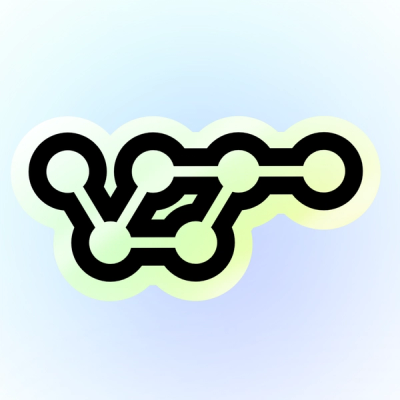
Security News
vlt Debuts New JavaScript Package Manager and Serverless Registry at NodeConf EU
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
array-tools
Advanced tools
Lightweight, use-anywhere toolkit for working with array data. 1.5k, compressed.
Lightweight, use-anywhere toolkit for working with array data. 1.5k, compressed.
> var a = require("array-tools");
There are four ways to use it.
> var remainder = a.without([ 1, 2, 3, 4, 5 ], 1)
> a.exists(remainder, 1)
false
> a([ 1, 2, 3, 4, 5 ]).without(1).exists(1);
false
var util = require("util");
var ArrayTools = require("array-tools");
// this class will inherit all array-tools methods
function CarCollection(cars){
ArrayTools.call(this, cars);
}
util.inherits(CarCollection, ArrayTools);
var cars = new CarCollection([
{ owner: "Me", model: "Citreon Xsara" },
{ owner: "Floyd", model: "Bugatti Veyron" }
]);
cars.findWhere({ owner: "Floyd" });
// returns { owner: "Floyd", model: "Bugatti Veyron" }
$ curl -s "https://api.github.com/users/75lb/repos?page=1&per_page=100" | array-tools pick full_name description
[
{
"full_name": "75lb/ansi-escape-sequences",
"description": "A simple library containing all known terminal ansi escape codes and sequences."
},
{
"full_name": "75lb/baldrick",
"description": "Your own private dogsbody. Does the shitty work you can't be arsed to do."
},
etc,
etc
]
Array
(e.g. where
, without
) can be chained.exists
, contains
) cannot be chained.Array.prototype
(e.g. .join
, .forEach
etc.) are also available in the chain. The same rules, regarding what can and cannot be chained, apply as above..val()
to terminate the chain and retrieve the output.> a([ 1, 2, 2, 3 ]).exists(1)
true
> a([ 1, 2, 2, 3 ]).without(1).exists(1)
false
> a([ 1, 2, 2, 3 ]).without(1).unique().val()
[ 2, 3 ]
> a([ 1, 2, 2, 3 ]).without(1).unique().join("-")
'2-3'
This library is tested in node versions 0.10, 0.11, 0.12, iojs and the following browsers:
As a library:
$ npm install array-tools --save
As a command-line tool:
$ npm install -g array-tools
Array
Array
Array
Array.<object>
Array
Array
Array
Array
Array
Array
Array
Array
boolean
object
*
*
Array
Takes any input and guarantees an array back.
arguments
) to a real arrayundefined
to an empty arraynull
) into an array containing that valueKind: static method of array-tools
Category: chainable
Param | Type | Description |
---|---|---|
any | * | the input value to convert to an array |
Example
> a.arrayify(undefined)
[]
> a.arrayify(null)
[ null ]
> a.arrayify(0)
[ 0 ]
> a.arrayify([ 1, 2 ])
[ 1, 2 ]
> function f(){ return a.arrayify(arguments); }
> f(1,2,3)
[ 1, 2, 3 ]
Array
Query a recordset, at any depth..
Kind: static method of array-tools
Category: chainable
Param | Type | Description |
---|---|---|
recordset | Array.<object> | the recordset to query |
query | query | the query definition |
Example
Say you have a recordset:
> data = [
{ name: "Dana", age: 30 },
{ name: "Yana", age: 20 },
{ name: "Zhana", age: 10 }
]
You can return records with properties matching an exact value:
> a.where(data, { age: 10 })
[ { name: 'Zhana', age: 10 } ]
All query expressions can be negated (where NOT the value). Prefix the property name with !
