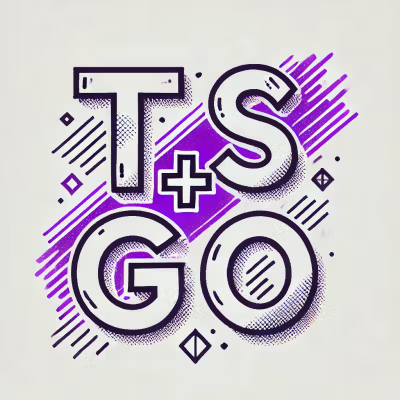
Security News
TypeScript is Porting Its Compiler to Go for 10x Faster Builds
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
application-compiler
Advanced tools
A Simple Compiler To Merge Multiple Files Into A Single File Application.
This package aims to bring an "include" method to Node applications that performs similar to include implementations in other languages such as PHP. Unlike Node's built in module system, this compiler aims to allow for the usage of simple calls such as include('/routes/api/v1/login.js')
in your code and produces a compiled javascript file with all include calls converted into their respective code simulating a direct include into global context.
Application Compiler can be installed using node package manager (npm
)
npm i application-compiler
Below are some examples making use of Compiler methods and properties.
const ApplicationCompiler = require('application-compiler');
// Create compiler instance for the root file which is index.js
const website_compiler = new ApplicationCompiler({
file_path: './index.js'
});
// Initiate automatic file writing
website_compiler.write_to({
path: './',
file_name: 'compiled_exec.js', // Run this using a process manager such as PM2
relative_errors: true, // We want custom error traces for faster debugging
});
// Bind an error logger
website_compiler.set_error_logger((path, error) => {
// Send the error and path to your own internal systems
});
// Bind an event logger to log underlying events during development
website_compiler.set_logger((message) => {
console.log(`[COMPILER] ${message}`);
});
const ApplicationCompiler = require('application-compiler');
// Create compiler instance for the root file which is index.js
const website_compiler = new ApplicationCompiler({
file_path: './index.js'
});
// Initiate a recalibration handler which will trigger custom processing on content changes
website_compiler.on_recalibration(() => {
let compiled_code = website_compiler.compiled;
// Do your own custom processing/compiled file writing here
});
// Bind an error logger
website_compiler.set_error_logger((path, error) => {
// Send the error and path to your own internal systems
});
// Bind an event logger to log underlying events during development
website_compiler.set_logger((message) => {
console.log(`[COMPILER] ${message}`);
});
Below is a breakdown of the Compiler
class generated when creating a application compiler instance.
file_path
[String
]: Path to the root/entry javascript file.
./master.js
watcher_delay
[Number
]: Delay to enforce between FileWatcher updates in milliseconds.
250
include_tag
[String
]: Name of include method used during compilation.
include
include
will convert all include(path)
to their respective compiled code.Property | Type | Description |
---|---|---|
compiled | String | Returns compiled application code. |
chunks | Object | Contains nested objects which represent compiled code. |
pool | WatcherPool | Contains underlying WatcherPool instance. |
watchers | Object | Contains FileWatcher instances with their handlers. |
write_to(Object: options)
: Begins automatic file writing on content changes.
options
: Automatic compilation options.
path
[String
]: Specifies where compiled file is written.file_name
[String
]: Specifies the name of the compiled file.
compiled_{root_file_name}.js
write_delay
[Number
]: Enforces delay between fast file writes in milliseconds.
250
relative_errors
[Boolean
]: Enables contextually relative Error traces for compile-time/syntax errors.
true
runtime_relative_errors
[Boolean
]: Enables contextually relative Error traces for run-time errors including uncaught promise exceptions.
true
require('application-compiler').log_relative_errors((String: error_trace) => { /* Your Code Here... */ })
process.exit(code)
at the end of your code for any custom handling above to ensure you restart the application.on_recalibration(Function: handler)
: Triggered when a file content change is detected and code is recompiled.
() => {}
set_error_handler(Function: handler)
: Sets error logger for all errors that occur in compiler.
(String: path, Error: error) => {}
path
: The path of the file where the internal error occured.error
: The error object of the error that has occured.FileSystem
errors.set_logger(Function: logger)
: Sets logger for logging compiler events.
(String: message) => {}
message
: Log messageEvent -> Hierarchy
INITIALIZED
: A new file has been loaded and is being watched.DETECTED_CHANGES
: A content change was detected triggering recalibration.DESTROYED
: This file has been destroyed and is no longer being watched./
root.js/routes.js/login.js
where root.js
is the file_path
and event occured in login.js
.destroy()
: Destroys compiler instance along with underlying WatcherPool
and nested file instances.FAQs
A Simple Compiler To Merge Multiple Files Into A Single File Application.
We found that application-compiler demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
Research
Security News
The Socket Research Team has discovered six new malicious npm packages linked to North Korea’s Lazarus Group, designed to steal credentials and deploy backdoors.
Security News
Socket CEO Feross Aboukhadijeh discusses the open web, open source security, and how Socket tackles software supply chain attacks on The Pair Program podcast.