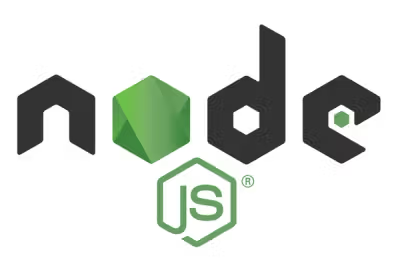
Security News
require(esm) Backported to Node.js 20, Paving the Way for ESM-Only Packages
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
arrow-orm
Advanced tools
API Builder ORM
Object relational mapping (ORM) framework for API Builder.
There are 4 main components to the ORM framework:
To define a model, you must give a set of fields and a connector.
var User = orm.Model.extend('user',{
fields: {
name: {
type: String,
default: 'Jeff',
}
},
connector: Connector
});
The first argument is the name of the model. The second argument is the definition of the model.
The definition of the model is an object with the following properties.
Name | Type | Description |
---|---|---|
actions | string[] | An array of method actions that are enabled for the model. If empty, all are enabled. |
autogen | boolean | A boolean to indicate the model was auto-generated. |
cache | boolean | Enables a LRU cache. |
connector | string | object | Identifies the connector to which this model belongs, required. |
countDescription | string | Changes the description for the method count . |
createDescription | string | Changes the description for the method create . |
deleteAllDescription | string | Changes the description for the method deleteAll . |
deleteDescription | string | Changes the description for the method delete . |
disabledActions | string[] | An array of actions that are disabled for the model. |
distinctDescription | string | Changes the description for the method distinct . |
fields | object | A collection of property fields belonging to the model. |
findAllDescription | string | Changes the description for the method findAll . |
findAndModifyDescription | string | Changes the description for the method findAndModify . |
findByIDDescription | string | Changes the description for the method findByID . |
findManyByIDDescription | string | Changes the description for the method findManyByID . |
metadata | object | Additional metadata about the model. |
plural | string | The plural name of the model. |
queryDescription | string | Changes the description for the method query . |
singular | string | The singular name of the model. |
updateDescription | string | Changes the description for the method update . |
upsertDescription | string | Changes the description for the method upsert . |
The fields
are a collection of properties where each key uniquely identifies the field, and each field has a set of properties that define it:
Name | Type | Description |
---|---|---|
custom | boolean | Indicates the field's value is custom and should be omitted when converting to payload (e.g. JSON). |
default | any | The default value. |
description | string | The description of the field. |
length | integer | For Strings and Arrays, the precise length of the field. |
limit | integer | In composite models where the field is joined with another and the type is "array", then this property limits the amount of records returned. |
maxlength | integer | For Strings and Arrays, the maximum length of the field. |
minlength | integer | For Strings and Arrays, the minimum length of the field. |
model | string | The name of the model from which this field originates (in composite models). |
name | string | The name of the field. |
optional | boolean | The field is optional (deprecated). |
readonly | boolean | The field is read-only. |
required | boolean | If true, the field is required. |
type | any | The column type, supporting values: string, date, number, array, object, and boolean; required. |
The metadata
is used to encode additional data about the Model that can be used for composite models or primary keys.
Name | Type | Description |
---|---|---|
primarykey | string | The field name that is identified as the primary key. The field cannot be a property of fields. |
primaryKeyDetails | object | An object that describes additional details about the primary key. |
table | string | In some connectors, this refers to the table name for the Model. |
schema | string | In some connectors, this refers to the table schema name or owner of the table for the Model. |
Name | Type | Description |
---|---|---|
autogenerated | boolean | Indicates the primary key is auto-generated. |
dataType | string | In some connectors, this describes the persisted datatype of the primary key. |
type | string | In some connectors, this describes the field type for the primary key, supporting values: string, date, number. |
The model has several instance methods:
Name | Description |
---|---|
count | Find count of a query. |
create | Create a new Model instance. |
delete | Deletes a Model instance. |
deleteAll | Removes all Model instances. |
distinct | Find unique values for a Model field name. |
extend | Create a new Model class from the current Model. |
find | Find one or more Models. |
findAll | Find all Models. |
findAndModify | Find a Model from a query and modify values. |
findByID | Find one Model by a primary key. |
findManyByID | Find many Models by a primary key. |
query | Find a Model from a query. |
update | Updates a Model instance. |
upsert | Updates or inserts a Model instance. |
A model can have custom functions by defining them in the definition as a property. They will automatically be available on the model instance.
var User = orm.Model.extend('user',{
fields: {
name: {
type: String,
required: true,
default: 'jeff'
}
},
connector: Connector,
// implement a function that will be on the Model and
// available to all instances
getProperName: function() {
// this points to the instance when this is invoked
return this.name.charAt(0).toUpperCase() + this.name.substring(1);
}
});
User.create(function(err,user){
console.log(user.getProperName());
});
One you've defined a model, you can then use it to create an Instance of the Model.
User.create({name:'Nolan'}, function(err,user){
// you now have a user instance
});
Instances has several methods for dealing with the model.
Name | Description |
---|---|
get | get the value of a field property |
set | set a value or a set of values (Object) |
isUnsaved | returns true if the instance has pending changes |
isDeleted | returns true if the instance has been deleted and cannot be used |
update | save any pending changes |
remove | remove this instance (delete it) |
getPrimaryKey | return the primary key value set by the Connector |
In addition to get
and set
, you can also use property accessors to get field values.
console.log('name is',user.name);
user.name = 'Rick';
If the Connector returns more than one Model instance, it will return it as a Collection, which is a container of Model instances.
A collection is an array and has additional helper functions for manipulating the collection.
You can get the length of the collection with the length
property.
To create a connector, you can either inherit from the Connector
class using utils.inherit
or extend:
var MyConnector = orm.Connector.extend({
constructor: function(){
},
findByID: function(Model, id, callback) {
}
});
Once you have created a Connector class, you can create a new instance:
var connector = new MyConnector({
url: 'http://foobar.com'
});
This code is proprietary, closed source software licensed to you by Axway. All Rights Reserved. You may not modify Axway’s code without express written permission of Axway. You are licensed to use and distribute your services developed with the use of this software and dependencies, including distributing reasonable and appropriate portions of the Axway code and dependencies. Except as set forth above, this code MUST not be copied or otherwise redistributed without express written permission of Axway. This module is licensed as part of the Axway Platform and governed under the terms of the Axway license agreement (General Conditions) located here: https://support.axway.com/en/auth/general-conditions; EXCEPT THAT IF YOU RECEIVED A FREE SUBSCRIPTION, LICENSE, OR SUPPORT SUBSCRIPTION FOR THIS CODE, NOTWITHSTANDING THE LANGUAGE OF THE GENERAL CONDITIONS, AXWAY HEREBY DISCLAIMS ALL SUPPORT AND MAINTENANCE OBLIGATIONS, AS WELL AS ALL EXPRESS AND IMPLIED WARRANTIES, INCLUDING BUT NOT LIMITED TO IMPLIED INFRINGEMENT WARRANTIES, WARRANTIES OF MERCHANTABILITY OR FITNESS FOR A PARTICULAR PURPOSE, AND YOU ACCEPT THE PRODUCT AS-IS AND WITH ALL FAULTS, SOLELY AT YOUR OWN RISK. Your right to use this software is strictly limited to the term (if any) of the license or subscription originally granted to you.
FAQs
API Builder ORM
The npm package arrow-orm receives a total of 96 weekly downloads. As such, arrow-orm popularity was classified as not popular.
We found that arrow-orm demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 20 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
Security News
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.