:
> a.where(data, { "!age": 10 })
[ { name: 'Dana', age: 30 }, { name: 'Yana', age: 20 } ]
match using a function:
> function over10(age){ return age > 10; }
> a.where(data, { age: over10 })
[ { name: 'Dana', age: 30 }, { name: 'Yana', age: 20 } ]
match using a regular expression
> a.where(data, { name: /ana/ })
[ { name: 'Dana', age: 30 },
{ name: 'Yana', age: 20 },
{ name: 'Zhana', age: 10 } ]
You can query to any arbitrary depth. So with deeper data, like:
> deepData = [
{ name: "Dana", favourite: { colour: "light red" } },
{ name: "Yana", favourite: { colour: "dark red" } },
{ name: "Zhana", favourite: { colour: [ "white", "red" ] } }
]
return records containing favourite.colour
values matching the regex /red/
> a.where(deepData, { favourite: { colour: /red/ } })
[ { name: 'Dana', favourite: { colour: 'light red' } },
{ name: 'Yana', favourite: { colour: 'dark red' } } ]
Prefix the property name with +
if the value you want to match could be singular or a member of an array.
> a.where(deepData, { favourite: { "+colour": /red/ } })
[ { name: 'Dana', favourite: { colour: 'light red' } },
{ name: 'Yana', favourite: { colour: 'dark red' } },
{ name: 'Zhana', favourite: { colour: [ "white", "red" ] } } ]
Array
Plucks the value of the specified property from each object in the input array
Kind: static method of array-tools
Category: chainable
Param | Type | Description |
---|---|---|
recordset | Array.<object> | The input recordset |
...property | string | Up to three property names - the first one found will be returned. |
Example
> var data = [
{ a: "Lionel", b: "Roger" },
{ a: "Luis", b: "Craig" },
{ b: "Peter" },
]
> a.pluck(data, "a")
[ 'Lionel', 'Luis' ]
> a.pluck(data, "a", "b")
[ 'Lionel', 'Luis', 'Peter' ]
Array.<object>
return a copy of the input recordset
containing objects having only the cherry-picked properties
Kind: static method of array-tools
Category: chainable
Param | Type | Description |
---|---|---|
recordset | Array.<object> | the input |
...property | string | the properties to include in the result |
Example
> data = [
{ name: "Dana", age: 30 },
{ name: "Yana", age: 20 },
{ name: "Zhana", age: 10 }
]
> a.pick(data, "name")
[ { name: 'Dana' }, { name: 'Yana' }, { name: 'Zhana' } ]
> a.pick(data, "name", "age")
[ { name: 'Dana', age: 30 },
{ name: 'Yana', age: 20 },
{ name: 'Zhana', age: 10 } ]
Array
Returns a new array with the same content as the input minus the specified values.
Kind: static method of array-tools
Category: chainable
Param | Type | Description |
---|---|---|
array | Array | the input array |
toRemove | * | a single, or array of values to omit |
Example
> a.without([ 1, 2, 3 ], 2)
[ 1, 3 ]
> a.without([ 1, 2, 3 ], [ 2, 3 ])
[ 1 ]
Array
merge two arrays into a single array of unique values
Kind: static method of array-tools
Category: chainable
Param | Type | Description |
---|---|---|
array1 | Array | First array |
array2 | Array | Second array |
idKey | string | the unique ID property name |
Example
> var array1 = [ 1, 2 ], array2 = [ 2, 3 ];
> a.union(array1, array2)
[ 1, 2, 3 ]
> var array1 = [ { id: 1 }, { id: 2 } ], array2 = [ { id: 2 }, { id: 3 } ];
> a.union(array1, array2)
[ { id: 1 }, { id: 2 }, { id: 3 } ]
> var array2 = [ { id: 2, blah: true }, { id: 3 } ]
> a.union(array1, array2)
[ { id: 1 },
{ id: 2 },
{ id: 2, blah: true },
{ id: 3 } ]
> a.union(array1, array2, "id")
[ { id: 1 }, { id: 2 }, { id: 3 } ]
Array
Returns the initial elements which both input arrays have in common
Kind: static method of array-tools
Category: chainable
Param | Type | Description |
---|---|---|
a | Array | first array to compare |
b | Array | second array to compare |
Example
> a.commonSequence([1,2,3], [1,2,4])
[ 1, 2 ]
Array
returns an array of unique values
Kind: static method of array-tools
Category: chainable
Param | Type | Description |
---|---|---|
array | Array | input array |
Example
> n = [1,6,6,7,1]
[ 1, 6, 6, 7, 1 ]
> a.unique(n)
[ 1, 6, 7 ]
Array
splice from index
until test
fails
Kind: static method of array-tools
Category: chainable
Param | Type | Description |
---|---|---|
array | Array | the input array |
index | number | the position to begin splicing from |
test | RegExp | the test to continue splicing while true |
...elementN | * | the elements to add to the array |
Example
> letters = ["a", "a", "b"]
[ 'a', 'a', 'b' ]
> a.spliceWhile(letters, 0, /a/, "x")
[ 'a', 'a' ]
> letters
[ 'x', 'b' ]
Array
Removes items from array
which satisfy the query. Modifies the input array, returns the extracted.
