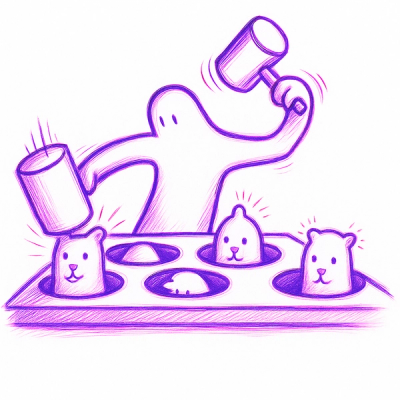
Research
/Security News
Contagious Interview Campaign Escalates With 67 Malicious npm Packages and New Malware Loader
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.
async-array-methods
Advanced tools
Async methods to manipulate arrays.
var methods = require('async-array-methods')
var AsyncArray = methods.Array
var filter = methods.filter
var map = methods.map
var forEach = methods.forEach
var reduce = methods.reduce
All methods return a promise.
Callbacks can be made asynchronous by returning a promise, or accepting a function argument, which will be called when the callback finishes.
Otherwise, the callback is treated as synchronous.
Signature: filter(arr, fn, context)
filter(
[1, 2, 3, 4],
function (v, i, a, next) {
process.nextTick(function () {
next(null, v % 2)
})
}
)
.then(function (res) {
// [1, 3]
console.log(res)
})
Signature: map(arr, fn, context)
fn
is called with each element in parallel.
map(
[1, 2, 3, 4],
function (v) {
return new Promise(function (rs) {
process.nextTick(function () {
rs(v << 2)
})
})
},
{ num: 2 }
)
// [4, 8, 12, 16]
.then(console.log.bind(console))
This method works like map
,
except that fn
is called with each element in sequence rather than in parallel.
Signature: map(arr, fn, context)
var n = 1
series(
[1, 2, 3, 4],
function (v, i, arr, next) {
var delay = rand()
console.log('i:', i, 'delay:', delay)
setTimeout(function() {
next(null, v << n++)
}, delay)
}
)
// i: 0 delay: 10
// i: 1 delay: 50
// i: 2 delay: 0
// i: 3 delay: 80
// [2, 8, 24, 64]
.then(log)
function rand() {
return Math.floor(Math.random() * 10) * 10
}
fn
is called with elements in sequence.
Signature: forEach(arr, fn, context)
var count = 0
forEach(
[1, 2, 3, 4],
function (v, i, _, next) {
process.nextTick(function () {
console.log(count++, i)
next(null, v << 2)
})
}
)
.then(function (arr) {
// [1, 2, 3, 4]
console.log(arr)
})
Signature: reduce(arr, fn, initial)
reduce(
[1, 2, 3, 4],
function (a, b, i, arr, next) {
process.nextTick(function () {
next(null, a + b)
})
}
)
// 10
.then(console.log.bind(console))
Signature: AsyncArray(arr)
Methods:
map
series
filter
forEach
reduce
var origin = AsyncArray([1, 2, 3, 4, 5, 6])
var odd = origin.filter(isOdd)
var even = origin.filter(isEven)
return odd.then(function (res) {
// [1, 3, 5]
console.log(res)
return even
})
.then(function (res) {
// [2, 4, 6]
console.log(res)
})
.then(function () {
return even.reduce(flagMap, {})
})
.then(function (res) {
// { 2: true, 4: true, 6: true }
console.log(res)
})
.then(function () {
// chain
return origin.filter(isOdd).reduce(flagMap, {})
})
.then(function (res) {
// { 1: true, 3: true, 5: true }
console.log(res)
})
function isOdd(n, i, arr, next) {
process.nextTick(function () {
next(null, n % 2)
})
}
function isEven(n, i, arr, next) {
return new Promise(function (resolve) {
process.nextTick(function () {
resolve((n + 1) % 2)
})
})
}
function flagMap(o, v) {
o[v] = true
return o
}
FAQs
Async methods to operate on collections
The npm package async-array-methods receives a total of 58 weekly downloads. As such, async-array-methods popularity was classified as not popular.
We found that async-array-methods demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.