}, | ||
error => { | ||
console.log("caught a stream error", error); | ||
} | ||
); | ||
``` | ||
It can't: | ||
`Note:` Existing document value is returned via `get()` method. | ||
- Configure mappings, change analyzers, or capture snapshots. All these are provided by [elasticsearch.js](https://www.elastic.co/guide/en/elasticsearch/client/javascript-api/current/index.html) - the official Elasticsearch JS client library. | ||
##### Console Output | ||
[Appbase.io - the database service](https://appbase.io) is opinionated about cluster setup and hence doesn't support the Elasticsearch devops APIs. See [rest.appbase.io](https://rest.appbase.io) for a full reference on the supported APIs. | ||
## 3. Live Examples | ||
<br /> | ||
<p>Check out the Live interactive Examples at <a href=" | ||
https://docs.appbase.io/api/examples/rest/" target="_blank">reactiveapps.io</a>.</p> | ||
<br/> | ||
[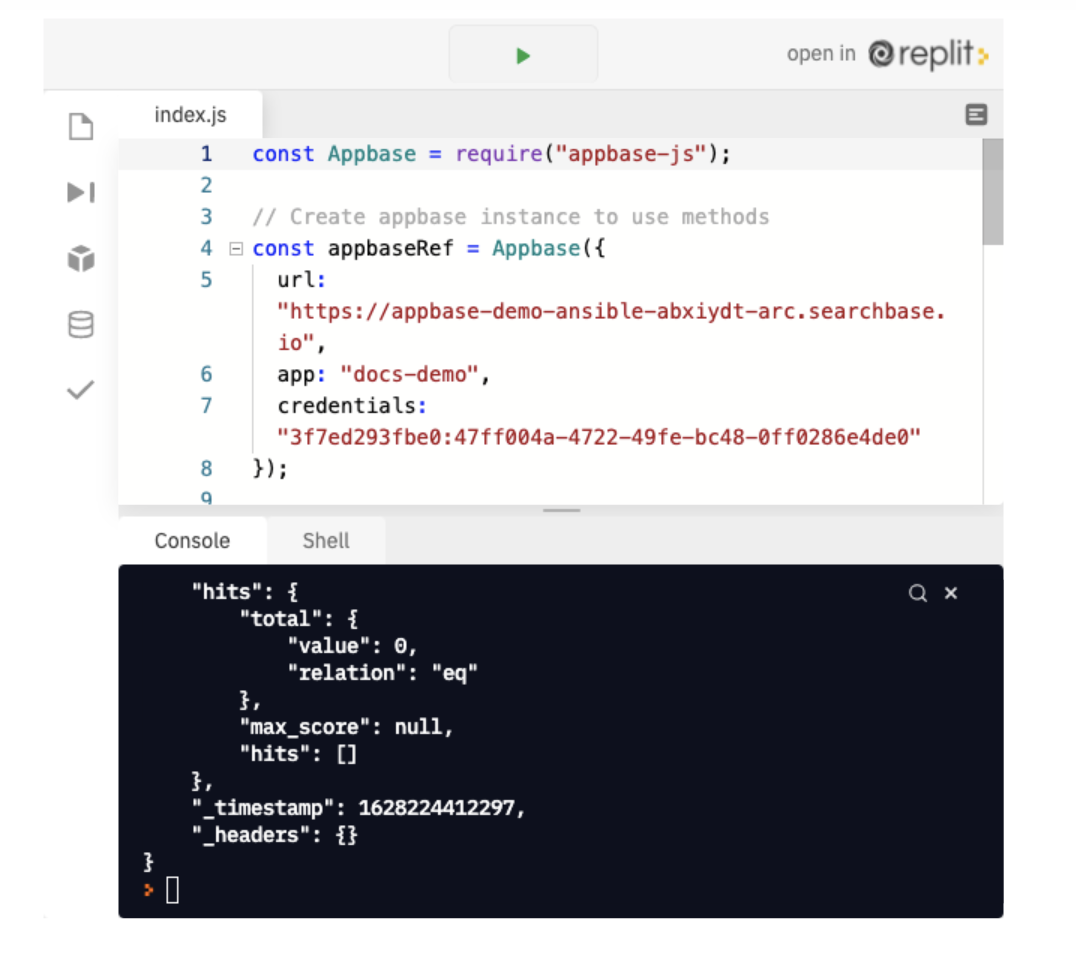](https://docs.appbase.io/api/examples/rest/) | ||
## 4. Installation | ||
We will fetch and install the **appbase-js** lib using npm. `4.0.0-beta` is the most current version. | ||
```js | ||
{ | ||
_index: "app`248", | ||
_type: "product", | ||
_id: "1", | ||
_version: 4, | ||
found: true, | ||
_source: { | ||
name: "A green door", | ||
price: 12.5, | ||
tags: [ "home", "green" ], | ||
stores: [ "Walmart", "Target" ] | ||
} | ||
} | ||
npm install appbase-js | ||
``` | ||
getStream() returns an object which has `stop` & `reconnect` properties. Check out the [getStreamTest.js](https://github.com/bietkul/appbase-js/blob/develop/__tests__/getStream.js) where we make an update to the document and see any further updates to it via the "data" callback. | ||
Adding it in the browser should be a one line script addition. | ||
#### Step 3: Apply queries on data streams | ||
```html | ||
<script | ||
defer | ||
src="https://unpkg.com/appbase-js/dist/appbase-js.umd.min.js" | ||
></script> | ||
``` | ||
Get continuous results by searching across the database streams. A query can be written using the [ElasticSearch Query DSL](https://www.elastic.co/guide/en/elasticsearch/reference/current/query-dsl.html) - which supports composing boolean, regex, geo, fuzzy, range queries. Let's stream the results of a simple **`match_all`** query on the `product` type: | ||
Alternatively, a UMD build of the library can be used directly from [jsDelivr](https://cdn.jsdelivr.net/npm/appbase-js/dist/). | ||
To write data to [appbase.io](https://appbase.io), we need to first create a reference object. We do this by passing the appbase.io API URL, app name, and credentials into the `Appbase` constructor: | ||
```js | ||
appbase | ||
.searchStream({ | ||
type: "product", | ||
body: { | ||
query: { | ||
match_all: {} | ||
} | ||
} | ||
}, | ||
data => { | ||
