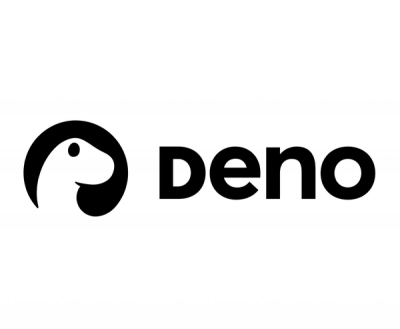
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
apxor-bi-widgets
Advanced tools
This project demonstrates the use of the `apxor-bi-widgets` package, which includes pre-built components for creating and rendering business intelligence visualizations. The sample app shows how to integrate and utilize these components effectively.
This project demonstrates the use of the apxor-bi-widgets
package, which includes pre-built components for creating and rendering business intelligence visualizations. The sample app shows how to integrate and utilize these components effectively.
To install apxor-bi-widgets, use npm:
npm install apxor-bi-widgets
# or
yarn add apxor-bi-widgets
# or
pnpm add apxor-bi-widgets
The BarGraph
component renders a bar graph based on the provided data.
Required Props:
data
: Array of objects with key
and value
propertiestitle
: String for the chart titleOptional Props:
[xAxisLabel]
: String representing X axis label[yAxisLabel]
: String representing Y axis label[showXAxisKeys]
: Boolean to show/hide X axis keys[showYAxisValues]
: Boolean to show/hide Y axis values[xAxisUnit]
: String representing the unit of measurement for X axis[yAxisUnit]
: String representing the unit of measurement for Y axis[showlegends]
: Boolean to show/hide legends[chartContainerStyles]
: Object for custom chart container styles[colorScheme="ColorsForStackedBar"]
: "SequentialRose" | "SequentialBlue" | "SequentialGreen" | "SequentialOrange" | "SequentialGray" | "DivergingGreenWhite" | "DivergingDarkGreenYellow" | "rainbowOf10" | "rainbowOf5" | "orangeShadeOf10" | "Random20" | "DivergingDarkPurpleBlueRed" | "ColorsForStackedBar"[height]
: Number for chart height[width]
: Number for chart width[legendSymbol]
: "circle" | "rectangle"[rootStyles]
: Object for custom root styles[titleStyles]
: Object for custom title styles[loading]
:Loading state if data is loadingThe BarGraphCreation
component provides an interface for creating and customizing bar graph.
Required Props:
xAxisProperties
: Array of strings for X axis propertiesyAxisProperties
: Array of { attribute: string; type: DATA_TYPE; }
filterProperties
: Array of filter property of { label: string; value: string; }
filterPropertieOperators
: Function that accepting operators of { label: string; value: string; }
based on the property type (string | float | int | datetime | date)filterPropertieValues
: Function that accepting values of { label: string; value: string; }
based on the selected propertyxAxisUnits
: Array of unit strings for X axisyAxisUnits
: Array of unit strings for Y axisonViewChart
: Function called when viewing a chart, returns a payload with all filled fieldsonSaveOrUpdateChart
: Function called when saving or updating a chart, returns a payload with all filled fieldsdashboardRedirectionOptions
: Array of { label: string; value: string; }
redirectionOptional Props:
[rootStyles]
: Object for custom root styles[isUpdate]
: Boolean key[updateProps]
: Object of all necessary fields for updating[isViewChartLoading]
:Loading state if view chart data is loading[expressionFormulaError]
: String of error for expression builderHere's a basic example of how to use the BarGraph
and BarGraphCreation
components from apxor-bi-widgets:
import {
ColorsSchemeForGraph,
DATA_TYPE,
OPERATOR,
BarGraph,
BarGraphCreation,
} from "apxor-bi-widgets";
import { useState } from "react";
// Sample data
const data = [
{ key: "Computer", value: 15 },
{ key: "Tab", value: 10 },
{ key: "Mobile", value: 20 },
{ key: "Phone", value: 25 },
];
function App() {
const [previewData, setPreviewData] = useState([]);
const [saveData, setSaveData] = useState([]);
return (
<div>
<h1>Business Intelligence Widgets Demo</h1>
<div>
<BarGraph
data={data}
title="Sample Bar Graph"
height={600}
width={600}
colorScheme="ColorsForStackedBar"
legendSymbol="circle"
showXAxisKeys={true}
showYAxisValues={true}
xAxisLabel="Days"
xAxisUnit="day"
yAxisUnit="sec"
yAxisLabel="Time"
/>
<BarGraphCreation
filterPropertieOperators={(type: DATA_TYPE) => {
// it will return selected property data type and it is accepting array of
// {
// label: string;
// value: string;
// }
// Implement your logic here
return [];
}}
filterPropertieValues={(property: string) => {
// it will return selected property and it is accepting array of
// {
// label: string;
// value: string;
