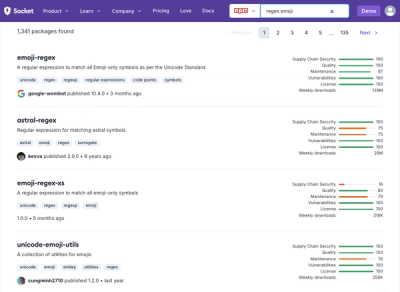
Security News
Weekly Downloads Now Available in npm Package Search Results
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
A timeout controller for asynchronous requests with defer.
areq is a timeout controller for asynchronous requests with Promise defers (e.g., Q.defer()). It tackles the event listener registering and timeout rejection for you.
$ npm install areq --save
var Q = require('q'),
Areq = require('areq'),
EventEmitter = require('events');
var myEmitter = new EventEmitter(),
areq = new Areq(myEmitter, 6000); // timeout after 6 seconds
var fooAsyncReq = function (callback) {
var deferred = Q.defer();
areq.register('some_event', deferred, function (result) {
if (result !== 'what_i_want') {
areq.reject('some_event', new Error('Bad response.'));
} else {
areq.resolve('some_event', result);
}
});
return deferred.promise.nodeify(callback);
};
fooAsyncReq(function (err, result) {
if (err)
console.log(err);
else
console.log(result);
});
Create an instance of Areq Class, which will be denoted as
areq
in this document.
Arguments
emitter
(EventEmitter): The emitter that emits the events for your listening to resolve the asynchronous responses.areqTimeout
(Number, optional): The default timeout in milliseconds. If elapsed time from the moment of a request sending out has reached this setting, the request will be rejected with a timeout error. If it is not given, a value of 30000 ms will be used as the default.Returns:
Example
var Areq = require('areq');
var areq = new Areq(foo_nwk_controller);
// foo_nwk_controller is your event emitter to dispatch messages from lower layer
Register an unique event to listen for the specific response coming from the emitter.
Arguments
evt
(String): The unique event according to the specific response.deferred
(Object): The defer object used in your method.listener
(Function): The event listener. With areq
, now you should use areq.resolve(evt, value)
and areq.reject(evt, err)
instead of using deferred.resolve(value)
and deferred.reject(err)
. areq.resolve()
and areq.reject()
will take care of the listener deregistering and timeout cleaning for you.time
(Number, optional): Register this areq with a timeout in milliseconds. A default value of 30000 will be used if not given.Returns:
Example
var myAreqMethod = function () {
var deferred = Q.defer(),
transId = my_nwk_controller.nextTransId(),
eventToListen = 'AF:incomingMsg:' + transId;
// event to listner maybe like this: AF:incomingMsg:172,
// where 172 is a unique transection id
areq.register(eventToListen, deferred, function (result) {
if (result !== 'what_i_want')
areq.reject(eventToListen, new Error('Bad response.'));
else
areq.resolve(eventToListen, result);
}, 10000); // if this reponse doesn't come back wihtin 10 secs,
// your myAreqMethod() will be rejected with a timeout error
return deferred.promise.nodeify(callback);
};
// now call your myAreqMethod() somewhere in the code
// (1) with thenable style
myAreqMethod().then(function (rsp) {
console.log(rsp);
}).fail(function (err) {
console.log(err);
}).done();
// (2) with err-back style
myAreqMethod(function (err, rsp) {
if (err)
console.log(err);
else
console.log(rsp);
});
Resolve the received response if the response is exactly that you need.
Arguments
evt
(String): The unique event according to the specific response.value
(Depends): The value to be resolved.Returns:
var myAreqMethod = function () {
var deferred = Q.defer(),
transId = my_nwk_controller.nextTransId(),
eventToListen = 'ZDO:incomingMsg:' + transId;
areq.register(eventToListen, deferred, function (rsp) {
if (rsp.status !== 0 && rsp.status !== 'SUCCESS')
areq.reject(eventToListen, new Error('Bad response.'));
else
areq.resolve(eventToListen, rsp);
}); // if this reponse doesn't come back wihtin default 30 secs,
// myAreqMethod() will be rejected with a timeout error
return deferred.promise.nodeify(callback);
};
// now call your myAreqMethod() somewhere in the code
myAreqMethod(function (err, rsp) {
if (err)
console.log(err);
else
console.log(rsp);
});
Reject the received response if the response is not what you need.
Arguments
evt
(String): The unique event according to the specific response.err
(Error): The reason why you reject this response.Returns:
Example
See the exmaple given with resolve() method.
Get record of the given event name. Returns undefined if not found.
Arguments
evt
(String): The unique event according to the specific response.Returns:
Example
areq.getRecord('AF:incomingMsg:6:11:162'); // { deferred: xxx, listener: yyy }
areq.getRecord('No_such_event_is_waiting'); // undefined
Checks if the event is pending. Usually, if you find someone is pending over there, it is suggested to change a new event to listen to. For example, get another transection id to make a new event name for your request.
Arguments
evt
(String): The unique event according to the specific response.Returns:
true
is the given event is pending, otherwise returns false
.Example
areq.isEventPending('AF:incomingMsg:6:11:161'); // true
areq.isEventPending('AF:incomingMsg:6:11:162'); // false
FAQs
A timeout controller for asynchronous requests with defer.
The npm package areq receives a total of 17 weekly downloads. As such, areq popularity was classified as not popular.
We found that areq demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
Security News
A Stanford study reveals 9.5% of engineers contribute almost nothing, costing tech $90B annually, with remote work fueling the rise of "ghost engineers."
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.