console.log(data); | ||
}, | ||
error => { | ||
console.log("caught a stream error", error); | ||
}) | ||
var appbaseRef = Appbase({ | ||
url: "https://appbase-demo-ansible-abxiydt-arc.searchbase.io", | ||
app: "good-books-demo", | ||
credentials: "c84fb24cbe08:db2a25b5-1267-404f-b8e6-cf0754953c68", | ||
}); | ||
``` | ||
##### Console Output | ||
**OR** | ||
```js | ||
{ | ||
took: 1, | ||
timed_out: false, | ||
_shards: { | ||
total: 1, | ||
successful: 1, | ||
failed: 0 | ||
}, | ||
hits: { | ||
total: 4, | ||
max_score: 1, | ||
hits: [ [Object], [Object], [Object], [Object] ] | ||
} | ||
} | ||
var appbaseRef = Appbase({ | ||
url: "https://c84fb24cbe08:db2a25b5-1267-404f-b8e6-cf0754953c68@appbase-demo-ansible-abxiydt-arc.searchbase.io", | ||
app: "good-books-demo", | ||
}); | ||
``` | ||
searchStream() also returns an object, which can be conveniently listened via the `onData` callback. Check out the [searchStreamTest.js](https://github.com/bietkul/appbase-js/blob/develop/__tests__/searchStreamTest.js) where we make an update that matches the query and see the results in the event stream. | ||
Credentials can also be directly passed as a part of the API URL. | ||
## API Reference | ||
## 5. Docs Manual | ||
For a complete API reference, check out [JS API Ref doc](http://docs.appbase.io/javascript/api-reference.html). | ||
### Global | ||
## 6. Other Projects You Might Like | ||
**[Appbase(args)](https://github.com/appbaseio/appbase-js/blob/master/appbase.js#L16)** | ||
- [**arc**](https://github.com/appbaseio/arc) API Gateway for ElasticSearch (Out of the box Security, Rate Limit Features, Record Analytics and Request Logs). | ||
Returns a **reference** object on which streaming requests can be performed. | ||
- [**searchbox**](https://github.com/appbaseio/searchox) A lightweight and performance focused searchbox UI libraries to query and display results from your ElasticSearch app (aka index). | ||
> **args** - A set of key/value pairs that configures the ElasticSearch Index | ||
> url: "https://scalr.api.appbase.io" | ||
> app: App name (equivalent to an ElasticSearch Index) | ||
> credentials: A `username:password` combination used for Basic Auth. | ||
- **Vanilla JS** - (~16kB Minified + Gzipped) | ||
- **React** - (~30kB Minified + Gzipped) | ||
- **Vue** - (~22kB Minified + Gzipped) | ||
Optionally (and like in the quick example above), `url` can contain the credentials field in the format: https://<credentials>@scalr.appbase.io. | ||
- [**dejavu**](https://github.com/appbaseio/dejavu) allows viewing raw data within an appbase.io (or Elasticsearch) app. **Soon to be released feature:** An ability to import custom data from CSV and JSON files, along with a guided walkthrough on applying data mappings. | ||