// }
// Implement your logic here
return [];
}}
filterProperties={[
{ name: "property1", type: "string" },
{ name: "property2", type: "int" },
]}
xAxisProperties={["dimension1", "dimension2"]}
yAxisProperties={[
{ attribute: "CreatedTimeStamp", type: "string"},
{ attribute: "Source",type: "string"}
]}
dashboardRedirectionOptions={[
{ label: "Dashboard 1", value: "/dashboard1" },
{ label: "Dashboard 2", value: "/dashboard2" },
]}
onViewChart={(payload) => {
setPreviewData(payload);
}}
onSaveOrUpdateChart={(payload) => {
setSaveData(payload);
}}
xAxisUnits={["min", "sec", "ms", "hour", "day", "week", "month", "year"]}
yAxisUnits={["min", "sec", "ms", "hour", "day", "week", "month", "year"]}
/>
</div>
</div>
);
}
export default App;
The payload structure you will get after clicking on onViewChart
, onSaveOrUpdateChart
, and updateProps
will accept the same format is as follows:
{
visualization: {
name: string;
xAxisProperty: string;
aliasForXAxis: string;
aliasForYAxis: string;
yAxisProperties: {
formula: string;
events: {
[key: string]: {
name: string;
data_type: "string" | "float" | "int" | "datetime" | "date" | string;
};
};
};
};
customFilters: {
name: string;
operator: "EQ" | "NEQ" | "LT" | "LTE" | "GT" | "GTE" | "R";
data_type: "string" | "float" | "int" | "datetime" | "date" | string;
value: string[];
}[];
customization: {
colorScheme: "SequentialRose" | "SequentialBlue" | "SequentialGreen" | "SequentialOrange" | "SequentialGray" | "DivergingGreenWhite" | "DivergingDarkGreenYellow" | "rainbowOf10" | "rainbowOf5" | "orangeShadeOf10" | "Random20" | "DivergingDarkPurpleBlueRed" | "ColorsForStackedBar";
xAxisUnit: string | null;
yAxisUnit: string | null;
showXAxisKeys: boolean;
showYAxisValues: boolean;
showlegends: boolean;
dashboardRedirectionUrl: string | null;
};
}
The StackedBarGraph
component renders a stacked bar graph based on the provided data.
Required Props:
data
: Array of { key: string; [category: string]: string | number; }
title
: String for the chart titleOptional Props:
[xAxisLabel]
: String representing X axis label[yAxisLabel]
: String representing Y axis label[showXAxisKeys]
: Boolean to show/hide X axis keys[showYAxisValues]
: Boolean to show/hide Y axis values[yAxisUnit]
: String representing the unit of measurement for Y axis[showlegends]
: Boolean to show/hide legends[chartContainerStyles]
: Object for custom chart container styles[colorScheme="ColorsForStackedBar"]
: "SequentialRose" | "SequentialBlue" | "SequentialGreen" | "SequentialOrange" | "SequentialGray" | "DivergingGreenWhite" | "DivergingDarkGreenYellow" | "rainbowOf10" | "rainbowOf5" | "orangeShadeOf10" | "Random20" | "DivergingDarkPurpleBlueRed" | "ColorsForStackedBar"[height]
: Number for chart height[width]
: Number for chart width[legendSymbol]
: "circle" | "rectangle"[rootStyles]
: Object for custom root styles[titleStyles]
: Object for custom title styles[loading]
:Loading state if data is loadingThe StackedBarGraphCreation
component provides an interface for creating and customizing stacked bar graph.
Required Props:
xAxisProperties
: Array of { attribute: string; type: DATA_TYPE; }
yAxisProperties
: Array of { attribute: string; type: DATA_TYPE; }
filterProperties
: Array of filter property of { label: string; value: string; }
filterPropertieOperators
: Function that accepting operators of { label: string; value: string; }
based on the property type (string | float | int | datetime | date)filterPropertieValues
: Function that accepting values of { label: string; value: string; }
based on the selected propertyxAxisUnits
: Array of unit strings for X axisyAxisUnits
: Array of unit strings for Y axisonViewChart
: Function called when viewing a chart, returns a payload with all filled fieldsonSaveOrUpdateChart
: Function called when saving or updating a chart, returns a payload with all filled fieldsdashboardRedirectionOptions
: Array of { label: string; value: string; }
redirectionfinalTransformationOptions
:Array of { label: string; value: string; }
Optional Props:
[rootStyles]
: Object for custom root styles[isUpdate]
: Boolean key[updateProps]
: Object of all necessary fields for updating[isViewChartLoading]
:Loading state if view chart data is loading[expressionFormulaError]
: String of error for expression builderHere's a basic example of how to use the StackedBarGraph
and StackedBarGraphCreation
