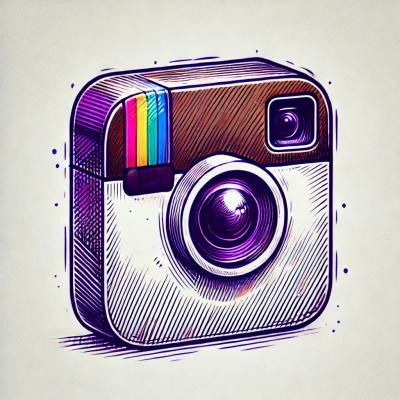
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
array-equal
Advanced tools
The array-equal npm package is a simple utility for checking if two arrays are equal. It performs a deep comparison to determine if the arrays contain the same elements in the same order.
Basic Array Equality Check
This feature allows you to check if two arrays are equal by comparing their elements in order. If all elements match, it returns true; otherwise, it returns false.
const arrayEqual = require('array-equal');
const arr1 = [1, 2, 3];
const arr2 = [1, 2, 3];
console.log(arrayEqual(arr1, arr2)); // true
Nested Array Equality Check
This feature allows you to check if two nested arrays are equal. It performs a deep comparison to ensure that all nested elements match.
const arrayEqual = require('array-equal');
const arr1 = [1, [2, 3], 4];
const arr2 = [1, [2, 3], 4];
console.log(arrayEqual(arr1, arr2)); // true
The deep-equal package provides a more comprehensive deep comparison between two values, including arrays, objects, and other data types. It is more versatile than array-equal as it can handle a wider range of comparisons.
The fast-deep-equal package is optimized for performance and provides a fast way to perform deep equality checks. It is similar to array-equal but is designed to be faster and more efficient.
The lodash.isequal function from the Lodash library provides a deep comparison between two values to determine if they are equivalent. It is part of the larger Lodash utility library, which offers a wide range of utility functions beyond equality checks.
Check if two arrays are equal
It checks that the elements and order are the same.
npm install array-equal
import arrayEqual from 'array-equal';
arrayEqual([1, 2, 3], [1, 2, 3]);
//=> true
arrayEqual([1, 2, 3], [1, 2, 3, 4]);
//=> false
FAQs
Check if two arrays are equal
The npm package array-equal receives a total of 2,700,950 weekly downloads. As such, array-equal popularity was classified as popular.
We found that array-equal demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.