### Reference | ||
- [**mirage**](https://github.com/appbaseio/mirage) ReactiveSearch components can be extended using custom Elasticsearch queries. For those new to Elasticsearch, Mirage provides an intuitive GUI for composing queries. | ||
**[reference.getStream(args, onData, onError, onClose)](https://github.com/appbaseio/appbase-js/blob/master/appbase.js#L99)** | ||
- [**ReactiveMaps**](https://github.com/appbaseio/reactivesearch/tree/next/packages/maps) is a similar project to Reactive Search that allows building realtime maps easily. | ||
Get continuous updates on a JSON document with a `type` and `id`.Returns an object. | ||
- [**reactivesearch**](https://github.com/appbaseio/reactivesearch) UI components library for Elasticsearch: Available for React and Vue. | ||
> **args** - A set of key/value pairs that makes the document URL | ||
> type: ElasticSearch Type, a string | ||
> id: Valid Document ID | ||
[⬆ Back to Top](#top) | ||
**[reference.searchStream(args, onData, onError, onClose)](https://github.com/appbaseio/appbase-js/blob/master/appbase.js#L103)** | ||
Get continuous updates on search queries (fuzzy, boolean, geolocation, range, full-text).Returns an object. | ||
> **args** - A set of key/value pairs that makes the document URL | ||
> type: ElasticSearch Type, a string | ||
> body: A JSON Query Body (Any query matching the [ElasticSearch Query DSL](https://www.elastic.co/guide/en/elasticsearch/reference/current/query-dsl.html)) | ||
<a href="https://appbase.io/support/"><img src="https://i.imgur.com/UL6B0uE.png" width="100%" /></a> |
Sorry, the diff of this file is too big to display
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Minified code
QualityThis package contains minified code. This may be harmless in some cases where minified code is included in packaged libraries, however packages on npm should not minify code.
Found 1 instance in 1 package
3
1
235336
22
2489
115
- Removedjson-stable-stringify@^1.0.1
- Removedstream@^0.0.2
- Removedws@^6.1.2
- Removedasync-limiter@1.0.1(transitive)
- Removedcall-bind@1.0.7(transitive)
- Removeddefine-data-property@1.1.4(transitive)
- Removedemitter-component@1.1.2(transitive)
- Removedes-define-property@1.0.0(transitive)
- Removedes-errors@1.3.0(transitive)
- Removedfunction-bind@1.1.2(transitive)
- Removedget-intrinsic@1.2.4(transitive)
- Removedgopd@1.0.1(transitive)
- Removedhas-property-descriptors@1.0.2(transitive)
- Removedhas-proto@1.0.3(transitive)
- Removedhas-symbols@1.0.3(transitive)
- Removedhasown@2.0.2(transitive)
- Removedisarray@2.0.5(transitive)
- Removedjson-stable-stringify@1.1.1(transitive)
- Removedjsonify@0.0.1(transitive)
- Removedobject-keys@1.1.1(transitive)
- Removedset-function-length@1.2.2(transitive)
- Removedstream@0.0.2(transitive)
- Removedws@6.2.2(transitive)