components from apxor-bi-widgets:
import {
ColorsSchemeForGraph,
DATA_TYPE,
OPERATOR,
StackedBarGraph,
StackedBarGraphCreation,
} from "apxor-bi-widgets";
import { useState } from "react";
// Sample data
const data = [
{ key: "January", desktop: 186, mobile: 80 },
{ key: "February", desktop: 305, mobile: 200 },
{ key: "March", desktop: 237, mobile: 120 },
{ key: "April", desktop: 73, mobile: 190 },
{ key: "May", desktop: 209, mobile: 130 },
{ key: "June", desktop: 214, mobile: 140 },
];
function App() {
const [previewData, setPreviewData] = useState([]);
const [saveData, setSaveData] = useState([]);
return (
<div>
<h1>Business Intelligence Widgets Demo</h1>
<div>
<StackedBarGraph
data={data}
title="Sample Stacked Bar Graph"
height={600}
width={600}
colorScheme="ColorsForStackedBar"
legendSymbol="circle"
showXAxisKeys={true}
showYAxisValues={true}
xAxisLabel="Days"
xAxisUnit="day"
yAxisUnit="sec"
yAxisLabel="Time"
/>
<StackedBarGraphCreation
filterPropertieOperators={(type: DATA_TYPE) => {
// it will return selected property data type and it is accepting array of
// {
// label: string;
// value: string;
// }
// Implement your logic here
return [];
}}
filterPropertieValues={(property: string) => {
// it will return selected property and it is accepting array of
// {
// label: string;
// value: string;
// }
// Implement your logic here
return [];
}}
filterProperties={[
{ name: "property1", type: "string" },
{ name: "property2", type: "int" },
]}
xAxisProperties={[
{ attribute: "CreatedTimeStamp", type: "string"},
{ attribute: "Source",type: "string"}
]}
yAxisProperties={[
{ attribute: "CreatedTimeStamp", type: "string"},
{ attribute: "Source",type: "string"}
]}
dashboardRedirectionOptions={[
{ label: "Dashboard 1", value: "/dashboard1" },
{ label: "Dashboard 2", value: "/dashboard2" },
]}
onViewChart={(payload) => {
setPreviewData(payload);
}}
onSaveOrUpdateChart={(payload) => {
setSaveData(payload);
}}
finalTransformationOptions={[
{
label: "Seconds To Timestamp",
value: "secondsToTimestamp",
},
{
label: "Minutes To Timestamp",
value: "minutesToTimestamp",
},
{
label: "In Days",
value: "toWeekDay",
},
]}
xAxisUnits={["min", "sec", "ms", "hour", "day", "week", "month", "year"]}
yAxisUnits={["min", "sec", "ms", "hour", "day", "week", "month", "year"]}
/>
</div>
</div>
);
}
export default App;
The payload structure you will get after clicking on onViewChart
, onSaveOrUpdateChart
, and updateProps
will accept the same format is as follows:
{
visualization: {
name: string;
xAxisProperty: string;
aliasForXAxis: string;
xAxisDataType: string;
yAxisDimensions: {
alias: string;
final_transformation: string;
formula: string;
events: {
[key: string]: {
name: string;
data_type: "string" | "float" | "int" | "datetime" | "date" | string;
};
};
dimention?: string;
dimention_type: "derived" | "normal";
}[];
};
customFilters: {
name: string;
operator: "EQ" | "NEQ" | "LT" | "LTE" | "GT" | "GTE" | "R";
data_type: "string" | "float" | "int" | "datetime" | "date" | string;
value: string[];
}[];
customization: {
colorScheme: "SequentialRose" | "SequentialBlue" | "SequentialGreen" | "SequentialOrange" | "SequentialGray" | "DivergingGreenWhite" | "DivergingDarkGreenYellow" | "rainbowOf10" | "rainbowOf5" | "orangeShadeOf10" | "Random20" | "DivergingDarkPurpleBlueRed" | "ColorsForStackedBar";
xAxisUnit: string | null;
yAxisUnit: string | null;
showXAxisKeys: boolean;
showYAxisValues: boolean;
showlegends: boolean;
dashboardRedirectionUrl: string | null;
};
}
The Aggregates
component renders a aggregates list based on the provided data.
Required Props:
type
: single | grouped
-Type of aggregatestitle
: String for the chart titledata
: if type is single
then data willl be { label: string; value: number; unit?: string; }
else Array of { label: string; value: number; unit?: string; }
Optional Props:
[colorForLabel]
: Color for label[colorForValue]
: Color for value[colorForUnit]
: Color for unit[fontSizeForLabel]
: Font size for label[fontSizeForValue]
: Font size for value[fontSizeForUnit]
: Font size for unit[backgroundColor]
: if type is single
[chartContainerStyles]
: Object for custom chart container styles[rootStyles]
: Object for custom root styles[titleStyles]
: Object for custom title styles[loading]
:Loading state if data is loadingThe AggregatesCreation
component provides an interface for creating and customizing aggregates.
