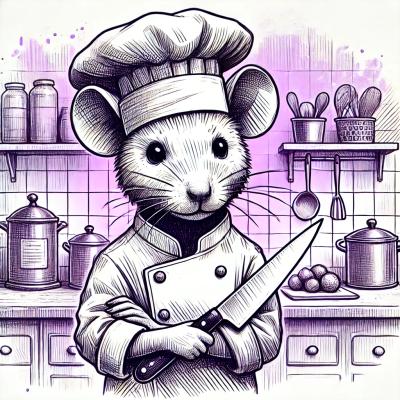
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
The archiver npm package is a streaming interface for archive generation, allowing users to create and manage different types of compressed files programmatically. It supports formats like ZIP and TAR and can be used for tasks such as creating backups, delivering files in a compressed format, or bundling project assets.
Creating ZIP archives
This code demonstrates how to create a ZIP file named 'example.zip' with a single file 'file.txt' included. It sets the compression level to 9 using zlib.
const fs = require('fs');
const archiver = require('archiver');
const output = fs.createWriteStream('example.zip');
const archive = archiver('zip', { zlib: { level: 9 } });
output.on('close', function() {
console.log(`Archive size: ${archive.pointer()} bytes`);
});
archive.pipe(output);
archive.append(fs.createReadStream('file.txt'), { name: 'file.txt' });
archive.finalize();
Creating TAR archives
This code snippet shows how to create a TAR file named 'example.tar' with gzip compression, including the file 'file.txt'.
const fs = require('fs');
const archiver = require('archiver');
const output = fs.createWriteStream('example.tar');
const archive = archiver('tar', { gzip: true });
output.on('close', function() {
console.log(`Archive size: ${archive.pointer()} bytes`);
});
archive.pipe(output);
archive.append(fs.createReadStream('file.txt'), { name: 'file.txt' });
archive.finalize();
Appending multiple files and directories
This example demonstrates how to append multiple files and directories to a ZIP archive. It includes a single file, a directory, and all JavaScript files in the current directory using a glob pattern.
const fs = require('fs');
const archiver = require('archiver');
const output = fs.createWriteStream('example.zip');
const archive = archiver('zip');
archive.pipe(output);
archive.file('file1.txt', { name: 'file1.txt' });
archive.directory('subdir/', 'new-subdir');
archive.glob('*.js');
archive.finalize();
JSZip is a JavaScript library for creating, reading, and editing .zip files. It works in many environments including the browser and Node.js. Compared to archiver, JSZip provides a more comprehensive API for manipulating ZIP files, including reading and editing existing archives, but it may not be as streamlined for simply generating archives.
The tar npm package provides the ability to create and extract .tar files. It is similar to archiver's TAR functionality but is more focused and does not support ZIP files. It is a good choice if you only need to work with TAR files.
Compressing is a node module that supports both tar and zip formats for compression and decompression. It offers a similar feature set to archiver but with a different API design. It might be used as an alternative if the API design aligns better with a developer's needs.
A streaming interface for archive generation
Visit the API documentation for a list of all methods available.
npm install archiver --save
// require modules
const fs = require('fs');
const archiver = require('archiver');
// create a file to stream archive data to.
const output = fs.createWriteStream(__dirname + '/example.zip');
const archive = archiver('zip', {
zlib: { level: 9 } // Sets the compression level.
});
// listen for all archive data to be written
// 'close' event is fired only when a file descriptor is involved
output.on('close', function() {
console.log(archive.pointer() + ' total bytes');
console.log('archiver has been finalized and the output file descriptor has closed.');
});
// This event is fired when the data source is drained no matter what was the data source.
// It is not part of this library but rather from the NodeJS Stream API.
// @see: https://nodejs.org/api/stream.html#stream_event_end
output.on('end', function() {
console.log('Data has been drained');
});
// good practice to catch warnings (ie stat failures and other non-blocking errors)
archive.on('warning', function(err) {
if (err.code === 'ENOENT') {
// log warning
} else {
// throw error
throw err;
}
});
// good practice to catch this error explicitly
archive.on('error', function(err) {
throw err;
});
// pipe archive data to the file
archive.pipe(output);
// append a file from stream
const file1 = __dirname + '/file1.txt';
archive.append(fs.createReadStream(file1), { name: 'file1.txt' });
// append a file from string
archive.append('string cheese!', { name: 'file2.txt' });
// append a file from buffer
const buffer3 = Buffer.from('buff it!');
archive.append(buffer3, { name: 'file3.txt' });
// append a file
archive.file('file1.txt', { name: 'file4.txt' });
// append files from a sub-directory and naming it `new-subdir` within the archive
archive.directory('subdir/', 'new-subdir');
// append files from a sub-directory, putting its contents at the root of archive
archive.directory('subdir/', false);
// append files from a glob pattern
archive.glob('file*.txt', {cwd:__dirname});
// finalize the archive (ie we are done appending files but streams have to finish yet)
// 'close', 'end' or 'finish' may be fired right after calling this method so register to them beforehand
archive.finalize();
Archiver ships with out of the box support for TAR and ZIP archives.
You can register additional formats with registerFormat
.
You can check if format already exists before to register a new one with isRegisteredFormat
.
FAQs
a streaming interface for archive generation
The npm package archiver receives a total of 3,790,537 weekly downloads. As such, archiver popularity was classified as popular.
We found that archiver demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.