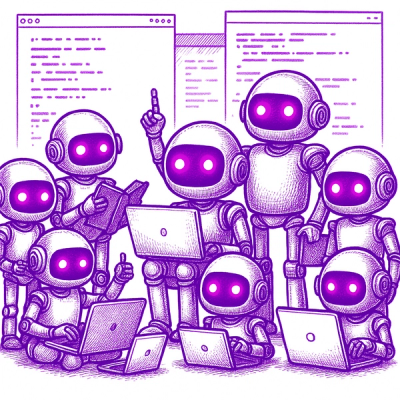
Security News
Open Source CAI Framework Handles Pen Testing Tasks up to 3,600× Faster Than Humans
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
An array convenience subclass for javascript.
$ npm install arc-array --save
The following example creates a new ArcArray, filters out empty and false values and iterates to a specific value before breaking
var ArcArray = require('arc-array');
//Equivalent to ['a','','b',false,'c']
var someArray = new ArcArray('a','','b',false,'c');
//Filter out '' and false values
someArray.quickFilter(['',false]);
//Loop through the array
someArray.each(function(_value,_index){
if(_value === 'b'){
//Break when we hit the value 'b'
return false;
}
});
Create a new ArcArray
object. Requires new
Loop over an array, calling callback each iteration. Break when false is explicitly returned.
callback is a required function that is called with 3 arguments passed in
thisContext is an optional object that will be available inside of the callback as 'this' if set, otherwise defaulting to the original array object
//Example of breaking each
var items = new ArcArray('a','b','c');
items.each(function(_index,_value,_array){
if(_value === 'b'){
return false; //Return explicit false to break
}
});
Similar to each, but accepts a second value that is passed in initially, and then potentially passed in on each subsequent iteration. And then eventually returned.
Default behavior still uses a hard false
return as a break, but this can be disabled optionally by passing in false as the third argument.
//Example of returnEach
var numbers = new ArcArray(1,2,3,4,5,6);
var even = numbers.each(function(_index,_value,_evenArray){
if(_value%2 === 0){
_evenArray.push(_value);
}
return _evenArray;
},[]);
//Even contains [2,4,6]
###.contains(val:Mixed)
This is a convenience function for return ([].indexOf(val) !== -1 ? true : false)
//Example of contains
var alpha = new ArcArray('a','b','c');
alpha.contains('a'); //returns true
alpha.contains('z'); //returns false
Filter out values in array that match values in passed in array. Returns original ArcArray
values is an array of values that are compared against individual values in the array. If a match is found, the value in the array is automatically removed.
//Example of quickFilter
var items = new ArcArray('a','b','c');
items.quickFilter(['b']); //items is reduced to ['a','c']
Use an ArcCheck object to perform complex evaluation on a value to decide whether or not it should be removed (see ArcCheck for more details on use). Returns original ArcArray.
Create a string based on the returned values from a callback on each index of an array.
callback is a function that receives each value of the array, and is expected to return a value that will be used to create the joined string.
//Example of joinCallback
var items = new ArcArray('item1','item2','item3');
var string = items.joinCallback(function(_val){
return '<li>'+_val+'</li>'
},'');
//String returned is: <li>item1</li><li>item2</li><li>item3</li>
###ArcArray.nativeBind() This is a static method that binds a method to the native global array prototype that transforms any array into an ArcArray object. This has a global effect and should be used carefully.
ArcArray.nativeBind();
var items = [1,'a','b',false].arc(); //This returns an ArcArray object
###ArcArray.wrap(array:Array) Accept an array, and if it is already an ArcArray return the same object, otherwise create a new ArcArray utilizing the passed in array
##Testing
npm test
FAQs
An array convenience subclass
The npm package arc-array receives a total of 713 weekly downloads. As such, arc-array popularity was classified as not popular.
We found that arc-array demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.