Required Props:
aggregatesProperties
: Array of { attribute: string; type: DATA_TYPE; }
filterProperties
: Array of filter property of { label: string; value: string; }
filterPropertieOperators
: Function that accepting operators of { label: string; value: string; }
based on the property type (string | float | int | datetime | date)filterPropertieValues
: Function that accepting values of { label: string; value: string; }
based on the selected propertyunits
: Array of unit strings for X axisonViewChart
: Function called when viewing a chart, returns a payload with all filled fieldsonSaveOrUpdateChart
: Function called when saving or updating a chart, returns a payload with all filled fieldsdashboardRedirectionOptions
: Array of { label: string; value: string; }
redirectionfinalTransformationOptions
: Array of { label: string; value: string; }
Optional Props:
[rootStyles]
: Object for custom root styles[isUpdate]
: Boolean key[updateProps]
: Object of all necessary fields for updating[isViewChartLoading]
:Loading state if view chart data is loading[expressionFormulaError]
: String of error for expression builderHere's a basic example of how to use the Aggregates
and AggregatesCreation
components from apxor-bi-widgets:
import {
ColorsSchemeForGraph,
DATA_TYPE,
OPERATOR,
Aggregates,
AggregatesCreation,
} from "apxor-bi-widgets";
import { useState } from "react";
// Sample data
const data = [
{
label: "Call Count",
value: 2000,
unit: "sec",
}, {
label: "Call Duration",
value: 10,
unit: "min",
},
];
function App() {
const [previewData, setPreviewData] = useState([]);
const [saveData, setSaveData] = useState([]);
return (
<div>
<h1>Business Intelligence Widgets Demo</h1>
<div>
<Aggregates
data={data}
title="Sample Aggregates"
type="grouped"
colorForLabel="#000000"
colorForValue="#000000"
colorForUnit="#000000"
fontSizeForLabel={14}
fontSizeForValue={18}
fontSizeForUnit={10}
/>
<AggregatesCreation
filterPropertieOperators={(type: DATA_TYPE) => {
// it will return selected property data type and it is accepting array of
// {
// label: string;
// value: string;
// }
// Implement your logic here
return [];
}}
filterPropertieValues={(property: string) => {
// it will return selected property and it is accepting array of
// {
// label: string;
// value: string;
// }
// Implement your logic here
return [];
}}
filterProperties={[
{ name: "property1", type: "string" },
{ name: "property2", type: "int" },
]}
aggregatesProperties={[
{ attribute: "CreatedTimeStamp", type: "string"},
{ attribute: "Source",type: "string"}
]}
dashboardRedirectionOptions={[
{ label: "Dashboard 1", value: "/dashboard1" },
{ label: "Dashboard 2", value: "/dashboard2" },
]}
onViewChart={(payload) => {
setPreviewData(payload);
}}
onSaveOrUpdateChart={(payload) => {
setSaveData(payload);
}}
finalTransformationOptions={[
{
label: "Seconds To Timestamp",
value: "secondsToTimestamp",
},
{
label: "Minutes To Timestamp",
value: "minutesToTimestamp",
},
{
label: "In Days",
value: "toWeekDay",
},
]}
units={["min", "sec", "ms", "hour", "day", "week", "month", "year"]}
/>
</div>
</div>
);
}
export default App;
The payload structure you will get after clicking on onViewChart
, onSaveOrUpdateChart
, and updateProps
will accept the same format is as follows:
{
visualization: {
name?: string;
aggregateType: "grouped" | "single";
aggregates: {
alias: string;
final_transformation: string;
formula: string;
events: {
[key: string]: {
name: string;
data_type: "string" | "float" | "int" | "datetime" | "date" | string;
};
};
filters: {
name: string;
operator: OPERATOR;
data_type: "string" | "float" | "int" | "datetime" | "date" | string;
value: string[];
}[];
unit: string;
}[];
};
customization: {
colorForLabel: string;
fontSizeForLabel: number;
colorForValue: string;
fontSizeForValue: number;
colorForUnit: string;
fontSizeForUnit: number;
backgroundColor?: string | null;
dashboardRedirectionUrl: string | null;
};
}
The LineGraph
component renders a line/area graph based on the provided data.
Required Props:
data
: Array of objects with key
and value
properties. if granularity=day
then key must be a date
title
: String for the chart titlechartType
: Type of chart. either line
or area
granularity
: Granularity. day
or hour
aliasOfMetric
: Alias of metricvalueType
: "average" | "min" | "max" | "median" | "sum" | "start" | "end"
Optional Props:
[colorForValue]
: Color for value[fontSizeForValue]
: Font size for value[colorForUnit]
:Color for unit[fontSizeForUnit]
: Font size for unit[unit]
: String representing the unit of measurement for value[enableXAxisLabels]
: Boolean to show/hide X axis labels[enableYAxisLabels]
: Boolean to show/hide Y axis labels[chartContainerStyles]
: Object for custom chart container styles[color]
: "SkyBlue" | "Charcoal" | "LightGreen" | "Peach" | "Lavender" | "Pink" | "Gold" | "Teal" | "Coral" | "Aqua"
[height]
: Number for chart height[width]
: Number for chart width[legendSymbol]
: "circle" | "rectangle"[rootStyles]
: Object for custom root styles[titleStyles]
: Object for custom title styles[loading]
:Loading state if data is loadingThe LineGraphCreation
component provides an interface for creating and customizing line graph.