Kind: static method of array-tools
Returns: Array
- the extracted items.
Category: chainable
Param | Type | Description |
---|---|---|
array | Array | the input array, modified directly |
query | function | object | Per item in the array, if either the function returns truthy or the exists query is satisfied, the item is extracted |
Array
flatten an array of arrays into a single array
Kind: static method of array-tools
Category: chainable
Since: 1.4.0
Param | Type | Description |
---|---|---|
array | Array | the input array |
Example
> numbers = [ 1, 2, [ 3, 4 ], 5 ]
> a.flatten(numbers)
[ 1, 2, 3, 4, 5 ]
Array
Sort an array of objects by one or more fields
Kind: static method of array-tools
Category: chainable
Since: 1.5.0
Param | Type | Description |
---|---|---|
recordset | Array.<object> | input array |
columns | string | Array.<string> | column name(s) to sort by |
customOrder | object | specific sort orders, per columns |
Example
> var fixture = [
{ a: 4, b: 1, c: 1},
{ a: 4, b: 3, c: 1},
{ a: 2, b: 2, c: 3},
{ a: 2, b: 2, c: 2},
{ a: 1, b: 3, c: 4},
{ a: 1, b: 1, c: 4},
{ a: 1, b: 2, c: 4},
{ a: 3, b: 3, c: 3},
{ a: 4, b: 3, c: 1}
];
> a.sortBy(fixture, ["a", "b", "c"])
[ { a: 1, b: 1, c: 4 },
{ a: 1, b: 2, c: 4 },
{ a: 1, b: 3, c: 4 },
{ a: 2, b: 2, c: 2 },
{ a: 2, b: 2, c: 3 },
{ a: 3, b: 3, c: 3 },
{ a: 4, b: 1, c: 1 },
{ a: 4, b: 3, c: 1 },
{ a: 4, b: 3, c: 1 } ]
boolean
returns true if a value, or nested object value exists in an array.. If value is a plain object, it is considered to be a query. If value
is a plain object and you want to search for it by reference, use .contains
.
Kind: static method of array-tools
Category: not chainable
Param | Type | Description |
---|---|---|
array | Array | the array to search |
value | * | the value to search for |
Example
> a.exists([ 1, 2, 3 ], 2)
true
> a.exists([ 1, 2, 3 ], [ 2, 3 ])
true
> a.exists([ { result: false }, { result: false } ], { result: true })
false
> a.exists([ { result: true }, { result: false } ], { result: true })
> a.exists([ { n: 1 }, { n: 2 }, { n: 3 } ], [ { n: 1 }, { n: 3 } ])
true
object
returns the first item from recordset
where key/value pairs
from query
are matched identically
Kind: static method of array-tools
Category: not chainable
Param | Type | Description |
---|---|---|
recordset | Array.<object> | the array to search |
query | object | an object containing the key/value pairs you want to match |
Example
> dudes = [{ name: "Jim", age: 8}, { name: "Clive", age: 8}, { name: "Hater", age: 9}]
[ { name: 'Jim', age: 8 },
{ name: 'Clive', age: 8 },
{ name: 'Hater', age: 9 } ]
> a.findWhere(dudes, { age: 8})
{ name: 'Jim', age: 8 }
*
Return the last item in an array.
Kind: static method of array-tools
Category: not chainable
Since: 1.7.0
Param | Type | Description |
---|---|---|
arr | Array | the input array |
*
Kind: static method of array-tools
Category: not chainable
Since: 1.8.0
Param | Type | Description |
---|---|---|
arr | Array | the input array |
toRemove | * | the item to remove |
Searches the array for the exact value supplied (strict equality). To query for value existance using an expression or function, use exists.
Kind: static method of array-tools
Returns: boolean
Category: not chainable
Since: 1.8.0
Param | Type | Description |
---|---|---|
arr | Array | the input array |
value | * | the value to look for |
© 2015 Lloyd Brookes 75pound@gmail.com. Documented by jsdoc-to-markdown.
FAQs
Lightweight, use-anywhere toolkit for working with array data.
We found that array-tools demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
Security News
Research
The Socket Research Team uncovered a malicious Python package typosquatting the popular 'fabric' SSH library, silently exfiltrating AWS credentials from unsuspecting developers.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.