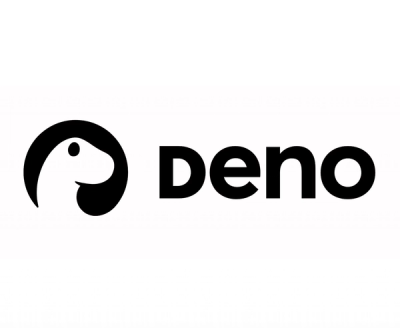
Security News
Deno 2.4 Brings Back deno bundle, Improves Dependency Management and Observability
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
array-mapcat
Advanced tools
Curried function deriving new array values by applying provided function to each item/index of provided array then applying `concat` to the results. Fast and compatible with modern or old browsers.
Curried function deriving new array values by applying provided function to each item/index of provided array then applying
concat
to the results. Fast and compatible with modern or old browsers.
npm install array-mapcat --save
You can also use Duo, Bower or download the files manually.
[].concat.apply([], [].map())
but in a more functional and compositionally friendly way.var mapcat = require('array-mapcat')
var splitword = (sentance) => sentance.split(/\s+/)
var sentences = [ 'two birds', 'three green peas' ]
mapcat(splitword, sentences)
//=> ['two', 'birds', 'three', 'green', 'peas']
var mapcat = require('array-mapcat')
var listmodel = [
{ list: [1, 2, 3] },
{ list: [4, 5, 6] },
{ list: [7, 8, 9] }
]
mapcat('list', listmodel)
//=> [1, 2, 3, 4, 5, 6, 7, 8, 9]
var mapcat = require('array-mapcat')
var listmodel = Promise.resolve([
{ list: [1, 2, 3] },
{ list: [4, 5, 6] },
{ list: [7, 8, 9] }
])
listmodel.then(mapcat('list'))
//=> [1, 2, 3, 4, 5, 6, 7, 8, 9]
mapcat(fn, list)
fn (Function|String)
Function to apply to each item.list (array)
Array to iterate over.(array)
Array resulting from applying provided function fn
to each item of list
then applying concat
to the results.SEE: contributing.md
0.1.3 - 2016-01-12
FAQs
Curried function deriving new array values by applying provided function to each item/index of provided array then applying `concat` to the results. Fast and compatible with modern or old browsers.
The npm package array-mapcat receives a total of 5 weekly downloads. As such, array-mapcat popularity was classified as not popular.
We found that array-mapcat demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.