Required Props:
metricProperties
: Array of { attribute: string; type: DATA_TYPE; }
filterProperties
: Array of filter property of { label: string; value: string; }
filterPropertieOperators
: Function that accepting operators of { label: string; value: string; }
based on the property type (string | float | int | datetime | date)filterPropertieValues
: Function that accepting values of { label: string; value: string; }
based on the selected propertyunits
: Array of unit strings for X axisonViewChart
: Function called when viewing a chart, returns a payload with all filled fieldsonSaveOrUpdateChart
: Function called when saving or updating a chart, returns a payload with all filled fieldsdashboardRedirectionOptions
: Array of { label: string; value: string; }
redirectionOptional Props:
[rootStyles]
: Object for custom root styles[isUpdate]
: Boolean key[updateProps]
: Object of all necessary fields for updating[isViewChartLoading]
:Loading state if view chart data is loading[expressionFormulaError]
: String of error for expression builderHere's a basic example of how to use the LineGraph
and LineGraphCreation
components from apxor-bi-widgets:
import {
ColorsSchemeForGraph,
DATA_TYPE,
OPERATOR,
LineGraph,
LineGraphCreation,
} from "apxor-bi-widgets";
import { useState } from "react";
// Sample data
const data = [
{ key: "15", value: 15 },
{ key: "10", value: 10 },
{ key: "20", value: 20 },
{ key: "25", value: 25 },
];
function App() {
const [previewData, setPreviewData] = useState([]);
const [saveData, setSaveData] = useState([]);
return (
<div>
<h1>Business Intelligence Widgets Demo</h1>
<div>
<LineGraph
data={data}
height={300}
width={600}
chartType="area"
granularity="hour"
aliasOfMetric="Count"
valueType="average"
title="Sample Line Graph"
enableYAxisLabels
enableXAxisLabels
unit="k"
fontSizeForValue={50}
colorForValue="red"
/>
<LineGraphCreation
filterPropertieOperators={(type: DATA_TYPE) => {
// it will return selected property data type and it is accepting array of
// {
// label: string;
// value: string;
// }
// Implement your logic here
return [];
}}
filterPropertieValues={(property: string) => {
// it will return selected property and it is accepting array of
// {
// label: string;
// value: string;
// }
// Implement your logic here
return [];
}}
filterProperties={[
{ name: "property1", type: "string" },
{ name: "property2", type: "int" },
]}
metricProperties={[
{ attribute: "CreatedTimeStamp", type: "string"},
{ attribute: "Source",type: "string"}
]}
dashboardRedirectionOptions={[
{ label: "Dashboard 1", value: "/dashboard1" },
{ label: "Dashboard 2", value: "/dashboard2" },
]}
onViewChart={(payload) => {
setPreviewData(payload);
}}
onSaveOrUpdateChart={(payload) => {
setSaveData(payload);
}}
units={["min", "sec", "ms", "hour", "day", "week", "month", "year"]}
/>
</div>
</div>
);
}
export default App;
The payload structure you will get after clicking on onViewChart
, onSaveOrUpdateChart
, and updateProps
will accept the same format is as follows:
{
visualization: {
name: string;
aliasForMetric: string;
metrics:{
formula: string;
events: {
[key: string]: {
name: string;
data_type: DATA_TYPE | string;
};
};
};
granularity: "hour" | "day";
};
customFilters: {
name: string;
operator: "EQ" | "NEQ" | "LT" | "LTE" | "GT" | "GTE" | "R";
data_type: "string" | "float" | "int" | "datetime" | "date" | string;
value: string[];
}[];
customization: {
color: `"SkyBlue" | "Charcoal" | "LightGreen" | "Peach" | "Lavender" | "Pink" | "Gold" | "Teal" | "Coral" | "Aqua"`;
chartType: "area" | "line";
lineValueType: LINE_VALUE_TYPE;
unit: string | null;
colorForValue?: string;
fontSizeForValue?: number;
colorForUnit?: string;
fontSizeForUnit?: number;
enableXAxisLabels: boolean;
enableYAxisLabels: boolean;
dashboardRedirectionUrl: string | null;
};
}
The PieChart
component renders a pie chart based on the provided data.
