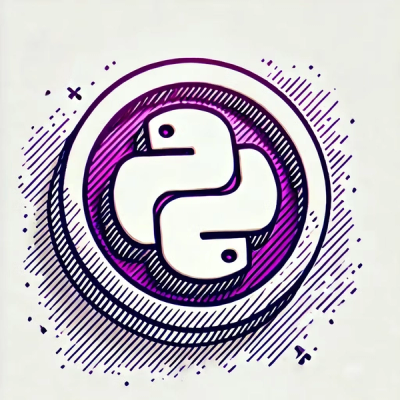
Product
Socket Now Supports pylock.toml Files
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
array-treeify
Advanced tools
array-treeify
Simple text trees from arrays using Unicode box-drawing characters. For your terminal and console displays.
array-treeify
transforms nested arrays into text trees with proper branching characters. Perfect for CLIs, debug outputs, or anywhere you need to visualize hierarchical data.
treeify([
'Lumon Industries',
[
'Board of Directors',
['Natalie (Representative)'],
'Departments',
[
'Macrodata Refinement (Cobel)',
['Milchick', 'Mark S.', ['Dylan G.', 'Irving B.', 'Helly R.']],
],
'Other Departments',
[
'Optics & Design',
'Wellness Center',
'Mammalians Nurturable',
'Choreography and Merriment',
],
],
])
Lumon Industries
ββ Board of Directors
β ββ Natalie (Representative)
ββ Departments
β ββ Macrodata Refinement (Cobel)
β ββ Milchick
β ββ Mark S.
β ββ Dylan G.
β ββ Irving B.
β ββ Helly R.
ββ Other Departments
ββ Optics & Design
ββ Wellness Center
ββ Mammalians Nurturable
ββ Choreography and Merriment
npm install array-treeify
function treeify(input: TreeInput, options?: {
chars?: TreeChars, // Custom characters for the tree
plain?: boolean // Use plain whitespace instead of Unicode box-drawing characters
}): string
array-treeify
accepts a simple, intuitive array structure that's easy to build and manipulate:
import {treeify} from 'array-treeify'
// Basic example
const eagan = [
'Kier Eagan',
[
'...',
[
'...',
'Jame Eagan',
['Helena Eagan']
],
'Ambrose Eagan',
],
]
console.log(treeify(eagan))
/*
Kier Eagan
ββ ...
β ββ ...
β ββ Jame Eagan
β ββ Helena Eagan
ββ Ambrose Eagan
*/
// Using custom characters
const resultCustomChars = treeify(
eagan,
{ chars: { branch: 'ββ’ ', lastBranch: 'ββ’ ', pipe: 'β ', space: ' ' },
})
/*
Kier Eagan
ββ’ ...
β ββ’ ...
β ββ’ Jame Eagan
β ββ’ Helena Eagan
ββ’ Ambrose Eagan
*/
// Using plain whitespace characters
console.log(treeify(eagan, { plain: true }))
/*
Kier Eagan
...
...
Jame Eagan
Helena Eagan
Ambrose Eagan
*/
// Nested example
const orgChart = [
'Lumon Industries',
[
'Board of Directors',
['Natalie (Representative)'],
'Department Heads',
[
'Cobel (MDR)',
['Milchick', 'Mark S.', ['Dylan G.', 'Irving B.', 'Helly R.']]
]
]
]
console.log(treeify(orgChart))
/*
Lumon Industries
ββ Board of Directors
β ββ Natalie (Representative)
ββ Department Heads
ββ Cobel (MDR)
ββ Milchick
ββ Mark S.
ββ Dylan G.
ββ Irving B.
ββ Helly R.
*/
Disclaimer: The exported
TreeInput
type (Array<string | TreeInput>
) is intentionally flexible to support dynamic and programmatic tree construction. However, TypeScript cannot enforce at the type level that the first element is a string. This requirement is checked at runtime by thetreeify
function, which will throw an error if the first element is not a string. Please ensure your input arrays follow this convention.
The treeify
function accepts arrays with the following structure:
['root', 'sibling', ['child1', 'child2']] // Root with 2 children
['root', ['child'], 'sibling', ['nephew', 'niece']] // 2 root nodes with children
['root', ['child', ['grandchild']]] // Grandchildren
chars
: Custom characters for the tree. Defaults to Unicode box-drawing characters.plain
: When true, uses plain whitespace characters instead of Unicode box-drawing characters.MIT Β© tbeseda
FAQs
Simple text tree diagrams from arrays.
The npm package array-treeify receives a total of 75,793 weekly downloads. As such, array-treeify popularity was classified as popular.
We found that array-treeify demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.
Research
Security News
Malicious Ruby gems typosquat Fastlane plugins to steal Telegram bot tokens, messages, and files, exploiting demand after Vietnamβs Telegram ban.