Required Props:
data
: Array of objects with key
and value
propertiestitle
: String for the chart titleshowDataLabels
: Boolean to show/hide data labelsshowDataValues
: Boolean to show/hide data valuesshowlegends
: Boolean to show/hide legendsunit
: String representing the unit of measurementOptional Props:
[chartContainerStyles]
: Object for custom chart container styles[chartType="pieChart"]
: "pieChart" | "donutChart"[colorScheme="ColorsForStackedBar"]
: "SequentialRose" | "SequentialBlue" | "SequentialGreen" | "SequentialOrange" | "SequentialGray" | "DivergingGreenWhite" | "DivergingDarkGreenYellow" | "rainbowOf10" | "rainbowOf5" | "orangeShadeOf10" | "Random20" | "DivergingDarkPurpleBlueRed" | "ColorsForStackedBar"[height]
: Number for chart height[legendSymbol]
: "circle" | "rectangle"[rootStyles]
: Object for custom root styles[titleStyles]
: Object for custom title styles[width]
: Number for chart width[loading]
:Loading state if data is loadingThe PieChartCreation
component provides an interface for creating and customizing pie charts.
Required Props:
dimensions
: Array of dimension stringsidentifiers
: Array of identifier stringsfilterProperties
: Array of filter property objectsfilterPropertieOperators
: Function that accepting operators based on the property type (string | float | int | datetime | date)filterPropertieValues
: Function that accepting values based on the selected propertyunits
: Array of unit stringsonViewChart
: Function called when viewing a chart, returns a payload with all filled fieldsonSaveOrUpdateChart
: Function called when saving or updating a chart, returns a payload with all filled fieldsdashboardRedirectionOptions
: Array of redirection optionsOptional Props:
[rootStyles]
: Object for custom root styles[isUpdate]
: Boolean key[updateProps]
: Object of all necessary fields for updating[isViewChartLoading]
:Loading state if view chart data is loadingHere's a basic example of how to use the PieChart
and PieChartCreation
components from apxor-bi-widgets:
import {
ColorsSchemeForGraph,
DATA_TYPE,
OPERATOR,
PieChart,
PieChartCreation,
} from "apxor-bi-widgets";
import { useState } from "react";
// Sample data for PieChart
const data = [
{ key: "Computer", value: 15 },
{ key: "Tab", value: 10 },
{ key: "Mobile", value: 20 },
{ key: "Phone", value: 25 },
];
function App() {
const [previewData, setPreviewData] = useState([]);
const [saveData, setSaveData] = useState([]);
return (
<div>
<h1>Business Intelligence Widgets Demo</h1>
<div>
<PieChart
data={data}
title="Sample Pie Chart"
showDataLabels
showDataValues
showlegends
unit="min"
chartType="pieChart"
colorScheme="ColorsForStackedBar"
height={300}
width={330}
legendSymbol="circle"
/>
<PieChartCreation
filterPropertieOperators={(type: DATA_TYPE) => {
// Implement your logic here
return [];
}}
dimensions={["dimension1", "dimension2"]}
identifiers={["identifier1", "identifier2"]}
filterPropertieValues={(property: string) => {
// it will return selected property and it is accepting array of string based on property
// Implement your logic here
return [];
}}
filterProperties={[
{ name: "property1", type: "string" },
{ name: "property2", type: "int" },
]}
dashboardRedirectionOptions={[
{ label: "Dashboard 1", value: "/dashboard1" },
{ label: "Dashboard 2", value: "/dashboard2" },
]}
onViewChart={(payload) => {
setPreviewData(payload);
}}
onSaveOrUpdateChart={(payload) => {
setSaveData(payload);
}}
units={["min", "sec", "ms", "hour", "day", "week", "month", "year"]}
/>
</div>
</div>
);
}
export default App;
The payload structure you will get after clicking on onViewChart
, onSaveOrUpdateChart
, and updateProps
will accept the same format is as follows:
{
visualization: {
name: string;
identifier: string;
dimension: string;
};
customFilters: {
name: string;
operator: "EQ" | "NEQ" | "LT" | "LTE" | "GT" | "GTE" | "R";
data_type: "string" | "float" | "int" | "datetime" | "date" | string;
value: string[];
}[];
customization: {
colorScheme: "SequentialRose" | "SequentialBlue" | "SequentialGreen" | "SequentialOrange" | "SequentialGray" | "DivergingGreenWhite" | "DivergingDarkGreenYellow" | "rainbowOf10" | "rainbowOf5" | "orangeShadeOf10" | "Random20" | "DivergingDarkPurpleBlueRed" | "ColorsForStackedBar";
chartType: "pieChart" | "donutChart";
unit: string | null;
showDataValues: boolean;
showDataLabels: boolean;
showlegends: boolean;
dashboardRedirectionUrl: string | null;
};
}
The Table
component renders a table widget based on the provided data.
Required Props:
data
: Array of any key & value
pairtitle
: String for the chart titleOptional Props:
[chartContainerStyles]
: Object for custom chart container styles[height]
: Number for chart height[width]
: Number for chart width[rootStyles]
: Object for custom root styles[titleStyles]
: Object for custom title styles[loading]
:Loading state if data is loadingThe TableCreation
component provides an interface for creating and customizing Table widget.
Required Props:
dimensionProperties
: Array of { attribute: string; type: DATA_TYPE; }
filterProperties
: Array of filter property of { label: string; value: string; }
filterPropertieOperators
: Function that accepting operators of { label: string; value: string; }
based on the property type (string | float | int | datetime | date)filterPropertieValues
: Function that accepting values of { label: string; value: string; }
based on the selected propertyonViewChart
: Function called when viewing a chart, returns a payload with all filled fieldsonSaveOrUpdateChart
: Function called when saving or updating a chart, returns a payload with all filled fieldsdashboardRedirectionOptions
: Array of { label: string; value: string; }
redirectionfinalTransformationOptions
:Array of { label: string; value: string; }
Optional Props:
[rootStyles]
: Object for custom root styles[isUpdate]
: Boolean key[updateProps]
: Object of all necessary fields for updating[isViewChartLoading]
:Loading state if view chart data is loading[expressionFormulaError]
: String of error for expression builderHere's a basic example of how to use the Table
and TableCreation
components from apxor-bi-widgets:
import {
ColorsSchemeForGraph,
DATA_TYPE,
OPERATOR,
Table,
TableCreation,
} from "apxor-bi-widgets";
import { useState } from "react";
// Sample data
const data = [
{ Customer: "preprod",Logins: "191",},
{ Customer: "ozoneca10", Logins: "83",},
{ Customer: "RokoSubuser", Logins: "4",},
];
function App() {
const [previewData, setPreviewData] = useState([]);
const [saveData, setSaveData] = useState([]);
return (
<div>
<h1>Business Intelligence Widgets Demo</h1>
<div>
<Table
data={data}
title="Sample Table Widget"
/>
<TableCreation
filterPropertieOperators={(type: DATA_TYPE) => {
// it will return selected property data type and it is accepting array of
// {
// label: string;
// value: string;
// }
// Implement your logic here
return [];
}}
filterPropertieValues={(property: string) => {
// it will return selected property and it is accepting array of
// {
// label: string;
// value: string;
// }
// Implement your logic here
return [];
}}
filterProperties={[
{ name: "property1", type: "string" },
{ name: "property2", type: "int" },
]}
dimensionProperties={[
{ attribute: "CreatedTimeStamp", type: "string"},
{ attribute: "Source",type: "string"}
]}
dashboardRedirectionOptions={[
{ label: "Dashboard 1", value: "/dashboard1" },
{ label: "Dashboard 2", value: "/dashboard2" },
]}
onViewChart={(payload) => {
setPreviewData(payload);
}}
onSaveOrUpdateChart={(payload) => {
setSaveData(payload);
}}
finalTransformationOptions={[
{
label: "Seconds To Timestamp",
value: "secondsToTimestamp",
},
{
label: "Minutes To Timestamp",
value: "minutesToTimestamp",
},
{
label: "In Days",
value: "toWeekDay",
},
]}
/>
</div>
</div>
);
}
export default App;
The payload structure you will get after clicking on onViewChart
, onSaveOrUpdateChart
, and updateProps
will accept the same format is as follows:
{
visualization: {
name: string;
dimensions: {
alias: string;
final_transformation: string;
formula: string;
events: {
[key: string]: {
name: string;
data_type: "string" | "float" | "int" | "datetime" | "date" | string;
};
};
dimention?: string;
dimentionDataType?: "string" | "float" | "int" | "datetime" | "date" | string;
dimention_type: "derived" | "normal";
}[];
};
customFilters: {
name: string;
operator: "EQ" | "NEQ" | "LT" | "LTE" | "GT" | "GTE" | "R";
data_type: "string" | "float" | "int" | "datetime" | "date" | string;
value: string[];
}[];
customization: {
dashboardRedirectionUrl: string | null;
};
}
The PieChart
component renders a pie chart based on the provided data.
Required Props:
data
: Array of objects with key
and value
propertiestitle
: String for the chart titleshowDataLabels
: Boolean to show/hide data labelsshowDataValues
: Boolean to show/hide data valuesshowlegends
: Boolean to show/hide legendsunit
: String representing the unit of measurementOptional Props:
[chartContainerStyles]
: Object for custom chart container styles[chartType="pieChart"]
: "pieChart" | "donutChart"[colorScheme="ColorsForStackedBar"]
: "SequentialRose" | "SequentialBlue" | "SequentialGreen" | "SequentialOrange" | "SequentialGray" | "DivergingGreenWhite" | "DivergingDarkGreenYellow" | "rainbowOf10" | "rainbowOf5" | "orangeShadeOf10" | "Random20" | "DivergingDarkPurpleBlueRed" | "ColorsForStackedBar"[height]
: Number for chart height[legendSymbol]
: "circle" | "rectangle"[rootStyles]
: Object for custom root styles[titleStyles]
: Object for custom title styles[width]
: Number for chart width[loading]
:Loading state if data is loadingThe AdvancedPieChartCreation
component provides an interface for creating and customizing advanced pie chart.
Required Props:
identifierProperties
: Array of { attribute: string; type: DATA_TYPE; }
dimensionProperties
: Array of { attribute: string; type: DATA_TYPE; }
filterProperties
: Array of filter property of { label: string; value: string; }
filterPropertieOperators
: Function that accepting operators of { label: string; value: string; }
based on the property type (string | float | int | datetime | date)filterPropertieValues
: Function that accepting values of { label: string; value: string; }
based on the selected propertyunits
: Array of unit strings for X axisonViewChart
: Function called when viewing a chart, returns a payload with all filled fieldsonSaveOrUpdateChart
: Function called when saving or updating a chart, returns a payload with all filled fieldsdashboardRedirectionOptions
: Array of { label: string; value: string; }
redirectionOptional Props:
[rootStyles]
: Object for custom root styles[isUpdate]
: Boolean key[updateProps]
: Object of all necessary fields for updating[isViewChartLoading]
:Loading state if view chart data is loading[expressionFormulaError]
: String of error for expression builderHere's a basic example of how to use the PieChart
and AdvancedPieChartCreation
components from apxor-bi-widgets:
import {
ColorsSchemeForGraph,
DATA_TYPE,
OPERATOR,
PieChart,
AdvancedPieChartCreation,
} from "apxor-bi-widgets";
import { useState } from "react";
// Sample data
const data = [
{ key: "15", value: 15 },
{ key: "10", value: 10 },
{ key: "20", value: 20 },
{ key: "25", value: 25 },
];
function App() {
const [previewData, setPreviewData] = useState([]);
const [saveData, setSaveData] = useState([]);
return (
<div>
<h1>Business Intelligence Widgets Demo</h1>
<div>
<PieChart
data={data}
title="Sample Pie Chart"
showDataLabels
showDataValues
showlegends
unit="min"
chartType="pieChart"
colorScheme="ColorsForStackedBar"
height={300}
width={330}
legendSymbol="circle"
/>
<AdvancedPieChartCreation
identifierProperties={[
{ attribute: "CreatedTimeStamp", type: "string"},
{ attribute: "Source",type: "string"}
]}
dimensionProperties={[
{ attribute: "CreatedTimeStamp", type: "string"},
{ attribute: "Source",type: "string"}
]}
filterPropertieOperators={(type: DATA_TYPE) => {
// it will return selected property data type and it is accepting array of
// {
// label: string;
// value: string;
// }
// Implement your logic here
return [];
}}
filterPropertieValues={(property: string) => {
// it will return selected property and it is accepting array of
// {
// label: string;
// value: string;
// }
// Implement your logic here
return [];
}}
filterProperties={[
{ name: "property1", type: "string" },
{ name: "property2", type: "int" },
]}
dashboardRedirectionOptions={[
{ label: "Dashboard 1", value: "/dashboard1" },
{ label: "Dashboard 2", value: "/dashboard2" },
]}
onViewChart={(payload) => {
setPreviewData(payload);
}}
onSaveOrUpdateChart={(payload) => {
setSaveData(payload);
}}
units={["min", "sec", "ms", "hour", "day", "week", "month", "year"]}
/>
</div>
</div>
);
}
export default App;
The payload structure you will get after clicking on onViewChart
, onSaveOrUpdateChart
, and updateProps
will accept the same format is as follows:
{
visualization: {
name: string;
identifies: {
alias?: string;
formula: string;
events: {
[key: string]: {
name: string;
data_type:"string" | "float" | "int" | "datetime" | "date" | string;
};
};
};
dimensions: {
alias?: string;
formula: string;
events: {
[key: string]: {
name: string;
data_type:"string" | "float" | "int" | "datetime" | "date" | string;
};
};
};
};
customFilters: {
name: string;
operator: "EQ" | "NEQ" | "LT" | "LTE" | "GT" | "GTE" | "R";
data_type: "string" | "float" | "int" | "datetime" | "date" | string;
value: string[];
}[];
customization: {
colorScheme: "SequentialRose" | "SequentialBlue" | "SequentialGreen" | "SequentialOrange" | "SequentialGray" | "DivergingGreenWhite" | "DivergingDarkGreenYellow" | "rainbowOf10" | "rainbowOf5" | "orangeShadeOf10" | "Random20" | "DivergingDarkPurpleBlueRed" | "ColorsForStackedBar";
chartType: "pieChart" | "donutChart";
unit: string | null;
showDataValues: boolean;
showDataLabels: boolean;
showlegends: boolean;
dashboardRedirectionUrl: string | null;
};
}
This project is licensed under the MIT License.
FAQs
This project demonstrates the use of the `apxor-bi-widgets` package, which includes pre-built components for creating and rendering business intelligence visualizations. The sample app shows how to integrate and utilize these components effectively.
The npm package apxor-bi-widgets receives a total of 1 weekly downloads. As such, apxor-bi-widgets popularity was classified as not popular.
We found that apxor-bi-widgets demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.